Description
Base class for terrain nodes that use one of the Chrono terrain formulations.
#include <ChVehicleCosimTerrainNodeChrono.h>
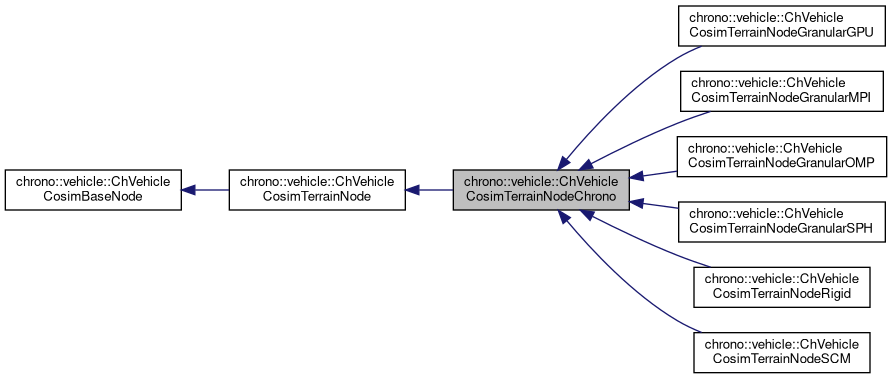
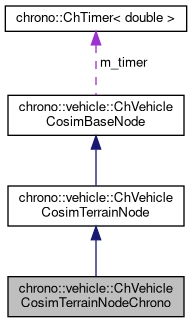
Classes | |
struct | ProxyBody |
Association between a proxy body and a mesh index. More... | |
struct | RigidObstacle |
Specification of a rigid obstacle. More... | |
Public Types | |
enum | Type { Type::RIGID, Type::SCM, Type::GRANULAR_OMP, Type::GRANULAR_GPU, Type::GRANULAR_MPI, Type::GRANULAR_SPH, Type::UNKNOWN } |
Type of Chrono terrain. More... | |
![]() | |
enum | NodeType { NodeType::MBS, NodeType::TERRAIN, NodeType::TIRE } |
Type of node participating in co-simulation. More... | |
enum | InterfaceType { InterfaceType::BODY, InterfaceType::MESH } |
Type of the tire-terrain communication interface. More... | |
Public Member Functions | |
Type | GetType () const |
Return the type of this terrain node. | |
void | SetProxyFixed (bool fixed) |
Set the proxy bodies as fixed to ground. | |
virtual double | GetInitHeight () const override final |
Return the terrain initial height. | |
void | AddRigidObstacle (const RigidObstacle &obstacle) |
Add a rigid obstacle. | |
![]() | |
virtual NodeType | GetNodeType () const override |
Return the node type as NodeType::TERRAIN. | |
void | EnableRuntimeVisualization (bool render, double render_fps=100) |
Enable/disable run-time visualization (default: false). More... | |
void | SetDimensions (double length, double width) |
Set the terrain patch dimensions. More... | |
virtual void | Initialize () override final |
Initialize this node. More... | |
virtual void | Synchronize (int step_number, double time) override final |
Synchronize this node. More... | |
virtual void | Advance (double step_size) override final |
Advance simulation. More... | |
virtual void | OutputData (int frame) override final |
Output logging and debugging data. | |
virtual void | OutputVisualizationData (int frame) override |
Output post-processing visualization data. More... | |
virtual int | GetNumContacts () const |
Return current number of contacts. More... | |
![]() | |
std::string | GetNodeTypeString () const |
Return the node type as a string. | |
bool | IsCosimNode () const |
Return true if this node is part of the co-simulation infrastructure. | |
void | SetStepSize (double step) |
Set the integration step size (default: 1e-4). | |
double | GetStepSize () const |
Get the integration step size. | |
void | SetOutDir (const std::string &dir_name, const std::string &suffix) |
Set the name of the output directory and an identifying suffix. More... | |
void | SetVerbose (bool verbose) |
Enable/disable verbose messages during simulation (default: true). | |
const std::string & | GetOutDirName () const |
Get the output directory name for this node. | |
double | GetStepExecutionTime () const |
Get the simulation execution time for the current step on this node. More... | |
double | GetTotalExecutionTime () const |
Get the cumulative simulation execution time on this node. | |
virtual void | WriteCheckpoint (const std::string &filename) const |
Write checkpoint to the specified file (which will be created in the output directory). | |
Static Public Member Functions | |
static std::string | GetTypeAsString (Type type) |
Return a string describing the type of this terrain node. | |
static Type | GetTypeFromString (const std::string &type) |
Infer the terrain node type from the given string. | |
static bool | ReadSpecfile (const std::string &specfile, rapidjson::Document &d) |
Read a JSON specification file for a Chrono terrain node. | |
static Type | GetTypeFromSpecfile (const std::string &specfile) |
Get the terrain type from the given JSON specification file. | |
static ChVector2 | GetSizeFromSpecfile (const std::string &specfile) |
Get the terrain dimensions (length and width) from the given JSON specification file. | |
![]() | |
static std::string | OutputFilename (const std::string &dir, const std::string &root, const std::string &ext, int frame, int frame_digits) |
Utility function for creating an output file name. More... | |
Protected Types | |
typedef std::vector< ProxyBody > | Proxies |
Protected Member Functions | |
ChVehicleCosimTerrainNodeChrono (Type type, double length, double width, ChContactMethod method) | |
Construct a base class terrain node. More... | |
virtual void | OnInitialize (unsigned int num_tires) override |
Initialize this Chrono terrain node. More... | |
virtual void | OnAdvance (double step_size) override |
Advance simulation. More... | |
virtual ChSystem * | GetSystem ()=0 |
Return a pointer to the underlying Chrono system. | |
virtual void | Construct ()=0 |
Construct the terrain (independent of the vehicle system). | |
virtual void | CreateWheelProxy (unsigned int i)=0 |
Create proxy body for the i-th tire. More... | |
virtual void | CreateMeshProxies (unsigned int i) |
