Description
Base class to set up a Chrono::FSI problem.
#include <ChFsiProblem.h>
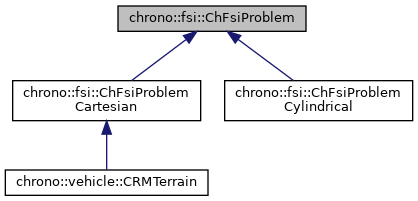
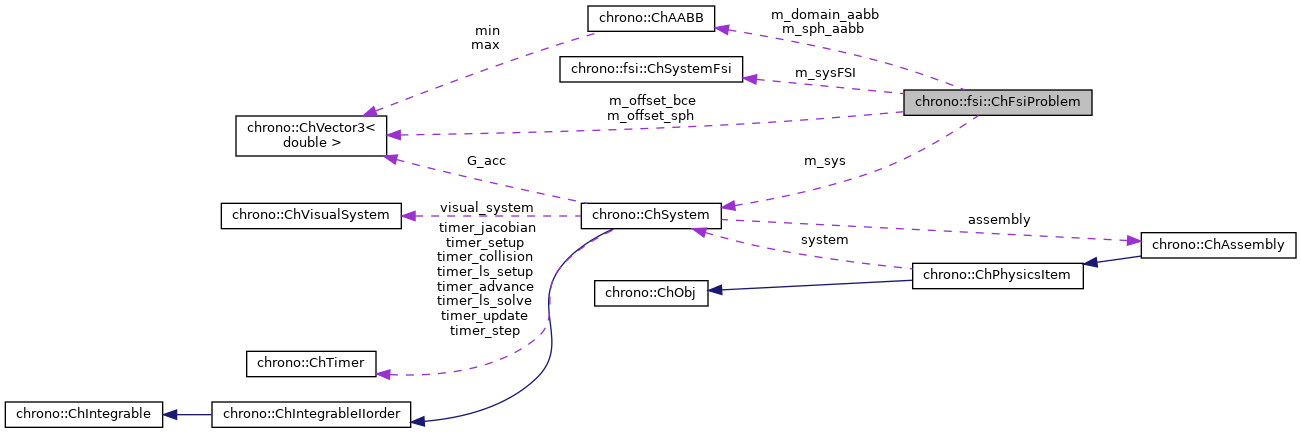
Classes | |
struct | CoordHash |
Hash function for a 3D integer grid coordinate. More... | |
class | ParticlePropertiesCallback |
Interface for callback to set initial particle pressure, density, viscosity, and velocity. More... | |
struct | RigidBody |
Specification of an FSI rigid body. More... | |
Public Types | |
enum | WavemakerType { PISTON, FLAP } |
Wave generator types. | |
Public Member Functions | |
void | SetVerbose (bool verbose) |
Enable verbose output during construction of ChFsiProblem (default: false). | |
ChSystem & | GetSystyemMBS () |
Access the underlying MBS system. | |
ChSystemFsi & | GetSystemFSI () |
Access the underlying FSI system. | |
size_t | AddRigidBody (std::shared_ptr< ChBody > body, const chrono::utils::ChBodyGeometry &geometry, bool check_embedded, bool use_grid_bce=false) |
Add a rigid body to the FSI problem. More... | |
size_t | AddRigidBodySphere (std::shared_ptr< ChBody > body, const ChVector3d &pos, double radius, bool use_grid_bce=false) |
size_t | AddRigidBodyBox (std::shared_ptr< ChBody > body, const ChFramed &pos, const ChVector3d &size) |
size_t | AddRigidBodyCylinderX (std::shared_ptr< ChBody > body, const ChFramed &pos, double radius, double length, bool use_grid_bce=false) |
size_t | AddRigidBodyMesh (std::shared_ptr< ChBody > body, const ChVector3d &pos, const std::string &obj_file, const ChVector3d &interior_point, double scale) |
void | RegisterParticlePropertiesCallback (std::shared_ptr< ParticlePropertiesCallback > callback) |
Register a callback for setting SPH particle initial properties. | |
void | SetComputationalDomainSize (ChAABB aabb) |
Explicitly set the computational domain limits. More... | |
void | Initialize () |
Complete construction of the FSI problem and initialize the FSI system. More... | |
std::shared_ptr< ChBody > | GetGroundBody () const |
Get the ground body. | |
size_t | GetNumSPHParticles () const |
Get number of SPH particles. | |
size_t | GetNumBoundaryBCEMarkers () const |
Get number of boundary BCE markers. | |
const ChAABB & | GetComputationalDomainSize () const |
Get limits of computational domain. | |
const ChAABB & | GetSPHBoundingBox () const |
Get limits of SPH volume. | |
const ChVector3d & | GetFsiBodyForce (std::shared_ptr< ChBody > body) const |
Return the FSI applied force on the specified body (as returned by AddRigidBody). More... | |
const ChVector3d & | GetFsiBodyTorque (std::shared_ptr< ChBody > body) const |
Return the FSI applied torque on on the specified body (as returned by AddRigidBody). More... | |
void | SaveInitialMarkers (const std::string &out_dir) const |
Save the set of initial SPH and BCE grid locations to files in the specified output directory. | |
Protected Types | |
typedef std::unordered_set< ChVector3i, CoordHash > | GridPoints |
typedef std::vector< ChVector3d > | RealPoints |
Protected Member Functions | |
ChFsiProblem (ChSystem &sys, double spacing) | |
Create a ChFsiProblem object. More... | |
virtual ChVector3i | Snap2Grid (const ChVector3d &point)=0 |
virtual ChVector3d | Grid2Point (const ChVector3i &p)=0 |
void | ProcessBody (RigidBody &b) |
Prune SPH markers that are inside the solid body volume. More... | |
int | ProcessBodyMesh (RigidBody &b, ChTriangleMeshConnected trimesh, const ChVector3d &interior_point) |
Prune SPH markers that are inside a body mesh volume. More... | |
Protected Attributes | |
ChSystemFsi | m_sysFSI |
underlying Chrono FSI system | |
