Description
Base class for a co-simulation node.
#include <ChVehicleCosimBaseNode.h>
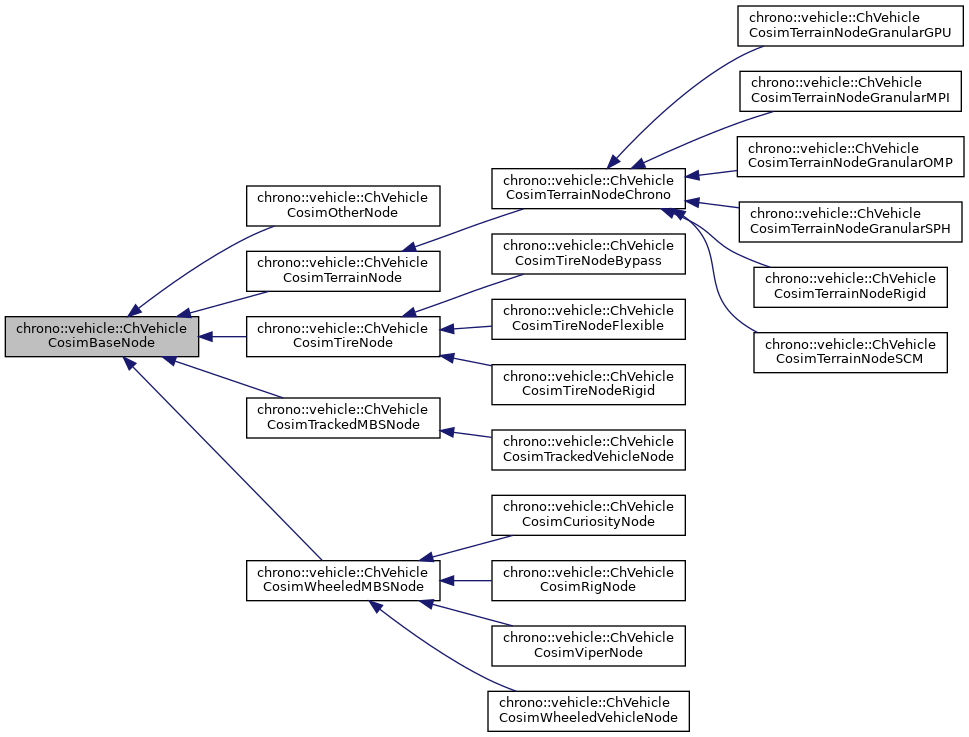
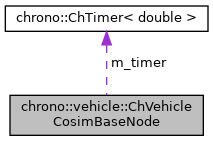
Classes | |
struct | MeshContact |
Mesh contact information (received from terrain node) More... | |
struct | MeshState |
Mesh state information (sent to terrain node) More... | |
Public Types | |
enum | NodeType { NodeType::MBS_WHEELED, NodeType::MBS_TRACKED, NodeType::TERRAIN, NodeType::TIRE } |
Type of node participating in co-simulation. More... | |
enum | InterfaceType { InterfaceType::BODY, InterfaceType::MESH } |
Type of the tire-terrain communication interface. More... | |
Public Member Functions | |
virtual NodeType | GetNodeType () const =0 |
Return the node type. | |
std::string | GetNodeTypeString () const |
Return the node type as a string. | |
bool | IsCosimNode () const |
Return true if this node is part of the co-simulation infrastructure. | |
void | SetStepSize (double step) |
Set the integration step size (default: 1e-4). | |
double | GetStepSize () const |
Get the integration step size. | |
void | SetOutDir (const std::string &dir_name, const std::string &suffix) |
Set the name of the output directory and an identifying suffix. More... | |
void | SetVerbose (bool verbose) |
Enable/disable verbose messages during simulation (default: true). | |
const std::string & | GetOutDirName () const |
Get the output directory name for this node. | |
double | GetStepExecutionTime () const |
Get the simulation execution time for the current step on this node. More... | |
double | GetTotalExecutionTime () const |
Get the cumulative simulation execution time on this node. | |
virtual void | Initialize () |
Initialize this node. More... | |
virtual void | Synchronize (int step_number, double time)=0 |
Synchronize this node. More... | |
virtual void | Advance (double step_size)=0 |
Advance simulation. More... | |
virtual void | OutputData (int frame)=0 |
Output logging and debugging data. | |
virtual void | OutputVisualizationData (int frame)=0 |
Output post-processing visualization data. More... | |
virtual void | WriteCheckpoint (const std::string &filename) const |
Write checkpoint to the specified file (which will be created in the output directory). | |
Static Public Member Functions | |
static std::string | OutputFilename (const std::string &dir, const std::string &root, const std::string &ext, int frame, int frame_digits) |
Utility function for creating an output file name. More... | |
Protected Member Functions | |
ChVehicleCosimBaseNode (const std::string &name) | |
void | SendGeometry (const ChVehicleGeometry &geom, int dest) const |
void | RecvGeometry (ChVehicleGeometry &geom, int source) const |
Protected Attributes | |
int | m_rank |
MPI rank of this node (in MPI_COMM_WORLD) | |
double | m_step_size |
integration step size | |
std::string | m_name |
name of the node | |
std::string | m_out_dir |
top-level output directory | |
std::string | m_node_out_dir |
node-specific output directory | |
std::ofstream | m_outf |
output file stream | |
unsigned int | m_num_wheeled_mbs_nodes |
unsigned int | m_num_tracked_mbs_nodes |
unsigned int | m_num_terrain_nodes |
unsigned int | m_num_tire_nodes |
ChTimer< double > | m_timer |
timer for integration cost | |
double | m_cum_sim_time |
cumulative integration cost | |
bool | m_verbose |
verbose messages during simulation? | |
Static Protected Attributes | |
static const double | m_gacc = -9.81 |
Member Enumeration Documentation
◆ InterfaceType
Type of the tire-terrain communication interface.
