Description
Class defining the Bullet geometric model for collision detection.
#include <ChCollisionModelBullet.h>
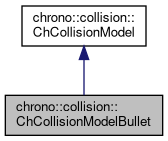
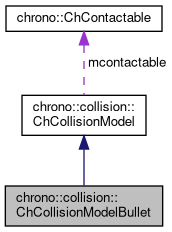
Public Member Functions | |
virtual int | ClearModel () override |
Delete all inserted geometries. More... | |
virtual int | BuildModel () override |
Complete the construction of the collision model (build the BV hierarchy). More... | |
virtual bool | AddSphere (std::shared_ptr< ChMaterialSurface > material, double radius, const ChVector<> &pos=ChVector<>()) override |
Add a sphere shape to this collision model. More... | |
virtual bool | AddEllipsoid (std::shared_ptr< ChMaterialSurface > material, double rx, double ry, double rz, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add an ellipsoid shape to this collision model. More... | |
virtual bool | AddBox (std::shared_ptr< ChMaterialSurface > material, double hx, double hy, double hz, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a box shape to this collision model. More... | |
virtual bool | AddCylinder (std::shared_ptr< ChMaterialSurface > material, double rx, double rz, double hy, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a cylinder to this collision model (default axis on Y direction). More... | |
virtual bool | AddCone (std::shared_ptr< ChMaterialSurface > material, double rx, double rz, double hy, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a cone to this collision model (default axis on Y direction). More... | |
virtual bool | AddCapsule (std::shared_ptr< ChMaterialSurface > material, double radius, double hlen, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a capsule to this collision model (default axis in Y direction). More... | |
virtual bool | AddRoundedBox (std::shared_ptr< ChMaterialSurface > material, double hx, double hy, double hz, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a rounded box shape to this collision model. More... | |
virtual bool | AddRoundedCylinder (std::shared_ptr< ChMaterialSurface > material, double rx, double rz, double hy, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a rounded cylinder to this collision model (default axis on Y direction). More... | |
virtual bool | AddRoundedCone (std::shared_ptr< ChMaterialSurface > material, double rx, double rz, double hy, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a rounded cone to this collision model (default axis on Y direction). More... | |
virtual bool | AddConvexHull (std::shared_ptr< ChMaterialSurface > material, const std::vector< ChVector< double >> &pointlist, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a convex hull to this collision model. More... | |
virtual bool | AddTriangleMesh (std::shared_ptr< ChMaterialSurface > material, std::shared_ptr< geometry::ChTriangleMesh > trimesh, bool is_static, bool is_convex, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1), double sphereswept_thickness=0.0) override |
Add a triangle mesh to this collision model. More... | |
virtual bool | AddTriangleMeshConcave (std::shared_ptr< ChMaterialSurface > material, std::shared_ptr< geometry::ChTriangleMesh > trimesh, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) |
CUSTOM for this class only: add a concave triangle mesh that will be managed by GImpact mesh-mesh algorithm. More... | |
virtual bool | AddTriangleMeshConcaveDecomposed (std::shared_ptr< ChMaterialSurface > material, std::shared_ptr< ChConvexDecomposition > mydecomposition, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) |
CUSTOM for this class only: add a concave triangle mesh that will be decomposed into a compound of convex shapes. More... | |
virtual bool | AddBarrel (std::shared_ptr< ChMaterialSurface > material, double Y_low, double Y_high, double R_vert, double R_hor, double R_offset, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a barrel-like shape to this collision model (main axis on Y direction). More... | |
virtual bool | Add2Dpath (std::shared_ptr< ChMaterialSurface > material, std::shared_ptr< geometry::ChLinePath > mpath, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1), const double thickness=0.001) override |
Add a 2D closed line, defined on the XY plane passing by pos and aligned as rot, that defines a 2D collision shape that will collide with another 2D line of the same type if aligned on the same plane. More... | |
virtual bool | AddPoint (std::shared_ptr< ChMaterialSurface > material, double radius=0, const ChVector<> &pos=ChVector<>()) override |
