Description
Interactive driver model using keyboard inputs.
Irrlicht-based GUI driver for the a vehicle. This class implements the functionality required by its base ChDriver class using keyboard inputs. As an Irrlicht event receiver, its OnEvent() callback is used to keep track and update the current driver inputs.
- See also
- ChDataDriver
#include <ChIrrGuiDriver.h>
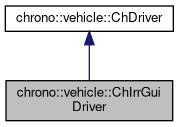

Public Types | |
enum | InputMode { LOCK, KEYBOARD, DATAFILE, JOYSTICK } |
Functioning modes for a ChIrrGuiDriver. More... | |
enum | JoystickAxes { AXIS_X = irr::SEvent::SJoystickEvent::AXIS_X, AXIS_Y = irr::SEvent::SJoystickEvent::AXIS_Y, AXIS_Z = irr::SEvent::SJoystickEvent::AXIS_Z, AXIS_R = irr::SEvent::SJoystickEvent::AXIS_R, AXIS_U = irr::SEvent::SJoystickEvent::AXIS_U, AXIS_V = irr::SEvent::SJoystickEvent::AXIS_V, NONE } |
Exposes the unnamed enum of irrlicht axes to enforce right values in API usage. | |
Public Member Functions | |
ChIrrGuiDriver (ChVehicleIrrApp &app) | |
Construct an Irrlicht GUI driver. More... | |
virtual bool | OnEvent (const irr::SEvent &event) override |
Intercept and process keyboard inputs. More... | |
virtual void | Synchronize (double time) override |
Update the state of this driver system at the specified time. | |
virtual void | Advance (double step) override |
Advance the state of this driver system by the specified time step. | |
void | SetThrottleDelta (double delta) |
Set the increment in throttle input for each recorded keypress (default 1/50). | |
void | SetSteeringDelta (double delta) |
Set the increment in steering input for each recorded keypress (default 1/50). | |
void | SetBrakingDelta (double delta) |
Set the increment in braking input for each recorded keypress (default 1/50). | |
void | SetStepsize (double val) |
Set the step size for integration of the internal driver dynamics. | |
void | SetGains (double steering_gain, double throttle_gain, double braking_gain) |
Set gains for internal dynamics. More... | |
void | SetInputDataFile (const std::string &filename) |
Set the input file for the underlying data driver. | |
void | SetInputMode (InputMode mode) |
Set the current functioning mode. | |
std::string | GetInputModeAsString () const |
Return the current functioning mode as a string. | |
void | SetJoystickAxes (JoystickAxes tr_ax, JoystickAxes br_ax, JoystickAxes st_ax, JoystickAxes cl_ax) |
Set joystick axes: throttle, brake, steering, clutch. | |
JoystickAxes | GetThrottleAxis (JoystickAxes tr_ax) const |
Get joystick axes for the throttle. | |
JoystickAxes | GetBrakeAxis (JoystickAxes br_ax) const |
Get joystick axes for the brake. | |
JoystickAxes | GetSteerAxis (JoystickAxes st_ax) const |
Get joystick axes for the steer. | |
JoystickAxes | GetClutchAxis (JoystickAxes cl_ax) const |
Get joystick axes for the clutch. | |
void | SetButtonCallback (int button, void(*cbfun)()) |
Feed button number and callback function to implement a custom callback. | |
![]() | |
ChDriver (ChVehicle &vehicle) | |
double | GetThrottle () const |
Get the driver throttle input (in the range [0,1]) | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]) | |
double | GetBraking () const |
Get the driver braking input (in the range [0,1]) | |
Inputs | GetInputs () const |
Get all current inputs at once. | |
virtual void | Initialize () |
Initialize this driver system. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
void | SetSteering (double val, double min_val=-1, double max_val=1) |
Overwrite the value for the driver steering input. | |
void | SetThrottle (double val, double min_val=0, double max_val=1) |
Overwrite the value for the driver throttle input. | |
void | SetBraking (double val, double min_val=0, double max_val=1) |
Overwrite the value for the driver braking input. | |
Protected Attributes | |
ChVehicleIrrApp & | m_app |
InputMode | m_mode |
current mode of the driver | |
double | m_steering_target |
current target value for steering input | |
double | m_throttle_target |
current target value for throttle input | |
double | m_braking_target |
current target value for braking input | |
double | m_stepsize |
time step for internal dynamics | |
double | m_steering_delta |
steering increment on each keypress | |
double | m_throttle_delta |
throttle increment on each keypress | |
double | m_braking_delta |
braking increment on each keypress | |
double | m_steering_gain |
gain for steering internal dynamics | |
double | m_throttle_gain |
gain for throttle internal dynamics | |
double | m_braking_gain |
gain for braking internal dynamics | |
int | m_dT |
JoystickAxes | throttle_axis = AXIS_Z |
JoystickAxes | brake_axis = AXIS_R |
JoystickAxes | steer_axis = AXIS_X |
JoystickAxes | clutch_axis = AXIS_Y |
int | callbackButton = -1 |
void(* | cb_fun )() = nullptr |
double | m_time_shift |
time at which mode was switched to DATAFILE | |
std::shared_ptr< ChDataDriver > | m_data_driver |
embedded data driver (for playback) | |
![]() | |
ChVehicle & | m_vehicle |
reference to associated vehicle | |
double | m_throttle |
current value of throttle input | |
double | m_steering |
current value of steering input | |
double | m_braking |
current value of braking input | |
Member Enumeration Documentation
◆ InputMode
Functioning modes for a ChIrrGuiDriver.
Enumerator | |
---|---|
LOCK | driver inputs locked at current values |
KEYBOARD | driver inputs from keyboard |
DATAFILE | driver inputs from data file |
JOYSTICK | driver inputs from joystick |
Constructor & Destructor Documentation
◆ ChIrrGuiDriver()
chrono::vehicle::ChIrrGuiDriver::ChIrrGuiDriver | ( | ChVehicleIrrApp & | app | ) |
Construct an Irrlicht GUI driver.
Activates joysticks, if available
Member Function Documentation
◆ OnEvent()
|
overridevirtual |
Intercept and process keyboard inputs.
Only handles joystick events
Driver only handles input every 16 ticks (~60 Hz)
Gear is set to reverse
Only interpret keyboard inputs.
◆ SetGains()
void chrono::vehicle::ChIrrGuiDriver::SetGains | ( | double | steering_gain, |
double | throttle_gain, | ||
double | braking_gain | ||
) |
Set gains for internal dynamics.
Default values are 4.0.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChIrrGuiDriver.h
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChIrrGuiDriver.cpp