Description
Deformable terrain model.
This class implements a deformable terrain based on the Soil Contact Model. Unlike RigidTerrain, the vertical coordinates of this terrain mesh can be deformed due to interaction with ground vehicles or other collision shapes.
#include <SCMDeformableTerrain.h>
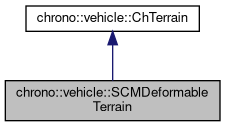
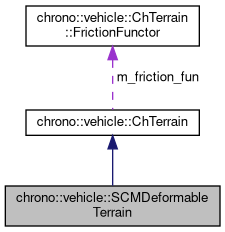
Classes | |
class | SoilParametersCallback |
Class to be used as a callback interface for location-dependent soil parameters. More... | |
Public Member Functions | |
SCMDeformableTerrain (ChSystem *system) | |
Construct a default SCMDeformableSoil. More... | |
void | SetPlane (ChCoordsys<> mplane) |
Set the plane reference. More... | |
void | SetSoilParameters (double Bekker_Kphi, double Bekker_Kc, double Bekker_n, double Mohr_cohesion, double Mohr_friction, double Janosi_shear, double elastic_K, double damping_R) |
Set the properties of the SCM soil model. More... | |
void | SetBulldozingFlow (bool mb) |
Enable/disable the creation of soil inflation at the side of the ruts (bulldozing effects). | |
bool | GetBulldozingFlow () const |
void | SetBulldozingParameters (double mbulldozing_erosion_angle, double mbulldozing_flow_factor=1.0, int mbulldozing_erosion_n_iterations=3, int mbulldozing_erosion_n_propagations=10) |
Set parameters controlling the creation of side ruts (bulldozing effects). More... | |
void | SetAutomaticRefinement (bool mr) |
Enable/disable dynamic mesh refinement. More... | |
bool | GetAutomaticRefinement () const |
void | SetAutomaticRefinementResolution (double mr) |
Set the resolution for automatic mesh refinement (specified in meters). More... | |
double | GetAutomaticRefinementResolution () const |
void | SetTestHighOffset (double moff) |
Set the vertical level up to which collision is tested (relative to the reference level at the sample point). More... | |
double | GetTestHighOffset () const |
void | SetPlotType (DataPlotType mplot, double mmin, double mmax) |
Set the color plot type for the soil mesh. More... | |
void | SetColor (ChColor color) |
Set visualization color. More... | |
void | SetTexture (const std::string tex_file, float tex_scale_x=1, float tex_scale_y=1) |
Set texture properties. More... | |
void | EnableMovingPatch (std::shared_ptr< ChBody > body, const ChVector<> &point_on_body, double dimX, double dimY) |
Enable moving patch an set parameters (default: disabled). More... | |
void | RegisterSoilParametersCallback (SoilParametersCallback *cb) |
Specify the callback object to set the soil parameters at given (x,y) locations. More... | |
virtual double | GetHeight (double x, double y) const override |
Get the terrain height at the specified (x,y) location. | |
virtual chrono::ChVector | GetNormal (double x, double y) const override |
Get the terrain normal at the specified (x,y) location. | |
virtual float | GetCoefficientFriction (double x, double y) const override |
Get the coefficient of friction at the specified (x,y) location. More... | |
const ChCoordsys & | GetPlane () const |
Get the current reference plane. The SCM terrain patch is in the (x,y) plane with normal along the Z axis. | |
const std::shared_ptr< ChTriangleMeshShape > | GetMesh () const |
Get the underlyinh triangular mesh. | |
void | Initialize (double height, double sizeX, double sizeY, int divX, int divY) |
Initialize the terrain system (flat). More... | |
void | Initialize (const std::string &mesh_file) |
Initialize the terrain system (mesh). More... | |
void | Initialize (const std::string &heightmap_file, const std::string &mesh_name, double sizeX, double sizeY, double hMin, double hMax) |
Initialize the terrain system (height map). More... | |
TerrainForce | GetContactForce (std::shared_ptr< ChBody > body) const |
Return the current cumulative contact force on the specified body (due to interaction with the SCM terrain). | |
void | PrintStepStatistics (std::ostream &os) const |
Print timing and counter information for last step. | |
![]() | |
virtual void | Synchronize (double time) |
Update the state of the terrain system at the specified time. | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
void | RegisterFrictionFunctor (FrictionFunctor *functor) |
Specify the functor object to provide the coefficient of friction at given (x,y) locations. | |
Additional Inherited Members | |
![]() | |
FrictionFunctor * | m_friction_fun |
functor for location-dependent coefficient of friction | |
Constructor & Destructor Documentation
◆ SCMDeformableTerrain()
chrono::vehicle::SCMDeformableTerrain::SCMDeformableTerrain | ( | ChSystem * | system | ) |
Construct a default SCMDeformableSoil.
