Description
Collision engine based on the Bullet library.
Contains both the broadphase and the narrow phase Bullet methods.
#include <ChCollisionSystemBullet.h>
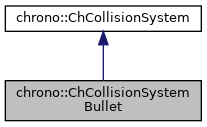
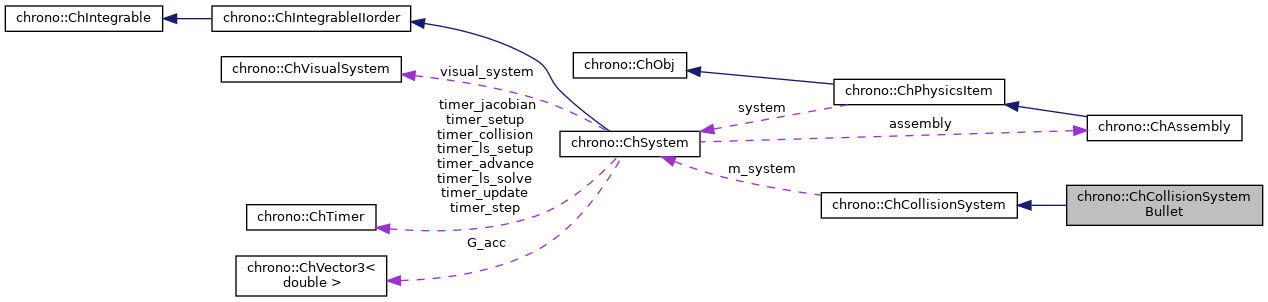
Public Member Functions | |
ChCollisionSystemBullet () | |
virtual void | Clear () override |
Clears all data instanced by this algorithm if any (like persistent contact manifolds) | |
virtual void | Add (std::shared_ptr< ChCollisionModel > model) override |
Add the specified collision model to the collision engine. | |
virtual void | Remove (std::shared_ptr< ChCollisionModel > model) override |
Remove the specified collision model from the collision engine. | |
virtual void | SetNumThreads (int nthreads) override |
Removes all collision models from the collision engine (custom data may be deallocated). More... | |
virtual void | Run () override |
Run the algorithm and finds all the contacts. More... | |
virtual ChAABB | GetBoundingBox () const override |
Return an AABB bounding all collision shapes in the system. | |
virtual void | ResetTimers () override |
Reset timers for collision detection. | |
virtual double | GetTimerCollisionBroad () const override |
Return the time (in seconds) for broadphase collision detection. | |
virtual double | GetTimerCollisionNarrow () const override |
Return the time (in seconds) for narrowphase collision detection. | |
virtual void | ReportContacts (ChContactContainer *mcontactcontainer) override |
After the Run() has completed, you can call this function to fill a 'contact container', that is an object inherited from class ChContactContainer. More... | |
virtual void | ReportProximities (ChProximityContainer *mproximitycontainer) override |
After the Run() has completed, you can call this function to fill a 'proximity container' (container of narrow phase pairs), that is an object inherited from class ChProximityContainer. More... | |
virtual bool | RayHit (const ChVector3d &from, const ChVector3d &to, ChRayhitResult &result) const override |
Perform a ray-hit test with all collision models. | |
virtual bool | RayHit (const ChVector3d &from, const ChVector3d &to, ChCollisionModel *model, ChRayhitResult &result) const override |
Perform a ray-hit test with the specified collision model. | |
virtual void | RegisterVisualizationCallback (std::shared_ptr< VisualizationCallback > callback) override |
Specify a callback object to be used for debug rendering of collision shapes. | |
virtual void | Visualize (int flags) override |
Method to trigger debug visualization of collision shapes. More... | |
cbtCollisionWorld * | GetBulletCollisionWorld () |
Get the underlying Bullet collision world. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
bool | IsInitialized () const |
Test if the collision system was initialized. | |
virtual void | Initialize () |
Initialize the collision system. More... | |
virtual void | BindAll () |
Process all collision models in the associated Chrono system. More... | |
virtual void | BindItem (std::shared_ptr< ChPhysicsItem > item) |
Process the collision models associated with the specified Chrono physics item. More... | |
void | UnbindItem (std::shared_ptr< ChPhysicsItem > item) |
Remove any collision models associated with the specified physics item from the collision engine. | |
virtual void | PreProcess () |
Optional synchronization operations, invoked before running the collision detection. | |
virtual void | PostProcess () |
Optional synchronization operations, invoked after running the collision detection. | |
void | RegisterBroadphaseCallback (std::shared_ptr< BroadphaseCallback > callback) |
Specify a callback object to be used each time a pair of 'near enough' collision shapes is found by the broad-phase collision step. More... | |
void | RegisterNarrowphaseCallback (std::shared_ptr< NarrowphaseCallback > callback) |
Specify a callback object to be used each time a collision pair is found during the narrow-phase collision detection step. More... | |
void | SetSystem (ChSystem *sys) |
Set associated Chrono system. | |
Static Public Member Functions | |
static void | SetContactBreakingThreshold (double threshold) |
Change default contact breaking/merging threshold tolerance of Bullet. More... | |
Protected Member Functions | |
bool | RayHit (const ChVector3d &from, const ChVector3d &to, ChRayhitResult &result, short int filter_group, short int filter_mask) const |
Perform a ray-hit test with all collision models. More... | |
bool | RayHit (const ChVector3d &from, const ChVector3d &to, ChCollisionModel *model, ChRayhitResult &result, short int filter_group, short int filter_mask) const |
Perform a ray-hit test with the specified collision model. More... | |
void | Remove (ChCollisionModelBullet *bt_model, bool erase) |
Remove the specified Bullet model from this collision system. More... | |
Protected Attributes | |
std::vector< std::shared_ptr< ChCollisionModelBullet > > | bt_models |
cbtCollisionConfiguration * | bt_collision_configuration |