Add a point-like sphere, that will collide with other geometries, but won't ever create contacts between them. More... | |
virtual bool | AddTriangleProxy (std::shared_ptr< ChMaterialSurface > material, ChVector<> *p1, ChVector<> *p2, ChVector<> *p3, ChVector<> *ep1, ChVector<> *ep2, ChVector<> *ep3, bool mowns_vertex_1, bool mowns_vertex_2, bool mowns_vertex_3, bool mowns_edge_1, bool mowns_edge_2, bool mowns_edge_3, double msphereswept_rad=0) |
Add a triangle from mesh. More... | |
virtual bool | AddCopyOfAnotherModel (ChCollisionModel *another) override |
Add all shapes already contained in another model. More... | |
virtual void | SetFamily (int mfamily) override |
By default, all collision objects belong to family n.0, but you can set family in range 0..15. More... | |
virtual int | GetFamily () override |
virtual void | SetFamilyMaskNoCollisionWithFamily (int mfamily) override |
By default, family mask is all turned on, so all families can collide with this object, but you can turn on-off some bytes of this mask so that some families do not collide. More... | |
virtual void | SetFamilyMaskDoCollisionWithFamily (int mfamily) override |
virtual bool | GetFamilyMaskDoesCollisionWithFamily (int mfamily) override |
Tells if the family mask of this collision object allows for the collision with another collision object belonging to a given family. More... | |
virtual void | SetFamilyGroup (short int group) override |
Set the collision family group of this model. More... | |
virtual void | SetFamilyMask (short int mask) override |
Set the collision mask for this model. More... | |
virtual void | GetAABB (ChVector<> &bbmin, ChVector<> &bbmax) const override |
Returns the axis aligned bounding box (AABB) of the collision model, i.e. More... | |
virtual void | SyncPosition () override |
Sets the position and orientation of the collision model as the current position of the corresponding ChContactable. | |
bool | SetSphereRadius (double coll_radius, double out_envelope) |
If the collision shape is a sphere, resize it and return true (if no sphere is found in this collision shape, return false). More... | |
virtual ChCoordsys | GetShapePos (int index) const override |
Return the position and orientation of the collision shape with specified index, relative to the model frame. | |
virtual std::vector< double > | GetShapeDimensions (int index) const override |
Return shape characteristic dimensions. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
virtual bool | AddConvexHullsFromFile (std::shared_ptr< ChMaterialSurface > material, ChStreamInAscii &mstream, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) |
Add a cluster of convex hulls specified in a '.chulls' file description. More... | |
ChContactable * | GetContactable () |
Get the pointer to the contactable object. | |
virtual void | SetContactable (ChContactable *mc) |
Set the pointer to the contactable object. More... | |
virtual ChPhysicsItem * | GetPhysicsItem () |
Get the pointer to the client owner ChPhysicsItem. More... | |
virtual short int | GetFamilyGroup () const |
Return the collision family group of this model. More... | |
virtual short int | GetFamilyMask () const |
Return the collision mask for this model. More... | |
virtual void | SetSafeMargin (double amargin) |
Set the suggested collision 'inward safe margin' for the shapes to be added from now on, using the AddBox, AddCylinder etc (where, if this margin is too high for some thin or small shapes, it may be clamped). More... | |
virtual float | GetSafeMargin () |
Returns the inward safe margin (see SetSafeMargin() ) | |
virtual void | SetEnvelope (double amargin) |
Set the suggested collision outward 'envelope' (used from shapes added, from now on, to this collision model). More... | |
virtual float | GetEnvelope () |
Return the outward safe margin (see SetEnvelope() ) | |
int | GetNumShapes () const |
Return the number of collision shapes in this model. | |
const std::vector< std::shared_ptr< ChCollisionShape > > & | GetShapes () const |
Get the list of collision shapes in this model. | |
std::shared_ptr< ChCollisionShape > | GetShape (int index) |
Get the collision shape with specified index. | |
void | SetShapeMaterial (int index, std::shared_ptr< ChMaterialSurface > mat) |
Set the contact material for the collision shape with specified index. | |
void | SetAllShapesMaterial (std::shared_ptr< ChMaterialSurface > mat) |
