chrono::vehicle::ChTrackAssemblyBand Class Reference
Description
Definition of a continuous band track assembly.
A track assembly consists of a sprocket, an idler (with tensioner mechanism), a set of suspensions (road-wheel assemblies), and a collection of track shoes. This is the base class for continuous band track assembly using rigid tread. Derived classes specify the actual template defintions, using different track shoes.
#include <ChTrackAssemblyBand.h>
Inheritance diagram for chrono::vehicle::ChTrackAssemblyBand:
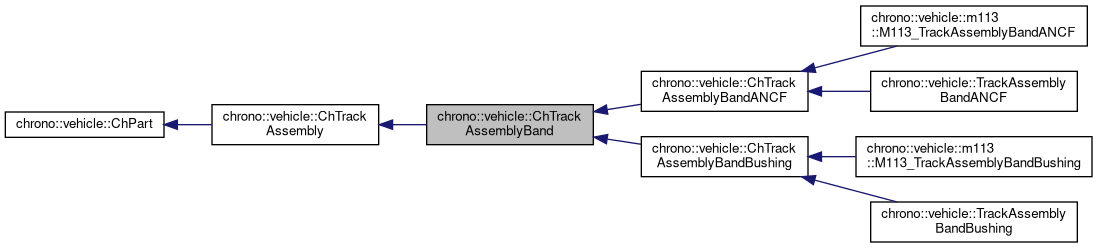
Collaboration diagram for chrono::vehicle::ChTrackAssemblyBand:
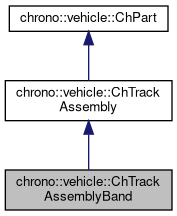
Public Member Functions | |
ChTrackAssemblyBand (const std::string &name, VehicleSide side) | |
virtual std::shared_ptr< ChSprocket > | GetSprocket () const override |
Get a handle to the sprocket. | |
![]() | |
VehicleSide | GetVehicleSide () const |
Return the vehicle side for this track assembly. | |
size_t | GetNumRoadWheelAssemblies () const |
Get the number of suspensions. | |
size_t | GetNumRollers () const |
Get the number of rollers. | |
virtual size_t | GetNumTrackShoes () const =0 |
Get the number of track shoes. | |
std::shared_ptr< ChIdler > | GetIdler () const |
Get a handle to the idler subsystem. | |
std::shared_ptr< ChTrackBrake > | GetBrake () const |
Get a handle to the brake subsystem. | |
const ChRoadWheelAssemblyList & | GetRoadWheelAssemblies () const |
Get the list of suspension subsystems. | |
std::shared_ptr< ChRoadWheelAssembly > | GetRoadWheelAssembly (size_t id) const |
Get a handle to the specified suspension subsystem. | |
std::shared_ptr< ChRoller > | GetRoller (size_t id) const |
Get a handle to the specified roller subsystem. | |
std::shared_ptr< ChRoadWheel > | GetRoadWheel (size_t id) const |
Get a handle to the specified road wheel subsystem. | |
virtual std::shared_ptr< ChTrackShoe > | GetTrackShoe (size_t id) const =0 |
Get a handle to the specified track shoe subsystem. | |
const ChVector & | GetTrackShoePos (size_t id) const |
Get the global location of the specified track shoe. More... | |
const ChQuaternion & | GetTrackShoeRot (size_t id) const |
Get the orientation of the specified track shoe. More... | |
const ChVector & | GetTrackShoeLinVel (size_t id) const |
Get the linear velocity of the specified track shoe. More... | |
ChVector | GetTrackShoeAngVel (size_t id) const |
Get the angular velocity of the specified track shoe. More... | |
BodyState | GetTrackShoeState (size_t id) const |
Get the complete state for the specified track shoe. More... | |
void | GetTrackShoeStates (BodyStates &states) const |
Get the complete states for all track shoes. More... | |
double | GetMass () const |
Get the total mass of the track assembly. More... | |
virtual const ChVector | GetSprocketLocation () const =0 |
Get the relative location of the sprocket subsystem. More... | |
virtual const ChVector | GetIdlerLocation () const =0 |
Get the relative location of the idler subsystem. More... | |
virtual const ChVector | GetRoadWhelAssemblyLocation (int which) const =0 |
Get the relative location of the specified suspension subsystem. More... | |
virtual const ChVector | GetRollerLocation (int which) const |
Get the relative location of the specified roller subsystem. More... | |
void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector<> &location, bool create_shoes=true) |
Initialize this track assembly subsystem. More... | |
void | SetSprocketVisualizationType (VisualizationType vis) |
Set visualization type for the sprocket subsystem. | |
void | SetIdlerVisualizationType (VisualizationType vis) |
void | SetRoadWheelAssemblyVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystems. | |
void | SetRoadWheelVisualizationType (VisualizationType vis) |
Set visualization type for the road-wheel subsystems. | |
void | SetRollerVisualizationType (VisualizationType vis) |
Set visualization type for the roller subsystems. | |
void | SetTrackShoeVisualizationType (VisualizationType vis) |
Set visualization type for the track shoe subsystems. | |
void | SetWheelCollisionType (bool roadwheel_as_cylinder, bool idler_as_cylinder, bool roller_as_cylinder) |
Set collision shape type for wheels. | |
void | Synchronize (double time, double braking, const TerrainForces &shoe_forces) |
