Description
Base class for a terrain node.
Implements required functionality for a co-simulation node (Initialize(), Synchronize(), Advance()) and all MPI communication.
A derived class must implement functions to:
- specify the communication interface type (SupportsMeshInterface())
- construct and initialize the concrete terrain object (OnInitialize())
- accept new spindle body states (UpdateWheelProxy() and/or UpdateMeshProxies())
- provide terrain forces acting on the spindle bodies (GetForceWheelProxy() and/or GetForcesMeshProxies())
- advance the dynamic state of the terrain (OnAdvance())
Optionally, a derived class may implement functions to:
- perform additional operations after a synchronization data exchange (OnSynchronize())
- perform additional data output (OnOutputData())
- provide run-time visualization (Render())
#include <ChVehicleCosimTerrainNode.h>
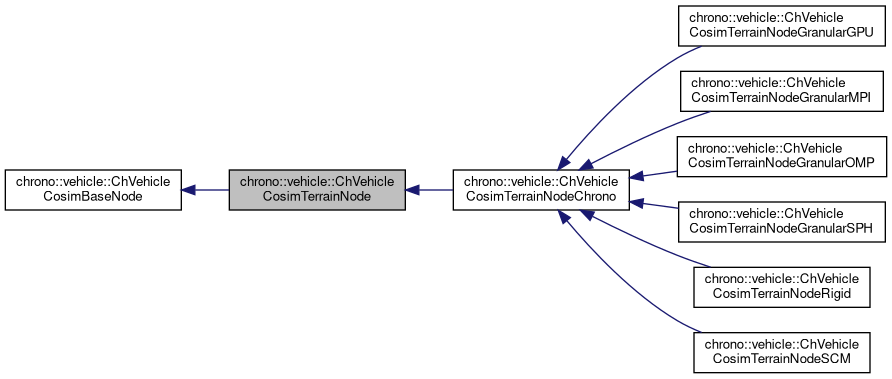
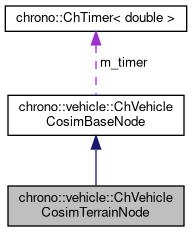
Public Member Functions | |
virtual NodeType | GetNodeType () const override |
Return the node type as NodeType::TERRAIN. | |
void | EnableRuntimeVisualization (bool render, double render_fps=100) |
Enable/disable run-time visualization (default: false). More... | |
void | SetDimensions (double length, double width) |
Set the terrain patch dimensions. More... | |
virtual void | Initialize () override final |
Initialize this node. More... | |
virtual void | Synchronize (int step_number, double time) override final |
Synchronize this node. More... | |
virtual void | Advance (double step_size) override final |
Advance simulation. More... | |
virtual void | OutputData (int frame) override final |
Output logging and debugging data. | |
virtual void | OutputVisualizationData (int frame) override |
Output post-processing visualization data. More... | |
virtual int | GetNumContacts () const |
Return current number of contacts. More... | |
![]() | |
std::string | GetNodeTypeString () const |
Return the node type as a string. | |
bool | IsCosimNode () const |
Return true if this node is part of the co-simulation infrastructure. | |
void | SetStepSize (double step) |
Set the integration step size (default: 1e-4). | |
double | GetStepSize () const |
Get the integration step size. | |
void | SetOutDir (const std::string &dir_name, const std::string &suffix) |
Set the name of the output directory and an identifying suffix. More... | |
void | SetVerbose (bool verbose) |
Enable/disable verbose messages during simulation (default: true). | |
const std::string & | GetOutDirName () const |
Get the output directory name for this node. | |
double | GetStepExecutionTime () const |
Get the simulation execution time for the current step on this node. More... | |
double | GetTotalExecutionTime () const |
Get the cumulative simulation execution time on this node. | |
virtual void | WriteCheckpoint (const std::string &filename) const |
Write checkpoint to the specified file (which will be created in the output directory). | |
Protected Member Functions | |
ChVehicleCosimTerrainNode (double length, double width) | |
Construct a terrain node to wrap a terrain patch of given length and width. | |
virtual bool | SupportsMeshInterface () const =0 |
Specify whether or not the terrain node supports the MESH communication interface. More... | |
virtual double | GetInitHeight () const =0 |
Return the terrain initial height. More... | |
virtual void | OnInitialize (unsigned int num_tires)=0 |
Perform any additional operations after the initial data exchange with the MBS node, including creating any required proxies for the specified number of tires. More... | |
virtual void | OnSynchronize (int step_number, double time) |
Perform any additional operations after the data exchange and synchronization with the MBS node. More... | |
