Description
Load representing a spring between two ChNodeXYZ, with given damping and spring constants, directed as the distance between the two.
#include <ChLoadsXYZnode.h>
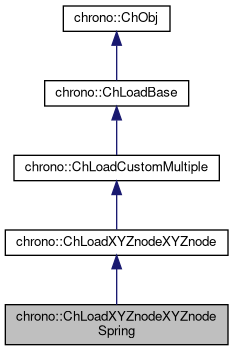
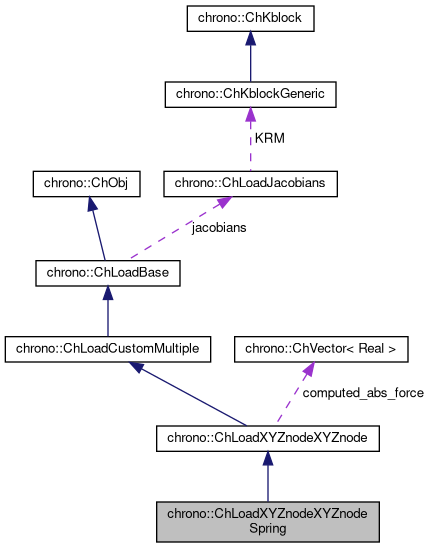
Public Member Functions | |
ChLoadXYZnodeXYZnodeSpring (std::shared_ptr< ChNodeXYZ > mnodeA, std::shared_ptr< ChNodeXYZ > mnodeB, double mK, double mR, double mD0=0) | |
virtual ChLoadXYZnodeXYZnodeSpring * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual void | ComputeForce (const ChVector<> &rel_pos, const ChVector<> &rel_vel, ChVector<> &abs_force) override |
Compute the force on the nodeA, in absolute coordsystem, given relative position of nodeA respect to B, in absolute basis. More... | |
void | SetStiffness (const double mstiffness) |
Set stiffness, along direction, es [N/m]. | |
double | GetStiffness () const |
void | SetDamping (const double mdamping) |
Set damping, along direction, es [Ns/m]. | |
double | GetDamping () const |
void | SetRestLength (const double mrest) |
Set initial spring length, es [m]. | |
double | GetRestLength () const |
void | SetStiff (bool ms) |
Use this to enable the stiff force computation (i.e. More... | |
![]() | |
ChLoadXYZnodeXYZnode (std::shared_ptr< ChNodeXYZ > mnodeA, std::shared_ptr< ChNodeXYZ > mnodeB) | |
virtual void | ComputeQ (ChState *state_x, ChStateDelta *state_w) override |
Compute Q, the generalized load. More... | |
ChVector | GetForce () const |
For diagnosis purposes, this can return the actual last computed value of the applied force, expressed in absolute coordinate system, assumed applied to node. | |
![]() | |
ChLoadCustomMultiple (std::vector< std::shared_ptr< ChLoadable >> &mloadables) | |
ChLoadCustomMultiple (std::shared_ptr< ChLoadable > mloadableA, std::shared_ptr< ChLoadable > mloadableB) | |
ChLoadCustomMultiple (std::shared_ptr< ChLoadable > mloadableA, std::shared_ptr< ChLoadable > mloadableB, std::shared_ptr< ChLoadable > mloadableC) | |
virtual int | LoadGet_ndof_x () override |
Gets the number of DOFs affected by this load (position part) | |
virtual int | LoadGet_ndof_w () override |
Gets the number of DOFs affected by this load (speed part) | |
virtual void | LoadGetStateBlock_x (ChState &mD) override |
Gets all the current DOFs packed in a single vector (position part) | |
virtual void | LoadGetStateBlock_w (ChStateDelta &mD) override |
Gets all the current DOFs packed in a single vector (speed part) | |
virtual void | LoadStateIncrement (const ChState &x, const ChStateDelta &dw, ChState &x_new) override |
Increment a packed state (ex. More... | |
virtual int | LoadGet_field_ncoords () override |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, = 1 for a thermal problem, etc. | |
virtual void | ComputeJacobian (ChState *state_x, ChStateDelta *state_w, ChMatrixRef mK, ChMatrixRef mR, ChMatrixRef mM) override |
Compute jacobians (default fallback). More... | |
virtual void | LoadIntLoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Adds the internal loads Q (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += forces * c. | |
virtual void | CreateJacobianMatrices () override |
Create the jacobian loads if needed, and also set the ChVariables referenced by the sparse KRM block. | |
virtual ChVectorDynamic & | GetQ () |
