Description
Class for one-degree-of-freedom mechanical parts with associated inertia (mass, or J moment of inertial for rotating parts).
In most cases these represent shafts that can be used to build 1D models of power trains. This is more efficient than simulating power trains modeled with full 3D ChBody objects.
#include <ChShaft.h>
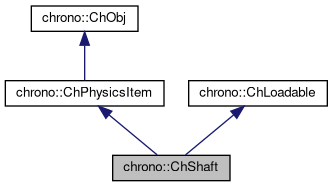
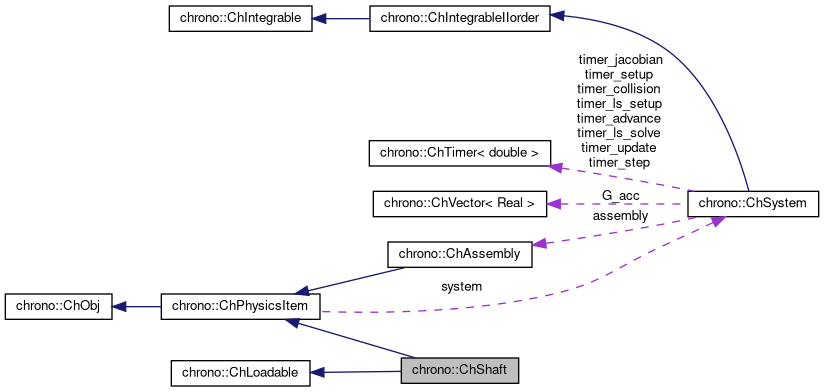
Public Member Functions | |
ChShaft (const ChShaft &other) | |
virtual ChShaft * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
void | SetShaftFixed (bool mev) |
Sets the 'fixed' state of the shaft. More... | |
bool | GetShaftFixed () const |
void | SetLimitSpeed (bool mlimit) |
Trick. More... | |
bool | GetLimitSpeed () const |
void | SetUseSleeping (bool ms) |
Trick. More... | |
bool | GetUseSleeping () const |
void | SetSleeping (bool ms) |
Force the shaft in sleeping mode or not (usually this state change is not handled by users, anyway, because it is mostly automatic). | |
bool | GetSleeping () const |
Tell if the shaft is actually in sleeping state. | |
bool | TrySleeping () |
Put the shaft in sleeping state if requirements are satisfied. | |
bool | IsActive () const |
Tell if the body is active, i.e. More... | |
void | SetId (unsigned int identifier) |
Set the shaft id for indexing (only used internally) | |
unsigned int | GetId () const |
Get the shaft id for indexing (only used internally) | |
virtual int | GetDOF () override |
Number of coordinates of the shaft. | |
ChVariablesShaft & | Variables () |
Returns reference to the encapsulated ChVariables,. | |
virtual void | IntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) override |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets. More... | |
virtual void | IntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T, bool full_update) override |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets. More... | |
virtual void | IntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) override |
From item's state acceleration to global acceleration vector. More... | |
virtual void | IntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) override |
From global acceleration vector to item's state acceleration. More... | |
virtual void | IntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) override |
Takes the F force term, scale and adds to R at given offset: R += c*F. More... | |
virtual void | IntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w. More... | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) override |
Prepare variables and constraints to accommodate a solution: More... | |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) override |
After a solver solution, fetch values from variables and constraints into vectors: More... | |
virtual void | VariablesFbReset () override |
Sets the 'fb' part of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) override |
Adds the current torques in the 'fb' part: qf+=torques*factor. | |
virtual void | VariablesQbLoadSpeed () override |
Initialize the 'qb' part of the ChVariables with the current value of shaft speed. More... | |
virtual void | VariablesFbIncrementMq () override |
Adds M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) override |
Fetches the shaft speed from the 'qb' part of the ChVariables (does not updates the full shaft state) and sets it as the current shaft speed. More... | |
virtual void | VariablesQbIncrementPosition (double step) override |
Increment shaft position by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | InjectVariables (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are variables of type ChVariables in this object (for further passing it to a solver) | |
virtual int | LoadableGet_ndof_x () override |
Gets the number of DOFs affected by this element (position part) | |
virtual int | LoadableGet_ndof_w () override |
Gets the number of DOFs affected by this element (speed part) | |
virtual void | LoadableGetStateBlock_x (int block_offset, ChState &mD) override |
Gets all the DOFs packed in a single vector (position part) | |
virtual void | LoadableGetStateBlock_w (int block_offset, ChStateDelta &mD) override |
Gets all the DOFs packed in a single vector (speed part) | |
virtual void | LoadableStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Increment all DOFs using a delta. More... | |
virtual int | Get_field_ncoords () override |
Number of coordinates in the interpolated field (e.g., 3 for a tetrahedron, 1 for a thermal problem, etc.). | |
virtual int | GetSubBlocks () override |
