Description
Base class for sparse direct linear solvers.
Sparse linear direct solvers. Cannot handle VI and complementarity problems, so it cannot be used with NSC formulations.
This base class manages the setup and solution of sparse linear systems in Chrono and defers to derived classes for performing the actual matrix factorization and then computing the solution vector. See ChSolverMkl (which implements Eigen's interface to the Intel MKL Pardiso solver) and ChSolverMumps (which interfaces to the MUMPS solver).
ChDirectSolverLS manages the detection and update of the matrix sparsity pattern, providing two main features:
- sparsity pattern lock
- sparsity pattern learning
The sparsity pattern lock skips sparsity identification or reserving memory for nonzeros on all but the first call to Setup. This feature is intended for problems where the system matrix sparsity pattern does not change significantly from call to call.
See LockSparsityPattern();
The sparsity pattern learning feature acquires the sparsity pattern in advance, in order to speed up matrix assembly. Enabled by default, the sparsity matrix learner identifies the exact matrix sparsity pattern (without actually setting any nonzeros).
See UseSparsityPatternLearner();
A further option allows the user to provide an estimate for the matrix sparsity (a value in [0,1], with 0 corresponding to a fully dense matrix). This value is used if the sparsity pattern learner is disabled if/when required to reserve space for matrix indices and nonzeros. See SetSparsityEstimate();
See ChSystemDescriptor for more information about the problem formulation and the data structures passed to the solver.
#include <ChDirectSolverLS.h>
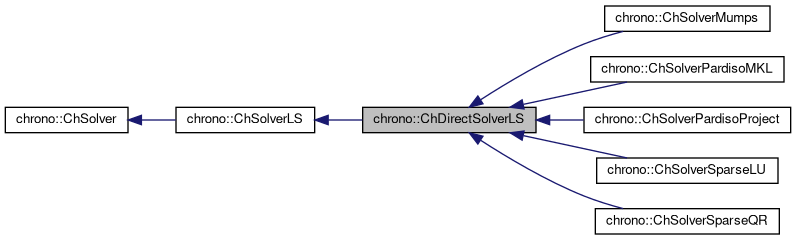
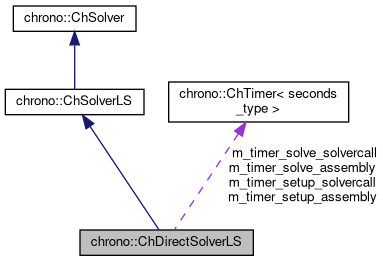
Public Types | |
enum | MatrixSymmetryType { MatrixSymmetryType::GENERAL, MatrixSymmetryType::SYMMETRIC_POSDEF, MatrixSymmetryType::SYMMETRIC_INDEF, MatrixSymmetryType::STRUCTURAL_SYMMETRIC } |
![]() | |
enum | Type { Type::PSOR = 0, Type::PSSOR, Type::PJACOBI, Type::PMINRES, Type::BARZILAIBORWEIN, Type::APGD, Type::ADDM, Type::SPARSE_LU, Type::SPARSE_QR, Type::PARDISO_MKL, Type::PARDISO_PROJECT, Type::MUMPS, Type::GMRES, Type::MINRES, Type::BICGSTAB, CUSTOM } |
Available types of solvers. More... | |
Public Member Functions | |
void | LockSparsityPattern (bool val) |
Enable/disable locking the sparsity pattern (default: false). More... | |
void | UseSparsityPatternLearner (bool val) |
Enable/disable use of the sparsity pattern learner (default: enabled). More... | |
void | ForceSparsityPatternUpdate () |
Force a call to the sparsity pattern learner to update sparsity pattern on the underlying matrix. More... | |
void | SetSparsityEstimate (double sparsity) |
Set estimate for matrix sparsity, a value in [0,1], with 0 indicating a fully dense matrix (default: 0.9). More... | |
virtual void | SetMatrixSymmetryType (MatrixSymmetryType symmetry) |
Set the matrix symmetry type (default: GENERAL). | |
void | UsePermutationVector (bool val) |
Enable/disable use of permutation vector (default: false). More... | |
void | LeverageRhsSparsity (bool val) |
Enable/disable leveraging sparsity in right-hand side vector (default: false). More... | |
virtual void | EnableNullPivotDetection (bool val, double threshold=0) |
Enable detection of null pivots. More... | |
void | ResetTimers () |
Reset timers for internal phases in Solve and Setup. | |
double | GetTimeSolve_Assembly () const |
Get cumulative time for assembly operations in Solve phase. | |
double | GetTimeSolve_SolverCall () const |
Get cumulative time for Pardiso calls in Solve phase. | |
double | GetTimeSetup_Assembly () const |
Get cumulative time for assembly operations in Setup phase. | |
double | GetTimeSetup_SolverCall () const |
Get cumulative time for Pardiso calls in Setup phase. | |
int | GetNumSetupCalls () const |
Return the number of calls to the solver's Setup function. | |
int | GetNumSolveCalls () const |
Return the number of calls to the solver's Setup function. | |
ChSparseMatrix & | GetMatrix () |
Get a handle to the underlying matrix. | |
ChSparseMatrix & | A () |
Get shortcut handle to underlying A matrix, for A*x=b. | |
ChVectorDynamic< double > & | x () |
Get shortcut handle to underlying x solution vector, for A*x=b. | |
ChVectorDynamic< double > & | b () |
Get shortcut handle to underlying b right hand-side known vector, for A*x=b. | |
virtual bool | Setup (ChSystemDescriptor &sysd) override |
Perform the solver setup operations. More... | |
virtual double | Solve (ChSystemDescriptor &sysd) override |
Solve linear system. More... | |
virtual bool | SetupCurrent () |
Generic setup-solve without passing through the ChSystemDescriptor, in cases where a sparse matrix has been already assembled. More... | |
virtual double | SolveCurrent () |
Generic setup-solve without passing through the ChSystemDescriptor, in cases where the a sparse matrix has been already assembled. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de serialization of transient data from archives. | |
![]() | |
virtual Type | GetType () const |
Return type of the solver. | |
