Description
Base class for a Pitman Arm steering subsystem.
Derived from ChSteering, but still an abstract base class.
The steering subsystem is modeled with respect to a right-handed frame with with X pointing towards the front, Y to the left, and Z up (ISO standard).
When attached to a chassis, both an offset and a rotation (as a quaternion) are provided.
#include <ChPitmanArm.h>
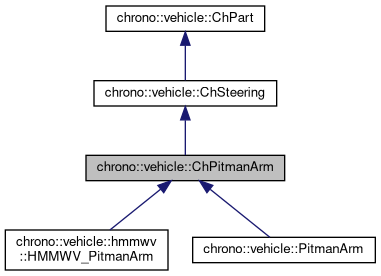
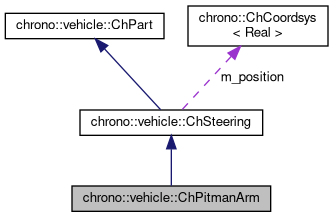
Public Member Functions | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual void | Initialize (std::shared_ptr< ChBodyAuxRef > chassis, const ChVector<> &location, const ChQuaternion<> &rotation) override |
Initialize this steering subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the steering subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the steering subsystem. | |
virtual void | Synchronize (double time, double steering) override |
Update the state of this steering subsystem at the current time. More... | |
virtual double | GetMass () const override |
Get the total mass of the steering subsystem. | |
virtual ChVector | GetCOMPos () const override |
Get the current global COM location of the steering subsystem. | |
virtual void | LogConstraintViolations () override |
Log current constraint violations. | |
![]() | |
ChSteering (const std::string &name) | |
const ChCoordsys & | GetPosition () const |
Get the position (location and orientation) of the steering subsystem relative to the chassis reference frame. | |
std::shared_ptr< ChBody > | GetSteeringLink () const |
Get a handle to the main link of the steering subsystems. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
void | SetContactFrictionCoefficient (float friction_coefficient) |
Set coefficient of friction. More... | |
void | SetContactRestitutionCoefficient (float restitution_coefficient) |
Set coefficient of restitution. More... | |
void | SetContactMaterialProperties (float young_modulus, float poisson_ratio) |
Set contact material properties. More... | |
void | SetContactMaterialCoefficients (float kn, float gn, float kt, float gt) |
Set contact material coefficients. More... | |
float | GetCoefficientFriction () const |
Get coefficient of friction for contact material. | |
float | GetCoefficientRestitution () const |
Get coefficient of restitution for contact material. | |
float | GetYoungModulus () const |
Get Young's modulus of elasticity for contact material. | |
float | GetPoissonRatio () const |
Get Poisson ratio for contact material. | |
float | GetKn () const |
Get normal stiffness coefficient for contact material. | |
float | GetKt () const |
Get tangential stiffness coefficient for contact material. | |
float | GetGn () const |
Get normal viscous damping coefficient for contact material. | |
float | GetGt () const |
Get tangential viscous damping coefficient for contact material. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Types | |
enum | PointId { STEERINGLINK, PITMANARM, REV, UNIV, REVSPH_R, REVSPH_S, TIEROD_PA, TIEROD_IA, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
enum | DirectionId { REV_AXIS, UNIV_AXIS_ARM, UNIV_AXIS_LINK, REVSPH_AXIS, NUM_DIRS } |
Identifiers for the various direction unit vectors. More... | |
Protected Member Functions | |
ChPitmanArm (const std::string &name, bool vehicle_frame_inertia=false) | |
Protected constructor. More... | |
void | SetVehicleFrameInertiaFlag (bool val) |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false). More... | |
virtual const ChVector | getLocation (PointId which)=0 |
Return the location of the specified hardpoint. More... | |
virtual const ChVector | getDirection (DirectionId which)=0 |
Return the unit vector for the specified direction. More... | |
virtual double | getSteeringLinkMass () const =0 |
Return the mass of the steering link body. | |
virtual double | getPitmanArmMass () const =0 |
Return the mass of the Pitman arm body. | |
virtual const ChVector & | getSteeringLinkInertiaMoments () const =0 |
Return the moments of inertia of the steering link body. | |
virtual const ChVector & | getSteeringLinkInertiaProducts () const =0 |
