chrono::vehicle::feda::FEDA_PitmanArm Class Reference
Description
Pitman-arm steering subsystem for the FEDA vehicle.
#include <FEDA_PitmanArm.h>
Inheritance diagram for chrono::vehicle::feda::FEDA_PitmanArm:
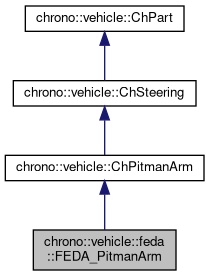
Collaboration diagram for chrono::vehicle::feda::FEDA_PitmanArm:
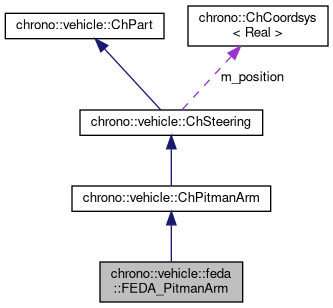
Public Member Functions | |
FEDA_PitmanArm (const std::string &name) | |
virtual double | getSteeringLinkMass () const override |
Return the mass of the steering link body. | |
virtual double | getPitmanArmMass () const override |
Return the mass of the Pitman arm body. | |
virtual double | getSteeringLinkRadius () const override |
Return the radius of the steering link body (visualization only). | |
virtual double | getPitmanArmRadius () const override |
Return the radius of the Pitman arm body (visualization only). | |
virtual const ChVector & | getSteeringLinkInertiaMoments () const override |
Return the moments of inertia of the steering link body. | |
virtual const ChVector & | getSteeringLinkInertiaProducts () const override |
Return the products of inertia of the steering link body. | |
virtual const ChVector & | getPitmanArmInertiaMoments () const override |
Return the moments of inertia of the Pitman arm body. | |
virtual const ChVector & | getPitmanArmInertiaProducts () const override |
Return the products of inertia of the Pitman arm body. | |
virtual double | getMaxAngle () const override |
Return the maximum rotation angle of the revolute joint. | |
virtual const ChVector | getLocation (PointId which) override |
Return the location of the specified hardpoint. More... | |
virtual const ChVector | getDirection (DirectionId which) override |
Return the unit vector for the specified direction. More... | |
![]() | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector<> &location, const ChQuaternion<> &rotation) override |
Initialize this steering subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the steering subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the steering subsystem. | |
virtual void | Synchronize (double time, double steering) override |
Update the state of this steering subsystem at the current time. More... | |
virtual double | GetMass () const override |
Get the total mass of the steering subsystem. | |
virtual ChVector | GetCOMPos () const override |
Get the current global COM location of the steering subsystem. | |
virtual void | LogConstraintViolations () override |
Log current constraint violations. | |
![]() | |
ChSteering (const std::string &name) | |
const ChCoordsys & | GetPosition () const |
Get the position (location and orientation) of the steering subsystem relative to the chassis reference frame. | |
std::shared_ptr< ChBody > | GetSteeringLink () const |
Get a handle to the main link of the steering subsystems. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
enum | PointId { STEERINGLINK, PITMANARM, REV, UNIV, REVSPH_R, REVSPH_S, TIEROD_PA, TIEROD_IA, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
enum | DirectionId { REV_AXIS, UNIV_AXIS_ARM, UNIV_AXIS_LINK, REVSPH_AXIS, NUM_DIRS } |
Identifiers for the various direction unit vectors. More... | |
![]() | |
ChPitmanArm (const std::string &name, bool vehicle_frame_inertia=false) | |
Protected constructor. More... | |
void | SetVehicleFrameInertiaFlag (bool val) |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false). More... | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
std::shared_ptr< ChBody > | m_arm |
handle to the Pitman arm body | |
std::shared_ptr< ChLinkMotorRotationAngle > | m_revolute |
handle to the chassis-arm revolute joint | |
std::shared_ptr< ChLinkRevoluteSpherical > | m_revsph |
handle to the revolute-spherical joint (idler arm) | |
std::shared_ptr< ChLinkUniversal > | m_universal |
handle to the arm-link universal joint | |
![]() | |
ChCoordsys | m_position |
location and orientation relative to chassis | |
std::shared_ptr< ChBody > | m_link |
handle to the main steering link | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
Member Function Documentation
◆ getDirection()
|
overridevirtual |
Return the unit vector for the specified direction.
The returned vector must be expressed in the suspension reference frame.
Implements chrono::vehicle::ChPitmanArm.
◆ getLocation()
Return the location of the specified hardpoint.
The returned location must be expressed in the suspension reference frame.
Implements chrono::vehicle::ChPitmanArm.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/feda/FEDA_PitmanArm.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/feda/FEDA_PitmanArm.cpp