chrono::vehicle::ChChassisRear Class Referenceabstract
Description
Base class for a rear chassis vehicle subsystem.
A rear chassis is attached (through one of the provided ChassisConnector templates) to another chassis. As such, a rear chassis is initialized with a position relative to the other chassis.
#include <ChChassis.h>
Inheritance diagram for chrono::vehicle::ChChassisRear:
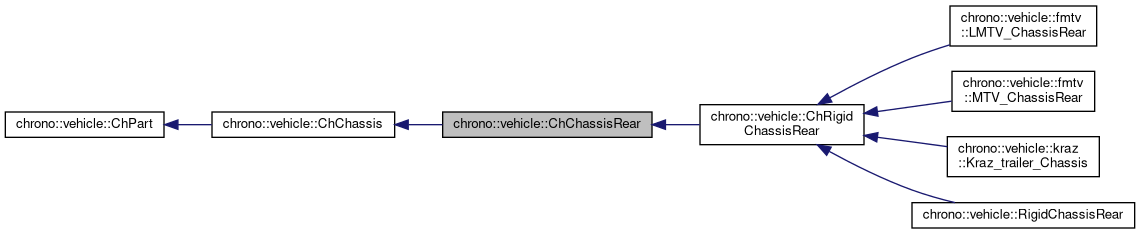
Collaboration diagram for chrono::vehicle::ChChassisRear:
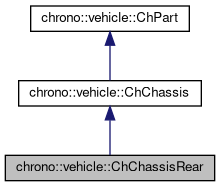
Public Member Functions | |
ChChassisRear (const std::string &name) | |
Construct a rear chassis subsystem with the specified name. | |
virtual const ChVector & | GetLocalPosFrontConnector () const =0 |
Get the location (in the local frame of this chassis) of the connection to the front chassis. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, int collision_family=0) |
Initialize the rear chassis relative to the specified front chassis. More... | |
![]() | |
ChChassis (const std::string &name, bool fixed=false) | |
Construct a chassis subsystem with the specified name. More... | |
virtual double | GetMass () const =0 |
Get the chassis mass. | |
virtual const ChMatrix33 & | GetInertia () const =0 |
Get the inertia tensor of the chassis body. More... | |
virtual const ChVector & | GetLocalPosCOM () const =0 |
Get the location of the center of mass in the chassis frame. | |
virtual const ChVector | GetLocalPosRearConnector () const |
Get the location (in the local frame of this chassis) of the connection to a rear chassis. | |
std::shared_ptr< ChBodyAuxRef > | GetBody () const |
Get a handle to the vehicle's chassis body. | |
ChSystem * | GetSystem () const |
Get a pointer to the containing system. | |
const ChVector & | GetPos () const |
Get the global location of the chassis reference frame origin. | |
ChQuaternion | GetRot () const |
Get the orientation of the chassis reference frame. More... | |
const ChVector & | GetCOMPos () const |
Get the global location of the chassis center of mass. | |
ChQuaternion | GetCOMRot () const |
Get the orientation of the chassis centroidal frame. More... | |
ChVector | GetDriverPos () const |
Get the global location of the driver. | |
double | GetSpeed () const |
Get the vehicle speed. More... | |
double | GetCOMSpeed () const |
Get the speed of the chassis COM. More... | |
ChVector | GetPointLocation (const ChVector<> &locpos) const |
Get the global position of the specified point. More... | |
ChVector | GetPointVelocity (const ChVector<> &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector | GetPointAcceleration (const ChVector<> &locpos) const |
Get the acceleration at the specified point. More... | |
virtual void | SetCollide (bool state)=0 |
Enable/disable contact for the chassis. More... | |
void | SetFixed (bool val) |
Set the "fixed to ground" status of the chassis body. | |
bool | IsFixed () const |
Return true if the chassis body is fixed to ground. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
void | AddMarker (const std::string &name, const ChCoordsys<> &pos) |
Add a marker on the chassis body at the specified position (relative to the chassis reference frame). More... | |
const std::vector< std::shared_ptr< ChMarker > > & | GetMarkers () const |
void | SetAerodynamicDrag (double Cd, double area, double air_density) |
Set parameters and enable aerodynamic drag force calculation. More... | |
virtual void | Synchronize (double time) |
Update the state of the chassis subsystem. More... | |
void | AddJoint (std::shared_ptr< ChVehicleJoint > joint) |
Utility function to add a joint (kinematic or bushing) to the vehicle system. | |
void | AddExternalForce (std::shared_ptr< ExternalForce > force) |
Utility force to add an external load to the chassis body. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Additional Inherited Members | |
![]() | |
static void | RemoveJoint (std::shared_ptr< ChVehicleJoint > joint) |
Utility function to remove a joint (kinematic or bushing) from the vehicle system. | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
std::shared_ptr< ChBodyAuxRef > | m_body |
handle to the chassis body | |
std::shared_ptr< ChLoadContainer > | m_bushings |
load container for vehicle bushings | |
std::vector< std::shared_ptr< ChMarker > > | m_markers |
list of user-defined markers | |
bool | m_fixed |
is the chassis body fixed to ground? | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
Member Function Documentation
◆ Initialize()
|
virtual |
Initialize the rear chassis relative to the specified front chassis.
The orientation is set to be the same as that of the front chassis while the location is based on the connector position on the front and rear chassis.
- Parameters
-
[in] chassis front chassis [in] collision_family chassis collision family
Reimplemented in chrono::vehicle::ChRigidChassisRear.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/ChChassis.h
- /builds/uwsbel/chrono/src/chrono_vehicle/ChChassis.cpp