Get the number of DOFs sub-blocks (e.g., 1 for a body, 4 for a tetrahedron, etc.). | |
virtual unsigned int | GetSubBlockOffset (int nblock) override |
Get the offset of the specified sub-block of DOFs in global vector. | |
virtual unsigned int | GetSubBlockSize (int nblock) override |
Get the size of the specified sub-block of DOFs in global vector. | |
virtual bool | IsSubBlockActive (int nblock) const override |
Check if the specified sub-block of DOFs is active. | |
virtual void | LoadableGetVariables (std::vector< ChVariables * > &mvars) override |
Get the pointers to the contained ChVariables, appending to the mvars vector. | |
void | SetNoSpeedNoAcceleration () override |
Set no speed and no accelerations (but does not change the position) | |
void | SetAppliedTorque (double mtorque) |
Set the torque applied to the shaft. | |
double | GetAppliedTorque () const |
Get the torque applied to the shaft. | |
void | SetPos (double mp) |
Set the angular position. | |
double | GetPos () const |
Get the angular position. | |
void | SetPos_dt (double mp) |
Set the angular velocity. | |
double | GetPos_dt () const |
Get the angular velocity. | |
void | SetPos_dtdt (double mp) |
Set the angular acceleration. | |
double | GetPos_dtdt () const |
Get the angular acceleration. | |
void | SetInertia (double newJ) |
Inertia of the shaft. More... | |
double | GetInertia () const |
void | SetMaxSpeed (float m_max_speed) |
Trick. More... | |
float | GetMaxSpeed () const |
void | ClampSpeed () |
When this function is called, the speed of the shaft is clamped into limits posed by max_speed and max_wvel - but remember to put the shaft in the SetLimitSpeed(true) mode. | |
void | SetSleepTime (float m_t) |
Set the amount of time which must pass before going automatically in sleep mode when the shaft has very small movements. | |
float | GetSleepTime () const |
void | SetSleepMinSpeed (float m_t) |
Set the max linear speed to be kept for 'sleep_time' before freezing. | |
float | GetSleepMinSpeed () const |
void | SetSleepMinWvel (float m_t) |
Set the max linear speed to be kept for 'sleep_time' before freezing. | |
float | GetSleepMinWvel () const |
virtual void | Update (double mytime, bool update_assets=true) override |
Update all auxiliary data of the shaft at given time. | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. More... | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem() | |
virtual void | SetSystem (ChSystem *m_system) |
Set the pointer to the parent ChSystem() and also add to new collision system / remove from old coll.system. | |
void | AddAsset (std::shared_ptr< ChAsset > masset) |
Add an optional asset (it can be used to define visualization shapes, es ChSphereShape, or textures, or custom attached properties that the user can define by creating his class inherited from ChAsset) | |
std::vector< std::shared_ptr< ChAsset > > & | GetAssets () |
Access to the list of optional assets. | |
std::shared_ptr< ChAsset > | GetAssetN (unsigned int num) |
Access the Nth asset in the list of optional assets. | |
virtual ChFrame | GetAssetsFrame (unsigned int nclone=0) |
Get the master coordinate system for assets that have some geometric meaning. More... | |
virtual unsigned int | GetAssetsFrameNclones () |
Optionally, a ChPhysicsItem can return multiple asset coordinate systems; this can be helpful if, for example, when a ChPhysicsItem contains 'clones' with the same assets (ex. More... | |
virtual bool | GetCollide () const |
Tell if the object is subject to collision. More... | |
virtual void | SyncCollisionModels () |
If this physical item contains one or more collision models, synchronize their coordinates and bounding boxes to the state of the item. | |
virtual void | AddCollisionModelsToSystem () |
If this physical item contains one or more collision models, add them to the system's collision engine. | |
virtual void | RemoveCollisionModelsFromSystem () |
If this physical item contains one or more collision models, remove them from the system's collision engine. | |
virtual void | GetTotalAABB (ChVector<> &bbmin, ChVector<> &bbmax) |
Get the entire AABB axis-aligned bounding box of the object. More... | |
virtual void | GetCenter (ChVector<> &mcenter) |
Get a symbolic 'center' of the object. More... | |
virtual void | StreamINstate (ChStreamInBinary &mstream) |
Method to deserialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | StreamOUTstate (ChStreamOutBinary &mstream) |
Method to serialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | Setup () |
This might recompute the number of coordinates, DOFs, constraints, in case this might change (ex in ChAssembly), as well as state offsets of contained items (ex in ChMesh) | |
