Description
Base class for a suspension test rig.
#include <ChSuspensionTestRig.h>
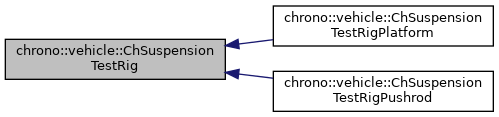
Public Member Functions | |
virtual | ~ChSuspensionTestRig () |
Destructor. | |
void | SetDriver (std::shared_ptr< ChSuspensionTestRigDriver > driver) |
Set driver system. | |
std::shared_ptr< ChSuspensionTestRigDriver > | GetDriver () const |
Get the attached driver (if any). | |
void | SetInitialRideHeight (double height) |
Set the initial ride height (relative to the chassis reference frame). More... | |
void | SetDisplacementLimit (double limit) |
Set the limits for post displacement (same for jounce and rebound). | |
void | IncludeSteeringMechanism (int index) |
Include the specified steering mechanism in testing. | |
void | IncludeSubchassis (int index) |
Include the specified subchassis in testing. | |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystem (default: PRIMITIVES). | |
void | SetSteeringVisualizationType (VisualizationType vis) |
Set visualization type for the steering subsystems (default: PRIMITIVES). | |
void | SetSubchassisVisualizationType (VisualizationType vis) |
Set visualization type for the subchassis subsystems (default: PRIMITIVES). | |
void | SetWheelVisualizationType (VisualizationType vis) |
Set visualization type for the wheel subsystems (default: NONE). | |
void | SetTireVisualizationType (VisualizationType vis) |
Set visualization type for the tire subsystems (default: PRIMITIVES). | |
void | Initialize () |
Initialize the suspension test rig. More... | |
void | Advance (double step) |
Advance the state of the suspension test rig by the specified time step. | |
ChWheeledVehicle & | GetVehicle () const |
Get a handle to the underlying vehicle system. | |
const ChVector3d & | GetSpindlePos (int axle, VehicleSide side) const |
Get the global location of the specified spindle. More... | |
ChQuaternion | GetSpindleRot (int axle, VehicleSide side) const |
Get the global rotation of the specified spindle. More... | |
const ChVector3d & | GetSpindleLinVel (int axle, VehicleSide side) const |
Get the linear velocity of the specified spindle (expressed in the global reference frame). More... | |
ChVector3d | GetSpindleAngVel (int axle, VehicleSide side) const |
Get the angular velocity of the specified spindle (expressed in the global reference frame). More... | |
double | GetSteeringInput () const |
Return current driver steering input. | |
virtual double | GetActuatorDisp (int axle, VehicleSide side)=0 |
Return the current post displacement for the specified axle and vehicle side. | |
virtual double | GetActuatorForce (int axle, VehicleSide side)=0 |
Return the current post actuation force for the specified axle and vehicle side. | |
double | GetWheelTravel (int axle, VehicleSide side) const |
Get wheel travel, defined here as the vertical displacement of the spindle center from its position at the initial specified ride height (or initial configuration, if an initial ride height is not specified). More... | |
virtual double | GetRideHeight (int axle) const =0 |
Get current ride height for the specified axle (relative to the chassis reference frame). More... | |
bool | DriverEnded () const |
Return true when driver stopped producing inputs. | |
void | LogConstraintViolations () |
Log current constraint violations. | |
void | SetOutput (ChVehicleOutput::Type type, const std::string &out_dir, const std::string &out_name, double output_step) |
Enable output for this vehicle system (default: false). More... | |
void | SetPlotOutput (double output_step) |
Enable data collection for plot generation (default: false). More... | |
void | PlotOutput (const std::string &out_dir, const std::string &out_name) |
Plot collected data. More... | |
Protected Member Functions | |
ChSuspensionTestRig (std::shared_ptr< ChWheeledVehicle > vehicle, std::vector< int > axle_index, double displ_limit) | |
Construct a test rig for specified sets of axles and sterring mechanisms of a given vehicle. More... | |
ChSuspensionTestRig (const std::string &spec_filename) | |
Construct a test rig from the given JSON specification file. More... | |
virtual void | InitializeRig ()=0 |
Create and initialize the bodies, joints, and actuators of the rig mechanism. | |
virtual double | CalcDisplacementOffset (int axle)=0 |
Calculate required actuator displacement offset to impose specified ride height. More... | |
virtual double | CalcTerrainHeight (int axle, VehicleSide side)=0 |
Calculate current terrain height under the specified post. More... | |
virtual void | UpdateActuators (std::vector< double > displ_left, std::vector< double > displ_speed_left, std::vector< double > displ_right, std::vector< double > displ_speed_right)=0 |
Update actuators at current time. | |
void | CollectPlotData (double time) |
Collect data for plot output. | |
Protected Attributes | |
std::shared_ptr< ChWheeledVehicle > | m_vehicle |
associated vehicle | |
int | m_naxles |
number of tested axles | |
std::vector< int > | m_axle_index |
list of tested vehicle axles in rig | |
std::vector< int > | m_steering_index |
list of tested vehicle steering mechanisms in rig | |
std::vector< int > | m_subchassis_index |
list of tested vehicle subchassis mechanisms in rig | |
double | m_ride_height |
ride height | |
double | m_displ_limit |
scale factor for post displacement | |
std::vector< double > | m_displ_offset |
post displacement offsets (to set reference positions) | |
Constructor & Destructor Documentation
◆ ChSuspensionTestRig() [1/2]
|
protected |
Construct a test rig for specified sets of axles and sterring mechanisms of a given vehicle.
