Description
Definition of a track assembly.
A track assembly consists of a sprocket, an idler (with tensioner mechanism), a set of rollers, a set of suspensions (road-wheel assemblies), and a collection of track shoes.
#include <ChTrackAssembly.h>
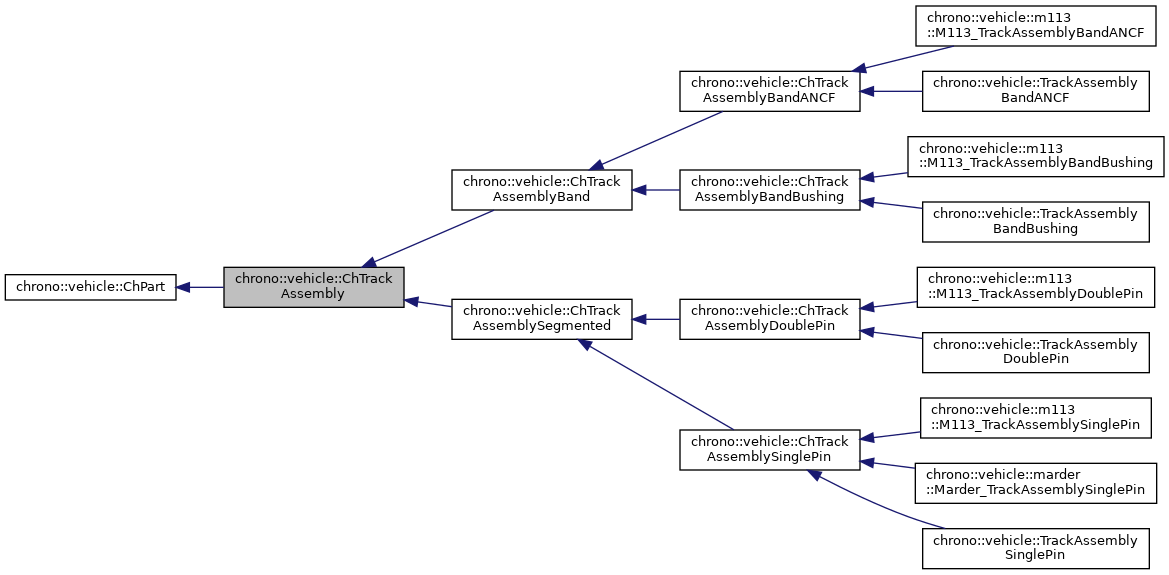
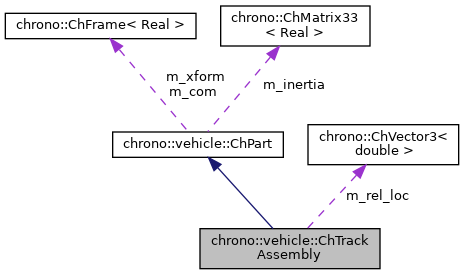
Public Member Functions | |
VehicleSide | GetVehicleSide () const |
Return the vehicle side for this track assembly. | |
size_t | GetNumTrackSuspensions () const |
Get the number of suspensions. | |
size_t | GetNumRollers () const |
Get the number of rollers. | |
virtual size_t | GetNumTrackShoes () const =0 |
Get the number of track shoes. | |
virtual std::shared_ptr< ChSprocket > | GetSprocket () const =0 |
Get a handle to the sprocket. | |
std::shared_ptr< ChIdler > | GetIdler () const |
Get a handle to the idler subsystem. | |
std::shared_ptr< ChTrackWheel > | GetIdlerWheel () const |
Get a handle to the idelr wheel subsystem. | |
std::shared_ptr< ChTrackBrake > | GetBrake () const |
Get a handle to the brake subsystem. | |
const ChTrackSuspensionList & | GetTrackSuspensions () const |
Get the list of suspension subsystems. | |
std::shared_ptr< ChTrackSuspension > | GetTrackSuspension (size_t id) const |
Get a handle to the specified suspension subsystem. | |
std::shared_ptr< ChTrackWheel > | GetRoller (size_t id) const |
Get a handle to the specified roller subsystem. | |
std::shared_ptr< ChTrackWheel > | GetRoadWheel (size_t id) const |
Get a handle to the specified road wheel subsystem. | |
virtual std::shared_ptr< ChTrackShoe > | GetTrackShoe (size_t id) const =0 |
Get a handle to the specified track shoe subsystem. | |
const ChVector3d & | GetTrackShoePos (size_t id) const |
Get the global location of the specified track shoe. More... | |
const ChQuaternion & | GetTrackShoeRot (size_t id) const |
Get the orientation of the specified track shoe. More... | |
const ChVector3d & | GetTrackShoeLinVel (size_t id) const |
Get the linear velocity of the specified track shoe. More... | |
ChVector3d | GetTrackShoeAngVel (size_t id) const |
Get the angular velocity of the specified track shoe. More... | |
ChVector3d | GetTrackShoeTension (size_t id) const |
Get track tension at the specified track shoe. More... | |
BodyState | GetTrackShoeState (size_t id) const |
Get the complete state for the specified track shoe. More... | |
void | GetTrackShoeStates (BodyStates &states) const |
Get the complete states for all track shoes. More... | |
virtual const ChVector3d | GetSprocketLocation () const =0 |
Get the relative location of the sprocket subsystem. More... | |
virtual const ChVector3d | GetIdlerLocation () const =0 |
Get the relative location of the idler subsystem. More... | |
virtual const ChVector3d | GetRoadWhelAssemblyLocation (int which) const =0 |
Get the relative location of the specified suspension subsystem. More... | |
virtual const ChVector3d | GetRollerLocation (int which) const |
Get the relative location of the specified roller subsystem. More... | |
void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector3d &location, bool create_shoes=true) |