Set the contact material for all collision shapes in the model (all shapes will share the material). More... | |
Protected Attributes | |
std::unique_ptr< btCollisionObject > | bt_collision_object |
Bullet collision object containing Bullet geometries. | |
std::shared_ptr< btCompoundShape > | bt_compound_shape |
Compound for models with more than one collision shape. | |
![]() | |
float | model_envelope |
Maximum envelope: surrounding volume from surface to the exterior. | |
float | model_safe_margin |
Maximum margin value to be used for fast penetration contact detection. | |
ChContactable * | mcontactable |
Pointer to the contactable object. | |
short int | family_group |
Collision family group. | |
short int | family_mask |
Collision family mask. | |
std::vector< std::shared_ptr< ChCollisionShape > > | m_shapes |
list of collision shapes in model | |
Friends | |
class | ChCollisionSystemBullet |
class | ChCollisionSystemBulletMulticore |
Additional Inherited Members | |
![]() | |
static void | SetDefaultSuggestedEnvelope (double menv) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision envelope (safe outward layer) as default. More... | |
static void | SetDefaultSuggestedMargin (double mmargin) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision margin (inward penetration layer) as default. More... | |
static double | GetDefaultSuggestedEnvelope () |
static double | GetDefaultSuggestedMargin () |
![]() | |
void | CopyShapes (ChCollisionModel *other) |
Copy the collision shapes from another model. | |
virtual float | GetSuggestedFullMargin () |
Member Function Documentation
◆ Add2Dpath()
|
overridevirtual |
Add a 2D closed line, defined on the XY plane passing by pos and aligned as rot, that defines a 2D collision shape that will collide with another 2D line of the same type if aligned on the same plane.
This is useful for mechanisms that work on a plane, and that require more precise collision that is not possible with current 3D shapes. For example, the line can contain concave or convex round fillets. Requirements:
- the line must be clockwise for inner material, (counterclockwise=hollow, material outside)
- the line must contain only ChLineSegment and ChLineArc sub-lines
- the sublines must follow in the proper order, with coincident corners, and must be closed.
- Parameters
-
material surface contact material mpath 2D curve path pos origin position in model coordinates rot rotation in model coordinates thickness line thickness
Reimplemented from chrono::collision::ChCollisionModel.
◆ AddBarrel()
|
overridevirtual |
Add a barrel-like shape to this collision model (main axis on Y direction).
The barrel shape is made by lathing an arc of an ellipse around the vertical Y axis. The center of the ellipse is on Y=0 level, and it is offsetted by R_offset from the Y axis in radial direction. The two radii of the ellipse are R_vert (for the vertical direction, i.e. the axis parallel to Y) and R_hor (for the axis that is perpendicular to Y). Also, the solid is clamped with two discs on the top and the bottom, at levels Y_low and Y_high.
- Parameters
-
material surface contact material Y_low bottom level Y_high top level R_vert ellipse semi-axis in vertical direction R_hor ellipse semi-axis in horizontal direction R_offset lateral offset (radius at top and bottom) pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddBox()
|
overridevirtual |
Add a box shape to this collision model.
- Parameters
-
material surface contact material hx x half-dimension hy y half-dimension hz z half-dimension pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddCapsule()
|
inlineoverridevirtual |
Add a capsule to this collision model (default axis in Y direction).
- Parameters
-
material surface contact material radius radius hlen half-length of capsule axis pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddCone()
|
inlineoverridevirtual |
Add a cone to this collision model (default axis on Y direction).
- Parameters
-
material surface contact material rx radius (X direction) rz radius (Z direction) hy half length pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddConvexHull()
|
overridevirtual |
Add a convex hull to this collision model.
A convex hull is simply a point cloud that describe a convex polytope. Connectivity between the vertexes, as faces/edges in triangle meshes is not necessary. Points are passed as a list which is then copied into the model.
