Description
Implementation of the HHT implicit integrator for II order systems.
This timestepper allows use of an adaptive time-step, as well as optional use of a modified Newton scheme for the solution of the resulting nonlinear problem.
#include <ChTimestepperHHT.h>
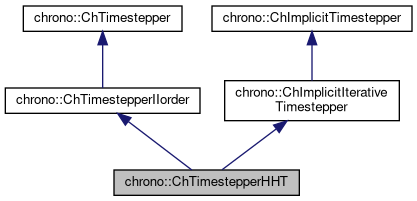
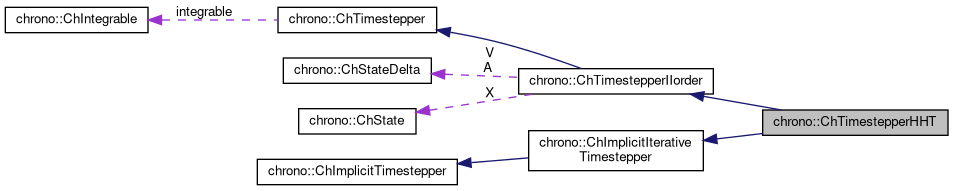
Public Types | |
enum | HHT_Mode { ACCELERATION, POSITION } |
![]() | |
enum | Type { EULER_IMPLICIT_LINEARIZED = 0, EULER_IMPLICIT_PROJECTED = 1, EULER_IMPLICIT = 2, TRAPEZOIDAL = 3, TRAPEZOIDAL_LINEARIZED = 4, HHT = 5, HEUN = 6, RUNGEKUTTA45 = 7, EULER_EXPLICIT = 8, LEAPFROG = 9, NEWMARK = 10, CUSTOM = 20 } |
Available methods for time integration (time steppers). | |
Public Member Functions | |
ChTimestepperHHT (ChIntegrableIIorder *intgr=nullptr) | |
virtual Type | GetType () const override |
Return type of the integration method. More... | |
void | SetAlpha (double malpha) |
Set the numerical damping parameter. More... | |
double | GetAlpha () |
Return the current value of the method parameter alpha. | |
void | SetMode (HHT_Mode mmode) |
Set the HHT formulation. | |
void | SetScaling (bool mscaling) |
Turn scaling on/off. | |
void | SetStepControl (bool val) |
Turn step size control on/off. More... | |
void | SetMinStepSize (double min_step) |
Set the minimum step size. More... | |
void | SetMaxItersSuccess (int iters) |
Set the maximum allowable number of iterations for counting a step towards a stepsize increase. | |
void | SetRequiredSuccessfulSteps (int num_steps) |
Set the minimum number of (internal) steps that require at most maxiters_success before considering a stepsize increase. | |
void | SetStepIncreaseFactor (double factor) |
Set the multiplicative factor for a stepsize increase. More... | |
void | SetStepDecreaseFactor (double factor) |
Set the multiplicative factor for a stepsize decrease. More... | |
void | SetModifiedNewton (bool val) |
Enable/disable modified Newton. More... | |
virtual void | Advance (const double dt) override |
Perform an integration timestep. More... | |
bool | GetConvergenceFlag () const |
Get an indicator to tell whether the iteration in current step tends to convergence or divergence. More... | |
void | SetThreshold_R (double mv) |
Set the threshold of norm of R, which is used to judge the trend of convergency. More... | |
double | GetThreshold_R () const |
Get the threshold of norm of R, which is used to judge the trend of convergency. | |
virtual void | ArchiveOUT (ChArchiveOut &archive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &archive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChTimestepperIIorder (ChIntegrableIIorder *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepperIIorder () |
Destructor. | |
virtual ChState & | get_X () |
Access the state, position part, at current time. | |
virtual ChStateDelta & | get_V () |
Access the state, speed part, at current time. | |
virtual ChStateDelta & | get_A () |
Access the acceleration, at current time. | |
virtual void | SetIntegrable (ChIntegrableIIorder *intgr) |
Set the integrable object. | |
![]() | |
ChTimestepper (ChIntegrable *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepper () |
Destructor. | |
virtual ChVectorDynamic & | get_L () |
Access the lagrangian multipliers, if any. | |
virtual void | SetIntegrable (ChIntegrable *intgr) |
Set the integrable object. | |
ChIntegrable * | GetIntegrable () |
Get the integrable object. | |
virtual double | GetTime () const |
Get the current time. | |
virtual void | SetTime (double mt) |
Set the current time. | |
void | SetVerbose (bool verb) |
Turn on/off logging of messages. | |
void | SetQcDoClamp (bool dc) |
Turn on/off clamping on the Qcterm. | |
void | SetQcClamping (double cl) |
