chrono::vehicle::citybus::CityBus_SolidAxleRear Class Reference
Description
Solid-axle rear suspension subsystem for the city bus vehicle.
#include <CityBus_SolidAxle.h>
Inheritance diagram for chrono::vehicle::citybus::CityBus_SolidAxleRear:
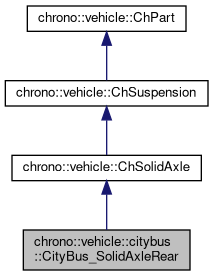
Collaboration diagram for chrono::vehicle::citybus::CityBus_SolidAxleRear:
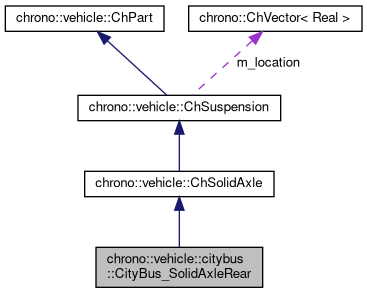
Public Member Functions | |
CityBus_SolidAxleRear (const std::string &name) | |
virtual double | getAxleTubeMass () const override |
Return the mass of the axle tube body. | |
virtual double | getSpindleMass () const override |
Return the mass of the spindle body. | |
virtual double | getULMass () const override |
Return the mass of the upper link body. | |
virtual double | getLLMass () const override |
Return the mass of the lower link body. | |
virtual double | getKnuckleMass () const override |
Return the mass of the knuckle body. | |
virtual double | getTierodMass () const override |
Return the mass of the tierod body. | |
virtual double | getDraglinkMass () const override |
Return the mass of the draglink body. | |
virtual double | getBellCrankMass () const override |
Return the mass of the bell crank body. | |
virtual double | getAxleTubeRadius () const override |
Return the radius of the axle tube body (visualization only). | |
virtual double | getSpindleRadius () const override |
Return the radius of the spindle body (visualization only). | |
virtual double | getSpindleWidth () const override |
Return the width of the spindle body (visualization only). | |
virtual double | getULRadius () const override |
Return the radius of the upper link body (visualization only). | |
virtual double | getLLRadius () const override |
Return the radius of the lower link body (visualization only). | |
virtual double | getKnuckleRadius () const override |
Return the radius of the knuckle body (visualization only). | |
virtual double | getTierodRadius () const override |
Return the radius of the tierod body (visualization only). | |
virtual double | getDraglinkRadius () const override |
Return the radius of the draglink body (visualization only). | |
virtual double | getBellCrankRadius () const override |
Return the radius of the bell crank body (visualization only). | |
virtual const ChVector & | getAxleTubeInertia () const override |
Return the moments of inertia of the axle tube body. | |
virtual const ChVector & | getSpindleInertia () const override |
Return the moments of inertia of the spindle body. | |
virtual const ChVector & | getULInertia () const override |
Return the moments of inertia of the upper link body. | |
virtual const ChVector & | getLLInertia () const override |
Return the moments of inertia of the lower link body. | |
virtual const ChVector & | getKnuckleInertia () const override |
Return the moments of inertia of the knuckle body. | |
virtual const ChVector & | getTierodInertia () const override |
Return the moments of inertia of the tierod body. | |
virtual const ChVector & | getDraglinkInertia () const override |
Return the moments of inertia of the draglink body. | |
virtual const ChVector & | getBellCrankInertia () const override |
Return the moments of inertia of the bell crank body. | |
virtual double | getAxleInertia () const override |
Return the inertia of the axle shaft. | |
virtual double | getSpringRestLength () const override |
Return the free (rest) length of the spring element. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getSpringForceFunctor () const override |
Return the functor object for spring force. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getShockForceFunctor () const override |
Return the functor object for shock force. | |
virtual const ChVector | getAxleTubeCOM () const override |
Return the center of mass of the axle tube. | |
![]() | |
ChSolidAxle (const std::string &name) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual bool | IsSteerable () const final override |
Specify whether or not this suspension can be steered. | |
virtual bool | IsIndependent () const final override |
Specify whether or not this is an independent suspension. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, std::shared_ptr< ChSubchassis > subchassis, std::shared_ptr< ChSteering > steering, const ChVector<> &location, double left_ang_vel=0, double right_ang_vel=0) override |
Initialize this suspension subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the suspension subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the suspension subsystem. | |
virtual double | GetMass () const override |
Get the total mass of the suspension subsystem. | |
virtual ChVector | GetCOMPos () const override |
Get the current global COM location of the suspension subsystem. | |
virtual double | GetTrack () override |
Get the wheel track for the suspension subsystem. | |
std::shared_ptr< ChLinkTSDA > | GetSpring (VehicleSide side) const |
Get a handle to the specified spring element. | |
std::shared_ptr< ChLinkTSDA > | GetShock (VehicleSide side) const |
Get a handle to the specified shock (damper) element. | |
virtual ChSuspension::Force | ReportSuspensionForce (VehicleSide side) const override |
Return current suspension forces (spring and shock) on the specified side. | |
double | GetSpringForce (VehicleSide side) const |
Get the force in the spring element. | |
