Description
template<class Real = double>
class chrono::ChFrame< Real >
Representation of a 3D transform.
A 'frame' coordinate system has a translation and a rotation respect to a 'parent' coordinate system, usually the absolute (world) coordinates. Differently from a simple ChCoordsys object, the ChFrame also stores the 3x3 rotation matrix implements, which permits some optimizations, especially when a large number of vectors must be transformed by the same frame.
See Coordinate Systems manual page.
#include <ChFrame.h>
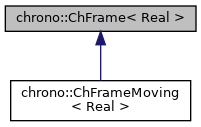
Public Member Functions | |
ChFrame (const ChVector3< Real > &v=ChVector3< Real >(0, 0, 0), const ChQuaternion< Real > &q=ChQuaternion< Real >(1, 0, 0, 0)) | |
Default constructor, or construct from pos and rot (as a quaternion) | |
ChFrame (const ChVector3< Real > &v, const ChMatrix33< Real > &R) | |
Construct from pos and rotation (as a 3x3 matrix) | |
ChFrame (const ChCoordsys< Real > &C) | |
Construct from a coordsys. | |
ChFrame (const ChVector3< Real > &v, const Real angle, const ChVector3< Real > &u) | |
Construct from position mv and rotation of angle alpha around unit vector mu. | |
ChFrame (const ChFrame< Real > &other) | |
Copy constructor, build from another frame. | |
ChFrame< Real > & | operator= (const ChFrame< Real > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrame< Real > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrame< Real > &other) const |
Returns true for different frames. | |
ChFrame< Real > | operator* (const ChFrame< Real > &F) const |
Transform another frame through this frame. More... | |
ChFrame< Real > | operator>> (const ChFrame< Real > &F) const |
Transform another frame through this frame. More... | |
ChVector3< Real > | operator* (const ChVector3< Real > &v) const |
Transform a vector through this frame (express in parent frame). More... | |
ChVector3< Real > | operator/ (const ChVector3< Real > &v) const |
Transform a vector through this frame (express from parent frame). More... | |
ChFrame< Real > & | operator>>= (const ChFrame< Real > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrame< Real > & | operator*= (const ChFrame< Real > &F) |
Transform this frame by post-multiplication with another frame. More... | |
ChFrame< Real > & | operator>>= (const ChVector3< Real > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrame< Real > & | operator>>= (const ChQuaternion< Real > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrame< Real > & | operator>>= (const ChCoordsys< Real > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
const ChCoordsys< Real > & | GetCoordsys () const |
Return both current rotation and translation as a ChCoordsys object. | |
const ChVector3< Real > & | GetPos () const |
Return the current translation vector. | |
const ChQuaternion< Real > & | GetRot () const |
Return the current rotation quaternion. | |
const ChMatrix33< Real > & | GetRotMat () const |
Return the current 3x3 rotation matrix. | |
ChVector3< Real > | GetRotAxis () const |
Get axis of finite rotation, in parent space. | |
Real | GetRotAngle () const |
Get angle of rotation about axis of finite rotation. | |
void | SetCoordsys (const ChCoordsys< Real > &C) |
Impose both translation and rotation as a single ChCoordsys. More... | |
void | SetCoordsys (const ChVector3< Real > &v, const ChQuaternion< Real > &q) |
Impose both translation and rotation. More... | |
void | SetRot (const ChQuaternion< Real > &q) |
Impose the rotation as a quaternion. More... | |
void | SetRot (const ChMatrix33< Real > &R) |
Impose the rotation as a 3x3 matrix. More... | |
void | SetPos (const ChVector3< Real > &pos) |
Impose the translation vector. | |
void | ConcatenatePreTransformation (const ChFrame< Real > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrame< Real > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
void | Move (const ChVector3< Real > &v) |
An easy way to move the frame by the amount specified by vector v, (assuming v expressed in parent coordinates) | |
void | Move (const ChCoordsys< Real > &C) |
Apply both translation and rotation, assuming both expressed in parent coordinates, as a vector for translation and quaternion for rotation,. | |
ChVector3< Real > | TransformPointLocalToParent (const ChVector3< Real > &v) const |
Transform a point from the local frame coordinate system to the parent coordinate system. | |
ChVector3< Real > | TransformPointParentToLocal (const ChVector3< Real > &v) const |
Transforms a point from the parent coordinate system to local frame coordinate system. | |
ChVector3< Real > | TransformDirectionLocalToParent (const ChVector3< Real > &d) const |
Transform a direction from the parent frame coordinate system to 'this' local coordinate system. | |
ChVector3< Real > | TransformDirectionParentToLocal (const ChVector3< Real > &d) const |