Update the state of this track assembly at the current time. More... | |
virtual void | SetOutput (bool state) override |
Enable/disable output for this subsystem. More... | |
void | LogConstraintViolations () |
Log current constraint violations. | |
bool | IsRoadwheelCylinder () const |
bool | IsIdlerCylinder () const |
bool | IsRolerCylinder () const |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Member Functions | |
bool | FindAssemblyPoints (std::shared_ptr< ChBodyAuxRef > chassis, int num_shoes, const std::vector< double > &connection_lengths, std::vector< ChVector2<>> &shoe_points) |
Assembly Algorithm Utility Functions. | |
void | FindCircleTangentPoints (ChVector2<> Circle1Pos, double Circle1Rad, ChVector2<> Circle2Pos, double Circle2Rad, ChVector2<> &Tan1Pnt1, ChVector2<> &Tan1Pnt2, ChVector2<> &Tan2Pnt1, ChVector2<> &Tan2Pnt2) |
void | CheckCircleCircle (bool &found, ChVector2<> &Point, ChMatrixDynamic<> &Features, int FeatureIdx, ChVector2<> &StartingPoint, double Radius) |
void | CheckCircleLine (bool &found, ChVector2<> &Point, ChMatrixDynamic<> &Features, int FeatureIdx, ChVector2<> &StartingPoint, double Radius) |
![]() | |
ChTrackAssembly (const std::string &name, VehicleSide side) | |
virtual bool | Assemble (std::shared_ptr< ChBodyAuxRef > chassis)=0 |
Assemble track shoes over wheels. More... | |
virtual void | RemoveTrackShoes ()=0 |
Remove all track shoes from assembly. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const override |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const override |
Output data for this subsystem's component list to the specified database. | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
Protected Attributes | |
std::shared_ptr< ChSprocketBand > | m_sprocket |
sprocket subsystem | |
double | m_sprocket_offset |
![]() | |
VehicleSide | m_side |
assembly on left/right vehicle side | |
std::shared_ptr< ChIdler > | m_idler |
idler (and tensioner) subsystem | |
std::shared_ptr< ChTrackBrake > | m_brake |
sprocket brake | |
ChRoadWheelAssemblyList | m_suspensions |
road-wheel assemblies | |
ChRollerList | m_rollers |
roller subsystems | |
bool | m_roadwheel_as_cylinder |
bool | m_idler_as_cylinder |
bool | m_roller_as_cylinder |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
Constructor & Destructor Documentation
◆ ChTrackAssemblyBand()
|
inline |
- Parameters
-
[in] name name of the subsystem [in] side assembly on left/right vehicle side
Member Function Documentation
◆ CheckCircleCircle()
|
protected |
- Parameters
-
found Does an intersection point exist between the circle formed by StartingPoint and Radius with the current circle belt feature Point Intersection Point, if it exists between the circle formed by StartingPoint and Radius with the current circle belt feature Features Table with the tagent or arc information for the entire belt wrap FeatureIdx Current belt feature to check the intersection of StartingPoint Current Point on the belt wrap Radius Length of the current belt segment that needs to be placed on the belt wrap
◆ CheckCircleLine()
|
protected |
- Parameters
-
found Does an intersection point exist between the circle formed by StartingPoint and Radius with the current circle belt feature Point Intersection Point, if it exists between the circle formed by StartingPoint and Radius with the current circle belt feature Features Table with the tagent or arc information for the entire belt wrap FeatureIdx Current belt feature to check the intersection of StartingPoint Current Point on the belt wrap Radius Length of the current belt segment that needs to be placed on the belt wrap
◆ FindCircleTangentPoints()
|
protected |
- Parameters
-
Circle1Pos Center Position of Circle 1 Circle1Rad Radius of Circle 1 Circle2Pos Center Position of Circle 2 Circle2Rad Radius of Circle 2 Tan1Pnt1 Point on Circle 1 for the first calculated outside tangent Tan1Pnt2 Point on Circle 2 for the first calculated outside tangent Tan2Pnt1 Point on Circle 1 for the second calculated outside tangent Tan2Pnt2 Point on Circle 2 for the second calculated outside tangent
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/track_assembly/ChTrackAssemblyBand.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/track_assembly/ChTrackAssemblyBand.cpp