double | GetSpringLength (VehicleSide side) const |
Get the current length of the spring element. | |
double | GetSpringDeformation (VehicleSide side) const |
Get the current deformation of the spring element. | |
double | GetShockForce (VehicleSide side) const |
Get the force in the shock (damper) element. | |
double | GetShockLength (VehicleSide side) const |
Get the current length of the shock (damper) element. | |
double | GetShockVelocity (VehicleSide side) const |
Get the current deformation velocity of the shock (damper) element. | |
virtual void | LogConstraintViolations (VehicleSide side) override |
Log current constraint violations. | |
void | LogHardpointLocations (const ChVector<> &ref, bool inches=false) |
![]() | |
ChSuspension (const std::string &name) | |
const ChVector & | GetLocation () const |
Get the location of the suspension subsystem relative to the chassis reference frame. More... | |
std::shared_ptr< ChBody > | GetSpindle (VehicleSide side) const |
Get a handle to the spindle body on the specified side. | |
std::shared_ptr< ChShaft > | GetAxle (VehicleSide side) const |
Get a handle to the axle shaft on the specified side. | |
std::shared_ptr< ChLinkLockRevolute > | GetRevolute (VehicleSide side) const |
Get a handle to the revolute joint on the specified side. | |
const ChVector & | GetSpindlePos (VehicleSide side) const |
Get the global location of the spindle on the specified side. | |
ChQuaternion | GetSpindleRot (VehicleSide side) const |
Get the orientation of the spindle body on the specified side. More... | |
const ChVector & | GetSpindleLinVel (VehicleSide side) const |
Get the linear velocity of the spindle body on the specified side. More... | |
ChVector | GetSpindleAngVel (VehicleSide side) const |
Get the angular velocity of the spindle body on the specified side. More... | |
double | GetAxleSpeed (VehicleSide side) const |
Get the angular speed of the axle on the specified side. | |
void | Synchronize () |
Synchronize this suspension subsystem. More... | |
void | ApplyAxleTorque (VehicleSide side, double torque) |
Apply the provided motor torque. More... | |
virtual std::shared_ptr< ChBody > | GetAntirollBody (VehicleSide side) const |
Specify the suspension body on the specified side to attach a possible antirollbar subsystem. More... | |
virtual std::shared_ptr< ChBody > | GetBrakeBody (VehicleSide side) const |
Specify the body on the specified side for a possible connection to brake subsystem. More... | |
void | ApplyParkingBrake (bool brake) |
Simple model of a parking brake. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
enum | PointId { SHOCK_A, SHOCK_C, KNUCKLE_L, KNUCKLE_U, LL_A, LL_C, UL_A, UL_C, SPRING_A, SPRING_C, TIEROD_K, SPINDLE, KNUCKLE_CM, LL_CM, UL_CM, BELLCRANK_TIEROD, BELLCRANK_AXLE, BELLCRANK_DRAGLINK, DRAGLINK_C, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
std::shared_ptr< ChBody > | m_axleTube |
handles to the axle tube body | |
std::shared_ptr< ChBody > | m_tierod |
handles to the tierod body | |
std::shared_ptr< ChBody > | m_bellCrank |
handles to the bellcrank body | |
std::shared_ptr< ChBody > | m_draglink |
handles to the draglink body | |
std::shared_ptr< ChBody > | m_knuckle [2] |
handles to the knuckle bodies (L/R) | |
std::shared_ptr< ChBody > | m_upperLink [2] |
handles to the upper link bodies (L/R) | |
std::shared_ptr< ChBody > | m_lowerLink [2] |
handles to the lower link bodies (L/R) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteBellCrank |
bellCrank-axle revolute joint (left) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalTierod |
knuckle-tierod spherical joint (left) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalDraglink |
draglink-chassis spherical joint (left) | |
std::shared_ptr< ChLinkUniversal > | m_universalDraglink |
draglink-bellCrank universal joint (left) | |
std::shared_ptr< ChLinkUniversal > | m_universalTierod |
knuckle-tierod universal joint (right) | |
std::shared_ptr< ChLinkLockPointPlane > | m_pointPlaneBellCrank |
bellCrank-tierod point-plane joint (left) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteKingpin [2] |
knuckle-axle tube revolute joints (L/R) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalUpperLink [2] |
upper link-axle tube spherical joints (L/R) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalLowerLink [2] |
lower link-axle tube spherical joints (L/R) | |
std::shared_ptr< ChLinkUniversal > | m_universalUpperLink [2] |
upper link-chassis universal joints (L/R) | |
std::shared_ptr< ChLinkUniversal > | m_universalLowerLink [2] |
lower link-chassis universal joints (L/R) | |
std::shared_ptr< ChLinkTSDA > | m_shock [2] |
handles to the spring links (L/R) | |
std::shared_ptr< ChLinkTSDA > | m_spring [2] |
handles to the shock links (L/R) | |
![]() | |
ChVector | m_location |
location relative to chassis | |
std::shared_ptr< ChBody > | m_spindle [2] |
handles to spindle bodies | |
std::shared_ptr< ChShaft > | m_axle [2] |
handles to axle shafts | |
std::shared_ptr< ChShaftsBody > | m_axle_to_spindle [2] |
handles to spindle-shaft connectors | |
std::shared_ptr< ChLinkLockRevolute > | m_revolute [2] |
handles to spindle revolute joints | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/citybus/CityBus_SolidAxle.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/citybus/CityBus_SolidAxle.cpp