Description
Class for rotational spring-damper elements with the torque specified through a callback object.
The torque is applied in the current direction of the relative axis of rotation. While a rotational spring-damper can be associated with any pair of bodies in the system, it is typically used in cases where the mechanism kinematics are such that the two bodies have a single relative rotational degree of freedom (e.g, between two bodies connected through a revolute, cylindrical, or screw joint).
#include <ChLinkRotSpringCB.h>
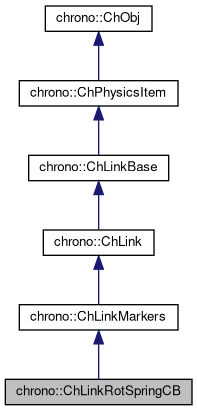
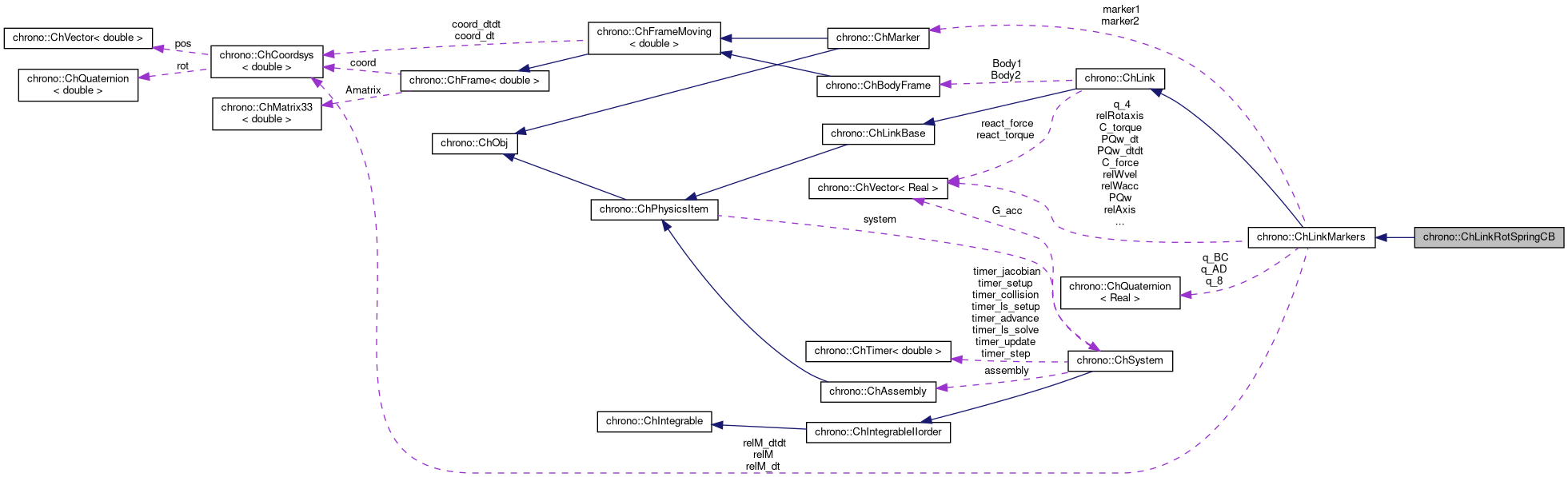
Classes | |
class | TorqueFunctor |
Class to be used as a callback interface for calculating the general spring-damper torque. More... | |
Public Member Functions | |
ChLinkRotSpringCB (const ChLinkRotSpringCB &other) | |
virtual ChLinkRotSpringCB * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
double | GetRotSpringAngle () const |
Get the current rotation angle about the relative rotation axis. | |
const ChVector & | GetRotSpringAxis () const |
Get the current relative axis of rotation. | |
double | GetRotSpringSpeed () const |
Get the current relative angular speed about the common rotation axis. | |
double | GetRotSpringTorque () const |
Get the current generated torque. | |
void | RegisterTorqueFunctor (std::shared_ptr< TorqueFunctor > functor) |
Specify the callback object for calculating the torque. | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. More... | |
![]() | |
ChMarker * | GetMarker1 () |
Return the 1st referenced marker (the 'slave' marker, owned by 1st body) | |
ChMarker * | GetMarker2 () |
Return the 2nd referenced marker (the 'master' marker, owned by 2nd body) | |
virtual void | SetUpMarkers (ChMarker *mark1, ChMarker *mark2) |
set the two markers associated with this link | |
void | SetMarkID1 (int mid) |
void | SetMarkID2 (int mid) |
int | GetMarkID1 () |
int | GetMarkID2 () |
bool | ReferenceMarkers (ChMarker *mark1, ChMarker *mark2) |
Shortcut: set markers and marker IDs at once. | |
virtual void | Initialize (std::shared_ptr< ChMarker > mark1, std::shared_ptr< ChMarker > mark2) |
Use this function after link creation, to initialize the link from two markers to join. More... | |
virtual void | Initialize (std::shared_ptr< ChBody > mbody1, std::shared_ptr< ChBody > mbody2, const ChCoordsys<> &mpos) |
Use this function after link creation, to initialize the link from two joined rigid bodies. More... | |
virtual void | Initialize (std::shared_ptr< ChBody > mbody1, std::shared_ptr< ChBody > mbody2, bool pos_are_relative, const ChCoordsys<> &mpos1, const ChCoordsys<> &mpos2) |
Use this function after link creation, to initialize the link from two joined rigid bodies. More... | |
virtual ChCoordsys | GetLinkRelativeCoords () override |
Get the link coordinate system, expressed relative to Body2 (the 'master' body). More... | |
virtual ChFrame | GetAssetsFrame (unsigned int nclone=0) override |
Get the master coordinate system for the assets (this will return the absolute coordinate system of the 'master' marker2) | |
virtual void | UpdateRelMarkerCoords () |
Updates auxiliary quantities for all relative degrees of freedom of the two markers. | |
virtual void | Update (double mytime, bool update_assets=true) override |
Complete link update: UpdateTime -> UpdateRelMarkerCoords -> UpdateForces. | |
virtual void | IntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) override |
Adds force to residual R, as R*= F*c NOTE: here the off offset in R is NOT used because add F at the TWO offsets of the two connected bodies, so it is assumed that offsets for Body1 and Body2 variables have been already set properly! | |
virtual void | ConstraintsFbLoadForces (double factor=1) override |
Overrides the empty behaviour of the parent ChLink implementation, which does not consider any user-imposed force between the two bodies. More... | |
const Coordsys & | GetRelM () const |
Relative position of marker 1 respect to marker 2. | |
const Coordsys & | GetRelM_dt () const |
Relative speed of marker 1 respect to marker 2. | |
const Coordsys & | GetRelM_dtdt () const |
Relative acceleration of marker 1 respect to marker 2. | |
