chrono::fsi::ChFsiInterface Class Reference
Description
Base class for processing the interface between Chrono and FSI modules.
#include <ChFsiInterface.h>
Inheritance diagram for chrono::fsi::ChFsiInterface:
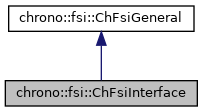
Collaboration diagram for chrono::fsi::ChFsiInterface:
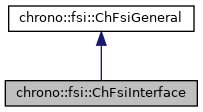
Public Member Functions | |
ChFsiInterface (ChSystemFsi_impl &fsi, std::shared_ptr< SimParams > params) | |
Constructor of the FSI interface class. | |
~ChFsiInterface () | |
Destructor of the FSI interface class. | |
void | LoadBodyState_Chrono2Fsi (std::shared_ptr< FsiBodyStateD > fsiBodyStateD) |
Copy rigid body states from ChSystem to FsiSystem, then to the GPU memory. | |
void | LoadMesh1DState_Chrono2Fsi (std::shared_ptr< FsiMeshStateD > fsiMesh1DState_D) |
Copy FEA mesh states from ChSystem to FsiSystem, then to the GPU memory. | |
void | LoadMesh2DState_Chrono2Fsi (std::shared_ptr< FsiMeshStateD > fsiMesh2DState_D) |
void | ApplyBodyForce_Fsi2Chrono () |
Read the surface-integrated pressure and viscous forces form the fluid/granular dynamics system, and add these forces and torques as external forces to the ChSystem rigid bodies. | |
void | ApplyMesh1DForce_Fsi2Chrono () |
Add forces and torques as external forces to the ChSystem flexible bodies. | |
void | ApplyMesh2DForce_Fsi2Chrono () |
![]() | |
ChFsiBase (std::shared_ptr< SimParams > params, std::shared_ptr< ChCounters > numObjects) | |
Constructor for the ChFsiBase class. More... | |
virtual | ~ChFsiBase () |
Destructor of the ChFsiBase class. | |
Friends | |
class | ChSystemFsi |
Additional Inherited Members | |
![]() | |
static void | computeGridSize (uint n, uint blockSize, uint &numBlocks, uint &numThreads) |
Compute number of blocks and threads for calculation on GPU. More... | |
![]() | |
std::shared_ptr< SimParams > | paramsH |
simulation parameters (host) | |
std::shared_ptr< ChCounters > | numObjectsH |
problem counters (host) | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_fsi/physics/ChFsiInterface.h
- /builds/uwsbel/chrono/src/chrono_fsi/physics/ChFsiInterface.cpp