Description
Base class for chrono vehicle systems.
The reference frame for a vehicle follows the ISO standard: Z-axis up, X-axis pointing forward, and Y-axis towards the left of the vehicle.
#include <ChVehicle.h>
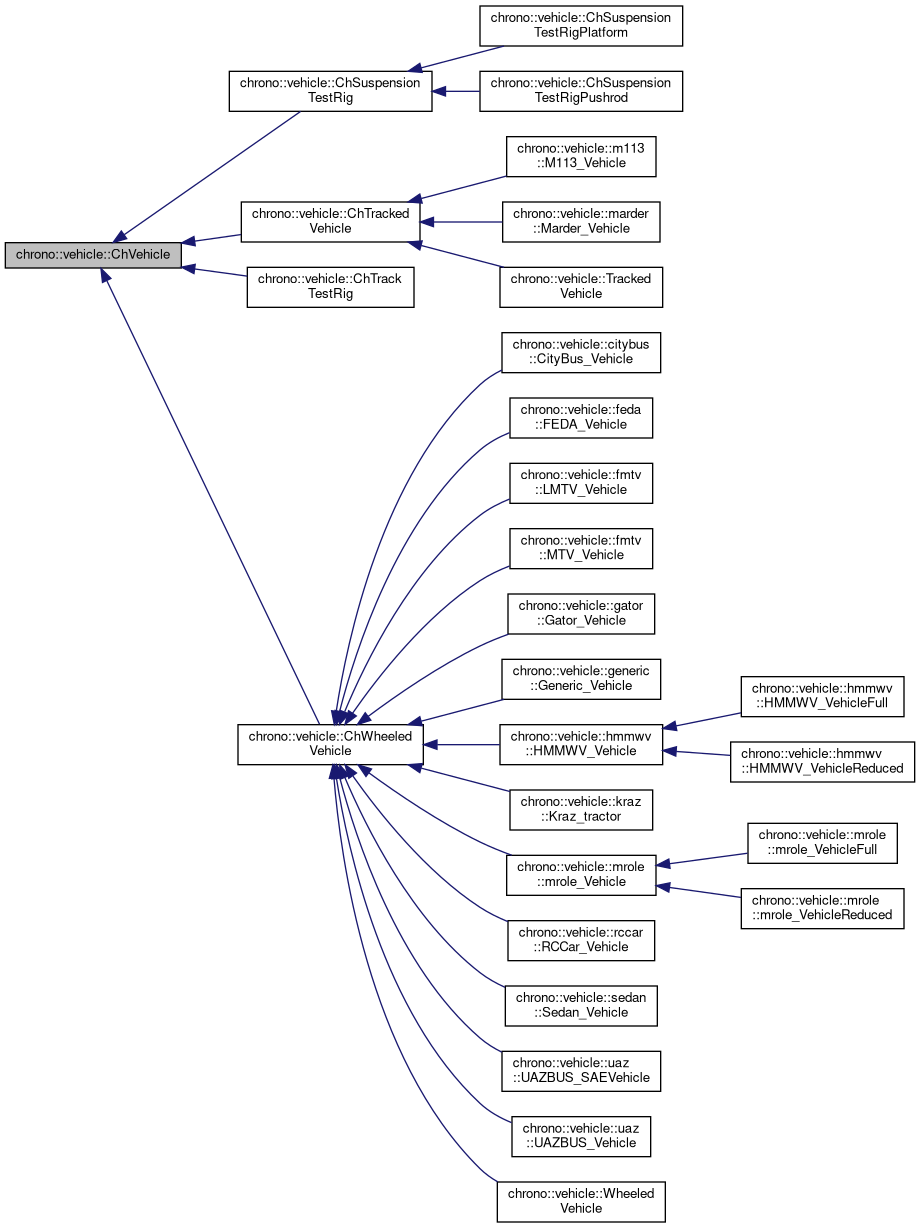
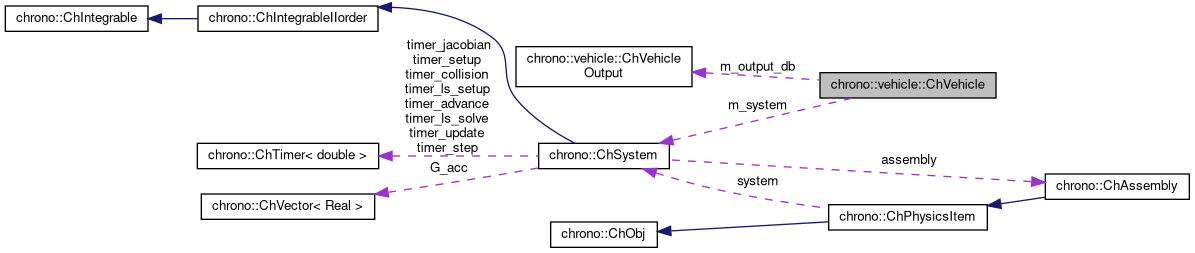
Public Member Functions | |
virtual | ~ChVehicle () |
Destructor. | |
const std::string & | GetName () const |
Get the name identifier for this vehicle. | |
void | SetName (const std::string &name) |
Set the name identifier for this vehicle. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle system template. | |
ChSystem * | GetSystem () |
Get a pointer to the Chrono ChSystem. | |
double | GetChTime () const |
Get the current simulation time of the underlying ChSystem. | |
std::shared_ptr< ChChassis > | GetChassis () const |
Get a handle to the vehicle's main chassis subsystem. | |
std::shared_ptr< ChChassisRear > | GetChassisRear (int id) const |
Get the specified specified rear chassis subsystem. | |
std::shared_ptr< ChChassisConnector > | GetChassisConnector (int id) const |
Get a handle to the specified chassis connector. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisBody () const |
Get a handle to the vehicle's chassis body. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisRearBody (int id) const |
Get a handle to the specified rear chassis body. | |
virtual std::shared_ptr< ChPowertrain > | GetPowertrain () const |
Get the powertrain attached to this vehicle. | |
virtual double | GetVehicleMass () const =0 |
Get the vehicle total mass. More... | |
virtual ChVector | GetVehicleCOMPos () const =0 |
Get the current global vehicle COM location. | |
const ChVector & | GetVehiclePos () const |
Get the vehicle location. More... | |
ChQuaternion | GetVehicleRot () const |
Get the vehicle orientation. More... | |
double | GetVehicleSpeed () const |
Get the vehicle speed. More... | |
double | GetVehicleSpeedCOM () const |
Get the speed of the chassis COM. More... | |
ChVector | GetVehiclePointLocation (const ChVector<> &locpos) const |
Get the global position of the specified point. More... | |
ChVector | GetVehiclePointVelocity (const ChVector<> &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector | GetVehiclePointAcceleration (const ChVector<> &locpos) const |
Get the acceleration at the specified point. More... | |
virtual std::shared_ptr< ChShaft > | GetDriveshaft () const =0 |
Get a handle to the vehicle's driveshaft body. | |