- Parameters
-
vehicle test vehicle axle_index list of tested vehicle axles displ_limit displacement limits
◆ ChSuspensionTestRig() [2/2]
|
protected |
Construct a test rig from the given JSON specification file.
An STR specification file includes the JSON specification for the underlying vehicle, the sets of tested vehicle subsystems, and the displacement limit for the rig posts.
Member Function Documentation
◆ CalcDisplacementOffset()
|
protectedpure virtual |
Calculate required actuator displacement offset to impose specified ride height.
Note: 'axle' is the index in the set of tested axles.
◆ CalcTerrainHeight()
|
protectedpure virtual |
Calculate current terrain height under the specified post.
Note: 'axle' is the index in the set of tested axles.
◆ GetRideHeight()
|
pure virtual |
Get current ride height for the specified axle (relative to the chassis reference frame).
This estimate uses the average of the left and right posts. Note: 'axle' is the index in the set of tested axles.
Implemented in chrono::vehicle::ChSuspensionTestRigPushrod, and chrono::vehicle::ChSuspensionTestRigPlatform.
◆ GetSpindleAngVel()
ChVector3d chrono::vehicle::ChSuspensionTestRig::GetSpindleAngVel | ( | int | axle, |
VehicleSide | side | ||
) | const |
Get the angular velocity of the specified spindle (expressed in the global reference frame).
Note: 'axle' is the index in the set of tested axles.
◆ GetSpindleLinVel()
const ChVector3d & chrono::vehicle::ChSuspensionTestRig::GetSpindleLinVel | ( | int | axle, |
VehicleSide | side | ||
) | const |
Get the linear velocity of the specified spindle (expressed in the global reference frame).
Note: 'axle' is the index in the set of tested axles.
◆ GetSpindlePos()
const ChVector3d & chrono::vehicle::ChSuspensionTestRig::GetSpindlePos | ( | int | axle, |
VehicleSide | side | ||
) | const |
Get the global location of the specified spindle.
Note: 'axle' is the index in the set of tested axles.
◆ GetSpindleRot()
ChQuaternion chrono::vehicle::ChSuspensionTestRig::GetSpindleRot | ( | int | axle, |
VehicleSide | side | ||
) | const |
Get the global rotation of the specified spindle.
Note: 'axle' is the index in the set of tested axles.
◆ GetWheelTravel()
double chrono::vehicle::ChSuspensionTestRig::GetWheelTravel | ( | int | axle, |
VehicleSide | side | ||
) | const |
Get wheel travel, defined here as the vertical displacement of the spindle center from its position at the initial specified ride height (or initial configuration, if an initial ride height is not specified).
Note: 'axle' is the index in the set of tested axles.
◆ Initialize()
void chrono::vehicle::ChSuspensionTestRig::Initialize | ( | ) |
Initialize the suspension test rig.
Initialize and prepare the associated vehicle and rig driver, then let derived classes construct the bodies, joints, and actuators of the rig mechanism.
◆ PlotOutput()
void chrono::vehicle::ChSuspensionTestRig::PlotOutput | ( | const std::string & | out_dir, |
const std::string & | out_name | ||
) |
Plot collected data.
When called (typically at the end of the simulation loop), the collected data is saved in ASCII format in a file with the specified name (and 'txt' extension) in the specified output directory. If the Chrono::Postprocess module is available (with gnuplot support), selected plots are generated.
◆ SetInitialRideHeight()
|
inline |
Set the initial ride height (relative to the chassis reference frame).
If not specified, the reference height is the suspension design configuration.
◆ SetOutput()
void chrono::vehicle::ChSuspensionTestRig::SetOutput | ( | ChVehicleOutput::Type | type, |
const std::string & | out_dir, | ||
const std::string & | out_name, | ||
double | output_step | ||
) |
Enable output for this vehicle system (default: false).
- Parameters
-
type type of output DB out_dir output directory name out_name rootname of output file output_step interval between output times
◆ SetPlotOutput()
void chrono::vehicle::ChSuspensionTestRig::SetPlotOutput | ( | double | output_step | ) |
Enable data collection for plot generation (default: false).
Collected data includes:
- [col 1] time (s)
- [col 2-5] left input, left spindle z (m), left spindle z velocity (m/s), left wheel travel (m)
- [col 6-7] left spring force (N), left shock force (N)
- [col 8-11] right input, right spindle z (m), right spindle z velocity (m/s), right wheel travel (m)
- [col 12-13] right spring force (N), right shock force (N)
- [col 14] estimated ride height (m)
- [col 15] left camber angle, gamma (deg)
- [col 16] right camber angle, gamma (deg)
- [col 17-19] application point for left tire force (m)
- [col 20-22] left tire force (N)
- [col 23-25] left tire moment (Nm)
- [col 26-28] application point for right tire force (m)
- [col 29-31] right tire force (N)
- [col 32-34] right tire moment (Nm) Tire forces are expressed in the global frame, as applied to the center of the associated wheel.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRig.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRig.cpp