Turn on/off clamping on the Qcterm. | |
![]() | |
void | SetMaxiters (int iters) |
Set the max number of iterations using the Newton Raphson procedure. | |
double | GetMaxiters () |
Get the max number of iterations using the Newton Raphson procedure. | |
void | SetRelTolerance (double rel_tol) |
Set the relative tolerance. More... | |
void | SetAbsTolerances (double abs_tolS, double abs_tolL) |
Set the absolute tolerances. More... | |
void | SetAbsTolerances (double abs_tol) |
Set the absolute tolerances. More... | |
int | GetNumIterations () const |
Return the number of iterations. | |
int | GetNumSetupCalls () const |
Return the number of calls to the solver's Setup function. | |
int | GetNumSolveCalls () const |
Return the number of calls to the solver's Solve function. | |
Additional Inherited Members | |
![]() | |
ChState | X |
ChStateDelta | V |
ChStateDelta | A |
![]() | |
ChIntegrable * | integrable |
double | T |
ChVectorDynamic | L |
bool | verbose |
bool | Qc_do_clamp |
double | Qc_clamping |
![]() | |
int | maxiters |
maximum number of iterations | |
double | reltol |
relative tolerance | |
double | abstolS |
absolute tolerance (states) | |
double | abstolL |
absolute tolerance (Lagrange multipliers) | |
int | numiters |
number of iterations | |
int | numsetups |
number of calls to the solver's Setup function | |
int | numsolves |
number of calls to the solver's Solve function | |
Member Function Documentation
◆ Advance()
|
overridevirtual |
◆ GetConvergenceFlag()
|
inline |
Get an indicator to tell whether the iteration in current step tends to convergence or divergence.
This could be helpful if you want to fall back to iterate again via using more rigorous stepper settings, such as smaller fixed time stepper, turning off ModifiedNerton,etc, WHEN you are not satisfied by current iteration result.
◆ GetType()
|
inlineoverridevirtual |
Return type of the integration method.
Default is CUSTOM. Derived classes should override this function.
Reimplemented from chrono::ChTimestepper.
◆ SetAlpha()
void chrono::ChTimestepperHHT::SetAlpha | ( | double | malpha | ) |
Set the numerical damping parameter.
It must be in the [-1/3, 0] interval. The closer to -1/3, the more damping. The closer to 0, the less damping (for 0, it is the trapezoidal method). The method coefficients gamma and beta are set automatically, based on alpha.
◆ SetMinStepSize()
|
inline |
Set the minimum step size.
An exception is thrown if the internal step size decreases below this limit.
◆ SetModifiedNewton()
|
inline |
Enable/disable modified Newton.
If enabled, the Newton matrix is evaluated, assembled, and factorized only once per step or if the Newton iteration does not converge with an out-of-date matrix. If disabled, the Newton matrix is evaluated at every iteration of the nonlinear solver. Modified Newton iteration is enabled by default.
◆ SetStepControl()
|
inline |
Turn step size control on/off.
Step size control is enabled by default.
◆ SetStepDecreaseFactor()
|
inline |
Set the multiplicative factor for a stepsize decrease.
Must be a value smaller than 1.
◆ SetStepIncreaseFactor()
|
inline |
Set the multiplicative factor for a stepsize increase.
Must be a value larger than 1.
◆ SetThreshold_R()
|
inline |
Set the threshold of norm of R, which is used to judge the trend of convergency.
For different systems, this threshold may be much different, such as a mini robot and a huge wind turbine. You could turn on 'verbose' of HHT stepper and look at the norm of R for your system firstly, and then set a suitable threshold of norm of R. This threshold should always be larger than the normal norm of R for your system.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepperHHT.h
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepperHHT.cpp