Description
Class defining the geometric model for collision detection.
A ChCollisionModel contains all geometric shapes on a rigid body, for collision purposes.
#include <ChCollisionModel.h>

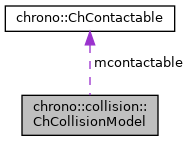
Public Member Functions | |
virtual ChCollisionSystemType | GetType () const =0 |
Return the type of this collision model. | |
virtual int | ClearModel ()=0 |
Delete all inserted geometries. More... | |
virtual int | BuildModel ()=0 |
Complete the construction of the collision model. More... | |
virtual bool | AddSphere (std::shared_ptr< ChMaterialSurface > material, double radius, const ChVector<> &pos=ChVector<>())=0 |
Add a sphere shape to this collision model. More... | |
virtual bool | AddEllipsoid (std::shared_ptr< ChMaterialSurface > material, double axis_x, double axis_y, double axis_z, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add an ellipsoid shape to this collision model. More... | |
virtual bool | AddBox (std::shared_ptr< ChMaterialSurface > material, double size_x, double size_y, double size_z, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a box shape to this collision model. More... | |
virtual bool | AddCylinder (std::shared_ptr< ChMaterialSurface > material, double radius, double height, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a cylinder to this collision model (axis in Z direction). More... | |
bool | AddCylinder (std::shared_ptr< ChMaterialSurface > material, double radius, const ChVector<> &p1, const ChVector<> &p2) |
Add a cylinder specified through a radius and end points. More... | |
virtual bool | AddCylindricalShell (std::shared_ptr< ChMaterialSurface > material, double radius, double height, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a cylindrical shell to this collision model (axis in Z direction). More... | |
virtual bool | AddCone (std::shared_ptr< ChMaterialSurface > material, double radius, double height, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a cone to this collision model (axis in Z direction). More... | |
virtual bool | AddCapsule (std::shared_ptr< ChMaterialSurface > material, double radius, double height, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a capsule to this collision model (axis in Z direction). More... | |
virtual bool | AddRoundedBox (std::shared_ptr< ChMaterialSurface > material, double size_x, double size_y, double size_z, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a rounded box shape to this collision model. More... | |
virtual bool | AddRoundedCylinder (std::shared_ptr< ChMaterialSurface > material, double radius, double height, double sphere_r, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a rounded cylinder to this collision model (axis in Z direction). More... | |
virtual bool | AddConvexHull (std::shared_ptr< ChMaterialSurface > material, const std::vector< ChVector< double >> &pointlist, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a convex hull to this collision model. More... | |
virtual bool | AddTriangleMesh (std::shared_ptr< ChMaterialSurface > material, std::shared_ptr< geometry::ChTriangleMesh > trimesh, bool is_static, bool is_convex, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1), double sphereswept_thickness=0.0)=0 |
Add a triangle mesh to this collision model. More... | |
virtual bool | AddBarrel (std::shared_ptr< ChMaterialSurface > material, double Y_low, double Y_high, double axis_vert, double axis_hor, double R_offset, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1))=0 |
Add a barrel-like shape to this collision model (main axis on Y direction). More... | |
virtual bool | Add2Dpath (std::shared_ptr< ChMaterialSurface > material, std::shared_ptr< geometry::ChLinePath > mpath, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1), const double thickness=0.001) |
Add a 2D closed line, defined on the XY plane passing by pos and aligned as rot, that defines a 2D collision shape that will collide with another 2D line of the same type if aligned on the same plane. More... | |
virtual bool | AddPoint (std::shared_ptr< ChMaterialSurface > material, double radius=0, const ChVector<> &pos=ChVector<>()) |
Add a point-like sphere, that will collide with other geometries, but won't ever create contacts between them. More... | |
virtual bool | AddCopyOfAnotherModel (ChCollisionModel *another)=0 |
Add all shapes already contained in another model. More... | |
virtual bool | AddConvexHullsFromFile (std::shared_ptr< ChMaterialSurface > material, ChStreamInAscii &mstream, const ChVector<> &pos=ChVector<>(), const ChMatrix33<> &rot=ChMatrix33<>(1)) |
Add a cluster of convex hulls specified in a '.chulls' file description. More... | |