- A BODY interface assumes communication is done at the wheel spindle or track shoe level. At a synchronization time, the terrain node receives the full state of the spindle or track shoe body and must send forces acting on that body, for each tire or track shoe present in the simulation. This type of interface should be used for track shoes, rigid tires, or when the terrain node also performs the dynamics of a flexible tire.
- A MESH interface assumes communication is done at the tire mesh level. At a synchronization time, the terrain node receives the tire mesh vertex states (positions and velocities) are must send forces acting on vertices of the mesh, for each object. This interface is typically used when flexible tires are simulated outside the terrain node (on separate tire nodes).
Enumerator | |
---|---|
BODY | exchange state and force for a single body (wheel spindle or track shoe) |
MESH | exchange state and force for a mesh (flexible tire mesh) |
◆ NodeType
Member Function Documentation
◆ Advance()
|
pure virtual |
Advance simulation.
This function is called after a synchronization to allow the node to advance its state by the specified time step. A node is allowed to take as many internal integration steps as required, but no inter-node communication should occur.
Implemented in chrono::vehicle::ChVehicleCosimWheeledMBSNode, chrono::vehicle::ChVehicleCosimTerrainNode, chrono::vehicle::ChVehicleCosimTrackedMBSNode, chrono::vehicle::ChVehicleCosimTireNodeFlexible, chrono::vehicle::ChVehicleCosimTireNodeRigid, chrono::vehicle::ChVehicleCosimOtherNode, and chrono::vehicle::ChVehicleCosimTireNodeBypass.
◆ GetStepExecutionTime()
|
inline |
Get the simulation execution time for the current step on this node.
This represents the time elapsed since the last synchronization point.
◆ Initialize()
|
virtual |
Initialize this node.
This function allows the node to initialize itself and, optionally, perform an initial data exchange with any other node. A derived class implementation should first call this base class function.
Reimplemented in chrono::vehicle::ChVehicleCosimTireNode, chrono::vehicle::ChVehicleCosimWheeledMBSNode, chrono::vehicle::ChVehicleCosimTerrainNode, and chrono::vehicle::ChVehicleCosimTrackedMBSNode.
◆ OutputFilename()
|
static |
Utility function for creating an output file name.
It generates and returns a string of the form "{dir}/{root}_{frame}.{ext}", where {frame} is printed using the format "%0{frame_digits}d".
◆ OutputVisualizationData()
|
pure virtual |
Output post-processing visualization data.
If implemented, this function should write a file in the "visualization" subdirectory of m_node_out_dir.
Implemented in chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP, chrono::vehicle::ChVehicleCosimTerrainNodeGranularGPU, chrono::vehicle::ChVehicleCosimWheeledMBSNode, chrono::vehicle::ChVehicleCosimTrackedMBSNode, chrono::vehicle::ChVehicleCosimTerrainNodeRigid, chrono::vehicle::ChVehicleCosimTerrainNodeGranularSPH, chrono::vehicle::ChVehicleCosimOtherNode, and chrono::vehicle::ChVehicleCosimTerrainNode.
◆ SetOutDir()
void chrono::vehicle::ChVehicleCosimBaseNode::SetOutDir | ( | const std::string & | dir_name, |
const std::string & | suffix | ||
) |
Set the name of the output directory and an identifying suffix.
Output files will be created in subdirectories named dir_name/[NodeName]suffix/ where [NodeName] is "MBS", "TIRE", or "TERRAIN".
◆ Synchronize()
|
pure virtual |
Synchronize this node.
This function is called at every co-simulation synchronization time to allow the node to exchange information with any other node.
Implemented in chrono::vehicle::ChVehicleCosimTireNode, chrono::vehicle::ChVehicleCosimWheeledMBSNode, chrono::vehicle::ChVehicleCosimTerrainNode, chrono::vehicle::ChVehicleCosimTrackedMBSNode, and chrono::vehicle::ChVehicleCosimOtherNode.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/ChVehicleCosimBaseNode.h
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/ChVehicleCosimBaseNode.cpp