ChVector | GetDriverPos () const |
Get the global location of the driver. | |
void | SetCollisionSystemType (collision::ChCollisionSystemType collsys_type) |
Change the default collision detection system. More... | |
void | SetOutput (ChVehicleOutput::Type type, const std::string &out_dir, const std::string &out_name, double output_step) |
Enable output for this vehicle system. More... | |
virtual void | Initialize (const ChCoordsys<> &chassisPos, double chassisFwdVel=0)=0 |
Initialize this vehicle at the specified global location and orientation. More... | |
void | SetChassisVisualizationType (VisualizationType vis) |
Set visualization mode for the chassis subsystem. | |
void | SetChassisRearVisualizationType (VisualizationType vis) |
Set visualization mode for the rear chassis subsystems. | |
void | SetChassisCollide (bool state) |
Enable/disable collision for the chassis subsystem. More... | |
virtual void | SetChassisVehicleCollide (bool state) |
Enable/disable collision between the chassis and all other vehicle subsystems. More... | |
void | SetChassisOutput (bool state) |
Enable/disable output from the chassis subsystem. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
virtual void | Advance (double step) |
Advance the state of this vehicle by the specified time step. More... | |
virtual void | LogConstraintViolations ()=0 |
Log current constraint violations. | |
virtual std::string | ExportComponentList () const =0 |
Return a JSON string with information on all modeling components in the vehicle system. More... | |
virtual void | ExportComponentList (const std::string &filename) const =0 |
Write a JSON-format file with information on all modeling components in the vehicle system. More... | |
virtual void | Output (int frame, ChVehicleOutput &database) const =0 |
Output data for all modeling components in the vehicle system. | |
Protected Member Functions | |
ChVehicle (const std::string &name, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a vehicle system with an underlying ChSystem. More... | |
ChVehicle (const std::string &name, ChSystem *system) | |
Construct a vehicle system using the specified ChSystem. More... | |
Static Protected Member Functions | |
template<typename T > | |
static bool | AnyOutput (const std::vector< std::shared_ptr< T >> &list) |
Utility function for testing if any subsystem in a list generates output. | |
Protected Attributes | |
std::string | m_name |
vehicle name | |