ChContactable * | GetContactable () |
Get the pointer to the contactable object. | |
virtual void | SetContactable (ChContactable *mc) |
Set the pointer to the contactable object. More... | |
virtual ChPhysicsItem * | GetPhysicsItem () |
Get the pointer to the client owner ChPhysicsItem. More... | |
virtual void | SyncPosition ()=0 |
Synchronize the position and orientation of the collision model to the associated contactable. | |
virtual void | SetFamily (int mfamily) |
By default, all collision objects belong to family n.0, but you can set family in range 0..15. More... | |
virtual int | GetFamily () |
virtual void | SetFamilyMaskNoCollisionWithFamily (int mfamily) |
By default, family mask is all turned on, so all families can collide with this object, but you can turn on-off some bytes of this mask so that some families do not collide. More... | |
virtual void | SetFamilyMaskDoCollisionWithFamily (int mfamily) |
virtual bool | GetFamilyMaskDoesCollisionWithFamily (int mfamily) |
Tells if the family mask of this collision object allows for the collision with another collision object belonging to a given family. More... | |
virtual short int | GetFamilyGroup () const |
Return the collision family group of this model. More... | |
virtual void | SetFamilyGroup (short int group) |
Set the collision family group of this model. More... | |
virtual short int | GetFamilyMask () const |
Return the collision mask for this model. More... | |
virtual void | SetFamilyMask (short int mask) |
Set the collision mask for this model. More... | |
virtual void | SetSafeMargin (double amargin) |
Set the suggested collision 'inward safe margin' for the shapes to be added from now on. More... | |
virtual float | GetSafeMargin () |
Returns the inward safe margin (see SetSafeMargin() ) | |
virtual void | SetEnvelope (double amargin) |
Set the suggested collision outward 'envelope' used from shapes added from now on. More... | |
virtual float | GetEnvelope () |
Return the outward safe margin (see SetEnvelope() ) | |
virtual void | GetAABB (ChVector<> &bbmin, ChVector<> &bbmax) const =0 |
Return the axis aligned bounding box (AABB) of the collision model, i.e. More... | |
virtual void | ArchiveOut (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
int | GetNumShapes () const |
Return the number of collision shapes in this model. | |
const std::vector< std::shared_ptr< ChCollisionShape > > & | GetShapes () const |
Get the list of collision shapes in this model. | |
std::shared_ptr< ChCollisionShape > | GetShape (int index) |
Get the collision shape with specified index. | |
virtual ChCoordsys | GetShapePos (int index) const =0 |
Return the position and orientation of the collision shape with specified index, relative to the model frame. | |
virtual std::vector< double > | GetShapeDimensions (int index) const =0 |
Return shape characteristic dimensions. More... | |
void | SetShapeMaterial (int index, std::shared_ptr< ChMaterialSurface > mat) |
Set the contact material for the collision shape with specified index. | |
void | SetAllShapesMaterial (std::shared_ptr< ChMaterialSurface > mat) |
Set the contact material for all collision shapes in the model (all shapes will share the material). More... | |
Static Public Member Functions | |
static void | SetDefaultSuggestedEnvelope (double menv) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision envelope (safe outward layer) as default. More... | |
static void | SetDefaultSuggestedMargin (double mmargin) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision margin (inward penetration layer) as default. More... | |
static double | GetDefaultSuggestedEnvelope () |
static double | GetDefaultSuggestedMargin () |
Protected Member Functions | |
void | CopyShapes (ChCollisionModel *other) |
Copy the collision shapes from another model. | |
virtual float | GetSuggestedFullMargin () |
Protected Attributes | |
float | model_envelope |
Maximum envelope: surrounding volume from surface to the exterior. | |
float | model_safe_margin |
Maximum margin value to be used for fast penetration contact detection. | |
ChContactable * | mcontactable |
Pointer to the contactable object. | |
short int | family_group |
Collision family group. | |
short int | family_mask |
Collision family mask. | |
std::vector< std::shared_ptr< ChCollisionShape > > | m_shapes |
list of collision shapes in model | |
Member Function Documentation
◆ Add2Dpath()
|
inlinevirtual |
Add a 2D closed line, defined on the XY plane passing by pos and aligned as rot, that defines a 2D collision shape that will collide with another 2D line of the same type if aligned on the same plane.