Transforms a direction from 'this' local coordinate system to parent frame coordinate system. | |
ChWrench< Real > | TransformWrenchLocalToParent (const ChWrench< Real > &w) const |
Transform a wrench from the local coordinate system to the parent coordinate system. | |
ChWrench< Real > | TransformWrenchParentToLocal (const ChWrench< Real > &w) const |
Transform a wrench from the parent coordinate system to the local coordinate system. | |
ChFrame< Real > | TransformLocalToParent (const ChFrame< Real > &F) const |
Transform a frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrame< Real > | TransformParentToLocal (const ChFrame< Real > &F) const |
Transform a frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrame< Real > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrame< Real > &other, Real tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
void | Normalize () |
Normalize the rotation, so that quaternion has unit length. | |
virtual void | SetIdentity () |
Sets to no translation and no rotation. | |
virtual void | Invert () |
Invert in place. More... | |
ChFrame< Real > | GetInverse () const |
Return the inverse transform. | |
virtual void | ArchiveOut (ChArchiveOut &archive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive) |
Method to allow de-serialization of transient data from archives. | |
Protected Attributes | |
ChCoordsys< Real > | m_csys |
position and rotation, as vector + quaternion | |
ChMatrix33< Real > | m_rmat |
3x3 orthogonal rotation matrix | |
Friends | |
class | FmuChronoComponentBase |
Member Function Documentation
◆ ConcatenatePostTransformation()
|
inline |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate.
This is equivalent to post-multiply this frame by the other frame F: this'= this * F or this'= F >> this
◆ ConcatenatePreTransformation()
|
inline |
Apply a transformation (rotation and translation) represented by another frame.
This is equivalent to pre-multiply this frame by the other frame F: this'= F * this or this' = this >> F
◆ Invert()
|
inlinevirtual |
Invert in place.
If w = A * v, after A.Invert() we have v = A * w;
Reimplemented in chrono::ChFrameMoving< Real >, and chrono::ChFrameMoving< double >.
◆ operator*() [1/2]
|
inline |
Transform another frame through this frame.
If A is this frame and F another frame expressed in A, then G = A * F is the frame F expresssed in the parent frame of A. For a sequence of transformations, i.e. a chain of coordinate systems, one can also write: G = F_1to0 * F_2to1 * F_3to2 * F; i.e., just like done with a sequence of Denavitt-Hartemberg matrix multiplications. This operation is not commutative.
◆ operator*() [2/2]
|
inline |
Transform a vector through this frame (express in parent frame).
If A is this frame and v a vector expressed in this frame, w = A * v is the vector expressed in the parent frame of A. For a sequence of transformations, i.e. a chain of coordinate systems, one can also write: w = F_1to0 * F_2to1 * F_3to2 * v; i.e., just like done with a sequence of Denavitt-Hartemberg matrix multiplications. This operation is not commutative. NOTE: since c++ operator execution is from left to right, in case of multiple transformations w = v >> C >> B >> A may be faster than w = A * B * C * v
◆ operator*=()
|
inline |
Transform this frame by post-multiplication with another frame.
If A is this frame, then A *= F means A' = A * F or A' = F >> A.
◆ operator/()
|
inline |
Transform a vector through this frame (express from parent frame).
If A is this frame and v a vector expressed in the parent frame of A, then w = A / v is the vector expressed in A. In other words, w = A * v implies v = A/w.
◆ operator>>()
|
inline |
Transform another frame through this frame.
If A is this frame and F another frame expressed in A, then G = F >> A is the frame F expresssed in the parent frame of A. For a sequence of transformations, i.e. a chain of coordinate systems, one can also write: G = F >> F_3to2 >> F_2to1 >> F_1to0; i.e., just like done with a sequence of Denavitt-Hartemberg matrix multiplications (but reverting order). This operation is not commutative.
◆ operator>>=()
|
inline |
Transform this frame by pre-multiplication with another frame.
If A is this frame, then A >>= F means A' = F * A or A' = A >> F.
◆ SetCoordsys() [1/2]
|
inline |
Impose both translation and rotation as a single ChCoordsys.
Note: the quaternion part must be already normalized.
◆ SetCoordsys() [2/2]
|
inline |
Impose both translation and rotation.
Note: the quaternion part must be already normalized.
◆ SetRot() [1/2]
|
inline |
Impose the rotation as a 3x3 matrix.
Note: the rotation matrix must be already orthogonal.
◆ SetRot() [2/2]
|
inline |
Impose the rotation as a quaternion.
Note: the quaternion must be already normalized.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono/core/ChFrame.h