Description
Module for multicore parallel simulation.
This module implements multicore parallel computing algorithms that can be used as a faster alternative to the default simulation algorithms in Chrono::Engine. This is achieved using OpenMP, CUDA, Thrust, etc.
For additional information, see:
- the installation guide
- the tutorials
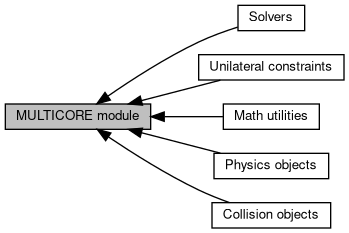
Modules | |
Physics objects | |
Unilateral constraints | |
Collision objects | |
Solvers | |
Math utilities | |
Classes | |
struct | chrono::shape_container |
Structure of arrays containing contact shape information. More... | |
struct | chrono::host_container |
Structure of arrays containing simulation data. More... | |
class | chrono::ChMulticoreDataManager |
Global data manager for Chrono::Multicore. More... | |
class | chrono::collision_measures |
Collision_measures. More... | |
class | chrono::solver_measures |
Solver measures. More... | |
class | chrono::measures_container |
Aggregate of collision and solver measures. More... | |
class | chrono::collision_settings |
Chrono::Multicore collision_settings. More... | |
class | chrono::solver_settings |
Chrono::Multicore solver_settings. More... | |
class | chrono::settings_container |
Aggregate of all settings for Chrono::Multicore. More... | |
struct | chrono::TimerData |
Wrapper class for a timer object. More... | |
class | chrono::ChTimerMulticore |
Utility class for managing a collection of timer objects. More... | |
Functions | |
template<typename Enumeration > | |
auto | chrono::as_integer (Enumeration const value) -> typename std::underlying_type< Enumeration >::type |
Explicit conversion of scoped enumeration to int (e.g. for streaming). | |
template<typename InputIterator1 , typename InputIterator2 , typename OutputIterator > | |
OutputIterator | chrono::Thrust_Expand (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, OutputIterator output) |
Utility to expand an input sequence by replicating each element a variable number of times. More... | |
Macro Definition Documentation
◆ Run_Length_Encode
#define Run_Length_Encode | ( | y, | |
z, | |||
w | |||
) |
◆ Thrust_Inclusive_Scan_Sum
#define Thrust_Inclusive_Scan_Sum | ( | x, | |
y | |||
) |
Enumeration Type Documentation
◆ BilateralType
Enumeration for bilateral constraint types.
◆ CollisionSystemType
|
strong |
◆ LoggingLevel
|
strong |
◆ NarrowPhaseType
|
strong |
◆ SolverMode
|
strong |
◆ SolverType
|
strong |
Iterative solver type.
◆ SystemType
|
strong |
Function Documentation
◆ Thrust_Expand()
OutputIterator chrono::Thrust_Expand | ( | InputIterator1 | first1, |
InputIterator1 | last1, | ||
InputIterator2 | first2, | ||
OutputIterator | output | ||
) |
Utility to expand an input sequence by replicating each element a variable number of times.
For example,
expand([2,2,2],[A,B,C]) -> [A,A,B,B,C,C] expand([3,0,1],[A,B,C]) -> [A,A,A,C] expand([1,3,2],[A,B,C]) -> [A,B,B,B,C,C]
The element counts are assumed to be non-negative integers (from Thrust's example expand.cu)