This is useful for mechanisms that work on a plane, and that require more precise collision that is not possible with current 3D shapes. For example, the line can contain concave or convex round fillets. Requirements:
- the line must be clockwise for inner material, (counterclockwise=hollow, material outside)
- the line must contain only ChLineSegment and ChLineArc sub-lines
- the sublines must follow in the proper order, with coincident corners, and must be closed.
- Parameters
-
material surface contact material mpath 2D curve path pos origin position in model coordinates rot rotation in model coordinates thickness line thickness
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ AddBarrel()
|
pure virtual |
Add a barrel-like shape to this collision model (main axis on Y direction).
The barrel shape is made by lathing an arc of an ellipse around the vertical Y axis. The center of the ellipse is on Y=0 level, and it is offsetted by R_offset from the Y axis in radial direction. The two axes of the ellipse are axis_vert (for the vertical direction, i.e. the axis parallel to Y) and axis_hor (for the axis that is perpendicular to Y). Also, the solid is clamped with two discs on the top and the bottom, at levels Y_low and Y_high.
- Parameters
-
material surface contact material Y_low bottom level Y_high top level axis_vert ellipse axis in vertical direction axis_hor ellipse axis in horizontal direction R_offset lateral offset (radius at top and bottom) pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ AddBox()
|
pure virtual |
Add a box shape to this collision model.
- Parameters
-
material surface contact material size_x x dimension size_y y dimension size_z z dimension pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelDistributed.
◆ AddCapsule()
|
pure virtual |
Add a capsule to this collision model (axis in Z direction).
- Parameters
-
material surface contact material radius radius height height of cylindrical portion pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelBullet.
◆ AddCone()
|
pure virtual |
Add a cone to this collision model (axis in Z direction).
- Parameters
-
material surface contact material radius radius height height pos base center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelBullet.
◆ AddConvexHull()
|
pure virtual |
Add a convex hull to this collision model.
A convex hull is simply a point cloud that describe a convex polytope. Connectivity between the vertexes, as faces/edges in triangle meshes is not necessary. Points are passed as a list which is then copied into the model.
- Parameters
-
material surface contact material pointlist list of hull points pos origin position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ AddConvexHullsFromFile()
|
virtual |
Add a cluster of convex hulls specified in a '.chulls' file description.
The file is an ascii text that contains lines with "[x] [y] [z]" coordinates of the convex hulls. Hulls are separated by lines with "hull". Inherited classes should not need to implement/overload this, because this base implementation basically calls AddConvexHull() n times while parsing the file, that is enough.
- Parameters
-
material surface contact material mstream input data file pos origin position in model coordinates rot rotation in model coordinates
◆ AddCopyOfAnotherModel()
|
pure virtual |
Add all shapes already contained in another model.
If possible, derived classes should implement this so that underlying shapes are shared (not copied) among the models.
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ AddCylinder() [1/2]
bool chrono::collision::ChCollisionModel::AddCylinder | ( | std::shared_ptr< ChMaterialSurface > | material, |
double | radius, | ||
const ChVector<> & | p1, | ||
const ChVector<> & | p2 | ||
) |
Add a cylinder specified through a radius and end points.
