Description
Base class for loads representing a concentrated force acting between two ChNodeXYZ.
Users should inherit from this and implement a custom ComputeForce(). Note: some predefined examples are provided, such as ChLoadNodeXYZNodeXYZSpring ChLoadNodeXYZNodeXYZBushing.
#include <ChLoadsNodeXYZ.h>
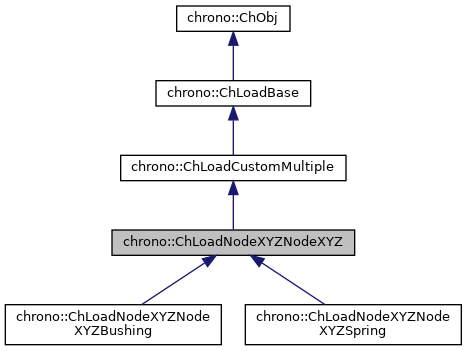
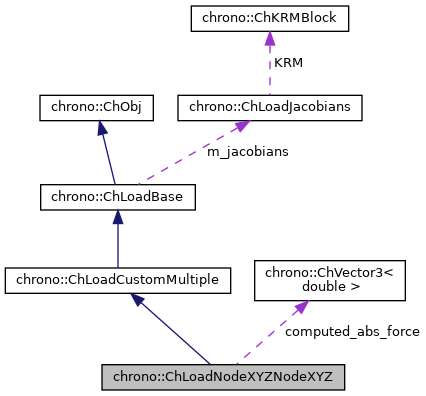
Public Member Functions | |
ChLoadNodeXYZNodeXYZ (std::shared_ptr< ChNodeXYZ > nodeA, std::shared_ptr< ChNodeXYZ > nodeB) | |
virtual void | ComputeForce (const ChVector3d &rel_pos, const ChVector3d &rel_vel, ChVector3d &abs_force)=0 |
Compute the force on the nodeA, in absolute coordsystem, given relative position of nodeA with respect to B. | |
virtual void | ComputeQ (ChState *state_x, ChStateDelta *state_w) override |
Compute Q, the generalized load. More... | |
ChVector3d | GetForce () const |
Return the last computed value of the applied force. More... | |
![]() | |
ChLoadCustomMultiple (std::vector< std::shared_ptr< ChLoadable >> &loadable_objects) | |
ChLoadCustomMultiple (std::shared_ptr< ChLoadable > loadableA, std::shared_ptr< ChLoadable > loadableB) | |
ChLoadCustomMultiple (std::shared_ptr< ChLoadable > loadableA, std::shared_ptr< ChLoadable > loadableB, std::shared_ptr< ChLoadable > loadableC) | |
virtual int | LoadGetNumCoordsPosLevel () override |
Gets the number of DOFs affected by this load (position part). | |
virtual int | LoadGetNumCoordsVelLevel () override |
Gets the number of DOFs affected by this load (speed part). | |
virtual void | LoadGetStateBlock_x (ChState &mD) override |
Gets all the current DOFs packed in a single vector (position part). | |
virtual void | LoadGetStateBlock_w (ChStateDelta &mD) override |
Gets all the current DOFs packed in a single vector (speed part). | |
virtual void | LoadStateIncrement (const ChState &x, const ChStateDelta &dw, ChState &x_new) override |
Increment a packed state (e.g., as obtained by LoadGetStateBlock_x()) by a given packed state-delta. More... | |
virtual int | LoadGetNumFieldCoords () override |
Number of coordinates in the interpolated field. More... | |
virtual void | ComputeJacobian (ChState *state_x, ChStateDelta *state_w) override |
Compute Jacobian matrices K=-dQ/dx, R=-dQ/dv, and M=-dQ/da. More... | |
virtual void | LoadIntLoadResidual_F (ChVectorDynamic<> &R, double c) override |
Add the internal loads Q (pasted at global offsets) into a global vector R, multiplied by a scaling factor c. More... | |
virtual void | LoadIntLoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, double c) override |
Increment a vector R with the matrix-vector product M*w, scaled by the factor c. More... | |
virtual void | LoadIntLoadLumpedMass_Md (ChVectorDynamic<> &Md, double &err, const double c) override |
Add the lumped mass to an Md vector, representing a mass diagonal matrix. More... | |
virtual void | CreateJacobianMatrices () override |
Create the Jacobian loads if needed and set the ChVariables referenced by the sparse KRM block. | |
virtual ChVectorDynamic & | GetQ () |
Access the generalized load vector Q. | |
![]() | |
ChLoadJacobians * | GetJacobians () |
Access the Jacobians (if any, i.e. if this is a stiff load). | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
![]() | |
ChObj (const ChObj &other) | |
virtual ChObj * | Clone () const =0 |
"Virtual" copy constructor. | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
virtual std::string & | ArchiveContainerName () |
Protected Member Functions | |
virtual bool | IsStiff () override |
Report if this is load is stiff. More... | |
virtual void | Update (double time) override |
Update, called at least at each time step. More... | |
![]() | |
int | GenerateUniqueIdentifier () |
Protected Attributes | |
ChVector3d | computed_abs_force |
![]() | |
ChLoadJacobians * | m_jacobians |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
Additional Inherited Members | |
![]() | |
std::vector< std::shared_ptr< ChLoadable > > | loadables |
ChVectorDynamic | load_Q |
Constructor & Destructor Documentation
◆ ChLoadNodeXYZNodeXYZ()
chrono::ChLoadNodeXYZNodeXYZ::ChLoadNodeXYZNodeXYZ | ( | std::shared_ptr< ChNodeXYZ > | nodeA, |
std::shared_ptr< ChNodeXYZ > | nodeB | ||
) |
- Parameters
-
nodeA node A to apply load to nodeB node B to apply load to, as reaction
Member Function Documentation
◆ ComputeQ()
|
overridevirtual |
Compute Q, the generalized load.
Called automatically at each Update().
- Parameters
-
state_x state position to evaluate Q state_w state speed to evaluate Q
Implements chrono::ChLoadBase.
◆ GetForce()
|
inline |
Return the last computed value of the applied force.
Used primarily for diagnostics, this function returns the force on the node expressed in absolute coordinates.
◆ IsStiff()
|
inlineoverrideprotectedvirtual |
Report if this is load is stiff.
If so, InjectKRMMatrices will provide the Jacobians of the load.
Implements chrono::ChLoadBase.
Reimplemented in chrono::ChLoadNodeXYZNodeXYZBushing, and chrono::ChLoadNodeXYZNodeXYZSpring.
◆ Update()
|
overrideprotectedvirtual |
Update, called at least at each time step.
- It recomputes the generalized load Q vector(s)
- It recomputes the Jacobian matrices K,R,M in case of stiff load Q and Jacobians assumed evaluated at the current state. Jacobians are automatically allocated if needed.
Reimplemented from chrono::ChLoadBase.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsNodeXYZ.h
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsNodeXYZ.cpp