Description
Material data for a collision surface for use with non-smooth (complementarity) contact method.
Notes:
- Rolling friction
Rolling resistant torque is Tr <= (normal force) * (rolling friction parameter)
The rolling friction parameter is usually a very low value. Measuring unit: m.
A non-zero value will make the simulation 2x slower! - Spinning friction
Spinning resistant torque is Ts <= (normal force) * (spinning friction parameter)
The spinning friction parameter is usually a very low value. Measuring unit: m.
A non-zero value will make the simulation 2x slower!
#include <ChMaterialSurfaceNSC.h>
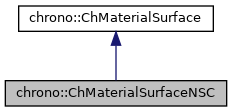
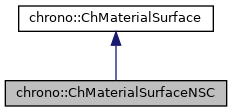
Public Member Functions | |
ChMaterialSurfaceNSC (const ChMaterialSurfaceNSC &other) | |
virtual ChMaterialSurfaceNSC * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual ChContactMethod | GetContactMethod () const override |
float | GetCohesion () |
The cohesion max. force for normal pulling traction in contacts. Measuring unit: N. Default 0. | |
void | SetCohesion (float mval) |
float | GetDampingF () |
The damping in contact, as a factor 'f': damping is a multiple of stiffness [K], that is: [R]=f*[K] Measuring unit: time, s. More... | |
void | SetDampingF (float mval) |
float | GetCompliance () |
Compliance of the contact, in normal direction. More... | |
void | SetCompliance (float mval) |
float | GetComplianceT () |
Compliance of the contact, in tangential direction. More... | |
void | SetComplianceT (float mval) |
float | GetComplianceRolling () |
Rolling compliance of the contact, if using a nonzero rolling friction. More... | |
void | SetComplianceRolling (float mval) |
float | GetComplianceSpinning () |
Spinning compliance of the contact, if using a nonzero rolling friction. More... | |
void | SetComplianceSpinning (float mval) |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
void | SetSfriction (float val) |
Static sliding friction coefficient. More... | |
float | GetSfriction () const |
void | SetKfriction (float val) |
Kinetic sliding friction coefficient. | |
float | GetKfriction () const |
void | SetFriction (float val) |
Set both static friction and kinetic friction at once, with same value. | |
void | SetRollingFriction (float val) |
Rolling friction coefficient. Usually around 1E-3. Default 0. | |
float | GetRollingFriction () const |
void | SetSpinningFriction (float val) |
Spinning friction coefficient. Usually around 1E-3. Default 0. | |
float | GetSpinningFriction () const |
void | SetRestitution (float val) |
Normal coefficient of restitution. In the range [0,1]. Default 0. | |
float | GetRestitution () const |
Public Attributes | |
float | cohesion |
float | dampingf |
float | compliance |
float | complianceT |
float | complianceRoll |
float | complianceSpin |
![]() | |
float | static_friction |
Static coefficient of friction. | |
float | sliding_friction |
Kinetic coefficient of friction. | |
float | rolling_friction |
Rolling coefficient of friction. | |
float | spinning_friction |
Spinning coefficient of friction. | |
float | restitution |
Coefficient of restitution. | |
Additional Inherited Members | |
![]() | |
static std::shared_ptr< ChMaterialSurface > | DefaultMaterial (ChContactMethod contact_method) |
Construct and return a contact material of the specified type with default properties. | |
![]() | |
ChMaterialSurface (const ChMaterialSurface &other) | |
Member Function Documentation
◆ GetCompliance()
|
inline |
Compliance of the contact, in normal direction.
It is the inverse of the stiffness [K] , so for zero value one has a perfectly rigid contact. Measuring unit: m/N. Default 0.
◆ GetComplianceRolling()
|
inline |
Rolling compliance of the contact, if using a nonzero rolling friction.
(If there is no rolling friction, this has no effect.) Measuring unit: rad/Nm. Default 0.
◆ GetComplianceSpinning()
|
inline |
Spinning compliance of the contact, if using a nonzero rolling friction.
(If there is no spinning friction, this has no effect.) Measuring unit: rad/Nm. Default 0.
◆ GetComplianceT()
|
inline |
Compliance of the contact, in tangential direction.
Measuring unit: m/N. Default 0.
◆ GetDampingF()
|
inline |
The damping in contact, as a factor 'f': damping is a multiple of stiffness [K], that is: [R]=f*[K] Measuring unit: time, s.
Default 0.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChMaterialSurfaceNSC.h
- /builds/uwsbel/chrono/src/chrono/physics/ChMaterialSurfaceNSC.cpp