Public Member Functions |
Protected Member Functions |
Protected Attributes |
Static Protected Attributes |
List of all members
chrono::vehicle::man::MAN_10t_Chassis Class Reference
Description
MAN 10t chassis subsystem.
#include <MAN_10t_Chassis.h>
Inheritance diagram for chrono::vehicle::man::MAN_10t_Chassis:
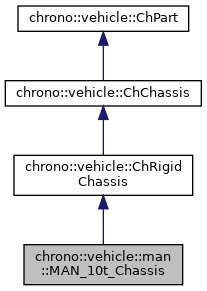
Collaboration diagram for chrono::vehicle::man::MAN_10t_Chassis:

Public Member Functions | |
MAN_10t_Chassis (const std::string &name, bool fixed=false, CollisionType chassis_collision_type=CollisionType::NONE) | |
virtual ChCoordsys | GetLocalDriverCoordsys () const override |
Get the local driver position and orientation. More... | |
![]() | |
ChRigidChassis (const std::string &name, bool fixed=false) | |
Construct a vehicle subsystem with the specified name. More... | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
bool | HasCollision () const |
Specifies whether or not collision shapes were defined. | |
bool | HasPrimitives () const |
Specifies whether or not visualization primitives were defined. | |
bool | HasMesh () const |
Specifies whether or not a visualization mesh was defined. | |
const std::string & | GetMeshFilename () const |
Get the name of the Wavefront file with chassis visualization mesh. More... | |
virtual void | Construct (ChVehicle *vehicle, const ChCoordsys<> &chassisPos, double chassisFwdVel, int collision_family) override |
Construct the rigid chassis at the specified global position and orientation. More... | |
virtual void | EnableCollision (bool state) override |
Enable/disable contact for the chassis. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () override final |
Remove all visualization assets from this subsystem. | |
![]() | |
virtual uint16_t | GetVehicleTag () const override |
Get the tag of the associated vehicle. More... | |
virtual const ChVector3d | GetLocalPosRearConnector () const |
Get the location (in the local frame of this chassis) of the connection to a rear chassis. | |
std::shared_ptr< ChBodyAuxRef > | GetBody () const |
Get a handle to the vehicle's chassis body. | |
ChSystem * | GetSystem () const |
Get a pointer to the containing system. | |
const ChVector3d & | GetPos () const |
Get the global location of the chassis reference frame origin. | |
ChQuaternion | GetRot () const |
Get the orientation of the chassis reference frame. More... | |
ChVector3d | GetDriverPos () const |
Get the global location of the driver. | |
double | GetSpeed () const |
Get the vehicle speed. More... | |
double | GetCOMSpeed () const |
Get the speed of the chassis COM. More... | |
double | GetRollRate () const |
Get the roll rate of the chassis. More... | |
double | GetPitchRate () const |
Get the pitch rate of the chassis. More... | |
double | GetYawRate () const |
Get the yaw rate of the chassis. More... | |
double | GetTurnRate () const |
Get the turn rate of the chassis. More... | |
ChVector3d | GetPointLocation (const ChVector3d &locpos) const |
Get the global position of the specified point. More... | |
ChVector3d | GetPointVelocity (const ChVector3d &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector3d | GetPointAcceleration (const ChVector3d &locpos) const |
Get the acceleration at the specified point. More... | |
void | Initialize (ChVehicle *vehicle, const ChCoordsys<> &chassisPos, double chassisFwdVel, int collision_family=0) |
Initialize the chassis at the specified global position and orientation. More... | |
void | SetFixed (bool val) |
Set the "fixed to ground" status of the chassis body. | |
bool | IsFixed () const |
Return true if the chassis body is fixed to ground. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
void | AddMarker (const std::string &name, const ChFrame<> &frame) |
Add a marker on the chassis body at the specified position (relative to the chassis reference frame). More... | |
const std::vector< std::shared_ptr< ChMarker > > & | GetMarkers () const |
void | SetAerodynamicDrag (double Cd, double area, double air_density) |
Set parameters and enable aerodynamic drag force calculation. More... | |
virtual void | Synchronize (double time) |
Update the state of the chassis subsystem. More... | |
void | AddJoint (std::shared_ptr< ChVehicleJoint > joint) |
Utility function to add a joint (kinematic or bushing) to the vehicle system. | |
void | AddExternalForceTorque (std::shared_ptr< ExternalForceTorque > load) |
Utility force to add an external load to the chassis body. | |
void | AddTerrainLoad (std::shared_ptr< ChLoadBase > terrain_load) |
Add a terrain load to the vehicle system. More... | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Member Functions | |
virtual double | GetBodyMass () const override |
virtual ChMatrix33 | GetBodyInertia () const override |
virtual ChFrame | GetBodyCOMFrame () const override |
![]() | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const override |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const override |
Output data for this subsystem's component list to the specified database. | |
![]() | |
ChChassis (const std::string &name, bool fixed=false) | |
Construct a chassis subsystem with the specified name. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
ChMatrix33 | m_body_inertia |
![]() | |
utils::ChBodyGeometry | m_geometry |
collection of visualization and collision shapes | |
![]() | |
ChVehicle * | m_vehicle |
associated vehicle | |
std::shared_ptr< ChBodyAuxRef > | m_body |
handle to the chassis body | |
std::shared_ptr< ChLoadContainer > | m_container_bushings |
load container for vehicle bushings | |
std::shared_ptr< ChLoadContainer > | m_container_external |
load container for external forces | |
std::shared_ptr< ChLoadContainer > | m_container_terrain |
load container for terrain forces | |
std::vector< std::shared_ptr< ExternalForceTorque > > | m_external_loads |
external loads | |
std::vector< std::shared_ptr< ChMarker > > | m_markers |
list of user-defined markers | |
bool | m_fixed |
is the chassis body fixed to ground? | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Static Protected Attributes | |
static const double | m_body_mass = 7085 + 1200 + 2500 |
static const ChVector3d | m_body_inertiaXX |
static const ChVector3d | m_body_inertiaXY |
static const ChVector3d | m_body_COM_loc |
static const ChCoordsys | m_driverCsys |
Additional Inherited Members | |
![]() | |
static void | RemoveJoint (std::shared_ptr< ChVehicleJoint > joint) |
Utility function to remove a joint (kinematic or bushing) from the vehicle system. | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Function Documentation
◆ GetLocalDriverCoordsys()
|
inlineoverridevirtual |
Get the local driver position and orientation.
This is a coordinate system relative to the chassis reference frame.
Implements chrono::vehicle::ChChassis.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/man/MAN_10t_Chassis.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/man/MAN_10t_Chassis.cpp