virtual void | Update (bool update_assets=true) |
As above, but does not require updating of time-dependent data. More... | |
virtual int | GetDOF_w () |
Get the number of scalar coordinates of variables derivatives (usually = DOF, but might be different than DOF, ex. More... | |
virtual int | GetDOC () |
Get the number of scalar constraints, if any, in this item. | |
virtual int | GetDOC_c () |
Get the number of scalar constraints, if any, in this item (only bilateral constr.) Children classes might override this. | |
virtual int | GetDOC_d () |
Get the number of scalar constraints, if any, in this item (only unilateral constr.) Children classes might override this. | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
virtual void | IntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) |
From item's reaction forces to global reaction vector. More... | |
virtual void | IntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) |
From global reaction vector to item's reaction forces. More... | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L. More... | |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | IntLoadConstraint_Ct (const unsigned int off, ChVectorDynamic<> &Qc, const double c) |
Takes the term Ct, scale and adds to Qc at given offset: Qc += c*Ct. More... | |
virtual void | InjectConstraints (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are constraints of type ChConstraint in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | ConstraintsBiReset () |
Sets to zero the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) |
Adds the current C (constraint violation) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Ct (double factor=1) |
Adds the current Ct (partial t-derivative, as in C_dt=0-> [Cq]*q_dt=-Ct) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsFbLoadForces (double factor=1) |
Adds the current link-forces, if any, (caused by springs, etc.) to the 'fb' vectors of the ChVariables referenced by encapsulated ChConstraints. | |
virtual void | ConstraintsLoadJacobians () |
Adds the current jacobians in encapsulated ChConstraints. | |
virtual void | ConstraintsFetch_react (double factor=1) |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints. More... | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are items of type ChKblock in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Gets the numerical identifier of the object. | |
void | SetIdentifier (int id) |
Sets the numerical identifier of the object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
const char * | GetName () const |
Gets the name of the object as C Ascii null-terminated string -for reading only! | |
void | SetName (const char myname[]) |
Sets the name of this object, as ascii string. | |
std::string | GetNameString () const |
Gets the name of the object as C Ascii null-terminated string. | |
void | SetNameString (const std::string &myname) |
Sets the name of this object, as std::string. | |
void | MFlagsSetAllOFF (int &mflag) |
void | MFlagsSetAllON (int &mflag) |
void | MFlagSetON (int &mflag, int mask) |
void | MFlagSetOFF (int &mflag, int mask) |
int | MFlagGet (int &mflag, int mask) |
virtual std::string & | ArchiveContainerName () |
Additional Inherited Members | |
![]() | |
ChSystem * | system |
parent system | |
std::vector< std::shared_ptr< ChAsset > > | assets |
set of assets | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
the time of simulation for the object | |
Member Function Documentation
◆ ArchiveIN()
|
overridevirtual |
Method to allow deserialization of transient data from archives.
Method to allow de serialization of transient data from archives.
Reimplemented from chrono::ChPhysicsItem.
◆ IntFromDescriptor()
|
overridevirtual |
After a solver solution, fetch values from variables and constraints into vectors:
- Parameters
-
off_v offset for v v vector to where the q 'unknowns' term of the variables will be copied off_L offset for L L vector to where L 'lagrangian ' term of the constraints will be copied
Reimplemented from chrono::ChPhysicsItem.
◆ IntLoadResidual_F()
|
overridevirtual |
Takes the F force term, scale and adds to R at given offset: R += c*F.
- Parameters
-
off offset in R residual R result: the R residual, R += c*F c a scaling factor
Reimplemented from chrono::ChPhysicsItem.
◆ IntLoadResidual_Mv()
|
overridevirtual |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w.
- Parameters
-
off offset in R residual R result: the R residual, R += c*M*v w the w vector c a scaling factor
Reimplemented from chrono::ChPhysicsItem.