- Parameters
-
material surface contact material radius radius p1 first end point p2 second end point
◆ AddCylinder() [2/2]
|
pure virtual |
Add a cylinder to this collision model (axis in Z direction).
- Parameters
-
material surface contact material radius radius height height pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelBullet.
◆ AddCylindricalShell()
|
pure virtual |
Add a cylindrical shell to this collision model (axis in Z direction).
- Parameters
-
material surface contact material radius radius height height pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelBullet.
◆ AddEllipsoid()
|
pure virtual |
Add an ellipsoid shape to this collision model.
- Parameters
-
material surface contact material axis_x x axis length axis_y y axis length axis_z z axis length pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ AddPoint()
|
inlinevirtual |
Add a point-like sphere, that will collide with other geometries, but won't ever create contacts between them.
- Parameters
-
material surface contact material radius node radius pos center position in model coordinates
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ AddRoundedBox()
|
pure virtual |
Add a rounded box shape to this collision model.
- Parameters
-
material surface contact material size_x x dimension size_y y dimension size_z z dimension sphere_r radius of sweeping sphere pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ AddRoundedCylinder()
|
pure virtual |
Add a rounded cylinder to this collision model (axis in Z direction).
- Parameters
-
material surface contact material radius radius height height sphere_r radius of sweeping sphere pos center position in model coordinates rot rotation in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ AddSphere()
|
pure virtual |
Add a sphere shape to this collision model.
- Parameters
-
material surface contact material radius sphere radius pos center position in model coordinates
Implemented in chrono::collision::ChCollisionModelBullet, chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelDistributed.
◆ AddTriangleMesh()
|
pure virtual |
Add a triangle mesh to this collision model.
Note: if possible, for better performance, avoid triangle meshes and prefer simplified representations as compounds of primitive convex shapes (boxes, sphers, etc).
- Parameters
-
material surface contact material trimesh the triangle mesh is_static true if model doesn't move. May improve performance. is_convex if true, a convex hull is used. May improve robustness. pos origin position in model coordinates rot rotation in model coordinates sphereswept_thickness outward sphere-swept layer (when supported)
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ BuildModel()
|
pure virtual |
Complete the construction of the collision model.
Addition of collision shapes must be done between calls to ClearModel() and BuildModel(). This function must be invoked after all geometric collision shapes have been added.
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ ClearModel()
|
pure virtual |
Delete all inserted geometries.
Addition of collision shapes must be done between calls to ClearModel() and BuildModel(). This function must be invoked before adding geometric collision shapes.
Implemented in chrono::collision::ChCollisionModelBullet, chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelDistributed.
◆ GetAABB()
|
pure virtual |
Return the axis aligned bounding box (AABB) of the collision model, i.e.
max-min along the x,y,z world axes. Remember that SyncPosition() should be invoked before calling this.
Implemented in chrono::collision::ChCollisionModelBullet, chrono::collision::ChCollisionModelChrono, and chrono::collision::ChCollisionModelDistributed.
◆ GetFamilyGroup()
|
inlinevirtual |
Return the collision family group of this model.
The collision family of this model is the position of the single set bit in the return value.
◆ GetFamilyMask()
|
inlinevirtual |
Return the collision mask for this model.
Each bit of the return value indicates whether this model collides with the corresponding family (bit set) or not (bit unset).
◆ GetFamilyMaskDoesCollisionWithFamily()
|
virtual |
Tells if the family mask of this collision object allows for the collision with another collision object belonging to a given family.
This default implementation uses the family mask.
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ GetPhysicsItem()
|
virtual |
Get the pointer to the client owner ChPhysicsItem.
Default: just casts GetContactable(). Just for backward compatibility. It might return null if contactable not inherited by ChPhysicsItem. TODO remove the need of ChPhysicsItem*, just use ChContactable* in all code
◆ GetShapeDimensions()
|
pure virtual |
Return shape characteristic dimensions.