Initialize this track assembly subsystem. More... | |
double | ReportTrackLength () const |
Return total assembled track length (sum of pitch over all track shoes). | |
ChTrackSuspension::ForceTorque | ReportSuspensionForce (size_t id) const |
Return current suspension forces or torques, as appropriate (spring and shock) for the specified suspension. More... | |
void | SetSprocketVisualizationType (VisualizationType vis) |
Set visualization type for the sprocket subsystem. | |
void | SetIdlerVisualizationType (VisualizationType vis) |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystems. | |
void | SetIdlerWheelVisualizationType (VisualizationType vis) |
Set visualization type for the idler-wheel subsystems. | |
void | SetRoadWheelVisualizationType (VisualizationType vis) |
Set visualization type for the road-wheel subsystems. | |
void | SetRollerVisualizationType (VisualizationType vis) |
Set visualization type for the roller subsystems. | |
void | SetTrackShoeVisualizationType (VisualizationType vis) |
Set visualization type for the track shoe subsystems. | |
void | SetWheelCollisionType (bool roadwheel_as_cylinder, bool idler_as_cylinder, bool roller_as_cylinder) |
Set collision shape type for wheels. | |
void | Synchronize (double time, double braking) |
Update the state of this track assembly at the current time. More... | |
void | Synchronize (double time, double braking, const TerrainForces &shoe_forces) |
Update the state of this track assembly at the current time. More... | |
virtual void | Advance (double step) |
Advance the state of this track assembly by the specified time step. | |
virtual void | SetOutput (bool state) override |
Enable/disable output for this subsystem. More... | |
void | LogConstraintViolations () |
Log current constraint violations. | |
bool | IsRoadwheelCylinder () const |
bool | IsIdlerCylinder () const |
bool | IsRolerCylinder () const |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
virtual uint16_t | GetVehicleTag () const |
Get the tag of the associated vehicle. More... | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Member Functions | |
ChTrackAssembly (const std::string &name, VehicleSide side) | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual bool | Assemble (std::shared_ptr< ChChassis > chassis)=0 |
Assemble track shoes over wheels. More... | |
virtual void | RemoveTrackShoes ()=0 |
Remove all track shoes from assembly. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const override |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const override |
Output data for this subsystem's component list to the specified database. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
VehicleSide | m_side |
assembly on left/right vehicle side | |
ChVector3d | m_rel_loc |
assembly location relative to chassis | |
std::shared_ptr< ChIdler > | m_idler |
idler (and tensioner) subsystem | |
std::shared_ptr< ChTrackBrake > | m_brake |
sprocket brake | |
ChTrackSuspensionList | m_suspensions |
road-wheel assemblies | |