- Parameters
-
material surface contact material pointlist list of hull points pos origin position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddCopyOfAnotherModel()
|
overridevirtual |
Add all shapes already contained in another model.
The 'another' model must be of ChCollisionModelBullet subclass.
Implements chrono::collision::ChCollisionModel.
◆ AddCylinder()
|
overridevirtual |
Add a cylinder to this collision model (default axis on Y direction).
- Parameters
-
material surface contact material rx radius (X direction) rz radius (Z direction) hy half length pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddEllipsoid()
|
overridevirtual |
Add an ellipsoid shape to this collision model.
- Parameters
-
material surface contact material rx x semi-axis ry y semi-axis rz z semi-axis pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddPoint()
|
overridevirtual |
Add a point-like sphere, that will collide with other geometries, but won't ever create contacts between them.
- Parameters
-
material surface contact material radius node radius pos center position in model coordinates
Reimplemented from chrono::collision::ChCollisionModel.
◆ AddRoundedBox()
|
inlineoverridevirtual |
Add a rounded box shape to this collision model.
- Parameters
-
material surface contact material hx x half-dimension hy y half-dimension hz z half-dimension sphere_r radius of sweeping sphere pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddRoundedCone()
|
inlineoverridevirtual |
Add a rounded cone to this collision model (default axis on Y direction).
- Parameters
-
material surface contact material rx radius (X direction) rz radius (Z direction) hy half length sphere_r radius of sweeping sphere pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddRoundedCylinder()
|
inlineoverridevirtual |
Add a rounded cylinder to this collision model (default axis on Y direction).
- Parameters
-
material surface contact material rx radius (X direction) rz radius (Z direction) hy half length sphere_r radius of sweeping sphere pos center position in model coordinates rot rotation in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddSphere()
|
overridevirtual |
Add a sphere shape to this collision model.
- Parameters
-
material surface contact material radius sphere radius pos center position in model coordinates
Implements chrono::collision::ChCollisionModel.
◆ AddTriangleMesh()
|
overridevirtual |
Add a triangle mesh to this collision model.
Note: if possible, for better performance, avoid triangle meshes and prefer simplified representations as compounds of primitive convex shapes (boxes, sphers, etc).
- Parameters
-
material surface contact material trimesh the triangle mesh is_static true if model doesn't move. May improve performance. is_convex if true, a convex hull is used. May improve robustness. pos origin position in model coordinates rot rotation in model coordinates sphereswept_thickness outward sphere-swept layer (when supported)
Implements chrono::collision::ChCollisionModel.
◆ AddTriangleMeshConcave()
|
virtual |
CUSTOM for this class only: add a concave triangle mesh that will be managed by GImpact mesh-mesh algorithm.
Note that, despite this can work with arbitrary meshes, there could be issues of robustness and precision, so when possible, prefer simplified representations as compounds of convex shapes of boxes/spheres/etc.. type.
- Parameters
-
material surface contact material trimesh the triangle mesh pos origin position in model coordinates rot rotation in model coordinates
◆ AddTriangleMeshConcaveDecomposed()
|
virtual |
CUSTOM for this class only: add a concave triangle mesh that will be decomposed into a compound of convex shapes.
Decomposition could be more efficient than AddTriangleMeshConcave(), but preprocessing decomposition might take a while, and decomposition result is often approximate. Therefore, despite this can work with arbitrary meshes, there could be issues of robustness and precision, so when possible, prefer simplified representations as compounds of convex shapes of boxes/spheres/etc.. type.
- Parameters
-
material surface contact material mydecomposition convex mesh decomposition pos origin position in model coordinates rot rotation in model coordinates
◆ AddTriangleProxy()
|
virtual |
Add a triangle from mesh.
For efficiency, points are stored as pointers. Thus, the user must take care of memory management and of dangling pointers.