virtual void | OnAdvance (double step_size)=0 |
Advance the state of the terrain system by the specified step. | |
virtual void | OnOutputData (int frame) |
Perform additional output at the specified frame (called from within OutputData). | |
virtual void | Render (double time) |
Render simulation. More... | |
virtual void | UpdateMeshProxies (unsigned int i, MeshState &mesh_state) |
Update the state of all proxy bodies for the i-th tire mesh. More... | |
virtual void | GetForcesMeshProxies (unsigned int i, MeshContact &mesh_contact) |
Collect cumulative contact forces on all proxy bodies for the i-th tire mesh. More... | |
virtual void | UpdateWheelProxy (unsigned int i, BodyState &spindle_state)=0 |
Update the state of the wheel proxy body for the i-th tire. More... | |
virtual void | GetForceWheelProxy (unsigned int i, TerrainForce &wheel_contact)=0 |
Collect cumulative contact force and torque on the wheel proxy body for the i-th tire. More... | |
![]() | |
ChVehicleCosimBaseNode (const std::string &name) | |
Protected Attributes | |
bool | m_render |
if true, perform run-time rendering | |
double | m_render_step |
time step between rendered frames | |
double | m_hdimX |
patch half-length (X direction) | |
double | m_hdimY |
patch half-width (Y direction) | |
InterfaceType | m_interface_type |
type of communication interface | |
std::vector< double > | m_tire_radius |
tire radius | |
std::vector< double > | m_tire_width |
tire width | |
std::vector< double > | m_load_mass |
vertical load on tire | |
std::vector< MaterialInfo > | m_mat_props |
tire contact material properties | |
std::vector< MeshData > | m_mesh_data |
tire mesh data | |
std::vector< MeshState > | m_mesh_state |
tire mesh state (used for MESH communication) | |
std::vector< BodyState > | m_spindle_state |
spindle state (used for BODY communication interface) | |
![]() | |
int | m_rank |
MPI rank of this node (in MPI_COMM_WORLD) | |
double | m_step_size |
integration step size | |
std::string | m_name |
name of the node | |
std::string | m_out_dir |
top-level output directory | |
std::string | m_node_out_dir |
node-specific output directory | |
std::ofstream | m_outf |
output file stream | |
unsigned int | m_num_mbs_nodes |
unsigned int | m_num_terrain_nodes |
unsigned int | m_num_tire_nodes |
ChTimer< double > | m_timer |
timer for integration cost | |
double | m_cum_sim_time |
cumulative integration cost | |
bool | m_verbose |
verbose messages during simulation? | |
Additional Inherited Members | |
![]() | |
enum | NodeType { NodeType::MBS, NodeType::TERRAIN, NodeType::TIRE } |
Type of node participating in co-simulation. More... | |
enum | InterfaceType { InterfaceType::BODY, InterfaceType::MESH } |
Type of the tire-terrain communication interface. More... | |
![]() | |
static std::string | OutputFilename (const std::string &dir, const std::string &root, const std::string &ext, int frame, int frame_digits) |
Utility function for creating an output file name. More... | |
![]() | |
static const double | m_gacc = -9.81 |
Member Function Documentation
◆ Advance()
|
finaloverridevirtual |
Advance simulation.
This function is called after a synchronization to allow the node to advance its state by the specified time step. A node is allowed to take as many internal integration steps as required, but no inter-node communication should occur.
Implements chrono::vehicle::ChVehicleCosimBaseNode.
◆ EnableRuntimeVisualization()
void chrono::vehicle::ChVehicleCosimTerrainNode::EnableRuntimeVisualization | ( | bool | render, |
double | render_fps = 100 |
||
) |
Enable/disable run-time visualization (default: false).
If enabled, rendering is done with the specified frequency. Note that a particular concrete terrain node may not support run-time visualization or may not render all physics elements.
