Description
Geometric object representing a Bspline spline.
#include <ChLineBSpline.h>
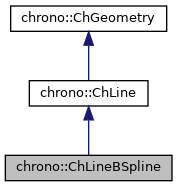
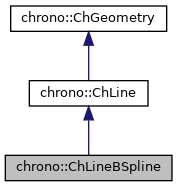
Public Member Functions | |
ChLineBSpline () | |
Constructor. By default, a segment (order = 1, two points on X axis, at -1, +1) | |
ChLineBSpline (int morder, const std::vector< ChVector3d > &mpoints, ChVectorDynamic<> *mknots=0) | |
Constructor from a given array of control points. More... | |
ChLineBSpline (const ChLineBSpline &source) | |
virtual ChLineBSpline * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual int | GetComplexity () const override |
Tell the complexity. | |
virtual ChVector3d | Evaluate (double U) const override |
Return a point on the line, given parametric coordinate U (in [0,1]). | |
virtual ChVector3d | GetTangent (double parU) const override |
Return the tangent unit vector at the parametric coordinate U (in [0,1]). | |
double | ComputeUfromKnotU (double u) const |
When using Evaluate() etc. More... | |
double | ComputeKnotUfromU (double U) const |
When using Evaluate() etc. More... | |
std::vector< ChVector3d > & | Points () |
Access the points. | |
ChVectorDynamic & | Knots () |
Access the knots. | |
int | GetOrder () |
Get the order of spline. | |
virtual void | Setup (int morder, const std::vector< ChVector3d > &mpoints, ChVectorDynamic<> *mknots=0) |
Initial easy setup from a given array of control points. More... | |
virtual void | SetClosed (bool mc) override |
Set as closed spline: start and end will overlap at 0 and 1 abscyssa as p(0)=p(1), and the Evaluate() and GetTangent() functions will operate in periodic way (abscyssa greater than 1 or smaller than 0 will wrap to 0..1 range). More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChLine (const ChLine &source) | |
virtual Type | GetType () const override |
"Virtual" copy constructor (covariant return type). More... | |
virtual bool | IsClosed () const |
Tell if the curve is closed. | |
virtual void | SetComplexity (int mc) |
virtual int | GetManifoldDimension () const override |
This is a line. | |
bool | FindNearestLinePoint (ChVector3d &point, double &resU, double approxU, double tol) const |
Find the parameter resU for the nearest point on curve to "point". | |
virtual double | Length (int sampling) const |
Returns curve length. Typical sampling 1..5 (1 already gives correct result with degree1 curves) | |
virtual ChVector3d | GetEndA () const |
Return the start point of the line. More... | |
virtual ChVector3d | GetEndB () const |
Return the end point of the line. More... | |
double | CurveCurveDist (ChLine *compline, int samples) const |
Returns adimensional information on "how much" this curve is similar to another in its overall shape (does not matter parametrization or start point). More... | |
double | CurveSegmentDist (ChLine *complinesegm, int samples) const |
Same as before, but returns "how near" is complinesegm to whatever segment of this line (does not matter the percentual of line). More... | |
double | CurveCurveDistMax (ChLine *compline, int samples) const |
Same as above, but instead of making average of the distances, these functions return the maximum of the distances... | |
double | CurveSegmentDistMax (ChLine *complinesegm, int samples) const |
![]() | |
ChGeometry (const ChGeometry &other) | |
virtual ChAABB | GetBoundingBox () const |
Compute bounding box along the directions of the shape definition frame. More... | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual ChVector3d | Baricenter () const |
Compute center of mass. | |
virtual void | Update () |
Generic update of internal data. | |
Public Attributes | |
std::vector< ChVector3d > | points |
ChVectorDynamic | knots |
int | p |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
![]() | |
bool | closed |
int | complexityU |
Constructor & Destructor Documentation
◆ ChLineBSpline()
chrono::ChLineBSpline::ChLineBSpline | ( | int | morder, |
const std::vector< ChVector3d > & | mpoints, | ||
ChVectorDynamic<> * | mknots = 0 |
||
) |
Constructor from a given array of control points.
Input data is copied. If the knots are not provided, a uniformly spaced knot vector is made.
- Parameters
-
morder order p: 1= linear, 2=quadratic, etc. mpoints control points, size n. Required: at least n >= p+1 mknots knots, size k. Required k=n+p+1. If not provided, initialized to uniform.
Member Function Documentation
◆ ComputeKnotUfromU()
|
inline |
When using Evaluate() etc.
you need U parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert U->u, where u is in knot range, calling this:
◆ ComputeUfromKnotU()
|
inline |
When using Evaluate() etc.
you need U parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert u->U, where u is in knot range, calling this:
◆ SetClosed()
|
overridevirtual |
Set as closed spline: start and end will overlap at 0 and 1 abscyssa as p(0)=p(1), and the Evaluate() and GetTangent() functions will operate in periodic way (abscyssa greater than 1 or smaller than 0 will wrap to 0..1 range).
The closure will change the knot vector (multiple start end knots will be lost) and will create auxiliary p control points at the end that will be wrapped to the beginning control point.
Reimplemented from chrono::ChLine.
◆ Setup()
|
virtual |
Initial easy setup from a given array of control points.
Input data is copied. If the knots are not provided, a uniformly spaced knot vector is made.
- Parameters
-
morder order p: 1= linear, 2=quadratic, etc. mpoints control points, size n. Required: at least n >= p+1 mknots knots, size k. Required k=n+p+1. If not provided, initialized to uniform.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChLineBSpline.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChLineBSpline.cpp