ChSystem * | m_system |
pointer to the Chrono system | |
bool | m_ownsSystem |
true if system created at construction | |
bool | m_output |
generate ouput for this vehicle system | |
ChVehicleOutput * | m_output_db |
vehicle output database | |
double | m_output_step |
output time step | |
double | m_next_output_time |
time for next output | |
int | m_output_frame |
current output frame | |
std::shared_ptr< ChChassis > | m_chassis |
handle to the main chassis subsystem | |
ChChassisRearList | m_chassis_rear |
list of rear chassis subsystems (can be empty) | |
ChChassisConnectorList | m_chassis_connectors |
list of chassis connector (must match m_chassis_rear) | |
Constructor & Destructor Documentation
◆ ChVehicle() [1/2]
|
protected |
Construct a vehicle system with an underlying ChSystem.
- Parameters
-
[in] name vehicle name [in] contact_method contact method
◆ ChVehicle() [2/2]
|
protected |
Construct a vehicle system using the specified ChSystem.
All physical components of the vehicle will be added to that system.
- Parameters
-
[in] name vehicle name [in] system containing mechanical system
Member Function Documentation
◆ Advance()
|
virtual |
Advance the state of this vehicle by the specified time step.
A call to ChSystem::DoStepDynamics is done only if the vehicle owns the underlying Chrono system. Otherwise, the caller is responsible for advancing the sate of the entire system.
Reimplemented in chrono::vehicle::ChWheeledVehicle, chrono::vehicle::ChTrackedVehicle, chrono::vehicle::ChTrackTestRig, and chrono::vehicle::ChSuspensionTestRig.
◆ ExportComponentList() [1/2]
|
pure virtual |
Return a JSON string with information on all modeling components in the vehicle system.
These include bodies, shafts, joints, spring-damper elements, markers, etc.
Implemented in chrono::vehicle::ChWheeledVehicle, and chrono::vehicle::ChTrackedVehicle.
◆ ExportComponentList() [2/2]
|
pure virtual |
Write a JSON-format file with information on all modeling components in the vehicle system.
These include bodies, shafts, joints, spring-damper elements, markers, etc.
Implemented in chrono::vehicle::ChWheeledVehicle, and chrono::vehicle::ChTrackedVehicle.
◆ GetVehicleMass()
|
pure virtual |
Get the vehicle total mass.
This includes the mass of the chassis and all vehicle subsystems.
Implemented in chrono::vehicle::ChWheeledVehicle, and chrono::vehicle::ChTrackedVehicle.
◆ GetVehiclePointAcceleration()
|
inline |
Get the acceleration at the specified point.
