Description
Performs a step of Newmark constrained implicit for II order DAE systems.
See Negrut et al. 2007.
#include <ChTimestepper.h>
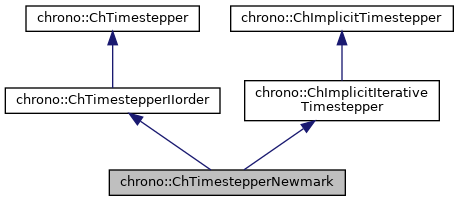

Public Member Functions | |
ChTimestepperNewmark (ChIntegrableIIorder *intgr=nullptr) | |
Constructors (default empty) | |
virtual Type | GetType () const override |
Return type of the integration method. More... | |
void | SetGammaBeta (double mgamma, double mbeta) |
Set the numerical damping parameter gamma and the beta parameter. More... | |
double | GetGamma () |
double | GetBeta () |
void | SetModifiedNewton (bool val) |
Enable/disable modified Newton. More... | |
virtual void | Advance (const double dt) override |
Performs an integration timestep. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChTimestepperIIorder (ChIntegrableIIorder *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepperIIorder () |
Destructor. | |
virtual ChState & | GetStatePos () |
Access the state, position part, at current time. | |
virtual ChStateDelta & | GetStateVel () |
Access the state, speed part, at current time. | |
virtual ChStateDelta & | GetStateAcc () |
Access the acceleration, at current time. | |
virtual void | SetIntegrable (ChIntegrableIIorder *intgr) |
Set the integrable object. | |
![]() | |
ChTimestepper (ChIntegrable *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepper () |
Destructor. | |
virtual ChVectorDynamic & | GetLagrangeMultipliers () |
Access the lagrangian multipliers, if any. | |
virtual void | SetIntegrable (ChIntegrable *intgr) |
Set the integrable object. | |
ChIntegrable * | GetIntegrable () |
Get the integrable object. | |
virtual double | GetTime () const |
Get the current time. | |
virtual void | SetTime (double mt) |
Set the current time. | |
void | SetVerbose (bool verb) |
Turn on/off logging of messages. | |
![]() | |
void | SetMaxIters (int iters) |
Set the max number of iterations using the Newton Raphson procedure. | |
double | GetMaxIters () |
Get the max number of iterations using the Newton Raphson procedure. | |
void | SetRelTolerance (double rel_tol) |
Set the relative tolerance. More... | |
void | SetAbsTolerances (double abs_tolS, double abs_tolL) |
Set the absolute tolerances. More... | |
void | SetAbsTolerances (double abs_tol) |
Set the absolute tolerances. More... | |
unsigned int | GetNumIterations () const |
Return the number of iterations. | |
unsigned int | GetNumSetupCalls () const |
Return the number of calls to the solver's Setup function. | |
unsigned int | GetNumSolveCalls () const |
Return the number of calls to the solver's Solve function. | |
Additional Inherited Members | |
![]() | |
enum | Type { EULER_IMPLICIT_LINEARIZED, EULER_IMPLICIT_PROJECTED, EULER_IMPLICIT, TRAPEZOIDAL, TRAPEZOIDAL_LINEARIZED, HHT, HEUN, RUNGEKUTTA45, EULER_EXPLICIT, LEAPFROG, NEWMARK, CUSTOM } |
Available methods for time integration (time steppers). | |
![]() | |
static std::string | GetTypeAsString (Type type) |
Return the integrator type as a string. | |
![]() | |
ChState | X |
ChStateDelta | V |
ChStateDelta | A |
![]() | |
ChIntegrable * | integrable |
double | T |
ChVectorDynamic | L |
bool | verbose |
bool | Qc_do_clamp |
double | Qc_clamping |
![]() | |
unsigned int | maxiters |
maximum number of iterations | |
double | reltol |
relative tolerance | |
double | abstolS |
absolute tolerance (states) | |
double | abstolL |
absolute tolerance (Lagrange multipliers) | |
unsigned int | numiters |
number of iterations | |
unsigned int | numsetups |
number of calls to the solver's Setup function | |
unsigned int | numsolves |
number of calls to the solver's Solve function | |
Member Function Documentation
◆ Advance()
|
overridevirtual |
Performs an integration timestep.
- Parameters
-
dt timestep to advance
Implements chrono::ChTimestepper.
◆ GetType()
|
inlineoverridevirtual |
Return type of the integration method.
Default is CUSTOM. Derived classes should override this function.
Reimplemented from chrono::ChTimestepper.
◆ SetGammaBeta()
void chrono::ChTimestepperNewmark::SetGammaBeta | ( | double | mgamma, |
double | mbeta | ||
) |
Set the numerical damping parameter gamma and the beta parameter.
Gamma: in the [1/2, 1] interval. For gamma = 1/2, no numerical damping For gamma > 1/2, more damping Beta: in the [0, 1] interval. For beta = 1/4, gamma = 1/2 -> constant acceleration method For beta = 1/6, gamma = 1/2 -> linear acceleration method Method is second order accurate only for gamma = 1/2
◆ SetModifiedNewton()
|
inline |
Enable/disable modified Newton.
If enabled, the Newton matrix is evaluated, assembled, and factorized only once per step. If disabled, the Newton matrix is evaluated at every iteration of the nonlinear solver. Modified Newton iteration is enabled by default.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepper.h
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepper.cpp