Description
Class for geometric model for collision detection.
A rigid body that interacts through contact must have a collision model.
#include <ChCollisionModelParallel.h>
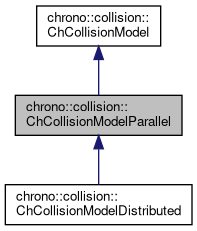
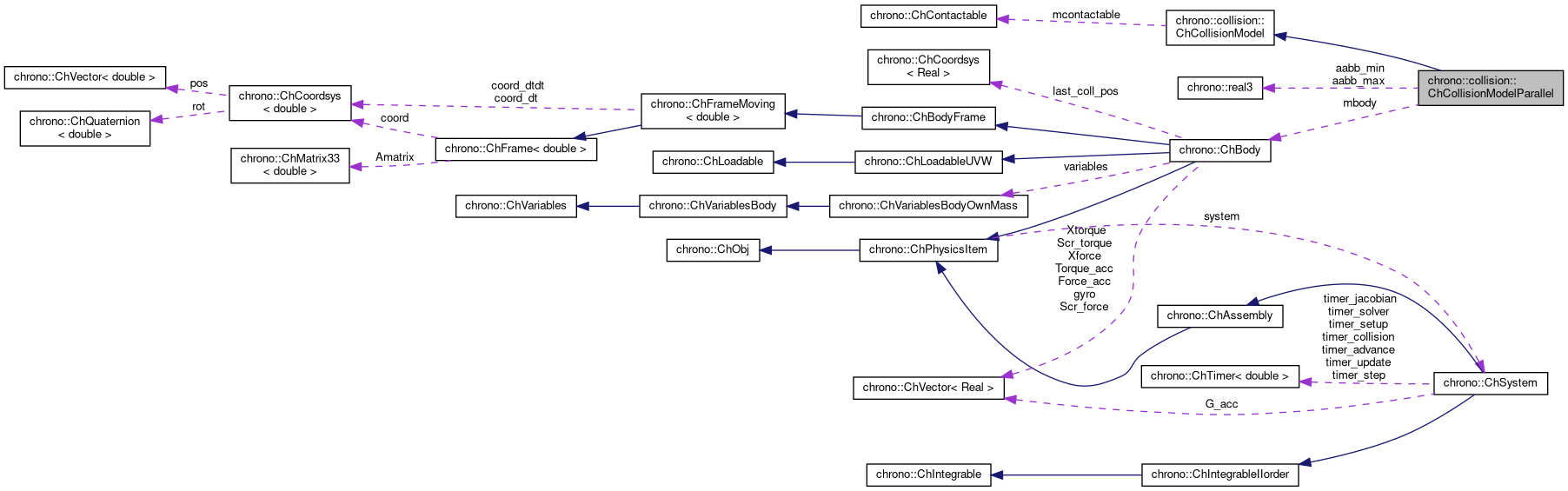
Public Member Functions | |
virtual int | ClearModel () override |
Deletes all inserted geometries. More... | |
virtual int | BuildModel () override |
Builds the BV hierarchy. More... | |
virtual void | SyncPosition () override |
Sets the position and orientation of the collision model as the rigid body current position. | |
virtual void | SetContactable (ChContactable *mc) override |
Sets the pointer to the contactable object. | |
virtual bool | AddSphere (double radius, const ChVector<> &pos=ChVector<>()) override |
Add a sphere shape to this model, for collision purposes. | |
virtual bool | AddEllipsoid (double rx, double ry, double rz, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add an ellipsoid shape to this model, for collision purposes. | |
virtual bool | AddBox (double hx, double hy, double hz, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a box shape to this model, for collision purposes. | |
virtual bool | AddRoundedBox (double hx, double hy, double hz, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a rounded box shape to this model, for collision purposes. | |
virtual bool | AddTriangle (ChVector<> A, ChVector<> B, ChVector<> C, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) |
Add a triangle shape to this model, for collision purposes. | |
virtual bool | AddCylinder (double rx, double ry, double rz, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a cylinder to this model (default axis on Y direction), for collision purposes. | |
virtual bool | AddRoundedCylinder (double rx, double rz, double hy, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a rounded cylinder to this model (default axis on Y direction), for collision purposes. | |
virtual bool | AddCone (double rx, double rz, double hy, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a cone to this model (default axis on Y direction), for collision purposes. | |
virtual bool | AddRoundedCone (double rx, double rz, double hy, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a rounded cone to this model (default axis on Y direction), for collision purposes. | |
virtual bool | AddCapsule (double radius, double hlen, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a capsule to this model (default axis in Y direction), for collision purposes. | |
virtual bool | AddConvexHull (const std::vector< ChVector< double > > &pointlist, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a convex hull to this model. More... | |
virtual bool | AddTriangleMesh (std::shared_ptr< geometry::ChTriangleMesh > trimesh, bool is_static, bool is_convex, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1), double sphereswept_thickness=0.0) override |
Add a triangle mesh to this model, passing a triangle mesh. More... | |
virtual bool | AddBarrel (double Y_low, double Y_high, double R_vert, double R_hor, double R_offset, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) override |
Add a barrel-like shape to this model (main axis on Y direction), for collision purposes. More... | |
virtual bool | AddCopyOfAnotherModel (ChCollisionModel *another) override |
Add all shapes already contained in another model. More... | |