◆ IntStateGather()
|
overridevirtual |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets.
- Parameters
-
off_x offset in x state vector x state vector, position part off_v offset in v state vector v state vector, speed part T time
Reimplemented from chrono::ChPhysicsItem.
◆ IntStateGatherAcceleration()
|
overridevirtual |
From item's state acceleration to global acceleration vector.
- Parameters
-
off_a offset in a accel. vector a acceleration part of state vector derivative
Reimplemented from chrono::ChPhysicsItem.
◆ IntStateScatter()
|
overridevirtual |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets.
- Parameters
-
off_x offset in x state vector x state vector, position part off_v offset in v state vector v state vector, speed part T time full_update perform complete update
Reimplemented from chrono::ChPhysicsItem.
◆ IntStateScatterAcceleration()
|
overridevirtual |
From global acceleration vector to item's state acceleration.
- Parameters
-
off_a offset in a accel. vector a acceleration part of state vector derivative
Reimplemented from chrono::ChPhysicsItem.
◆ IntToDescriptor()
|
overridevirtual |
Prepare variables and constraints to accommodate a solution:
- Parameters
-
off_v offset for v and R v vector that will be copied into the q 'unknowns' term of the variables (for warm starting) R vector that will be copied into the F 'force' term of the variables off_L offset for L and Qc L vector that will be copied into the L 'lagrangian ' term of the constraints (for warm starting) Qc vector that will be copied into the Qb 'constraint' term of the constraints
Reimplemented from chrono::ChPhysicsItem.
◆ IsActive()
|
inline |
Tell if the body is active, i.e.
it is neither fixed to ground nor it is in sleep mode.
◆ LoadableStateIncrement()
|
inlineoverridevirtual |
Increment all DOFs using a delta.
Default is sum, but may override if ndof_x is different than ndof_w, for example with rotation quaternions and angular w vel. This could be invoked, for example, by the BDF differentiation that computes the jacobians.
Implements chrono::ChLoadable.
◆ SetInertia()
void chrono::ChShaft::SetInertia | ( | double | newJ | ) |
Inertia of the shaft.
Must be positive. Try not to mix bodies with too high/too low values of mass, for numerical stability.
◆ SetLimitSpeed()
|
inline |
Trick.
Set the maximum shaft velocity (beyond this limit it will be clamped). This is useful in virtual reality and real-time simulations. The realism is limited, but the simulation is more stable.
◆ SetMaxSpeed()
|
inline |
Trick.
Set the maximum velocity (beyond this limit it will be clamped). This is useful in virtual reality and real-time simulations, to increase robustness at the cost of realism. This limit is active only if you set SetLimitSpeed(true);
◆ SetShaftFixed()
|
inline |
Sets the 'fixed' state of the shaft.
If true, it does not rotate despite constraints, forces, etc.
◆ SetUseSleeping()
|
inline |
Trick.
If use sleeping= true, shafts which do not rotate for too long time will be deactivated, for optimization. The realism is limited, but the simulation is faster.
◆ VariablesFbIncrementMq()
|
overridevirtual |
Adds M*q (masses multiplied current 'qb') to Fb, ex.
if qb is initialized with v_old using VariablesQbLoadSpeed, this method can be used in timestepping schemes that do: M*v_new = M*v_old + forces*dt
Reimplemented from chrono::ChPhysicsItem.
◆ VariablesQbIncrementPosition()
|
overridevirtual |
Increment shaft position by the 'qb' part of the ChVariables, multiplied by a 'step' factor.
pos+=qb*step
Reimplemented from chrono::ChPhysicsItem.
◆ VariablesQbLoadSpeed()
|
overridevirtual |
Initialize the 'qb' part of the ChVariables with the current value of shaft speed.
Note: since 'qb' is the unknown , this function seems unnecessary, unless used before VariablesFbIncrementMq()
Reimplemented from chrono::ChPhysicsItem.
◆ VariablesQbSetSpeed()
|
overridevirtual |
Fetches the shaft speed from the 'qb' part of the ChVariables (does not updates the full shaft state) and sets it as the current shaft speed.
If 'step' is not 0, also computes the approximate acceleration of the shaft using backward differences, that is accel=(new_speed-old_speed)/step.
Reimplemented from chrono::ChPhysicsItem.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChShaft.h
- /builds/uwsbel/chrono/src/chrono/physics/ChShaft.cpp