chrono::vehicle::citybus::CityBus_Pac02Tire Class Reference
Description
PAC89 tire model for the CityBus vehicle.
#include <CityBus_Pac02Tire.h>
Inheritance diagram for chrono::vehicle::citybus::CityBus_Pac02Tire:
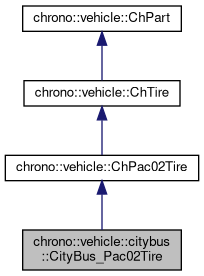
Collaboration diagram for chrono::vehicle::citybus::CityBus_Pac02Tire:
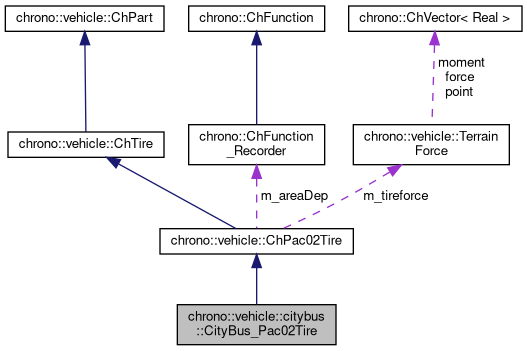
Public Member Functions | |
CityBus_Pac02Tire (const std::string &name) | |
virtual double | GetNormalStiffnessForce (double depth) const override |
Return the vertical tire stiffness contribution to the normal force. | |
virtual double | GetNormalDampingForce (double depth, double velocity) const override |
Return the vertical tire damping contribution to the normal force. | |
virtual double | GetMass () const override |
Get the tire mass. More... | |
virtual ChVector | GetInertia () const override |
Get the tire moments of inertia. More... | |
virtual double | GetVisualizationWidth () const override |
Get visualization width. | |
virtual void | SetPac02Params () override |
Set the parameters in the Pac89 model. | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the rigid tire subsystem. | |
virtual void | RemoveVisualizationAssets () override final |
Remove visualization assets for the rigid tire subsystem. | |
![]() | |
ChPac02Tire (const std::string &name) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual double | GetRadius () const override |
Get the tire radius. | |
virtual TerrainForce | ReportTireForce (ChTerrain *terrain) const override |
Report the tire force and moment. | |
void | SetGammaLimit (double gamma_limit) |
Set the limit for camber angle (in degrees). Default: 3 degrees. | |
virtual double | GetWidth () const override |
Get the width of the tire. | |
virtual double | GetDeflection () const override |
Get the tire deflection. | |
double | GetSlipAngle_internal () const |
Get the slip angle used in Pac89 (expressed in radians). More... | |
double | GetLongitudinalSlip_internal () const |
Get the longitudinal slip used in Pac89. More... | |
double | GetCamberAngle_internal () |
Get the camber angle used in Pac89 (expressed in radians). More... | |
![]() | |
ChTire (const std::string &name) | |
void | SetStepsize (double val) |
Set the value of the integration step size for the underlying dynamics (if applicable). More... | |
double | GetStepsize () const |
Get the current value of the integration step size. | |
void | SetCollisionType (CollisionType collision_type) |
Set the collision type for tire-terrain interaction. More... | |
virtual double | ReportMass () const |
Report the tire mass. More... | |
double | GetSlipAngle () const |
Return the tire slip angle calculated based on the current state of the associated wheel body. More... | |
double | GetLongitudinalSlip () const |
Return the tire longitudinal slip calculated based on the current state of the associated wheel body. More... | |
double | GetCamberAngle () const |
Return the tire camber angle calculated based on the current state of the associated wheel body. More... | |
const std::string & | GetMeshFilename () const |
Get the name of the Wavefront file with tire visualization mesh. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Additional Inherited Members | |
![]() | |
enum | CollisionType { SINGLE_POINT, FOUR_POINTS, ENVELOPE } |
![]() | |
static ChVector | EstimateInertia (double tire_width, double aspect_ratio, double rim_diameter, double tire_mass, double t_factor=2) |
Utility function for estimating the tire moments of inertia. More... | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
virtual TerrainForce | GetTireForce () const override |
Get the tire force and moment. More... | |
virtual void | Initialize (std::shared_ptr< ChWheel > wheel) override |
Initialize this tire by associating it to the specified wheel. | |
virtual void | Synchronize (double time, const ChTerrain &terrain) override |
Update the state of this tire system at the current time. More... | |
virtual void | Advance (double step) override |
Advance the state of this tire by the specified time step. | |
double | CalcFx (double kappa, double Fz, double gamma) |
double | CalcFy (double alpha, double Fz, double gamma) |