- Parameters
-
material surface contact material p1 points to vertex1 coords p2 points to vertex2 coords p3 points to vertex3 coords ep1 points to neighbouring vertex at edge1 if any ep2 points to neighbouring vertex at edge1 if any ep3 points to neighbouring vertex at edge1 if any mowns_vertex_1 vertex is owned by this triangle (otherwise, owned by neighbour) mowns_vertex_2 vertex is owned by this triangle (otherwise, owned by neighbour) mowns_vertex_3 vertex is owned by this triangle (otherwise, owned by neighbour) mowns_edge_1 edge is owned by this triangle (otherwise, owned by neighbour) mowns_edge_2 edge is owned by this triangle (otherwise, owned by neighbour) mowns_edge_3 edge is owned by this triangle (otherwise, owned by neighbour) msphereswept_rad sphere swept triangle ('fat' triangle, improves robustness)
◆ BuildModel()
|
overridevirtual |
Complete the construction of the collision model (build the BV hierarchy).
Addition of collision shapes must be done between calls to ClearModel() and BuildModel(). This function must be invoked after all geometric collision shapes have been added.
Implements chrono::collision::ChCollisionModel.
◆ ClearModel()
|
overridevirtual |
Delete all inserted geometries.
Addition of collision shapes must be done between calls to ClearModel() and BuildModel(). This function must be invoked before adding geometric collision shapes.
Implements chrono::collision::ChCollisionModel.
◆ GetAABB()
|
overridevirtual |
Returns the axis aligned bounding box (AABB) of the collision model, i.e.
max-min along the x,y,z world axes. Remember that SyncPosition() should be invoked before calling this.
Implements chrono::collision::ChCollisionModel.
◆ GetFamilyMaskDoesCollisionWithFamily()
|
overridevirtual |
Tells if the family mask of this collision object allows for the collision with another collision object belonging to a given family.
NOTE: this function has NO effect if used before you add the body to a ChSystem, using AddBody(). This default implementation uses the family mask.
Reimplemented from chrono::collision::ChCollisionModel.
◆ GetShapeDimensions()
|
overridevirtual |
Return shape characteristic dimensions.
The following collision shapes are supported:
SPHERE radius BOX x-halfdim y-halfdim z-halfdim ELLIPSOID x-radius y-radius z-radius CYLINDER x-radius z-radius halflength
Implements chrono::collision::ChCollisionModel.
◆ SetFamily()
|
overridevirtual |
By default, all collision objects belong to family n.0, but you can set family in range 0..15.
This is used when the objects collided with another: the contact is created only if the family is within the 'family mask' of the other, and viceversa. NOTE: these functions have NO effect if used before you add the body to a ChSystem, using AddBody(). Use after AddBody(). These default implementations use the family group.
Reimplemented from chrono::collision::ChCollisionModel.
◆ SetFamilyGroup()
|
overridevirtual |
Set the collision family group of this model.
This is an alternative way of specifying the collision family for this object. The value family_group must have a single bit set (i.e. it must be a power of 2). The corresponding family is then the bit position.
Reimplemented from chrono::collision::ChCollisionModel.
◆ SetFamilyMask()
|
overridevirtual |
Set the collision mask for this model.
Any set bit in the specified mask indicates that this model collides with all objects whose family is equal to the bit position.
Reimplemented from chrono::collision::ChCollisionModel.
◆ SetFamilyMaskNoCollisionWithFamily()
|
overridevirtual |
By default, family mask is all turned on, so all families can collide with this object, but you can turn on-off some bytes of this mask so that some families do not collide.
When two objects collide, the contact is created only if the family is within the 'family mask' of the other, and viceversa. NOTE: these functions have NO effect if used before you add the body to a ChSystem, using AddBody(). Use after AddBody(). These default implementations use the family mask.
Reimplemented from chrono::collision::ChCollisionModel.
◆ SetSphereRadius()
bool chrono::collision::ChCollisionModelBullet::SetSphereRadius | ( | double | coll_radius, |
double | out_envelope | ||
) |
If the collision shape is a sphere, resize it and return true (if no sphere is found in this collision shape, return false).
It can also change the outward envelope; the inward margin is automatically the radius of the sphere.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/collision/ChCollisionModelBullet.h
- /builds/uwsbel/chrono/src/chrono/collision/ChCollisionModelBullet.cpp