The user is responsible for calling various Set methods before Initialize.
- Parameters
-
[in] system pointer to the containing multibody system
Member Function Documentation
◆ EnableMovingPatch()
void chrono::vehicle::SCMDeformableTerrain::EnableMovingPatch | ( | std::shared_ptr< ChBody > | body, |
const ChVector<> & | point_on_body, | ||
double | dimX, | ||
double | dimY | ||
) |
Enable moving patch an set parameters (default: disabled).
If enabled, ray-casting is performed only for the SCM vertices that are within the specified range (dimX, dimY) of the given point on the specified reference body.
- Parameters
-
[in] body monitored body [in] point_on_body patch center, relative to body [in] dimX patch X dimension [in] dimY patch Y dimension
◆ GetCoefficientFriction()
|
overridevirtual |
Get the coefficient of friction at the specified (x,y) location.
This coefficient of friction value may be used by certain tire models to modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it). For SCMDeformableTerrain, this function defers to the user-provided functor object of type ChTerrain::FrictionFunctor, if one was specified. Otherwise, it returns the constant value of 0.8.
Implements chrono::vehicle::ChTerrain.
◆ Initialize() [1/3]
void chrono::vehicle::SCMDeformableTerrain::Initialize | ( | const std::string & | heightmap_file, |
const std::string & | mesh_name, | ||
double | sizeX, | ||
double | sizeY, | ||
double | hMin, | ||
double | hMax | ||
) |
Initialize the terrain system (height map).
The initial undeformed mesh is provided via the specified BMP file as a height map
- Parameters
-
[in] heightmap_file filename for the height map (BMP) [in] mesh_name name of the mesh asset [in] sizeX terrain dimension in the X direction [in] sizeY terrain dimension in the Y direction [in] hMin minimum height (black level) [in] hMax maximum height (white level)
◆ Initialize() [2/3]
void chrono::vehicle::SCMDeformableTerrain::Initialize | ( | const std::string & | mesh_file | ) |
Initialize the terrain system (mesh).
The initial undeformed mesh is provided via a Wavefront .obj file.
- Parameters
-
[in] mesh_file filename of the input mesh (.OBJ file in Wavefront format)
◆ Initialize() [3/3]
void chrono::vehicle::SCMDeformableTerrain::Initialize | ( | double | height, |
double | sizeX, | ||
double | sizeY, | ||
int | divX, | ||
int | divY | ||
) |
Initialize the terrain system (flat).
This version creates a flat array of points.
- Parameters
-
[in] height terrain height [in] sizeX terrain dimension in the X direction [in] sizeY terrain dimension in the Y direction [in] divX number of divisions in the X direction [in] divY number of divisions in the Y direction
◆ RegisterSoilParametersCallback()
void chrono::vehicle::SCMDeformableTerrain::RegisterSoilParametersCallback | ( | SoilParametersCallback * | cb | ) |
Specify the callback object to set the soil parameters at given (x,y) locations.
To use constant soil parameters throughout the entire patch, use SetSoilParameters.
◆ SetAutomaticRefinement()
void chrono::vehicle::SCMDeformableTerrain::SetAutomaticRefinement | ( | bool | mr | ) |
Enable/disable dynamic mesh refinement.
If enabled, additional points are added under contact patches, up to the desired resolution.
◆ SetAutomaticRefinementResolution()
void chrono::vehicle::SCMDeformableTerrain::SetAutomaticRefinementResolution | ( | double | mr | ) |
Set the resolution for automatic mesh refinement (specified in meters).