Return the products of inertia of the steering link body. | |
virtual const ChVector & | getPitmanArmInertiaMoments () const =0 |
Return the moments of inertia of the Pitman arm body. | |
virtual const ChVector & | getPitmanArmInertiaProducts () const =0 |
Return the products of inertia of the Pitman arm body. | |
virtual double | getSteeringLinkRadius () const =0 |
Return the radius of the steering link body (visualization only). | |
virtual double | getPitmanArmRadius () const =0 |
Return the radius of the Pitman arm body (visualization only). | |
virtual double | getMaxAngle () const =0 |
Return the maximum rotation angle of the revolute joint. | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
Protected Attributes | |
std::shared_ptr< ChBody > | m_arm |
handle to the Pitman arm body | |
std::shared_ptr< ChLinkMotorRotationAngle > | m_revolute |
handle to the chassis-arm revolute joint | |
std::shared_ptr< ChLinkRevoluteSpherical > | m_revsph |
handle to the revolute-spherical joint (idler arm) | |
std::shared_ptr< ChLinkUniversal > | m_universal |
handle to the arm-link universal joint | |
![]() | |
ChCoordsys | m_position |
location and orientation relative to chassis | |
std::shared_ptr< ChBody > | m_link |
handle to the main steering link | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
float | m_friction |
contact coefficient of friction | |
float | m_restitution |
contact coefficient of restitution | |
float | m_young_modulus |
contact material Young modulus | |
float | m_poisson_ratio |
contact material Poisson ratio | |
float | m_kn |
normal contact stiffness | |
float | m_gn |
normal contact damping | |
float | m_kt |
tangential contact stiffness | |
float | m_gt |
tangential contact damping | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
Member Enumeration Documentation
◆ DirectionId
|
protected |
◆ PointId
|
protected |
Identifiers for the various hardpoints.
Constructor & Destructor Documentation
◆ ChPitmanArm()
|
protected |
Protected constructor.
- Parameters
-
[in] name name of the subsystem [in] vehicle_frame_inertia inertia specified in vehicle-aligned centroidal frames?
Member Function Documentation
◆ AddVisualizationAssets()
|
overridevirtual |
Add visualization assets for the steering subsystem.
This default implementation uses primitives.
Reimplemented from chrono::vehicle::ChPart.
◆ getDirection()
|
protectedpure virtual |
Return the unit vector for the specified direction.
The returned vector must be expressed in the suspension reference frame.
Implemented in chrono::vehicle::PitmanArm, and chrono::vehicle::hmmwv::HMMWV_PitmanArm.
◆ getLocation()
|
protectedpure virtual |
Return the location of the specified hardpoint.
The returned location must be expressed in the suspension reference frame.
Implemented in chrono::vehicle::PitmanArm, and chrono::vehicle::hmmwv::HMMWV_PitmanArm.
◆ Initialize()
|
overridevirtual |
Initialize this steering subsystem.
The steering subsystem is initialized by attaching it to the specified chassis body at the specified location (with respect to and expressed in the reference frame of the chassis) and with specified orientation (with respect to the chassis reference frame).
- Parameters
-
[in] chassis handle to the chassis body [in] location location relative to the chassis frame [in] rotation orientation relative to the chassis frame
Implements chrono::vehicle::ChSteering.
◆ SetVehicleFrameInertiaFlag()
|
inlineprotected |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false).
Note that this function must be called before Initialize().
◆ Synchronize()
|
overridevirtual |
Update the state of this steering subsystem at the current time.
The steering subsystem is provided the current steering driver input (a value between -1 and +1). Positive steering input indicates steering to the left. This function is called during the vehicle update.
- Parameters
-
[in] time current time [in] steering current steering input [-1,+1]
Implements chrono::vehicle::ChSteering.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/steering/ChPitmanArm.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/steering/ChPitmanArm.cpp