double | GetRelAngle () const |
Relative rotation angle of marker 1 respect to marker 2 (best with revolute joints..). | |
const ChVector & | GetRelAxis () const |
Relative finite rotation axis of marker 1 respect to marker 2. | |
const ChVector & | GetRelRotaxis () const |
const ChVector & | GetRelWvel () const |
Relative angular speed of marker 1 respect to marker 2. | |
const ChVector & | GetRelWacc () const |
Relative angular acceleration of marker 1 respect to marker 2. | |
double | GetDist () const |
Relative 'polar' distance of marker 1 respect to marker 2. | |
double | GetDist_dt () const |
Relative speed of marker 1 respect to marker 2, along the polar distance vector. | |
const ChVector & | GetC_force () const |
Get the total applied force accumulators (force, momentum) in link coords. More... | |
const ChVector & | GetC_torque () const |
![]() | |
ChLink (const ChLink &other) | |
int | GetLeftDOF () |
Get the number of free degrees of freedom left by this link, between two bodies. | |
virtual int | GetNumCoords () override |
Get the number of scalar variables affected by constraints in this link. | |
ChBodyFrame * | GetBody1 () |
Get the constrained body '1', the 'slave' body. | |
ChBodyFrame * | GetBody2 () |
Get the constrained body '2', the 'master' body. | |
virtual ChCoordsys | GetLinkAbsoluteCoords () override |
Get the link coordinate system in absolute reference. More... | |
virtual ChVector | Get_react_force () override |
Get reaction force, expressed in link coordinate system. | |
virtual ChVector | Get_react_torque () override |
Get reaction torque, expressed in link coordinate system. | |
virtual int | RestoreRedundant () |
If some constraint is redundant, return to normal state //***OBSOLETE***. | |
virtual void | UpdateTime (double mytime) |
Given new time, current body state, update time-dependent quantities in link state, for example motion laws, moving markers, etc. More... | |
virtual void | Update (bool update_assets=true) override |
As above, but with current time. | |
virtual void | UpdatedExternalTime (double prevtime, double time) |
Called from a external package (i.e. a plugin, a CAD app.) to report that time has changed. | |
![]() | |
ChLinkBase (const ChLinkBase &other) | |
bool | IsValid () |
Tells if the link data is currently valid. More... | |
void | SetValid (bool mon) |
Set the status of link validity. | |
bool | IsDisabled () |
Tells if all constraints of this link are currently turned on or off by the user. | |
virtual void | SetDisabled (bool mdis) |
User can use this to enable/disable all the constraint of the link as desired. | |
bool | IsBroken () |
Tells if the link is broken, for excess of pulling/pushing. | |
virtual void | SetBroken (bool mon) |
Set the 'broken' status vof this link. | |
bool | IsActive () |
An important function! Tells if the link is currently active, in general, that is tells if it must be included into the system solver or not. More... | |
virtual ChVectorDynamic | GetConstraintViolation () const |
Get the current constraint violations. | |
virtual bool | IsRequiringWaking () |
Tells if this link requires that the connected ChBody objects must be waken if they are sleeping. More... | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem() | |
virtual void | SetSystem (ChSystem *m_system) |
Set the pointer to the parent ChSystem() and also add to new collision system / remove from old coll.system. | |
void | AddAsset (std::shared_ptr< ChAsset > masset) |
Add an optional asset (it can be used to define visualization shapes, es ChSphereShape, or textures, or custom attached properties that the user can define by creating his class inherited from ChAsset) | |
std::vector< std::shared_ptr< ChAsset > > & | GetAssets () |
Access to the list of optional assets. | |
std::shared_ptr< ChAsset > | GetAssetN (unsigned int num) |
Access the Nth asset in the list of optional assets. | |
virtual unsigned int | GetAssetsFrameNclones () |
Optionally, a ChPhysicsItem can return multiple asset coordinate systems; this can be helpful if, for example, when a ChPhysicsItem contains 'clones' with the same assets (ex. More... | |
virtual bool | GetCollide () const |
Tell if the object is subject to collision. More... | |
