chrono::vehicle::ReissnerTire Class Reference
Description
Tire with Reissner shells, constructed with data from file (JSON format).
#include <ReissnerTire.h>
Inheritance diagram for chrono::vehicle::ReissnerTire:
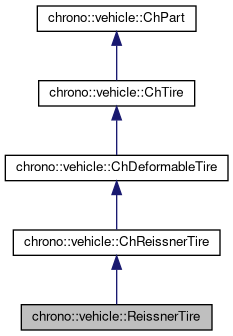
Collaboration diagram for chrono::vehicle::ReissnerTire:
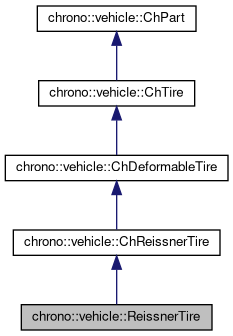
Public Member Functions | |
ReissnerTire (const std::string &filename) | |
ReissnerTire (const rapidjson::Document &d) | |
virtual double | GetRadius () const override |
Get the tire radius. | |
virtual double | GetRimRadius () const override |
Get the rim radius (inner tire radius). | |
virtual double | GetWidth () const override |
Get the tire width. | |
virtual double | GetDefaultPressure () const override |
Get the default tire pressure. | |
virtual std::vector< std::shared_ptr< fea::ChNodeFEAbase > > | GetConnectedNodes () const override |
Return list of nodes connected to the rim. | |
virtual void | CreateMesh (const ChFrameMoving<> &wheel_frame, VehicleSide side) override |
Create the FEA nodes and elements. More... | |
![]() | |
ChReissnerTire (const std::string &name) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
![]() | |
ChDeformableTire (const std::string &name) | |
Construct a deformable tire with the specified name. | |
void | SetContactSurfaceType (ContactSurfaceType type) |
Set the type of contact surface. | |
ContactSurfaceType | GetContactSurfaceType () const |
void | SetContactNodeRadius (double radius) |
Set radius of contact nodes. More... | |
double | GetContactNodeRadius () const |
void | SetContactFaceThickness (double thickness) |
Set thickness of contact faces (radius of swept sphere). More... | |
double | GetContactFaceThickness () const |
std::shared_ptr< ChMaterialSurfaceSMC > | GetContactMaterial () const |
Get the tire contact material. More... | |
void | EnablePressure (bool val) |
Enable/disable tire pressure (default: true). | |
bool | IsPressureEnabled () const |
void | EnableContact (bool val) |
Enable/disable tire contact (default: true). | |
bool | IsContactEnabled () const |
void | EnableRimConnection (bool val) |
Enable/disable tire-rim connection (default: true). | |
bool | IsRimConnectionEnabled () const |
fea::ChVisualizationFEAmesh * | GetMeshVisualization () const |
Get a handle to the mesh visualization. | |
std::shared_ptr< fea::ChMesh > | GetMesh () const |
Get the underlying FEA mesh. | |
std::shared_ptr< fea::ChContactSurface > | GetContactSurface () const |
Get the mesh contact surface. More... | |
std::shared_ptr< ChLoadContainer > | GetLoadContainer () const |
Get the load container associated with this tire. | |
void | SetPressure (double pressure) |
Set the tire pressure. | |
double | GetTireMass () const |
Calculate and return the tire mass. More... | |
virtual double | ReportMass () const override |
Report the tire mass. | |
virtual TerrainForce | ReportTireForce (ChTerrain *terrain) const override |
Report the tire force and moment. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override final |
Add visualization assets for the rigid tire subsystem. | |
virtual void | RemoveVisualizationAssets () override final |
Remove visualization assets for the rigid tire subsystem. | |
![]() | |
ChTire (const std::string &name) | |
void | SetStepsize (double val) |
Set the value of the integration step size for the underlying dynamics (if applicable). More... | |
double | GetStepsize () const |
Get the current value of the integration step size. | |
void | SetCollisionType (CollisionType collision_type) |
Set the collision type for tire-terrain interaction. More... | |
double | GetSlipAngle () const |
Return the tire slip angle calculated based on the current state of the associated wheel body. More... | |
double | GetLongitudinalSlip () const |
Return the tire longitudinal slip calculated based on the current state of the associated wheel body. More... | |
double | GetCamberAngle () const |
Return the tire camber angle calculated based on the current state of the associated wheel body. More... | |
virtual double | GetDeflection () const |
Report the tire deflection. | |
const std::string & | GetMeshFilename () const |
Get the name of the Wavefront file with tire visualization mesh. More... | |
virtual void | Synchronize (double time, const ChTerrain &terrain) |
Update the state of this tire system at the current time. More... | |
virtual void | Advance (double step) |
Advance the state of this tire by the specified time step. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Additional Inherited Members | |
![]() | |
enum | ContactSurfaceType { NODE_CLOUD, TRIANGLE_MESH } |
Type of the mesh contact surface. | |
![]() | |
enum | CollisionType { SINGLE_POINT, FOUR_POINTS, ENVELOPE } |
![]() | |