Create proxy bodies for the i-th tire mesh. More... | |
![]() | |
ChVehicleCosimTerrainNode (double length, double width) | |
Construct a terrain node to wrap a terrain patch of given length and width. | |
virtual bool | SupportsMeshInterface () const =0 |
Specify whether or not the terrain node supports the MESH communication interface. More... | |
virtual void | OnSynchronize (int step_number, double time) |
Perform any additional operations after the data exchange and synchronization with the MBS node. More... | |
virtual void | OnOutputData (int frame) |
Perform additional output at the specified frame (called from within OutputData). | |
virtual void | Render (double time) |
Render simulation. More... | |
virtual void | UpdateMeshProxies (unsigned int i, MeshState &mesh_state) |
Update the state of all proxy bodies for the i-th tire mesh. More... | |
virtual void | GetForcesMeshProxies (unsigned int i, MeshContact &mesh_contact) |
Collect cumulative contact forces on all proxy bodies for the i-th tire mesh. More... | |
virtual void | UpdateWheelProxy (unsigned int i, BodyState &spindle_state)=0 |
Update the state of the wheel proxy body for the i-th tire. More... | |
virtual void | GetForceWheelProxy (unsigned int i, TerrainForce &wheel_contact)=0 |
Collect cumulative contact force and torque on the wheel proxy body for the i-th tire. More... | |
![]() | |
ChVehicleCosimBaseNode (const std::string &name) | |
Protected Attributes | |
Type | m_type |
terrain type | |
ChContactMethod | m_method |
contact method (SMC or NSC) | |
std::shared_ptr< ChMaterialSurface > | m_material_terrain |
material properties for terrain bodies | |
double | m_init_height |
terrain initial height | |
std::vector< Proxies > | m_proxies |
proxy bodies for each tire | |
bool | m_fixed_proxies |
are proxy bodies fixed to ground? | |
std::vector< RigidObstacle > | m_obstacles |
list of rigid obstacles | |
![]() | |
bool | m_render |
if true, perform run-time rendering | |
double | m_render_step |
time step between rendered frames | |
double | m_hdimX |
patch half-length (X direction) | |
double | m_hdimY |
patch half-width (Y direction) | |
InterfaceType | m_interface_type |
type of communication interface | |
std::vector< double > | m_tire_radius |
tire radius | |
std::vector< double > | m_tire_width |
tire width | |
std::vector< double > | m_load_mass |
vertical load on tire | |
std::vector< MaterialInfo > | m_mat_props |
tire contact material properties | |
std::vector< MeshData > | m_mesh_data |
tire mesh data | |
std::vector< MeshState > | m_mesh_state |
tire mesh state (used for MESH communication) | |
std::vector< BodyState > | m_spindle_state |
spindle state (used for BODY communication interface) | |
![]() | |
int | m_rank |
MPI rank of this node (in MPI_COMM_WORLD) | |
double | m_step_size |
integration step size | |
std::string | m_name |
name of the node | |
std::string | m_out_dir |
top-level output directory | |
std::string | m_node_out_dir |
node-specific output directory | |
std::ofstream | m_outf |
output file stream | |
unsigned int | m_num_mbs_nodes |
unsigned int | m_num_terrain_nodes |
unsigned int | m_num_tire_nodes |
ChTimer< double > | m_timer |
timer for integration cost | |
double | m_cum_sim_time |
cumulative integration cost | |
bool | m_verbose |
verbose messages during simulation? | |
Additional Inherited Members | |
![]() | |
static const double | m_gacc = -9.81 |
Member Enumeration Documentation
◆ Type
Type of Chrono terrain.
Constructor & Destructor Documentation
◆ ChVehicleCosimTerrainNodeChrono()
|
protected |
Construct a base class terrain node.
- Parameters
-
type terrain type length terrain patch length width terain patch width method contact method (SMC or NSC)
Member Function Documentation
◆ CreateMeshProxies()
|
inlineprotectedvirtual |
Create proxy bodies for the i-th tire mesh.
Use information in the m_mesh_data struct (vertex positions expressed in local frame).
◆ CreateWheelProxy()
|
protectedpure virtual |
Create proxy body for the i-th tire.
Use information in the m_mesh_data struct (vertex positions expressed in local frame).
◆ OnAdvance()
|
overrideprotectedvirtual |
Advance simulation.
This function is called after a synchronization to allow the node to advance its state by the specified time step. A node is allowed to take as many internal integration steps as required, but no inter-node communication should occur.
Implements chrono::vehicle::ChVehicleCosimTerrainNode.
◆ OnInitialize()
|
overrideprotectedvirtual |
Initialize this Chrono terrain node.
Construct the terrain system and the proxy bodies.
Implements chrono::vehicle::ChVehicleCosimTerrainNode.
Reimplemented in chrono::vehicle::ChVehicleCosimTerrainNodeGranularSPH.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/terrain/ChVehicleCosimTerrainNodeChrono.h
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/terrain/ChVehicleCosimTerrainNodeChrono.cpp