Description
This is a base class for serializing into archives.
#include <ChArchive.h>
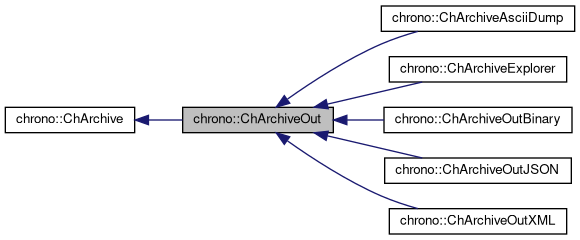
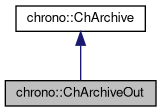
Public Member Functions | |
void | SetCutAllPointers (bool mcut) |
If you enable SetCutAllPointers(true), no serialization happens for objects referenced via pointers. More... | |
std::unordered_set< void * > & | CutPointers () |
Access the container of pointers that must not be serialized. More... | |
void | UnbindExternalPointer (void *mptr, size_t ID) |
Use the following to declare pointer(s) that must not be de-serialized but rather be 'unbind' and be saved just as unique IDs. More... | |
void | UnbindExternalPointer (std::shared_ptr< void > mptr, size_t ID) |
Use the following to declare pointer(s) that must not be de-serialized but rather be 'unbind' and be saved just as unique IDs. More... | |
virtual void | out (ChNameValue< bool > bVal)=0 |
virtual void | out (ChNameValue< int > bVal)=0 |
virtual void | out (ChNameValue< double > bVal)=0 |
virtual void | out (ChNameValue< float > bVal)=0 |
virtual void | out (ChNameValue< char > bVal)=0 |
virtual void | out (ChNameValue< unsigned int > bVal)=0 |
virtual void | out (ChNameValue< std::string > bVal)=0 |
virtual void | out (ChNameValue< unsigned long > bVal)=0 |
virtual void | out (ChNameValue< unsigned long long > bVal)=0 |
virtual void | out (ChNameValue< ChEnumMapperBase > bVal)=0 |
virtual void | out (ChValue &bVal, bool tracked, size_t obj_ID)=0 |
virtual void | out_ref (ChValue &bVal, bool already_inserted, size_t obj_ID, size_t ext_ID)=0 |
virtual void | out_array_pre (ChValue &bVal, size_t msize)=0 |
virtual void | out_array_between (ChValue &bVal, size_t msize)=0 |
virtual void | out_array_end (ChValue &bVal, size_t msize)=0 |
template<class T > | |
void | out (ChNameValue< ChEnumMapper< T > > bVal) |
template<class T , size_t N> | |
void | out (ChNameValue< T[N]> bVal) |
template<class T > | |
void | out (ChNameValue< std::vector< T > > bVal) |
template<class T > | |
void | out (ChNameValue< std::list< T > > bVal) |
template<class T , class Tv > | |
void | out (ChNameValue< std::pair< T, Tv > > bVal) |
template<class T , class Tv > | |
void | out (ChNameValue< std::unordered_map< T, Tv > > bVal) |
template<class T > | |
void | out (ChNameValue< std::shared_ptr< T > > bVal) |
template<class T > | |
void | out (ChNameValue< T * > bVal) |
template<class T > | |
void | out (ChNameValue< T > bVal) |
template<class T > | |
ChArchiveOut & | operator<< (ChNameValue< T > bVal) |
Operator to allow easy serialization as myarchive << mydata;. | |
void | VersionWrite (int iv) |
template<class T > | |
void | VersionWrite () |
![]() | |
void | SetUseVersions (bool muse) |
By default, version numbers are saved in archives Use this to turn off version info in archives (either save/load both with version info, or not, do not mix because it could give problems in binary archives.). | |
void | SetClusterClassVersions (bool mcl) |
If true, the version number is not saved in each class: rather, it is saved only the first time that class is encountered. More... | |
Protected Member Functions | |
void | PutPointer (void *object, bool &already_stored, size_t &obj_ID) |
Find a pointer in pointer map: eventually add it to map if it was not previously inserted. More... | |
virtual void | out_version (int mver, const std::type_info &mtype) |
Protected Attributes | |
std::unordered_map< void *, size_t > | internal_ptr_id |
size_t | currentID |
std::unordered_map< void *, size_t > | external_ptr_id |
std::unordered_set< void * > | cut_pointers |
bool | cut_all_pointers |
![]() | |
bool | cluster_class_versions |
std::unordered_map< std::type_index, int > | class_versions |
bool | use_versions |
Member Function Documentation
◆ CutPointers()
|
inline |
Access the container of pointers that must not be serialized.
This is in case SetCutAllPointers(true) is too extreme. So you can selectively 'cut' the network of pointers when serializing an object that has a network of sub objects. Works also for shared pointers, but remember to store the embedded pointer, not the shared pointer itself. For instance: myarchive.CutPointers().insert(my_raw_pointer); // normal pointers myarchive.CutPointers().insert(my_shared_pointer.get()); // shared pointers The cut pointers are serialized as null pointers.
◆ PutPointer()
|
inlineprotected |
Find a pointer in pointer map: eventually add it to map if it was not previously inserted.
Returns already_stored=false if was already inserted. Return 'obj_ID' offset in vector in any case. For null pointers, always return 'already_stored'=true, and 'obj_ID'=0.
◆ SetCutAllPointers()
|
inline |
If you enable SetCutAllPointers(true), no serialization happens for objects referenced via pointers.
This can be useful to save a single object, regardless of the fact that it contains pointers to other 'children' objects. Cut pointers are turned into null pointers.
◆ UnbindExternalPointer() [1/2]
|
inline |
Use the following to declare pointer(s) that must not be de-serialized but rather be 'unbind' and be saved just as unique IDs.
Note, the IDs can be whatever integer > 0. Use unique IDs per each pointer. Note, the same IDs must be used when de-serializing pointers in ArchiveIN.
◆ UnbindExternalPointer() [2/2]
|
inline |
Use the following to declare pointer(s) that must not be de-serialized but rather be 'unbind' and be saved just as unique IDs.
Note, the IDs can be whatever integer > 0. Use unique IDs per each pointer. Note, the same IDs must be used when de-serializing pointers in ArchiveIN.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono/serialization/ChArchive.h