Description
Class which defines a contact surface for FEA elements, using a mesh of triangles.
Differently from ChContactSurfaceNodeCloud, this also captures the FEAnodes-vs-FEAfaces and FEAedge-vs-FEAedges cases, but it has a higher computational overhead
#include <ChContactSurfaceMesh.h>
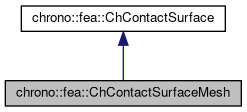
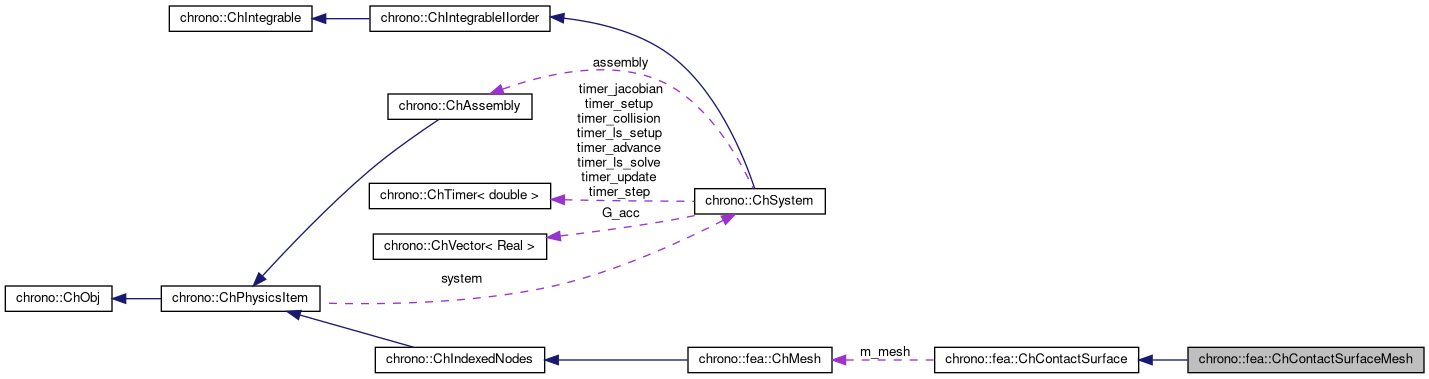
Public Member Functions | |
ChContactSurfaceMesh (std::shared_ptr< ChMaterialSurface > material, ChMesh *mesh=nullptr) | |
void | AddFacesFromBoundary (double sphere_swept=0.0, bool ccw=true) |
Given a FEA mesh (ex a mesh of tetrahedrons) it finds the faces on the outer boundary. More... | |
std::vector< std::shared_ptr< ChContactTriangleXYZ > > & | GetTriangleList () |
As AddFacesFromBoundary, but only for faces containing selected nodes in node_set. More... | |
std::vector< std::shared_ptr< ChContactTriangleXYZROT > > & | GetTriangleListRot () |
Get the list of triangles, for nodes with rotational dofs too. | |
unsigned int | GetNumTriangles () const |
Get the number of triangles. | |
unsigned int | GetNumVertices () const |
Get the number of vertices. | |
virtual void | SurfaceSyncCollisionModels () override |
Functions to interface this with ChPhysicsItem container. | |
virtual void | SurfaceAddCollisionModelsToSystem (ChSystem *msys) override |
virtual void | SurfaceRemoveCollisionModelsFromSystem (ChSystem *msys) override |
![]() | |
ChContactSurface (std::shared_ptr< ChMaterialSurface > material, ChMesh *mesh=nullptr) | |
ChMesh * | GetMesh () |
Get owner mesh. | |
void | SetMesh (ChMesh *mesh) |
Set owner mesh. | |
std::shared_ptr< ChMaterialSurface > & | GetMaterialSurface () |
Get the surface contact material. | |
Additional Inherited Members | |
![]() | |
std::shared_ptr< ChMaterialSurface > | m_material |
contact material properties | |
ChMesh * | m_mesh |
associated mesh | |
Member Function Documentation
◆ AddFacesFromBoundary()
void chrono::fea::ChContactSurfaceMesh::AddFacesFromBoundary | ( | double | sphere_swept = 0.0 , |
bool | ccw = true |
||
) |
Given a FEA mesh (ex a mesh of tetrahedrons) it finds the faces on the outer boundary.
That is, it scans all the finite elements already added in the parent ChMesh and adds the faces that are not shared (ie. the faces on the boundary 'skin'). For shells, the argument 'ccw' indicates whether the face vertices are provided in a counter-clockwise (default) or clockwise order, this has a reason: shells collisions are oriented and might work only from the "outer" side. Supported elements that generate boundary skin:
- solids:
- ChElementTetrahedron: all solid tetrahedrons
- ChElementHexahedron: all solid hexahedrons
- shells:
- ChElementShellANCF_3423 ANCF: shells (only one side)
- ChElementShellANCF_3443 ANCF: shells (only one side)
- ChElementShellANCF_3833 ANCF: shells (only one side)
- ChElementShellReissner: Reissner 4-nodes shells (only one side)
- beams:
- ChElementCableANCF: ANCF beams (as sphere-swept lines, i.e. sequence of capsules)
- ChElementBeamEuler: Euler-Bernoulli beams (as sphere-swept lines, i.e. sequence of capsules) More will follow in future.
- Parameters
-
sphere_swept radius of swept sphere ccw indicate clockwise or counterclockwise vertex ordering
◆ GetTriangleList()
|
inline |
As AddFacesFromBoundary, but only for faces containing selected nodes in node_set.
Get the list of triangles.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChContactSurfaceMesh.h
- /builds/uwsbel/chrono/src/chrono/fea/ChContactSurfaceMesh.cpp