Description
Specialized class for representing a 6-DOF item for a system, that is a 3D rigid body, with mass matrix and associate variables (a 6 element vector, ex.speed) Differently from the 'naive' implementation ChVariablesGeneric, here a full 6x6 mass matrix is not built, since only the 3x3 inertia matrix and the mass value are enough.
This is very similar to ChVariablesBodyOwnMass, but the mass and inertia values are shared, that can be useful for problems with thousands of equally-shaped objects.
#include <ChVariablesBodySharedMass.h>
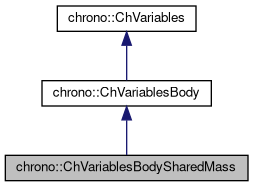
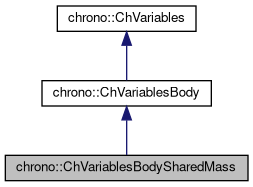
Public Member Functions | |
ChVariablesBodySharedMass & | operator= (const ChVariablesBodySharedMass &other) |
Assignment operator: copy from other object. | |
ChSharedMassBody * | GetSharedMass () |
Get the pointer to shared mass. | |
void | SetSharedMass (ChSharedMassBody *ms) |
Set pointer to shared mass. | |
virtual double | GetBodyMass () const override |
Get the mass associated with translation of body. | |
virtual ChMatrix33 & | GetBodyInertia () override |
Access the 3x3 inertia matrix. | |
virtual const ChMatrix33 & | GetBodyInertia () const override |
virtual ChMatrix33 & | GetBodyInvInertia () override |
Access the 3x3 inertia matrix inverted. | |
virtual const ChMatrix33 & | GetBodyInvInertia () const override |
virtual void | Compute_invMb_v (ChVectorRef result, ChVectorConstRef vect) const override |
Computes the product of the inverse mass matrix by a vector, and set in result: result = [invMb]*vect. | |
virtual void | Compute_inc_invMb_v (ChVectorRef result, ChVectorConstRef vect) const override |
Computes the product of the inverse mass matrix by a vector, and increment result: result += [invMb]*vect. | |
virtual void | Compute_inc_Mb_v (ChVectorRef result, ChVectorConstRef vect) const override |
Computes the product of the mass matrix by a vector, and set in result: result = [Mb]*vect. | |
virtual void | MultiplyAndAdd (ChVectorRef result, ChVectorConstRef vect, const double c_a) const override |
Computes the product of the corresponding block in the system matrix (ie. More... | |
virtual void | DiagonalAdd (ChVectorRef result, const double c_a) const override |
Add the diagonal of the mass matrix scaled by c_a, to 'result'. More... | |
virtual void | Build_M (ChSparseMatrix &storage, int insrow, int inscol, const double c_a) override |
Build the mass matrix (for these variables) scaled by c_a, storing it in 'storage' sparse matrix, at given column/row offset. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChVariablesBody & | operator= (const ChVariablesBody &other) |
Assignment operator: copy from other object. | |
virtual int | Get_ndof () const override |
The number of scalar variables in the vector qb (dof=degrees of freedom) | |
void * | GetUserData () |
void | SetUserData (void *mdata) |
![]() | |
ChVariables (int m_ndof) | |
ChVariables & | operator= (const ChVariables &other) |
Assignment operator: copy from other object. | |
void | SetDisabled (bool mdis) |
Deactivates/freezes the variable (these variables won't be modified by the system solver). | |
bool | IsDisabled () const |
Check if the variables have been deactivated (these variables won't be modified by the system solver). | |
bool | IsActive () const |
Check if these variables are currently active. More... | |
ChVectorRef | Get_qb () |
Returns reference to qb, body-relative part of degrees of freedom q in system: More... | |
virtual void | Compute_fb () |
Compute fb, body-relative part of known vector f in system. More... | |
ChVectorRef | Get_fb () |
Returns reference to fb, body-relative part of known vector f in system. More... | |
void | SetOffset (int moff) |
Set offset in global q vector (set automatically by ChSystemDescriptor) | |
int | GetOffset () const |
Get offset in global q vector. | |
Additional Inherited Members | |
![]() | |
int | offset |
offset in global q state vector (needed by some solvers) | |
Member Function Documentation
◆ Build_M()
|
overridevirtual |
Build the mass matrix (for these variables) scaled by c_a, storing it in 'storage' sparse matrix, at given column/row offset.
Note, most iterative solvers don't need to know mass matrix explicitly. Optimized: doesn't fill unneeded elements except mass and 3x3 inertia.
Implements chrono::ChVariables.
◆ DiagonalAdd()
|
overridevirtual |
Add the diagonal of the mass matrix scaled by c_a, to 'result'.
NOTE: the 'result' vector must already have the size of system unknowns, ie the size of the total variables & constraints in the system; the procedure will use the ChVariable offset (that must be already updated) as index.
Implements chrono::ChVariables.
◆ MultiplyAndAdd()
|
overridevirtual |
Computes the product of the corresponding block in the system matrix (ie.
the mass matrix) by 'vect', scale by c_a, and add to 'result'. NOTE: the 'vect' and 'result' vectors must already have the size of the total variables&constraints in the system; the procedure will use the ChVariable offsets (that must be already updated) to know the indexes in result and vect.
Implements chrono::ChVariables.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChVariablesBodySharedMass.h
- /builds/uwsbel/chrono/src/chrono/solver/ChVariablesBodySharedMass.cpp