The mesh is refined, as needed, until the largest side of a triangle reaches trhe specified resolution. Triangles out of contact patches are not refined.
◆ SetBulldozingParameters()
void chrono::vehicle::SCMDeformableTerrain::SetBulldozingParameters | ( | double | mbulldozing_erosion_angle, |
double | mbulldozing_flow_factor = 1.0 , |
||
int | mbulldozing_erosion_n_iterations = 3 , |
||
int | mbulldozing_erosion_n_propagations = 10 |
||
) |
Set parameters controlling the creation of side ruts (bulldozing effects).
- Parameters
-
mbulldozing_erosion_angle angle of erosion of the displaced material (in degrees!) mbulldozing_flow_factor growth of lateral volume respect to pressed volume mbulldozing_erosion_n_iterations number of erosion refinements per timestep mbulldozing_erosion_n_propagations number of concentric vertex selections subject to erosion
◆ SetColor()
void chrono::vehicle::SCMDeformableTerrain::SetColor | ( | ChColor | color | ) |
Set visualization color.
- Parameters
-
[in] color color of the visualization material
◆ SetPlane()
void chrono::vehicle::SCMDeformableTerrain::SetPlane | ( | ChCoordsys<> | mplane | ) |
Set the plane reference.
By default, the reference plane is horizontal with Z up (ISO vehicle reference frame). To set as Y up, call SetPlane(ChCoordys(VNULL, Q_from_AngX(-CH_C_PI_2)));
◆ SetPlotType()
void chrono::vehicle::SCMDeformableTerrain::SetPlotType | ( | DataPlotType | mplot, |
double | mmin, | ||
double | mmax | ||
) |
Set the color plot type for the soil mesh.
When a scalar plot is used, also define the range in the pseudo-color colormap.
◆ SetSoilParameters()
void chrono::vehicle::SCMDeformableTerrain::SetSoilParameters | ( | double | Bekker_Kphi, |
double | Bekker_Kc, | ||
double | Bekker_n, | ||
double | Mohr_cohesion, | ||
double | Mohr_friction, | ||
double | Janosi_shear, | ||
double | elastic_K, | ||
double | damping_R | ||
) |
Set the properties of the SCM soil model.
These parameters are described in: "Parameter Identification of a Planetary Rover Wheel-Soil Contact Model via a Bayesian Approach", A.Gallina, R. Krenn et al. Note that the original SCM model does not include the K and R coefficients. A very large value of K and R=0 reproduce the original SCM.
- Parameters
-
Bekker_Kphi Kphi, frictional modulus in Bekker model Bekker_Kc Kc, cohesive modulus in Bekker model Bekker_n n, exponent of sinkage in Bekker model (usually 0.6...1.8) Mohr_cohesion Cohesion in, Pa, for shear failure Mohr_friction Friction angle (in degrees!), for shear failure Janosi_shear J , shear parameter, in meters, in Janosi-Hanamoto formula (usually few mm or cm) elastic_K elastic stiffness K, per unit area, [Pa/m] (must be larger than Kphi) damping_R vertical damping R, per unit area [Pa s/m] (proportional to vertical speed)
◆ SetTestHighOffset()
void chrono::vehicle::SCMDeformableTerrain::SetTestHighOffset | ( | double | moff | ) |
Set the vertical level up to which collision is tested (relative to the reference level at the sample point).
Since the contact is unilateral, this could be zero. However, when computing bulldozing flow, one might also need to know if in the surrounding there is some potential future contact: so it might be better to use a positive value (but not higher than the max. expected height of the bulldozed rubble, to avoid slowdown of collision tests).
◆ SetTexture()
void chrono::vehicle::SCMDeformableTerrain::SetTexture | ( | const std::string | tex_file, |
float | tex_scale_x = 1 , |
||
float | tex_scale_y = 1 |
||
) |
Set texture properties.
- Parameters
-
[in] tex_file texture filename [in] tex_scale_x texture scale in X [in] tex_scale_y texture scale in Y
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/SCMDeformableTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/SCMDeformableTerrain.cpp