cbtCollisionDispatcher * | bt_dispatcher |
cbtBroadphaseInterface * | bt_broadphase |
cbtCollisionWorld * | bt_collision_world |
cbtCollisionAlgorithmCreateFunc * | m_collision_capsule_box |
cbtCollisionAlgorithmCreateFunc * | m_collision_box_capsule |
cbtCollisionAlgorithmCreateFunc * | m_collision_cylshell_box |
cbtCollisionAlgorithmCreateFunc * | m_collision_box_cylshell |
cbtCollisionAlgorithmCreateFunc * | m_collision_arc_seg |
cbtCollisionAlgorithmCreateFunc * | m_collision_seg_arc |
cbtCollisionAlgorithmCreateFunc * | m_collision_arc_arc |
cbtCollisionAlgorithmCreateFunc * | m_collision_cetri_cetri |
void * | m_tmp_mem |
cbtCollisionAlgorithmCreateFunc * | m_emptyCreateFunc |
cbtIDebugDraw * | m_debug_drawer |
![]() | |
bool | m_initialized |
ChSystem * | m_system |
associated Chrono system | |
std::shared_ptr< BroadphaseCallback > | broad_callback |
user callback for each near-enough pair of shapes | |
std::shared_ptr< NarrowphaseCallback > | narrow_callback |
user callback for each collision pair | |
std::shared_ptr< VisualizationCallback > | vis_callback |
user callback for debug visualization | |
int | m_vis_flags |
Friends | |
class | ChCollisionModelBullet |
Additional Inherited Members | |
![]() | |
enum | Type { Type::BULLET, Type::MULTICORE } |
Supported collision systems. More... | |
enum | VisualizationModes { VIS_None = 0, VIS_Shapes = 1 << 0, VIS_Aabb = 1 << 1, VIS_Contacts = 1 << 2, VIS_MAX_MODES } |
Enumeration of supported flags for collision debug visualization. More... | |
Constructor & Destructor Documentation
◆ ChCollisionSystemBullet()
chrono::ChCollisionSystemBullet::ChCollisionSystemBullet | ( | ) |
bt_dispatcher->registerCollisionCreateFunc(SPHERE_SHAPE_PROXYTYPE, CYLINDER_SHAPE_PROXYTYPE, m_collision_sph_cyl); /bt_dispatcher->registerCollisionCreateFunc(CYLINDER_SHAPE_PROXYTYPE, SPHERE_SHAPE_PROXYTYPE, m_collision_cyl_sph);
Member Function Documentation
◆ RayHit() [1/2]
|
protected |
Perform a ray-hit test with the specified collision model.
This version allows specifying the Bullet collision filter group and mask (see cbtBroadphaseProxy::CollisionFilterGroups).
◆ RayHit() [2/2]
|
protected |
Perform a ray-hit test with all collision models.
This version allows specifying the Bullet collision filter group and mask (see cbtBroadphaseProxy::CollisionFilterGroups).
◆ Remove()
|
protected |
Remove the specified Bullet model from this collision system.
If erase=true, also remove from the bt_models list.
◆ ReportContacts()
|
overridevirtual |
After the Run() has completed, you can call this function to fill a 'contact container', that is an object inherited from class ChContactContainer.
For instance ChSystem, after each Run() collision detection, calls this method multiple times for all contact containers in the system, The basic behavior of the implementation is the following: collision system will call in sequence the functions BeginAddContact(), AddContact() (x n times), EndAddContact() of the contact container.
std::cout << " typeA=" << icontact.shapeA->m_type << " typeB=" << icontact.shapeB->m_type << std::endl;
Implements chrono::ChCollisionSystem.
◆ ReportProximities()
|
overridevirtual |
After the Run() has completed, you can call this function to fill a 'proximity container' (container of narrow phase pairs), that is an object inherited from class ChProximityContainer.
For instance ChSystem, after each Run() collision detection, calls this method multiple times for all proximity containers in the system, The basic behavior of the implementation is the following: collision system will call in sequence the functions BeginAddProximities(), AddProximity() (x n times), EndAddProximities() of the proximity container.
Implements chrono::ChCollisionSystem.
◆ Run()
|
overridevirtual |
Run the algorithm and finds all the contacts.
(Contacts will be managed by the Bullet persistent contact cache).
Implements chrono::ChCollisionSystem.
◆ SetContactBreakingThreshold()
|
static |
Change default contact breaking/merging threshold tolerance of Bullet.
This is the static gContactBreakingThreshold scalar in Bullet. Call this function only once, before running the simulation.
◆ SetNumThreads()
|
overridevirtual |
Removes all collision models from the collision engine (custom data may be deallocated).
Set the number of OpenMP threads for collision detection.
Reimplemented from chrono::ChCollisionSystem.
◆ Visualize()
|
overridevirtual |
Method to trigger debug visualization of collision shapes.
The 'flags' argument can be any of the VisualizationModes enums, or a combination thereof (using bit-wise operators). The calling program must invoke this function from within the simulation loop. No-op if a visualization callback was not specified with RegisterVisualizationCallback().
Reimplemented from chrono::ChCollisionSystem.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/collision/bullet/ChCollisionSystemBullet.h
- /builds/uwsbel/chrono/src/chrono/collision/bullet/ChCollisionSystemBullet.cpp