double | CalcMx (double Fy, double Fz, double gamma) |
double | CalcMy (double Fx, double Fy, double gamma) |
double | CalcMz (double alpha, double Fz, double gamma, double Fy) |
double | CalcTrail (double alpha, double Fz, double gamma) |
double | CalcMres (double alpha, double Fz, double gamma) |
double | CalcFxComb (double kappa, double alpha, double Fz, double gamma) |
double | CalcFyComb (double kappa, double alpha, double Fz, double gamma) |
double | CalcMzComb (double kappa, double alpha, double Fz, double gamma, double Fx, double Fy) |
![]() | |
void | CalculateKinematics (double time, const WheelState &wheel_state, const ChTerrain &terrain) |
Calculate kinematics quantities based on the given state of the associated wheel body. More... | |
double | GetOffset () const |
Get offset from spindle center. More... | |
std::shared_ptr< ChTriangleMeshShape > | AddVisualizationMesh (const std::string &mesh_file_left, const std::string &mesh_file_right) |
Add mesh visualization to the body associated with this tire (a wheel spindle body). More... | |
void | RemoveVisualizationMesh (std::shared_ptr< ChTriangleMeshShape > trimesh_shape) |
Remove the specified mesh shape from the visualization assets of the body associated with this tire (a wheel spindle body). | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static bool | DiscTerrainCollision (const ChTerrain &terrain, const ChVector<> &disc_center, const ChVector<> &disc_normal, double disc_radius, ChCoordsys<> &contact, double &depth) |
Perform disc-terrain collision detection. More... | |
static bool | DiscTerrainCollision4pt (const ChTerrain &terrain, const ChVector<> &disc_center, const ChVector<> &disc_normal, double disc_radius, double width, ChCoordsys<> &contact, double &depth, double &camber_angle) |
Perform disc-terrain collision detection considering the curvature of the road surface. More... | |
static bool | DiscTerrainCollisionEnvelope (const ChTerrain &terrain, const ChVector<> &disc_center, const ChVector<> &disc_normal, double disc_radius, const ChFunction_Recorder &areaDep, ChCoordsys<> &contact, double &depth) |
Collsion algorithm based on a paper of J. More... | |
static void | ConstructAreaDepthTable (double disc_radius, ChFunction_Recorder &areaDep) |
Utility function to construct a loopkup table for penetration depth as function of intersection area, for a given tire radius. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
double | m_kappa |
longitudinal slip ratio | |
double | m_alpha |
slip angle | |
double | m_gamma |
camber angle | |
double | m_gamma_limit |
limit camber angle | |
bool | m_use_friction_ellipsis |
double | m_mu |
Road friction. | |
double | m_Shf |
double | m_Cy |
double | m_By |
VehicleSide | m_measured_side |
bool | m_allow_mirroring |
unsigned int | m_use_mode |
double | m_kappa_c |
double | m_alpha_c |
double | m_mu_x_act |
double | m_mu_x_max |
double | m_mu_y_act |
double | m_mu_y_max |
Pac02ScalingFactors | m_PacScal |
Pac02Coeff | m_PacCoeff |
ChFunction_Recorder | m_areaDep |
ContactData | m_data |
TireStates | m_states |
TerrainForce | m_tireforce |
std::shared_ptr< ChCylinderShape > | m_cyl_shape |
visualization cylinder asset | |
std::shared_ptr< ChTexture > | m_texture |
visualization texture asset | |
![]() | |
std::shared_ptr< ChWheel > | m_wheel |
associated wheel subsystem | |
double | m_stepsize |
tire integration step size (if applicable) | |
CollisionType | m_collision_type |
method used for tire-terrain collision | |
std::string | m_vis_mesh_file |
name of OBJ file for visualization of this tire (may be empty) | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
Member Function Documentation
◆ GetInertia()
|
inlineoverridevirtual |
Get the tire moments of inertia.
Note that these should not include the inertia of the wheel (rim).
Implements chrono::vehicle::ChTire.
◆ GetMass()
|
inlineoverridevirtual |
Get the tire mass.
Note that this should not include the mass of the wheel (rim).
Implements chrono::vehicle::ChTire.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/citybus/CityBus_Pac02Tire.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/citybus/CityBus_Pac02Tire.cpp