ChTrackWheelList | m_rollers |
roller subsystems | |
bool | m_roadwheel_as_cylinder |
bool | m_idler_as_cylinder |
bool | m_roller_as_cylinder |
std::vector< std::shared_ptr< ChLoadBodyForce > > | m_shoe_terrain_forces |
terrain force loads on track shoes | |
std::vector< std::shared_ptr< ChLoadBodyTorque > > | m_shoe_terrain_torques |
terrain torque loads on track shoes | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Friends | |
class | ChTrackedVehicle |
class | ChTrackTestRig |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Constructor & Destructor Documentation
◆ ChTrackAssembly()
|
protected |
- Parameters
-
[in] name name of the subsystem [in] side assembly on left/right vehicle side
Member Function Documentation
◆ Assemble()
|
protectedpure virtual |
Assemble track shoes over wheels.
Return true if the track shoes were initialized in a counter clockwise direction and false otherwise.
◆ ExportComponentList()
|
overrideprotectedvirtual |
Export this subsystem's component list to the specified JSON object.
Derived classes should override this function and first invoke the base class implementation, followed by calls to the various static Export***List functions, as appropriate.
Reimplemented from chrono::vehicle::ChPart.
◆ GetIdlerLocation()
|
pure virtual |
Get the relative location of the idler subsystem.
The track assembly reference frame is ISO, with origin at the sprocket center.
Implemented in chrono::vehicle::m113::M113_TrackAssemblyBandANCF, chrono::vehicle::m113::M113_TrackAssemblyDoublePin, chrono::vehicle::m113::M113_TrackAssemblySinglePin, chrono::vehicle::TrackAssemblyBandANCF, chrono::vehicle::TrackAssemblyBandBushing, chrono::vehicle::TrackAssemblyDoublePin, chrono::vehicle::TrackAssemblySinglePin, chrono::vehicle::m113::M113_TrackAssemblyBandBushing, and chrono::vehicle::marder::Marder_TrackAssemblySinglePin.
◆ GetRoadWhelAssemblyLocation()
|
pure virtual |
Get the relative location of the specified suspension subsystem.
The track assembly reference frame is ISO, with origin at the sprocket center.
Implemented in chrono::vehicle::m113::M113_TrackAssemblyBandANCF, chrono::vehicle::m113::M113_TrackAssemblyDoublePin, chrono::vehicle::m113::M113_TrackAssemblySinglePin, chrono::vehicle::TrackAssemblyBandANCF, chrono::vehicle::TrackAssemblyBandBushing, chrono::vehicle::TrackAssemblyDoublePin, chrono::vehicle::TrackAssemblySinglePin, chrono::vehicle::m113::M113_TrackAssemblyBandBushing, and chrono::vehicle::marder::Marder_TrackAssemblySinglePin.
◆ GetRollerLocation()
|
inlinevirtual |
Get the relative location of the specified roller subsystem.
The track assembly reference frame is ISO, with origin at the sprocket center.
Reimplemented in chrono::vehicle::TrackAssemblyBandANCF, chrono::vehicle::TrackAssemblyBandBushing, chrono::vehicle::TrackAssemblyDoublePin, chrono::vehicle::TrackAssemblySinglePin, and chrono::vehicle::marder::Marder_TrackAssemblySinglePin.
◆ GetSprocketLocation()
|
pure virtual |
Get the relative location of the sprocket subsystem.
The track assembly reference frame is ISO, with origin at the sprocket center.
