chrono::vehicle::m113::M113_ShaftsPowertrain Class Reference
Description
Shafts-based powertrain model for the M113 vehicle.
#include <M113_ShaftsPowertrain.h>
Inheritance diagram for chrono::vehicle::m113::M113_ShaftsPowertrain:
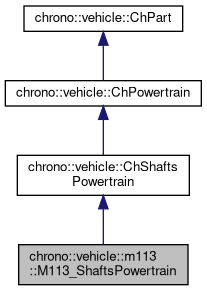
Collaboration diagram for chrono::vehicle::m113::M113_ShaftsPowertrain:
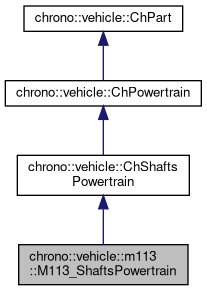
Public Member Functions | |
M113_ShaftsPowertrain (const std::string &name) | |
virtual void | SetGearRatios (std::vector< double > &fwd, double &rev) override |
Set the transmission gear ratios (one or more forward gear ratios and a single reverse gear ratio). | |
virtual double | GetMotorBlockInertia () const override |
Inertias of the component ChShaft objects. | |
virtual double | GetCrankshaftInertia () const override |
virtual double | GetIngearShaftInertia () const override |
virtual double | GetPowershaftInertia () const override |
virtual double | GetUpshiftRPM () const override |
Upshift and downshift rotation speeds (in RPM) | |
virtual double | GetDownshiftRPM () const override |
virtual void | SetEngineTorqueMap (std::shared_ptr< ChFunction_Recorder > &map) override |
Engine speed-torque map. | |
virtual void | SetEngineLossesMap (std::shared_ptr< ChFunction_Recorder > &map) override |
Engine speed-torque braking effect because of losses. | |
virtual void | SetTorqueConverterCapacityFactorMap (std::shared_ptr< ChFunction_Recorder > &map) override |
Set the capacity factor map. More... | |
virtual void | SetTorqeConverterTorqueRatioMap (std::shared_ptr< ChFunction_Recorder > &map) override |
Set the torque ratio map. More... | |
![]() | |
ChShaftsPowertrain (const std::string &name, const ChVector<> &dir_motor_block=ChVector<>(1, 0, 0)) | |
Construct a shafts-based powertrain model. | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual double | GetMotorSpeed () const override |
Return the current engine speed. | |
virtual double | GetMotorTorque () const override |
Return the current engine torque. | |
virtual double | GetTorqueConverterSlippage () const override |
Return the value of slippage in the torque converter. | |
virtual double | GetTorqueConverterInputTorque () const override |
Return the input torque to the torque converter. | |
virtual double | GetTorqueConverterOutputTorque () const override |
Return the output torque from the torque converter. | |
virtual double | GetTorqueConverterOutputSpeed () const override |
Return the torque converter output shaft speed. | |
virtual double | GetOutputTorque () const override |
Return the output torque from the powertrain. More... | |
void | SetGearShiftLatency (double ml) |
Use this to define the gear shift latency, in seconds. | |
double | GetGearShiftLatency (double ml) |
Use this to get the gear shift latency, in seconds. | |
![]() | |
int | GetCurrentTransmissionGear () const |
Return the current transmission gear. More... | |
void | SetDriveMode (DriveMode mode) |
Set the drive mode. | |
DriveMode | GetDriveMode () const |
Return the current drive mode. | |
void | SetTransmissionMode (TransmissionMode mode) |
Set the transmission mode (automatic or manual). More... | |
TransmissionMode | GetTransmissionMode () const |
Get the current transmission mode. | |
void | ShiftUp () |
Shift up. | |
void | ShiftDown () |
Shift down. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Additional Inherited Members | |
![]() | |
enum | DriveMode { DriveMode::FORWARD, DriveMode::NEUTRAL, DriveMode::REVERSE } |
Driving modes. More... | |
enum | TransmissionMode { TransmissionMode::AUTOMATIC, TransmissionMode::MANUAL } |
Transmission mode. More... | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
ChPowertrain (const std::string &name) | |
void | SetGear (int gear) |
Shift to the specified gear. More... | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
TransmissionMode | m_transmission_mode |
transmission mode (automatic or manual) | |
DriveMode | m_drive_mode |
drive mode (neutral, forward, or reverse) | |
std::shared_ptr< ChDriveline > | m_driveline |
associated driveline subsystem | |
std::vector< double > | m_gear_ratios |
gear ratios (0: reverse, 1+: forward) | |
int | m_current_gear |
current transmission gear (0: reverse, 1+: forward) | |
double | m_current_gear_ratio |
current gear ratio (positive for forward, negative for reverse) | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
Member Function Documentation
◆ SetTorqeConverterTorqueRatioMap()
|
overridevirtual |
Set the torque ratio map.
Specify torque ratio as a function of the speed ratio.
Implements chrono::vehicle::ChShaftsPowertrain.
◆ SetTorqueConverterCapacityFactorMap()
|
overridevirtual |
Set the capacity factor map.
Specify the capacity factor as a function of the speed ratio.
Implements chrono::vehicle::ChShaftsPowertrain.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/m113/M113_ShaftsPowertrain.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/m113/M113_ShaftsPowertrain.cpp