Description
Simple finite element with two nodes and a spring/damper between the two nodes.
This element is mass-less, so if used in dynamic analysis, the two nodes must be set with non-zero point mass.
#include <ChElementSpring.h>
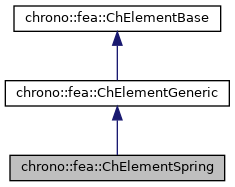
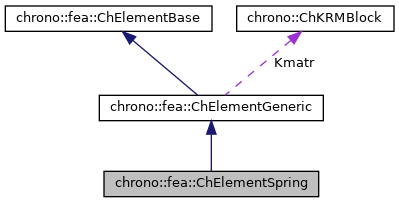
Public Member Functions | |
virtual unsigned int | GetNumNodes () override |
Get the number of nodes used by this element. | |
virtual unsigned int | GetNumCoordsPosLevel () override |
Get the number of coordinates in the field used by the referenced nodes. More... | |
virtual unsigned int | GetNodeNumCoordsPosLevel (unsigned int n) override |
Get the number of coordinates from the specified node that are used by this element. More... | |
virtual std::shared_ptr< ChNodeFEAbase > | GetNode (unsigned int n) override |
Access the nth node. | |
virtual void | SetNodes (std::shared_ptr< ChNodeFEAxyz > nodeA, std::shared_ptr< ChNodeFEAxyz > nodeB) |
virtual void | GetStateBlock (ChVectorDynamic<> &mD) override |
Fills the D vector with the current field values at the nodes of the element, with proper ordering. More... | |
virtual void | ComputeKRMmatricesGlobal (ChMatrixRef H, double Kfactor, double Rfactor=0, double Mfactor=0) override |
Sets H as the global stiffness matrix K, scaled by Kfactor. More... | |
virtual void | ComputeInternalForces (ChVectorDynamic<> &Fi) override |
Computes the internal forces (ex. More... | |
virtual void | SetSpringCoefficient (double ms) |
Set the stiffness of the spring that connects the two nodes (N/m) | |
virtual double | GetSpringCoefficient () |
virtual void | SetDampingCoefficient (double md) |
Set the damping of the damper that connects the two nodes (Ns/M) | |
virtual double | GetDampingCoefficient () |
virtual double | GetCurrentForce () |
Get the current force transmitted along the spring direction, including the effect of the damper. More... | |
![]() | |
ChKRMBlock & | Kstiffness () |
Access the proxy to stiffness, for sparse solver. | |
virtual void | EleIntLoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Add the internal forces (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += forces * c This default implementation is SLIGHTLY INEFFICIENT. | |
virtual void | EleIntLoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Add the product of element mass M by a vector w (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += M * w * c This default implementation is VERY INEFFICIENT. | |
virtual void | EleIntLoadLumpedMass_Md (ChVectorDynamic<> &Md, double &error, const double c) override |
Adds the lumped mass to a Md vector, representing a mass diagonal matrix. More... | |
virtual void | EleIntLoadResidual_F_gravity (ChVectorDynamic<> &R, const ChVector3d &G_acc, const double c) override |
Add the contribution of gravity loads, multiplied by a scaling factor c, as: R += M * g * c This default implementation is VERY INEFFICIENT. More... | |
virtual void | ComputeGravityForces (ChVectorDynamic<> &Fg, const ChVector3d &G_acc) override |
Compute the gravitational forces. More... | |
virtual void | ComputeMmatrixGlobal (ChMatrixRef M) override |
Calculate the mass matrix, expressed in global reference. More... | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) override |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) override |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
virtual void | VariablesFbLoadInternalForces (double factor=1.) override |
Add the internal forces, expressed as nodal forces, into the encapsulated ChVariables. | |
virtual void | VariablesFbIncrementMq () override |
Add M*q (internal masses multiplied current 'qb'). | |
![]() | |
virtual unsigned int | GetNumCoordsPosLevelActive () |
Get the actual number of active degrees of freedom. More... | |
virtual unsigned int | GetNodeNumCoordsPosLevelActive (unsigned int n) |
Get the actual number of active coordinates from the specified node that are used by this element. More... | |
virtual void | ComputeNodalMass () |
Compute element's nodal masses. | |
virtual void | Update () |
Update, called at least at each time step. More... | |
virtual void | EleDoIntegration () |
This is optionally implemented if there is some internal state that requires integration. | |
Additional Inherited Members | |
![]() | |
ChKRMBlock | Kmatr |
Member Function Documentation
◆ ComputeInternalForces()
|
overridevirtual |
Computes the internal forces (ex.
the actual position of nodes is not in relaxed reference position) and set values in the Fi vector.
Implements chrono::fea::ChElementBase.
◆ ComputeKRMmatricesGlobal()
|
overridevirtual |
Sets H as the global stiffness matrix K, scaled by Kfactor.
Optionally, also superimposes global damping matrix R, scaled by Rfactor, and global mass matrix M multiplied by Mfactor. (For the spring matrix there is no need to corotate local matrices: we already know a closed form expression.)
Implements chrono::fea::ChElementBase.
◆ GetCurrentForce()
|
virtual |
Get the current force transmitted along the spring direction, including the effect of the damper.
Positive if pulled. (N)
◆ GetNodeNumCoordsPosLevel()
|
inlineoverridevirtual |
Get the number of coordinates from the specified node that are used by this element.
Note that this may be different from the value returned by GetNode(n)->GetNumCoordsVelLevel().
Implements chrono::fea::ChElementBase.
◆ GetNumCoordsPosLevel()
|
inlineoverridevirtual |
Get the number of coordinates in the field used by the referenced nodes.
This is for example the size (number of rows/columns) of the local stiffness matrix.
Implements chrono::fea::ChElementBase.
◆ GetStateBlock()
|
overridevirtual |
Fills the D vector with the current field values at the nodes of the element, with proper ordering.
If the D vector has not the size of this->GetNumCoordsPosLevel(), it will be resized.
Implements chrono::fea::ChElementBase.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChElementSpring.h
- /builds/uwsbel/chrono/src/chrono/fea/ChElementSpring.cpp