void | SetVerbose (bool mv) |
Set verbose output from solver. | |
Protected Member Functions | |
virtual bool | FactorizeMatrix ()=0 |
Factorize the current sparse matrix and return true if successful. | |
virtual bool | SolveSystem ()=0 |
Solve the linear system using the current factorization and right-hand side vector. More... | |
virtual void | PrintErrorMessage ()=0 |
Display an error message corresponding to the last failure. More... | |
virtual bool | SolveRequiresMatrix () const override |
Indicate whether or not the Solve() phase requires an up-to-date problem matrix. More... | |
Protected Attributes | |
ChSparseMatrix | m_mat |
problem matrix | |
int | m_dim |
problem size | |
MatrixSymmetryType | m_symmetry |
symmetry of problem matrix | |
double | m_sparsity |
user-supplied estimate of matrix sparsity | |
ChVectorDynamic< double > | m_rhs |
right-hand side vector | |
ChVectorDynamic< double > | m_sol |
solution vector | |
int | m_solve_call |
counter for calls to Solve | |
int | m_setup_call |
counter for calls to Setup | |
bool | m_lock |
is the matrix sparsity pattern locked? | |
bool | m_use_learner |
use the sparsity pattern learner? | |
bool | m_force_update |
force a call to the sparsity pattern learner? | |
bool | m_use_perm |
use of the permutation vector? | |
bool | m_use_rhs_sparsity |
leverage right-hand side sparsity? | |
bool | m_null_pivot_detection |
enable detection of zero pivots? | |
ChTimer | m_timer_setup_assembly |
timer for matrix assembly | |
ChTimer | m_timer_setup_solvercall |
timer for factorization | |
ChTimer | m_timer_solve_assembly |
timer for RHS assembly | |
ChTimer | m_timer_solve_solvercall |
timer for solution | |
![]() | |
bool | verbose |
Member Enumeration Documentation
◆ MatrixSymmetryType
|
strong |
Member Function Documentation
◆ EnableNullPivotDetection()
|
inlinevirtual |
Enable detection of null pivots.
A concrete direct sparse solver may or may not support this feature.
Reimplemented in chrono::ChSolverMumps.
◆ ForceSparsityPatternUpdate()
|
inline |
Force a call to the sparsity pattern learner to update sparsity pattern on the underlying matrix.
Such a call may be needed in a situation where the sparsity pattern is locked, but a change in the problem size or structure occurred. This function has no effect if the sparsity pattern learner is disabled.
◆ LeverageRhsSparsity()
|
inline |
Enable/disable leveraging sparsity in right-hand side vector (default: false).
A concrete direct sparse solver may or may not support this feature.
◆ LockSparsityPattern()
|
inline |
Enable/disable locking the sparsity pattern (default: false).
If enabled, the sparsity pattern of the problem matrix is assumed to be unchanged from call to call. Enable this option whenever possible to improve performance.
◆ PrintErrorMessage()
|
protectedpure virtual |
Display an error message corresponding to the last failure.
This function is only called if Factorize or Solve returned false.
◆ SetSparsityEstimate()
|
inline |
Set estimate for matrix sparsity, a value in [0,1], with 0 indicating a fully dense matrix (default: 0.9).
Only used if the sparsity pattern learner is disabled.
◆ Setup()
|
overridevirtual |
Perform the solver setup operations.
Here, sysd is the system description with constraints and variables. Returns true if successful and false otherwise.
Reimplemented from chrono::ChSolver.
Reimplemented in chrono::ChSolverPardisoProject.
◆ SetupCurrent()
|
virtual |
Generic setup-solve without passing through the ChSystemDescriptor, in cases where a sparse matrix has been already assembled.
Performs the solver setup operations, assuming someone has already filled A() matrix before calling this.
◆ Solve()
|
overridevirtual |
Solve linear system.
Here, sysd is the system description with constraints and variables.
Implements chrono::ChSolver.
◆ SolveCurrent()
|
virtual |
Generic setup-solve without passing through the ChSystemDescriptor, in cases where the a sparse matrix has been already assembled.
Here, sysd is the system description with constraints and variables. Performs the solver setup operations, assuming someone has already filled the b() vector before calling this. Call x() afterward to get results.
◆ SolveRequiresMatrix()
|
inlineoverrideprotectedvirtual |
Indicate whether or not the Solve() phase requires an up-to-date problem matrix.
Typically, direct solvers only require the matrix for their Setup() phase.
Implements chrono::ChSolver.
◆ SolveSystem()
|
protectedpure virtual |
Solve the linear system using the current factorization and right-hand side vector.
Load the solution vector (already of appropriate size) and return true if succesful.
◆ UsePermutationVector()
|
inline |
Enable/disable use of permutation vector (default: false).
A concrete direct sparse solver may or may not support this feature.
◆ UseSparsityPatternLearner()
|
inline |
Enable/disable use of the sparsity pattern learner (default: enabled).
Disable for smaller problems where the overhead may be too large.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChDirectSolverLS.h
- /builds/uwsbel/chrono/src/chrono/solver/ChDirectSolverLS.cpp