static ChVector | EstimateInertia (double tire_width, double aspect_ratio, double rim_diameter, double tire_mass, double t_factor=2) |
Utility function for estimating the tire moments of inertia. More... | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
virtual void | CreatePressureLoad () override final |
Create the ChLoad for applying pressure to the tire. | |
virtual void | CreateContactSurface () override final |
Create the contact surface for the tire mesh. | |
virtual void | CreateRimConnections (std::shared_ptr< ChBody > wheel) override final |
Create the tire-rim connections. | |
![]() | |
virtual double | GetMass () const final |
Get the tire mass. More... | |
virtual ChVector | GetInertia () const final |
Get the tire moments of inertia. More... | |
virtual void | Initialize (std::shared_ptr< ChWheel > wheel) override |
Initialize this tire by associating it to the specified wheel. | |
virtual TerrainForce | GetTireForce () const override |
Get the tire force and moment. More... | |
![]() | |
void | CalculateKinematics (double time, const WheelState &wheel_state, const ChTerrain &terrain) |
Calculate kinematics quantities based on the given state of the associated wheel body. More... | |
double | GetOffset () const |
Get offset from spindle center. More... | |
std::shared_ptr< ChTriangleMeshShape > | AddVisualizationMesh (const std::string &mesh_file_left, const std::string &mesh_file_right) |
Add mesh visualization to the body associated with this tire (a wheel spindle body). More... | |
void | RemoveVisualizationMesh (std::shared_ptr< ChTriangleMeshShape > trimesh_shape) |
Remove the specified mesh shape from the visualization assets of the body associated with this tire (a wheel spindle body). | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static bool | DiscTerrainCollision (const ChTerrain &terrain, const ChVector<> &disc_center, const ChVector<> &disc_normal, double disc_radius, ChCoordsys<> &contact, double &depth) |
Perform disc-terrain collision detection. More... | |
static bool | DiscTerrainCollision4pt (const ChTerrain &terrain, const ChVector<> &disc_center, const ChVector<> &disc_normal, double disc_radius, double width, ChCoordsys<> &contact, double &depth, double &camber_angle) |
Perform disc-terrain collision detection considering the curvature of the road surface. More... | |
static bool | DiscTerrainCollisionEnvelope (const ChTerrain &terrain, const ChVector<> &disc_center, const ChVector<> &disc_normal, double disc_radius, const ChFunction_Recorder &areaDep, ChCoordsys<> &contact, double &depth) |
Collsion algorithm based on a paper of J. More... | |
static void | ConstructAreaDepthTable (double disc_radius, ChFunction_Recorder &areaDep) |
Utility function to construct a loopkup table for penetration depth as function of intersection area, for a given tire radius. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
std::shared_ptr< fea::ChMesh > | m_mesh |
tire mesh | |
std::shared_ptr< ChLoadContainer > | m_load_container |
load container (for pressure load) | |
std::vector< std::shared_ptr< fea::ChLinkPointFrame > > | m_connections |
tire-wheel point connections | |
std::vector< std::shared_ptr< fea::ChLinkDirFrame > > | m_connectionsD |
tire-wheel direction connections | |
std::vector< std::shared_ptr< ChLinkMateFix > > | m_connectionsF |
tire-wheel fix connection (point+rotation) | |
bool | m_connection_enabled |
enable tire connections to rim | |
bool | m_pressure_enabled |
enable internal tire pressure | |
bool | m_contact_enabled |
enable tire-terrain contact | |
double | m_pressure |
internal tire pressure | |
ContactSurfaceType | m_contact_type |
type of contact surface model (node cloud or mesh) | |
double | m_contact_node_radius |
node radius (for node cloud contact surface) | |
double | m_contact_face_thickness |
face thickness (for mesh contact surface) | |
std::shared_ptr< ChMaterialSurfaceSMC > | m_contact_mat |
tire contact material | |
std::shared_ptr< fea::ChVisualizationFEAmesh > | m_visualization |
tire mesh visualization | |
![]() | |
std::shared_ptr< ChWheel > | m_wheel |
associated wheel subsystem | |
double | m_stepsize |
tire integration step size (if applicable) | |
CollisionType | m_collision_type |
method used for tire-terrain collision | |
std::string | m_vis_mesh_file |
name of OBJ file for visualization of this tire (may be empty) | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
Member Function Documentation
◆ CreateMesh()
|
overridevirtual |
Create the FEA nodes and elements.
The wheel rotational axis is assumed to be the Y axis.
- Parameters
-
wheel_frame frame of associated wheel side left/right vehicle side
Implements chrono::vehicle::ChDeformableTire.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/tire/ReissnerTire.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/tire/ReissnerTire.cpp