ChSystem & | m_sys |
associated Chrono MBS system | |
double | m_spacing |
particle and marker spacing | |
std::shared_ptr< ChBody > | m_ground |
ground body | |
GridPoints | m_sph |
SPH particle grid locations. | |
GridPoints | m_bce |
boundary BCE marker grid locations | |
ChVector3d | m_offset_sph |
SPH particles offset. | |
ChVector3d | m_offset_bce |
boundary BCE particles offset | |
ChAABB | m_domain_aabb |
computational domain bounding box | |
ChAABB | m_sph_aabb |
SPH volume bounding box. | |
std::vector< RigidBody > | m_bodies |
list of FSI rigid bodies | |
std::unordered_map< std::shared_ptr< ChBody >, size_t > | m_fsi_bodies |
std::shared_ptr< ParticlePropertiesCallback > | m_props_cb |
callback for particle properties | |
bool | m_verbose |
if true, write information to standard output | |
bool | m_initialized |
set to 'true' once terrain is initialized | |
Constructor & Destructor Documentation
◆ ChFsiProblem()
|
protected |
Create a ChFsiProblem object.
No SPH parameters are set.
Member Function Documentation
◆ AddRigidBody()
size_t chrono::fsi::ChFsiProblem::AddRigidBody | ( | std::shared_ptr< ChBody > | body, |
const chrono::utils::ChBodyGeometry & | geometry, | ||
bool | check_embedded, | ||
bool | use_grid_bce = false |
||
) |
Add a rigid body to the FSI problem.
BCE markers are created for the provided geometry (which may or may not match the body collision geometry). By default, where applicable, BCE markers are created using polar coordinates (in layers starting from the shape surface). Generation of BCE markers on a uniform Cartesian grid can be enforced setting use_grid_bce=true. Creation of FSI bodies embedded in the fluid phase is allowed (SPH markers inside the body geometry volume are pruned). To check for possible overlap with SPH particles, set 'check_embedded=true'. This function must be called before Initialize().
◆ GetFsiBodyForce()
const ChVector3d & chrono::fsi::ChFsiProblem::GetFsiBodyForce | ( | std::shared_ptr< ChBody > | body | ) | const |
Return the FSI applied force on the specified body (as returned by AddRigidBody).
The force is applied at the body COM and is expressed in the absolute frame. An exception is thrown if the given body was not added through AddRigidBody.
◆ GetFsiBodyTorque()
const ChVector3d & chrono::fsi::ChFsiProblem::GetFsiBodyTorque | ( | std::shared_ptr< ChBody > | body | ) | const |
Return the FSI applied torque on on the specified body (as returned by AddRigidBody).
The torque is expressed in the absolute frame. An exception is thrown if the given body was not added through AddRigidBody.
◆ Initialize()
void chrono::fsi::ChFsiProblem::Initialize | ( | ) |
Complete construction of the FSI problem and initialize the FSI system.
After this call, no additional solid bodies should be added to the FSI problem.
◆ ProcessBody()
|
protected |
Prune SPH markers that are inside the solid body volume.
Treat separately primitive shapes (use explicit test for interior points) and mesh shapes (use ProcessBodyMesh).
◆ ProcessBodyMesh()
|
protected |
Prune SPH markers that are inside a body mesh volume.
Voxelize the body mesh (at the scaling resolution) and identify grid nodes inside the boundary defined by the body BCEs. Note that this assumes the BCE markers form a watertight boundary.
◆ SetComputationalDomainSize()
|
inline |
Explicitly set the computational domain limits.
By default, this is set so that it encompasses all SPH particles and BCE markers.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_fsi/ChFsiProblem.h
- /builds/uwsbel/chrono/src/chrono_fsi/ChFsiProblem.cpp