Description
Base class for representing objects that introduce 'variables' (also referred as 'v') and their associated mass submatrices for a sparse representation of the problem.
See ChSystemDescriptor for more information about the overall problem and data representation.
Each ChVariables object must be able to compute a mass submatrix, that will be assembled directly inside the global matrix Z, (in particular inside the block H or M). Because of this there is no need for ChVariables and derived classes to actually store their mass submatrix in memory: they just need to be able to compute it!
Moreover, in some cases, the mass submatrix is not even needed. In fact, some Chrono solvers are matrix-free. This means that each ChVariables (and derived) object can be asked not to assemble its mass submatrix, but instead to provide operation related to it. E.g. M*x, M\x, +=M*x, and so on... Each derived class must implement these methods!
Because of this, the ChVariables class does not include any mass submatrix by default
#include <ChVariables.h>
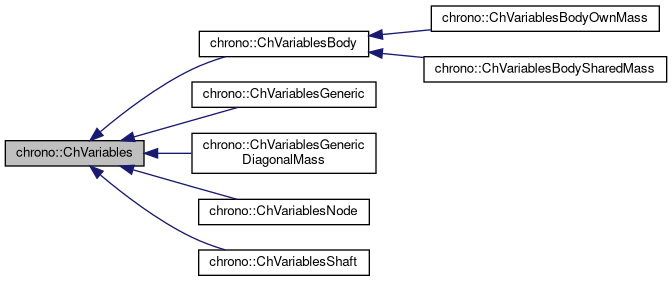
Public Member Functions | |
ChVariables (int m_ndof) | |
ChVariables & | operator= (const ChVariables &other) |
Assignment operator: copy from other object. | |
void | SetDisabled (bool mdis) |
Deactivates/freezes the variable (these variables won't be modified by the system solver). | |
bool | IsDisabled () const |
Check if the variables have been deactivated (these variables won't be modified by the system solver). | |
bool | IsActive () const |
Check if these variables are currently active. More... | |
virtual int | Get_ndof () const |
The number of scalar variables in the vector qb (dof=degrees of freedom) *** This function MUST BE OVERRIDDEN by specialized inherited classes. | |
ChVectorRef | Get_qb () |
Returns reference to qb, body-relative part of degrees of freedom q in system: More... | |
virtual void | Compute_fb () |
Compute fb, body-relative part of known vector f in system. More... | |
ChVectorRef | Get_fb () |
Returns reference to fb, body-relative part of known vector f in system. More... | |
virtual void | Compute_invMb_v (ChVectorRef result, ChVectorConstRef vect) const =0 |
Computes the product of the inverse mass matrix by a vector, and store in result: result = [invMb]*vect. | |
virtual void | Compute_inc_invMb_v (ChVectorRef result, ChVectorConstRef vect) const =0 |
Computes the product of the inverse mass matrix by a vector, and increment result: result += [invMb]*vect. | |
virtual void | Compute_inc_Mb_v (ChVectorRef result, ChVectorConstRef vect) const =0 |
Computes the product of the mass matrix by a vector, and increment result: result = [Mb]*vect. | |
virtual void | MultiplyAndAdd (ChVectorRef result, ChVectorConstRef vect, const double c_a) const =0 |
Computes the product of the corresponding block in the system matrix (ie. More... | |
virtual void | DiagonalAdd (ChVectorRef result, const double c_a) const =0 |
Add the diagonal of the mass matrix scaled by c_a, to 'result', as a vector. More... | |
virtual void | Build_M (ChSparseMatrix &storage, int insrow, int inscol, const double c_a)=0 |
Build the mass submatrix (for these variables) multiplied by c_a, storing it in 'storage' sparse matrix, at given column/row offset. More... | |
void | SetOffset (int moff) |
Set offset in global q vector (set automatically by ChSystemDescriptor) | |
int | GetOffset () const |
Get offset in global q vector. | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) |
virtual void | ArchiveIN (ChArchiveIn &marchive) |
Protected Attributes | |
int | offset |
offset in global q state vector (needed by some solvers) | |
Member Function Documentation
◆ Build_M()
|
pure virtual |
Build the mass submatrix (for these variables) multiplied by c_a, storing it in 'storage' sparse matrix, at given column/row offset.
Most iterative solvers don't need to know this matrix explicitly. *** This function MUST BE OVERRIDDEN by specialized inherited classes
Implemented in chrono::ChVariablesBodySharedMass, chrono::ChVariablesShaft, chrono::ChVariablesBodyOwnMass, chrono::ChVariablesGeneric, chrono::ChVariablesNode, and chrono::ChVariablesGenericDiagonalMass.
◆ Compute_fb()
|
inlinevirtual |
Compute fb, body-relative part of known vector f in system.
*** This function MAY BE OVERRIDDEN by specialized inherited classes (e.g., for impulsive multibody simulation, this may be fb = dt*Forces+[M]*previous_v ). Another option is to set values into fb vectors, accessing them by Get_fb() from an external procedure, for each body, before starting the solver.
◆ DiagonalAdd()
|
pure virtual |
Add the diagonal of the mass matrix scaled by c_a, to 'result', as a vector.
NOTE: the 'result' vector must already have the size of system unknowns, ie the size of the total variables & constraints in the system; the procedure will use the ChVariable offset (that must be already updated) as index.
Implemented in chrono::ChVariablesBodySharedMass, chrono::ChVariablesShaft, chrono::ChVariablesBodyOwnMass, chrono::ChVariablesGeneric, chrono::ChVariablesNode, and chrono::ChVariablesGenericDiagonalMass.
◆ Get_fb()
|
inline |
Returns reference to fb, body-relative part of known vector f in system.
| M -Cq'|*|q|- | f|= |0| , c>0, l>0, l*r=0; | Cq 0 | |l| |-b| |c|
This function can be used to set values of fb vector before starting the solver.
◆ Get_qb()
|
inline |
Returns reference to qb, body-relative part of degrees of freedom q in system:
| M -Cq'|*|q|- | f|= |0| , c>0, l>0, l*r=0; | Cq 0 | |l| |-b| |c|
◆ IsActive()
|
inline |
Check if these variables are currently active.
In general, tells if they must be included into the system solver or not.
◆ MultiplyAndAdd()
|
pure virtual |
Computes the product of the corresponding block in the system matrix (ie.
the mass matrix) by 'vect', scale by c_a, and add to 'result'. NOTE: the 'vect' and 'result' vectors must already have the size of the total variables&constraints in the system; the procedure will use the ChVariable offset (that must be already updated) to know the indexes in result and vect.
Implemented in chrono::ChVariablesBodySharedMass, chrono::ChVariablesShaft, chrono::ChVariablesBodyOwnMass, chrono::ChVariablesGeneric, chrono::ChVariablesNode, and chrono::ChVariablesGenericDiagonalMass.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChVariables.h
- /builds/uwsbel/chrono/src/chrono/solver/ChVariables.cpp