virtual void | SyncCollisionModels () |
If this physical item contains one or more collision models, synchronize their coordinates and bounding boxes to the state of the item. | |
virtual void | AddCollisionModelsToSystem () |
If this physical item contains one or more collision models, add them to the system's collision engine. | |
virtual void | RemoveCollisionModelsFromSystem () |
If this physical item contains one or more collision models, remove them from the system's collision engine. | |
virtual void | GetTotalAABB (ChVector<> &bbmin, ChVector<> &bbmax) |
Get the entire AABB axis-aligned bounding box of the object. More... | |
virtual void | GetCenter (ChVector<> &mcenter) |
Get a symbolic 'center' of the object. More... | |
virtual void | StreamINstate (ChStreamInBinary &mstream) |
Method to deserialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | StreamOUTstate (ChStreamOutBinary &mstream) |
Method to serialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | Setup () |
This might recompute the number of coordinates, DOFs, constraints, in case this might change (ex in ChAssembly), as well as state offsets of contained items (ex in ChMesh) | |
virtual void | SetNoSpeedNoAcceleration () |
Set zero speed (and zero accelerations) in state, without changing the position. More... | |
virtual int | GetDOF () |
Get the number of scalar coordinates (variables), if any, in this item. More... | |
virtual int | GetDOF_w () |
Get the number of scalar coordinates of variables derivatives (usually = DOF, but might be different than DOF, ex. More... | |
virtual int | GetDOC () |
Get the number of scalar constraints, if any, in this item. | |
virtual int | GetDOC_c () |
Get the number of scalar constraints, if any, in this item (only bilateral constr.) Children classes might override this. | |
virtual int | GetDOC_d () |
Get the number of scalar constraints, if any, in this item (only unilateral constr.) Children classes might override this. | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
virtual void | IntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets. More... | |
virtual void | IntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T, bool full_update) |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets. More... | |
virtual void | IntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) |
From item's state acceleration to global acceleration vector. More... | |
virtual void | IntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) |
From global acceleration vector to item's state acceleration. More... | |
virtual void | IntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) |
From item's reaction forces to global reaction vector. More... | |
virtual void | IntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) |
From global reaction vector to item's reaction forces. More... | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w. More... | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L. More... | |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | IntLoadConstraint_Ct (const unsigned int off, ChVectorDynamic<> &Qc, const double c) |
Takes the term Ct, scale and adds to Qc at given offset: Qc += c*Ct. More... | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) |
Prepare variables and constraints to accommodate a solution: More... | |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) |
After a solver solution, fetch values from variables and constraints into vectors: More... | |
virtual void | VariablesFbReset () |
Sets the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) |
Adds the current forces (applied to item) into the encapsulated ChVariables, in the 'fb' part: qf+=forces*factor. | |
virtual void | VariablesQbLoadSpeed () |
Initialize the 'qb' part of the ChVariables with the current value of speeds. More... | |
virtual void | VariablesFbIncrementMq () |
Adds M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) |
Fetches the item speed (ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | InjectVariables (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are variables of type ChVariables in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | InjectConstraints (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are constraints of type ChConstraint in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | ConstraintsBiReset () |
