chrono::ChFunctionConstJerk Class Reference
Description
Ramp function composed by seven segments with constant jerk.
(aka. 'seven segments', 'double-S', 'trapezoidal acceleration').
#include <ChFunctionConstJerk.h>
Inheritance diagram for chrono::ChFunctionConstJerk:
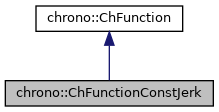
Collaboration diagram for chrono::ChFunctionConstJerk:
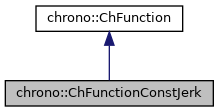
Public Member Functions | |
ChFunctionConstJerk () | |
Default constructor. | |
ChFunctionConstJerk (double q0, double q1, double v0, double v1, double T, double Ta, double Tj) | |
Simplified case constant jerk, with imposed boundary conditions and times. More... | |
ChFunctionConstJerk (double q0, double q1, double vmax, double amax, double jmax) | |
Simplified case constant jerk. More... | |
ChFunctionConstJerk (bool &feasible, double q0, double q1, double v0, double v1, double vmax, double amax, double jmax) | |
General case constant jerk. More... | |
ChFunctionConstJerk (const ChFunctionConstJerk &other) | |
virtual ChFunctionConstJerk * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual Type | GetType () const override |
Get type of ChFunction. | |
void | Setup (double q0, double q1, double v0, double v1, double T, double Ta, double Tj) |
Setup internal data of constant jerk, imposed times case. | |
void | Setup (double q0, double q1, double vmax, double amax, double jmax) |
Setup internal data of constant jerk, simplified case for minimization of motion time with v0 = v1 = 0. | |
void | Setup (bool &feasible, double q0, double q1, double v0, double v1, double vmax, double amax, double jmax) |
Setup internal data of constant jerk, general case for minimization of motion time. | |
virtual double | GetVal (double x) const override |
Position: return the y value of the function, at position x. | |
virtual double | GetDer (double x) const override |
Velocity: return the dy/dx derivative of the function, at position x. | |
virtual double | GetDer2 (double x) const override |
Acceleration: return the ddy/dxdx double derivative of the function, at position x. | |
virtual double | GetDer3 (double x) const override |
Jerk: return the dddy/dxdxdx triple derivative of the function, at position x. | |
double | GetDisplacement () const |
Get total displacement. | |
double | GetDuration () const |
Get total motion time. | |
void | GetBoundaryConditions (double &q0, double &q1, double &v0, double &v1) |
Get boundary conditions. | |
void | GetImposedLimits (double &vmax, double &amax, double &jmax) |
Get imposed limits on velocity, acceleration and jerk. | |
void | GetTimes (double &T, double &Ta, double &Tv, double &Td, double &Tj1, double &Tj2) |
Get internal motion times. | |
void | GetReachedLimits (double &vlim, double &alim_a, double &alim_d) |
Get maximum velocity/acceleration/deceleration effectively reached during motion law. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChFunction (const ChFunction &other) | |
virtual double | GetDerN (double x, int der_order) const |
Return the Nth derivative of the function (up to 3rd derivative). More... | |
virtual double | GetWeight (double x) const |
Return the weight of the function. More... | |
virtual void | Update (double x) |
Update the function at the provided value of its argument. | |
virtual double | GetMax (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the maximum of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetMin (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the minimum of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetMean (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the mean of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetSquaredMean (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the squared mean of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetIntegral (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the integral of the function (or its der_order derivative) over the range [xmin, xmax]. More... | |
virtual double | GetPositiveAccelerationCoeff () const |
Compute the positive acceleration coefficient. More... | |
virtual double | GetNegativeAccelerationCoeff () const |
Compute the negative acceleration coefficient. More... | |
virtual double | GetVelocityCoefficient () const |
Compute the velocity coefficient. More... | |
virtual void | OutputToASCIIFile (std::ostream &file, double xmin, double xmax, int samples, char delimiter) |
Store X-Y pairs to an ASCII File. More... | |
virtual ChMatrixDynamic | SampleUpToDerN (double xmin, double xmax, double step, int derN=0) |
Sample function on given interval [xmin, xmax], up to derN derivative (0 being the function ouput itself). More... | |
double | operator() (double arg) const |
Alias operator of the GetVal function. | |
Additional Inherited Members | |
![]() | |
enum | Type { BSPLINE, CONSTANT, CONSTACC, CONSTJERK, CUSTOM, CYCLOIDAL, DERIVATIVE, FILLET3, INTEGRAL, INTERP, LAMBDA, MIRROR, OPERATOR, POLY, POLY23, POLY345, RAMP, REPEAT, SEQUENCE, SINE, SINE_STEP } |
Enumeration of function types. | |
Constructor & Destructor Documentation
◆ ChFunctionConstJerk() [1/3]
chrono::ChFunctionConstJerk::ChFunctionConstJerk | ( | double | q0, |
double | q1, | ||
double | v0, | ||
double | v1, | ||
double | T, | ||
double | Ta, | ||
double | Tj | ||
) |
Simplified case constant jerk, with imposed boundary conditions and times.
Eg. for q1 > q0, it produces a motion profile characterized by
- time: [0, Tj, Ta-Tj, Ta, Ta+Tv, Ta+Tv+Tj, T-Tj, T]
- jerk: [+jmax, 0, -jmax, 0, -jmax, 0, +jmax]
- acceleration: [+lin, const, -lin, zero, -lin, const, +lin]
NB: Ta = (0..1/2) * T; Tj = (0..1/2) * Ta
- Parameters
-
q0 start position q1 end position v0 start velocity v1 end velocity T total motion time Ta acceleration time (corresponds to first accel trapezoid) -> NB: Ta = (0..1/2) * T Tj jerk time (corresponds to first jerk square wave) -> NB: Tj = (0..1/2) * Ta
◆ ChFunctionConstJerk() [2/3]
chrono::ChFunctionConstJerk::ChFunctionConstJerk | ( | double | q0, |
double | q1, | ||
double | vmax, | ||
double | amax, | ||
double | jmax | ||
) |
Simplified case constant jerk.
Under
- imposed boundary positions
- (assumed) zero boundary velocities
- (assumed) zero boundary accelerations
- symmetric kinematic constraints on max |velocity|, |acceleration|, |jerk| minimizes total motion time.
- Parameters
-
q0 start position q1 end position vmax kinematic constraint: (abs) max allowed velocity amax kinematic constraint: (abs) max allowed acceleration jmax kinematic constraint: (abs) max allowed jerk
◆ ChFunctionConstJerk() [3/3]
chrono::ChFunctionConstJerk::ChFunctionConstJerk | ( | bool & | feasible, |
double | q0, | ||
double | q1, | ||
double | v0, | ||
double | v1, | ||
double | vmax, | ||
double | amax, | ||
double | jmax | ||
) |
General case constant jerk.
Under
- imposed boundary positions
- imposed boundary velocities
- (assumed) zero boundary accelerations
- symmetric kinematic constraints on max |velocity|, |acceleration|, |jerk| attempts to minimize total motion time.
NB: if desired motion law is not feasible, everything is set to zero (try to relax constraints).
- Parameters
-
feasible output: will be set to true if desired motlaw is feasible, false otherwise q0 start position q1 end position v0 start velocity v1 end velocity vmax kinematic constraint: (abs) max allowed velocity amax kinematic constraint: (abs) max allowed acceleration jmax kinematic constraint: (abs) max allowed jerk
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/functions/ChFunctionConstJerk.h
- /builds/uwsbel/chrono/src/chrono/functions/ChFunctionConstJerk.cpp