Access the generalized load vector Q. | |
![]() | |
ChLoadJacobians * | GetJacobians () |
Access the jacobians (if any, i.e. if this is a stiff load) | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are item(s) of type ChKblock in this object (for further passing it to a solver) Basically does nothing, but inherited classes must specialize this. | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Gets the numerical identifier of the object. | |
void | SetIdentifier (int id) |
Sets the numerical identifier of the object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
const char * | GetName () const |
Gets the name of the object as C Ascii null-terminated string -for reading only! | |
void | SetName (const char myname[]) |
Sets the name of this object, as ascii string. | |
std::string | GetNameString () const |
Gets the name of the object as C Ascii null-terminated string. | |
void | SetNameString (const std::string &myname) |
Sets the name of this object, as std::string. | |
void | MFlagsSetAllOFF (int &mflag) |
void | MFlagsSetAllON (int &mflag) |
void | MFlagSetON (int &mflag, int mask) |
void | MFlagSetOFF (int &mflag, int mask) |
int | MFlagGet (int &mflag, int mask) |
virtual void | ArchiveOUT (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
virtual std::string & | ArchiveContainerName () |
Protected Member Functions | |
virtual bool | IsStiff () override |
Inherited classes could override this and return true, if the load benefits from a jacobian when using implicit integrators. More... | |
![]() | |
virtual void | Update (double time) override |
Update: this is called at least at each time step. More... | |
Protected Attributes | |
double | K |
double | R |
double | d_0 |
bool | is_stiff |
![]() | |
ChVector | computed_abs_force |
![]() | |
ChLoadJacobians * | jacobians |
![]() | |
double | ChTime |
the time of simulation for the object | |
Additional Inherited Members | |
![]() | |
std::vector< std::shared_ptr< ChLoadable > > | loadables |
ChVectorDynamic | load_Q |
Constructor & Destructor Documentation
◆ ChLoadXYZnodeXYZnodeSpring()
chrono::ChLoadXYZnodeXYZnodeSpring::ChLoadXYZnodeXYZnodeSpring | ( | std::shared_ptr< ChNodeXYZ > | mnodeA, |
std::shared_ptr< ChNodeXYZ > | mnodeB, | ||
double | mK, | ||
double | mR, | ||
double | mD0 = 0 |
||
) |
- Parameters
-
mnodeA node to apply load to mnodeB node to apply load to as reaction mK stiffness, mR damping, mD0 initial rest length
Member Function Documentation
◆ ComputeForce()
|
overridevirtual |
Compute the force on the nodeA, in absolute coordsystem, given relative position of nodeA respect to B, in absolute basis.
Compute the force on the node, in absolute coordsystem, given position of node as abs_pos.
Implements chrono::ChLoadXYZnodeXYZnode.
◆ IsStiff()
|
inlineoverrideprotectedvirtual |
Inherited classes could override this and return true, if the load benefits from a jacobian when using implicit integrators.
Reimplemented from chrono::ChLoadXYZnodeXYZnode.
◆ SetStiff()
|
inline |
Use this to enable the stiff force computation (i.e.
it enables the automated computation of the jacobian by numerical differentiation to elp the convergence of implicit integrators, but adding CPU overhead).
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsXYZnode.h
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsXYZnode.cpp