A derived class should implement this function for as many of the following shapes as possible.
SPHERE radius BOX x-halfdim y-halfdim z-halfdim ELLIPSOID x-radius y-radius z-radius CYLINDER x-radius z-radius halflength CONE x-radius z-radius halfheight CAPSULE radius halflength ROUNDEDBOX x-halfdim y-halfdim z-halfdim sphere_rad ROUNDEDCYL x-radius z-radius halflength sphere_rad
Implemented in chrono::collision::ChCollisionModelBullet, and chrono::collision::ChCollisionModelChrono.
◆ SetAllShapesMaterial()
void chrono::collision::ChCollisionModel::SetAllShapesMaterial | ( | std::shared_ptr< ChMaterialSurface > | mat | ) |
Set the contact material for all collision shapes in the model (all shapes will share the material).
This function is useful in adjusting contact material properties for objects imported from outside (e.g., from SolidWorks).
◆ SetContactable()
|
inlinevirtual |
Set the pointer to the contactable object.
A derived class may override this, but should always invoke this base class implementation.
Reimplemented in chrono::collision::ChCollisionModelChrono.
◆ SetDefaultSuggestedEnvelope()
|
static |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision envelope (safe outward layer) as default.
Easier than calling SetEnvelope() all the times.
◆ SetDefaultSuggestedMargin()
|
static |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision margin (inward penetration layer) as default.
If you call it again later, it will have no effect, except for shapes created later. Easier than calling SetMargin() all the times.
◆ SetEnvelope()
|
inlinevirtual |
Set the suggested collision outward 'envelope' used from shapes added from now on.
This 'envelope' is a surrounding invisible volume which extends outward from the surface, and it is used to detect contacts a bit before shapes come into contact, i.e. when dist>0. However contact points will stay on the true surface of the geometry, not on the external surface of the envelope. Call this BEFORE adding the shapes into the model. Side effect: AABB are 'expanded' outward by this amount, so if you exaggerate with this value, CD might be slower and too sensible. On the other hand, if you set this value to 0, contacts are detected only for dist<=0, thus causing unstable simulation.
◆ SetFamily()
|
virtual |
By default, all collision objects belong to family n.0, but you can set family in range 0..15.
This is used when the objects collided with another: the contact is created only if the family is within the 'family mask' of the other, and viceversa. These default implementations use the family group.
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ SetFamilyGroup()
|
virtual |
Set the collision family group of this model.
This is an alternative way of specifying the collision family for this object. The value family_group must have a single bit set (i.e. it must be a power of 2). The corresponding family is then the bit position.
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ SetFamilyMask()
|
virtual |
Set the collision mask for this model.
Any set bit in the specified mask indicates that this model collides with all objects whose family is equal to the bit position.
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ SetFamilyMaskNoCollisionWithFamily()
|
virtual |
By default, family mask is all turned on, so all families can collide with this object, but you can turn on-off some bytes of this mask so that some families do not collide.
When two objects collide, the contact is created only if the family is within the 'family mask' of the other, and viceversa. These default implementations use the family mask.
Reimplemented in chrono::collision::ChCollisionModelBullet.
◆ SetSafeMargin()
|
inlinevirtual |
Set the suggested collision 'inward safe margin' for the shapes to be added from now on.
If this margin is too high for some thin or small shapes, it may be clamped. If dist<0 and inter-penetration occurs (e.g. due to numerical errors) within this 'safe margin' inward range, collision detection is still fast and reliable (beyond this, for deep penetrations, CD still works, but might be slower and less reliable) Call this BEFORE adding the shapes into the model. Side effect: think of the margin as a radius of a 'smoothing' fillet on all corners of the shapes - that's why you cannot exceed with this.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/collision/ChCollisionModel.h
- /builds/uwsbel/chrono/src/chrono/collision/ChCollisionModel.cpp