Sets to zero the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) |
Adds the current C (constraint violation) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Ct (double factor=1) |
Adds the current Ct (partial t-derivative, as in C_dt=0-> [Cq]*q_dt=-Ct) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsLoadJacobians () |
Adds the current jacobians in encapsulated ChConstraints. | |
virtual void | ConstraintsFetch_react (double factor=1) |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints. More... | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are items of type ChKblock in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Gets the numerical identifier of the object. | |
void | SetIdentifier (int id) |
Sets the numerical identifier of the object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
const char * | GetName () const |
Gets the name of the object as C Ascii null-terminated string -for reading only! | |
void | SetName (const char myname[]) |
Sets the name of this object, as ascii string. | |
std::string | GetNameString () const |
Gets the name of the object as C Ascii null-terminated string. | |
void | SetNameString (const std::string &myname) |
Sets the name of this object, as std::string. | |
void | MFlagsSetAllOFF (int &mflag) |
void | MFlagsSetAllON (int &mflag) |
void | MFlagSetON (int &mflag, int mask) |
void | MFlagSetOFF (int &mflag, int mask) |
int | MFlagGet (int &mflag, int mask) |
virtual std::string & | ArchiveContainerName () |
Protected Member Functions | |
virtual void | UpdateForces (double time) override |
Include the rotational spring-damper custom torque. | |
![]() | |
ChLinkMarkers (const ChLinkMarkers &other) | |
Protected Attributes | |
std::shared_ptr< TorqueFunctor > | m_torque_fun |
functor for torque calculation | |
double | m_torque |
resulting torque along relative axis of rotation | |
![]() | |
ChMarker * | marker1 |
slave coordsys | |
ChMarker * | marker2 |
master coordsys, =0 if liked to ground | |
int | markID1 |
unique identifier for markers 1 & 2, | |
int | markID2 |
when using plugin dynamic hierarchies | |
Coordsys | relM |
relative marker position 2-1 | |
Coordsys | relM_dt |
relative marker speed | |
Coordsys | relM_dtdt |
relative marker acceleration | |
double | relAngle |
relative angle of rotation | |
ChVector | relAxis |
relative axis of rotation | |
ChVector | relRotaxis |
relative rotaion vector =angle*axis | |
ChVector | relWvel |
relative angular speed | |
ChVector | relWacc |
relative angular acceleration | |
double | dist |
the distance between the two origins of markers, | |
double | dist_dt |
the speed between the two origins of markers | |
ChVector | C_force |
internal force applied by springs/dampers/actuators | |
ChVector | C_torque |
internal torque applied by springs/dampers/actuators | |
ChVector | PQw |
ChVector | PQw_dt |
ChVector | PQw_dtdt |
ChQuaternion | q_AD |
ChQuaternion | q_BC |
ChQuaternion | q_8 |
ChVector | q_4 |
![]() | |
ChBodyFrame * | Body1 |
first connected body | |
ChBodyFrame * | Body2 |
second connected body | |
ChVector | react_force |
store the xyz reactions, expressed in local coordinate system of link; | |
ChVector | react_torque |
store the torque reactions, expressed in local coordinate system of link; | |
![]() | |
bool | disabled |
all constraints of link disabled because of user needs | |
bool | valid |
link data is valid | |
bool | broken |
link is broken because of excessive pulling/pushing. | |
![]() | |
ChSystem * | system |
parent system | |
std::vector< std::shared_ptr< ChAsset > > | assets |
set of assets | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
the time of simulation for the object | |
Member Function Documentation
◆ ArchiveIN()
|
overridevirtual |
Method to allow deserialization of transient data from archives.
Method to allow de serialization of transient data from archives.
Reimplemented from chrono::ChLinkMarkers.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChLinkRotSpringCB.h
- /builds/uwsbel/chrono/src/chrono/physics/ChLinkRotSpringCB.cpp