virtual void | GetAABB (ChVector<> &bbmin, ChVector<> &bbmax) const override |
Return the axis aligned bounding box for this collision model. | |
ChBody * | GetBody () const |
Return a pointer to the associated body. | |
void | SetBody (ChBody *body) |
Set the pointer to the owner rigid body. | |
int | GetNObjects () const |
Return the number of objects in this model. | |
![]() | |
virtual bool | Add2Dpath (std::shared_ptr< geometry::ChLinePath > mpath, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1), const double thickness=0.001) |
Add a 2D closed line, defined on the XY plane passing by pos and aligned as rot, that defines a 2D collision shape that will collide with another 2D line of the same type if aligned on the same plane. More... | |
virtual bool | AddPoint (double radius=0, const ChVector<> &pos=ChVector<>()) |
Add a point-like sphere, that will collide with other geometries, but won't ever create contacts between them. More... | |
virtual bool | AddConvexHullsFromFile (ChStreamInAscii &mstream, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) |
Add a cluster of convex hulls by a '.chulls' file description. More... | |
ChContactable * | GetContactable () |
Gets the pointer to the contactable object. | |
virtual ChPhysicsItem * | GetPhysicsItem () |
Gets the pointer to the client owner ChPhysicsItem. More... | |
virtual void | SetFamily (int mfamily) |
By default, all collision objects belong to family n.0, but you can set family in range 0..15. More... | |
virtual int | GetFamily () |
virtual void | SetFamilyMaskNoCollisionWithFamily (int mfamily) |
By default, family mask is all turned on, so all families can collide with this object, but you can turn on-off some bytes of this mask so that some families do not collide. More... | |
virtual void | SetFamilyMaskDoCollisionWithFamily (int mfamily) |
virtual bool | GetFamilyMaskDoesCollisionWithFamily (int mfamily) |
Tells if the family mask of this collision object allows for the collision with another collision object belonging to a given family. More... | |
virtual short int | GetFamilyGroup () const |
Return the collision family group of this model. More... | |
virtual void | SetFamilyGroup (short int group) |
Set the collision family group of this model. More... | |
virtual short int | GetFamilyMask () const |
Return the collision mask for this model. More... | |
virtual void | SetFamilyMask (short int mask) |
Set the collision mask for this model. More... | |
virtual void | SetSafeMargin (double amargin) |
Sets the suggested collision 'inward safe margin' for the shapes to be added from now on, using the AddBox, AddCylinder etc (where, if this margin is too high for some thin or small shapes, it may be clamped). More... | |
virtual float | GetSafeMargin () |
Returns the inward safe margin (see SetSafeMargin() ) | |
virtual void | SetEnvelope (double amargin) |
Sets the suggested collision outward 'envelope' (used from shapes added, from now on, to this collision model). More... | |
virtual float | GetEnvelope () |
Returns the outward safe margin (see SetEnvelope() ) | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
Public Attributes | |
std::vector< ConvexModel > | mData |
std::vector< real3 > | local_convex_data |
real3 | aabb_min |
real3 | aabb_max |
Protected Attributes | |
ChBody * | mbody |
unsigned int | nObjects |
![]() | |
float | model_envelope |
float | model_safe_margin |
ChContactable * | mcontactable |