Implemented in chrono::vehicle::m113::M113_TrackAssemblyBandANCF, chrono::vehicle::m113::M113_TrackAssemblyDoublePin, chrono::vehicle::m113::M113_TrackAssemblySinglePin, chrono::vehicle::TrackAssemblyBandANCF, chrono::vehicle::TrackAssemblyBandBushing, chrono::vehicle::TrackAssemblyDoublePin, chrono::vehicle::TrackAssemblySinglePin, chrono::vehicle::m113::M113_TrackAssemblyBandBushing, and chrono::vehicle::marder::Marder_TrackAssemblySinglePin.
◆ GetTrackShoeAngVel()
|
inline |
Get the angular velocity of the specified track shoe.
Return the angular velocity of the shoe body frame, expressed in the global reference frame.
◆ GetTrackShoeLinVel()
|
inline |
Get the linear velocity of the specified track shoe.
Return the linear velocity of the shoe body center, expressed in the global reference frame.
◆ GetTrackShoePos()
|
inline |
Get the global location of the specified track shoe.
The returned location is that of the shoe body in the track shoe subsystem.
◆ GetTrackShoeRot()
|
inline |
Get the orientation of the specified track shoe.
The track shoe body orientation is returned as a quaternion representing a rotation with respect to the global reference frame. This is the orientation of the shoe body in the track shoe subsystem.
◆ GetTrackShoeState()
BodyState chrono::vehicle::ChTrackAssembly::GetTrackShoeState | ( | size_t | id | ) | const |
Get the complete state for the specified track shoe.
This includes the location, orientation, linear and angular velocities, all expressed in the global reference frame.
◆ GetTrackShoeStates()
void chrono::vehicle::ChTrackAssembly::GetTrackShoeStates | ( | BodyStates & | states | ) | const |
Get the complete states for all track shoes.
These include the locations, orientations, linear and angular velocities for all track shoes in this track assembly, all expressed in the global reference frame. It is assumed that the vector of body states was properly sized.
◆ GetTrackShoeTension()
|
inline |
Get track tension at the specified track shoe.
Return is the force due to the connections of this track shoe, expressed in the track shoe reference frame.
◆ Initialize()
void chrono::vehicle::ChTrackAssembly::Initialize | ( | std::shared_ptr< ChChassis > | chassis, |
const ChVector3d & | location, | ||
bool | create_shoes = true |
||
) |
Initialize this track assembly subsystem.
The subsystem is initialized by attaching it to the specified chassis at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the track assembly reference frame is always aligned with the chassis reference frame.
- Parameters
-
[in] chassis chassis subsystem [in] location location relative to the chassis frame [in] create_shoes control creation of the actual track
◆ InitializeInertiaProperties()
|
overrideprotectedvirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ ReportSuspensionForce()
ChTrackSuspension::ForceTorque chrono::vehicle::ChTrackAssembly::ReportSuspensionForce | ( | size_t | id | ) | const |
Return current suspension forces or torques, as appropriate (spring and shock) for the specified suspension.
Different suspension types will load different quantities in the output struct.
◆ SetOutput()
|
overridevirtual |
Enable/disable output for this subsystem.
This function overrides the output setting for all components of this track assembly.
Reimplemented from chrono::vehicle::ChPart.
◆ Synchronize() [1/2]
void chrono::vehicle::ChTrackAssembly::Synchronize | ( | double | time, |
double | braking | ||
) |
Update the state of this track assembly at the current time.
- Parameters
-
[in] time current time [in] braking braking driver input
◆ Synchronize() [2/2]
void chrono::vehicle::ChTrackAssembly::Synchronize | ( | double | time, |
double | braking, | ||
const TerrainForces & | shoe_forces | ||
) |
Update the state of this track assembly at the current time.
This version is called if co-simulating with an external terain system.
- Parameters
-
[in] time current time [in] braking braking driver input [in] shoe_forces vector of tire force structures
◆ UpdateInertiaProperties()
|
overrideprotectedvirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/ChTrackAssembly.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/ChTrackAssembly.cpp