◆ GetForcesMeshProxies()
|
inlineprotectedvirtual |
Collect cumulative contact forces on all proxy bodies for the i-th tire mesh.
Load indices of vertices in contact and the corresponding vertex forces (expressed in absolute frame) into the provided MeshContact struct.
◆ GetForceWheelProxy()
|
protectedpure virtual |
Collect cumulative contact force and torque on the wheel proxy body for the i-th tire.
Load contact forces (expressed in absolute frame) into the provided TerrainForce struct.
◆ GetInitHeight()
|
protectedpure virtual |
Return the terrain initial height.
This value must be available before the call to Initialize() (and therefore, before the derived class's OnInitialize() is called).
Implemented in chrono::vehicle::ChVehicleCosimTerrainNodeChrono.
◆ GetNumContacts()
|
inlinevirtual |
Return current number of contacts.
(concrete terrain specific)
◆ Initialize()
|
finaloverridevirtual |
Initialize this node.
This function allows the node to initialize itself and, optionally, perform an initial data exchange with any other node.
Reimplemented from chrono::vehicle::ChVehicleCosimBaseNode.
◆ OnInitialize()
|
protectedpure virtual |
Perform any additional operations after the initial data exchange with the MBS node, including creating any required proxies for the specified number of tires.
A derived class has access to the following vectors (of size equal to the number of tires):
- radius for each tire (through m_tire_radius)
- width for each tire (through m_tire_width)
- mesh information for each tire (through m_mesh_data)
- contact material for each tire (through m_mat_props)
- vertical load on each tire (through m_load_mass)
Implemented in chrono::vehicle::ChVehicleCosimTerrainNodeChrono, and chrono::vehicle::ChVehicleCosimTerrainNodeGranularSPH.
◆ OnSynchronize()
|
inlineprotectedvirtual |
Perform any additional operations after the data exchange and synchronization with the MBS node.
A derived class has access to the following vectors (of size equal to the number of tires):
- full dynamic state of the spindle bodies (through m_spindle_state) when using the BODY communication interface
- state of the mesh vertices (through m_mesh_state) when using the MESH communication interface
◆ OutputVisualizationData()
|
inlineoverridevirtual |
Output post-processing visualization data.
If implemented, this function should write a file in the "visualization" subdirectory of m_node_out_dir.
Implements chrono::vehicle::ChVehicleCosimBaseNode.
Reimplemented in chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP, chrono::vehicle::ChVehicleCosimTerrainNodeGranularGPU, chrono::vehicle::ChVehicleCosimTerrainNodeRigid, and chrono::vehicle::ChVehicleCosimTerrainNodeGranularSPH.
◆ Render()
|
inlineprotectedvirtual |
Render simulation.
This function is called from Advance() at the frequency spoecified in the call to EnableRuntimeVisualization(). Any call to Render occurs after a call to OnAdvance().
◆ SetDimensions()
void chrono::vehicle::ChVehicleCosimTerrainNode::SetDimensions | ( | double | length, |
double | width | ||
) |
Set the terrain patch dimensions.
If invoked, this function must be called before Initialize.
◆ SupportsMeshInterface()
|
protectedpure virtual |
Specify whether or not the terrain node supports the MESH communication interface.
See ChVehicleCosimBaseNode::InterfaceType. A terrain that also supports the MESH communication interface must override the functions UpdateMeshProxies() and GetForcesMeshProxies().
◆ Synchronize()
|
finaloverridevirtual |
Synchronize this node.
This function is called at every co-simulation synchronization time to allow the node to exchange information with any other node.
Implements chrono::vehicle::ChVehicleCosimBaseNode.
◆ UpdateMeshProxies()
|
inlineprotectedvirtual |
Update the state of all proxy bodies for the i-th tire mesh.
Use information in the provided MeshState struct (vertex positions and velocities expressed in absolute frame).
◆ UpdateWheelProxy()
|
protectedpure virtual |
Update the state of the wheel proxy body for the i-th tire.
Use information in the provided BodyState struct (pose and velocities expressed in absolute frame).
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/ChVehicleCosimTerrainNode.h
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/ChVehicleCosimTerrainNode.cpp