The point is assumed to be given relative to the main chassis reference frame. The returned acceleration is expressed in the chassis reference frame.
◆ GetVehiclePointLocation()
|
inline |
Get the global position of the specified point.
The point is assumed to be given relative to the main chassis reference frame. The returned location is expressed in the global reference frame.
◆ GetVehiclePointVelocity()
|
inline |
Get the global velocity of the specified point.
The point is assumed to be given relative to the main chassis reference frame. The returned velocity is expressed in the global reference frame.
◆ GetVehiclePos()
|
inline |
Get the vehicle location.
This is the global location of the main chassis reference frame origin.
◆ GetVehicleRot()
|
inline |
Get the vehicle orientation.
This is the main chassis orientation, returned as a quaternion representing a rotation with respect to the global reference frame.
◆ GetVehicleSpeed()
|
inline |
Get the vehicle speed.
Return the speed measured at the origin of the main chassis reference frame.
◆ GetVehicleSpeedCOM()
|
inline |
Get the speed of the chassis COM.
Return the speed measured at the main chassis center of mass.
◆ Initialize()
|
pure virtual |
Initialize this vehicle at the specified global location and orientation.
- Parameters
-
[in] chassisPos initial global position and orientation [in] chassisFwdVel initial chassis forward velocity
Implemented in chrono::vehicle::ChTrackedVehicle, chrono::vehicle::ChWheeledVehicle, chrono::vehicle::uaz::UAZBUS_SAEVehicle, chrono::vehicle::feda::FEDA_Vehicle, chrono::vehicle::rccar::RCCar_Vehicle, chrono::vehicle::fmtv::LMTV_Vehicle, chrono::vehicle::fmtv::MTV_Vehicle, chrono::vehicle::uaz::UAZBUS_Vehicle, chrono::vehicle::sedan::Sedan_Vehicle, chrono::vehicle::citybus::CityBus_Vehicle, chrono::vehicle::hmmwv::HMMWV_VehicleFull, chrono::vehicle::gator::Gator_Vehicle, chrono::vehicle::mrole::mrole_VehicleFull, chrono::vehicle::generic::Generic_Vehicle, chrono::vehicle::m113::M113_Vehicle, chrono::vehicle::marder::Marder_Vehicle, chrono::vehicle::kraz::Kraz_tractor, chrono::vehicle::hmmwv::HMMWV_VehicleReduced, chrono::vehicle::mrole::mrole_VehicleReduced, chrono::vehicle::WheeledVehicle, and chrono::vehicle::TrackedVehicle.
◆ SetChassisCollide()
void chrono::vehicle::ChVehicle::SetChassisCollide | ( | bool | state | ) |
Enable/disable collision for the chassis subsystem.
This function controls contact of the chassis with all other collision shapes in the simulation.
◆ SetChassisVehicleCollide()
|
inlinevirtual |
Enable/disable collision between the chassis and all other vehicle subsystems.
Note that some of these collisions may be always disabled, as set by the particular derived vehicle class.
Reimplemented in chrono::vehicle::ChWheeledVehicle, and chrono::vehicle::ChTrackedVehicle.
◆ SetCollisionSystemType()
void chrono::vehicle::ChVehicle::SetCollisionSystemType | ( | collision::ChCollisionSystemType | collsys_type | ) |
Change the default collision detection system.
Note that this function should be called before initialization of the vehicle system in order to create consistent collision models.
◆ SetOutput()
void chrono::vehicle::ChVehicle::SetOutput | ( | ChVehicleOutput::Type | type, |
const std::string & | out_dir, | ||
const std::string & | out_name, | ||
double | output_step | ||
) |
Enable output for this vehicle system.
- Parameters
-
[in] type type of output DB [in] out_dir output directory name [in] out_name rootname of output file [in] output_step interval between output times
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/ChVehicle.h
- /builds/uwsbel/chrono/src/chrono_vehicle/ChVehicle.cpp