short int | family_group |
short int | family_mask |
Additional Inherited Members | |
![]() | |
static void | SetDefaultSuggestedEnvelope (double menv) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision envelope (safe outward layer) as default. More... | |
static void | SetDefaultSuggestedMargin (double mmargin) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision margin (inward penetration layer) as default. More... | |
static double | GetDefaultSuggestedEnvelope () |
static double | GetDefaultSuggestedMargin () |
![]() | |
virtual float | GetSuggestedFullMargin () |
Member Function Documentation
◆ AddBarrel()
|
overridevirtual |
Add a barrel-like shape to this model (main axis on Y direction), for collision purposes.
The barrel shape is made by lathing an arc of an ellipse around the vertical Y axis. The center of the ellipse is on Y=0 level, and it is ofsetted by R_offset from the Y axis in radial direction. The two radii of the ellipse are R_vert (for the vertical direction, i.e. the axis parellel to Y) and R_hor (for the axis that is perpendicular to Y). Also, the solid is clamped with two discs on the top and the bottom, at levels Y_low and Y_high. Currently not supported.
Implements chrono::collision::ChCollisionModel.
◆ AddConvexHull()
|
overridevirtual |
Add a convex hull to this model.
A convex hull is simply a point cloud that describe a convex polytope. Connectivity between the vertexes, as faces/edges in triangle meshes is not necessary. Points are passed as a list, that is instantly copied into the model.
Implements chrono::collision::ChCollisionModel.
◆ AddCopyOfAnotherModel()
|
overridevirtual |
Add all shapes already contained in another model.
Thank to the adoption of shared pointers, underlying shapes are shared (not copied) among the models; this will save memory when you must simulate thousands of objects with the same collision shape. The 'another' model must be of ChModelBullet subclass.
Implements chrono::collision::ChCollisionModel.
◆ AddTriangleMesh()
|
overridevirtual |
Add a triangle mesh to this model, passing a triangle mesh.
Add a triangle mesh to this model.
Note: if possible, for better performance, avoid triangle meshes and prefer simplified representations as compounds of primitive convex shapes (boxes, sphers, etc).
- Parameters
-
trimesh the triangle mesh is_static true if model doesn't move. May improve performance. is_convex if true, a convex hull is used. May improve robustness. pos displacement respect to COG rot the rotation of the mesh sphereswept_thickness outward sphere-swept layer (when supported)
Implements chrono::collision::ChCollisionModel.
◆ BuildModel()
|
overridevirtual |
Builds the BV hierarchy.
Call this function AFTER adding the geometric description. MUST be inherited by child classes! (ex for bulding BV hierarchies)
Implements chrono::collision::ChCollisionModel.
◆ ClearModel()
|
overridevirtual |
Deletes all inserted geometries.
Also, if you begin the definition of a model, AFTER adding the geometric description, remember to call the ClearModel(). MUST be inherited by child classes! (ex for resetting also BV hierarchies)
Implements chrono::collision::ChCollisionModel.
Reimplemented in chrono::collision::ChCollisionModelDistributed.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_parallel/collision/ChCollisionModelParallel.h
- /builds/uwsbel/chrono/src/chrono_parallel/collision/ChCollisionModelParallel.cpp