Description
Custom FSI interface between a Chrono multibody system and the SPH-based fluid system.
This custom FSI interface is paired with ChFluidSystemSPH and provides a more efficient coupling with a Chrono MBS that a generic FSI interface does, because it works directly with the data structures of ChFluidSystemSPH.
#include <ChFsiInterfaceSPH.h>
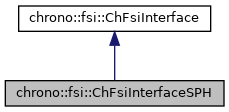
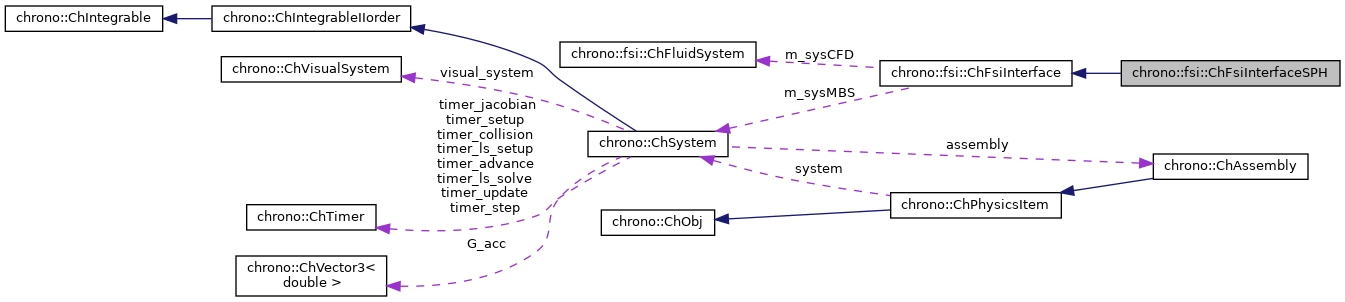
Public Member Functions | |
ChFsiInterfaceSPH (ChSystem &sysMBS, ChFluidSystemSPH &sysSPH) | |
virtual void | ExchangeSolidStates () override |
Exchange solid phase state information between the MBS and fluid system. More... | |
virtual void | ExchangeSolidForces () override |
Exchange solid phase force information between the multibody and fluid systems. More... | |
![]() | |
void | SetVerbose (bool verbose) |
FsiBody & | AddFsiBody (std::shared_ptr< ChBody > body) |
Add a rigid body. | |
FsiMesh1D & | AddFsiMesh1D (std::shared_ptr< fea::ChContactSurfaceSegmentSet > surface) |
Add a flexible solid with segment set contact to the FSI system. | |
FsiMesh2D & | AddFsiMesh2D (std::shared_ptr< fea::ChContactSurfaceMesh > surface) |
Add a flexible solid with surface mesh contact to the FSI system. | |
virtual void | Initialize () |
Initialize the FSI interface. | |
unsigned int | GetNumBodies () const |
Get the number of FSI bodies. | |
unsigned int | GetNumMeshes1D () const |
Get the number of FSI 1-D meshes. | |
unsigned int | GetNumElements1D () const |
Get the number of FSI 1-D mesh elements (segments). | |
unsigned int | GetNumNodes1D () const |
Get the number of FSI 1-D mesh nodes. | |
unsigned int | GetNumMeshes2D () const |
Get the number of FSI 2-D meshes. | |
unsigned int | GetNumElements2D () const |
Get the number of FSI 2-D mesh elements (segments). | |
unsigned int | GetNumNodes2D () const |
Get the number of FSI 2-D mesh nodes. | |
const ChVector3d & | GetFsiBodyForce (size_t i) const |
Return the FSI applied force on the body with specified index. More... | |
const ChVector3d & | GetFsiBodyTorque (size_t i) const |
Return the FSI applied torque on the body with specified index. More... | |
void | AllocateStateVectors (std::vector< FsiBodyState > &body_states, std::vector< FsiMeshState > &mesh1D_states, std::vector< FsiMeshState > &mesh2D_states) const |
Utility function to allocate state vectors. | |
void | AllocateForceVectors (std::vector< FsiBodyForce > &body_forces, std::vector< FsiMeshForce > &mesh_forces1D, std::vector< FsiMeshForce > &mesh_forces2D) const |
Utility function to allocate force vectors. | |
bool | CheckStateVectors (const std::vector< FsiBodyState > &body_states, const std::vector< FsiMeshState > &mesh1D_states, const std::vector< FsiMeshState > &mesh2D_states) const |
Utility function to check sizes of state vectors. | |
bool | CheckForceVectors (const std::vector< FsiBodyForce > &body_forces, const std::vector< FsiMeshForce > &mesh_forces1D, const std::vector< FsiMeshForce > &mesh_forces2D) const |
Utility function to check sizes of force vectors. | |
void | StoreSolidStates (std::vector< FsiBodyState > &body_states, std::vector< FsiMeshState > &mesh1D_states, std::vector< FsiMeshState > &mesh2D_states) |
Utility function to get current solid phase states from the multibody system in the provided structures. More... | |
void | LoadSolidForces (std::vector< FsiBodyForce > &body_forces, std::vector< FsiMeshForce > &mesh1D_forces, std::vector< FsiMeshForce > &mesh2D_forces) |
Utility function to apply forces in the provided structures to the multibody system. More... | |
Additional Inherited Members | |
![]() | |
ChFsiInterface (ChSystem &sysMBS, ChFluidSystem &sysCFD) | |
![]() | |
bool | m_verbose |
ChSystem & | m_sysMBS |
ChFluidSystem & | m_sysCFD |
std::vector< FsiBody > | m_fsi_bodies |
rigid bodies exposed to the FSI system | |
std::vector< FsiMesh1D > | m_fsi_meshes1D |
FEA meshes with 1-D segments exposed to the FSI system. | |
std::vector< FsiMesh2D > | m_fsi_meshes2D |
FEA meshes with 2-D faces exposed to the FSI system. | |
Member Function Documentation
◆ ExchangeSolidForces()
|
overridevirtual |
Exchange solid phase force information between the multibody and fluid systems.
Transfer rigid and flex forces from the SPH data manager on the GPU to the host, then apply fluid forces and torques as external loads in the MBS. Directly access SPH data manager.
Implements chrono::fsi::ChFsiInterface.
◆ ExchangeSolidStates()
|
overridevirtual |
Exchange solid phase state information between the MBS and fluid system.
Extract FSI body states and mesh node states from the MBS, copy to the SPH data manager on the host, and then transfer to GPU memory. Directly access SPH data manager.
Implements chrono::fsi::ChFsiInterface.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_fsi/sph/ChFsiInterfaceSPH.h
- /builds/uwsbel/chrono/src/chrono_fsi/sph/ChFsiInterfaceSPH.cpp