Description
Base class for a suspension test rig.
#include <ChSuspensionTestRig.h>

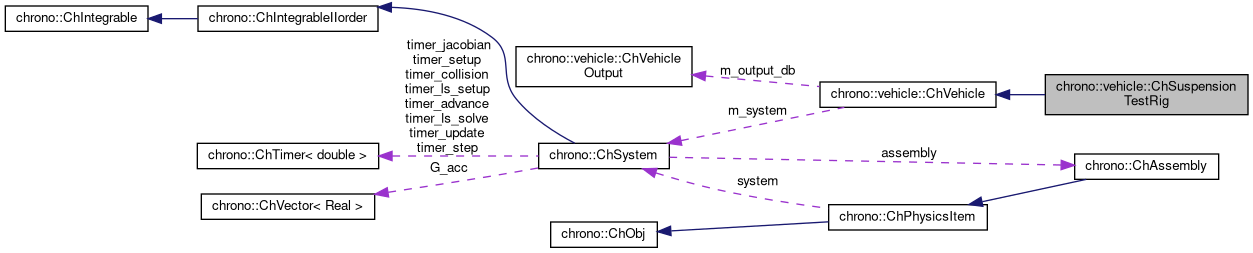
Public Member Functions | |
virtual | ~ChSuspensionTestRig () |
Destructor. | |
void | SetDriver (std::shared_ptr< ChDriverSTR > driver) |
Set driver system. | |
void | SetInitialRideHeight (double height) |
Set the initial ride height (relative to the chassis reference frame). More... | |
void | SetDisplacementLimit (double limit) |
Set the limits for post displacement (same for jounce and rebound). | |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystem (default: PRIMITIVES). | |
void | SetSteeringVisualizationType (VisualizationType vis) |
Set visualization type for the steering subsystems (default: PRIMITIVES). | |
void | SetWheelVisualizationType (VisualizationType vis) |
Set visualization type for the wheel subsystems (default: NONE). | |
void | SetTireVisualizationType (VisualizationType vis) |
Set visualization type for the tire subsystems (default: PRIMITIVES). | |
void | SetSuspensionOutput (bool state) |
Enable/disable output from the suspension subsystem. More... | |
void | SetSteeringOutput (bool state) |
Enable/disable output from the steering subsystem (if one exists). More... | |
void | SetAntirollbarOutput (bool state) |
Enable/disable output from the anti-roll bar subsystem (if one exists). More... | |
void | Initialize () |
Initialize this suspension test rig. | |
virtual void | Advance (double step) override |
Advance the state of the suspension test rig by the specified time step. | |
const ChVector & | GetSpindlePos (VehicleSide side) const |
Get the global location of the specified spindle. | |
ChQuaternion | GetSpindleRot (VehicleSide side) const |
Get the global rotation of the specified spindle. | |
const ChVector & | GetSpindleLinVel (VehicleSide side) const |
Get the linear velocity of the specified spindle (expressed in the global reference frame). | |
ChVector | GetSpindleAngVel (VehicleSide side) const |
Get the angular velocity of the specified spindle (expressed in the global reference frame). | |
double | GetSteeringInput () const |
double | GetLeftInput () const |
double | GetRightInput () const |
virtual double | GetActuatorDisp (VehicleSide side)=0 |
virtual double | GetActuatorForce (VehicleSide side)=0 |
bool | HasSteering () const |
Return true if a steering system is attached. | |
bool | HasAntirollbar () const |
Return true if an anti-roll bar system is attached. | |
std::shared_ptr< ChSuspension > | GetSuspension () const |
Get the suspension subsystem. | |
std::shared_ptr< ChSteering > | GetSteering () const |
Get the steering subsystem. | |
std::shared_ptr< ChAntirollBar > | GetAntirollBar () const |
Get the anti-roll bar subsystem. | |
std::shared_ptr< ChWheel > | GetWheel (VehicleSide side) const |
Get a handle to the specified wheel subsystem. | |
double | GetMass () const |
Get the rig total mass. More... | |
TerrainForce | ReportTireForce (VehicleSide side) const |
Get the tire force and moment on the specified side. | |
ChSuspension::Force | ReportSuspensionForce (VehicleSide side) const |
Get the suspension forces on the specified side. | |
double | GetWheelTravel (VehicleSide side) const |
Get wheel travel, defined here as the vertical displacement of the spindle center from its position at the initial specified ride height (or initial configuration, if an initial ride height is not specified). | |
virtual double | GetRideHeight () const =0 |
Get current ride height (relative to the chassis reference frame). More... | |
virtual void | LogConstraintViolations () override |
Log current constraint violations. | |
void | SetPlotOutput (double output_step) |
Enable/disable data collection for plot generation (default: false). More... | |
virtual void | PlotOutput (const std::string &out_dir, const std::string &out_name)=0 |
Plot collected data. More... | |
![]() | |
virtual | ~ChVehicle () |
Destructor. | |
const std::string & | GetName () const |
Get the name identifier for this vehicle. | |
void | SetName (const std::string &name) |
Set the name identifier for this vehicle. | |
ChSystem * | GetSystem () |
Get a pointer to the Chrono ChSystem. | |
double | GetChTime () const |
Get the current simulation time of the underlying ChSystem. | |
std::shared_ptr< ChChassis > | GetChassis () const |
Get a handle to the vehicle's main chassis subsystem. | |
std::shared_ptr< ChChassisRear > | GetChassisRear (int id) const |
Get the specified specified rear chassis subsystem. | |
std::shared_ptr< ChChassisConnector > | GetChassisConnector (int id) const |
Get a handle to the specified chassis connector. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisBody () const |
Get a handle to the vehicle's chassis body. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisRearBody (int id) const |
Get a handle to the specified rear chassis body. | |
virtual std::shared_ptr< ChPowertrain > | GetPowertrain () const |
Get the powertrain attached to this vehicle. | |
const ChVector & | GetVehiclePos () const |
Get the vehicle location. More... | |
ChQuaternion | GetVehicleRot () const |
Get the vehicle orientation. More... | |
double | GetVehicleSpeed () const |
Get the vehicle speed. More... | |
double | GetVehicleSpeedCOM () const |
Get the speed of the chassis COM. More... | |
ChVector | GetVehiclePointLocation (const ChVector<> &locpos) const |
Get the global position of the specified point. More... | |
ChVector | GetVehiclePointVelocity (const ChVector<> &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector | GetVehiclePointAcceleration (const ChVector<> &locpos) const |
Get the acceleration at the specified point. More... | |
ChVector | GetDriverPos () const |
Get the global location of the driver. | |
void | SetOutput (ChVehicleOutput::Type type, const std::string &out_dir, const std::string &out_name, double output_step) |
Enable output for this vehicle system. More... | |
void | SetChassisVisualizationType (VisualizationType vis) |
Set visualization mode for the chassis subsystem. | |
void | SetChassisRearVisualizationType (VisualizationType vis) |
Set visualization mode for the rear chassis subsystems. | |
void | SetChassisCollide (bool state) |
Enable/disable collision for the chassis subsystem. More... | |
virtual void | SetChassisVehicleCollide (bool state) |
Enable/disable collision between the chassis and all other vehicle subsystems. More... | |
void | SetChassisOutput (bool state) |
Enable/disable output from the chassis subsystem. | |
Protected Member Functions | |
ChSuspensionTestRig (ChWheeledVehicle &vehicle, int axle_index, int steering_index, double displ_limit, std::shared_ptr< ChTire > tire_left, std::shared_ptr< ChTire > tire_right, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a test rig for a specified axle of a given vehicle. More... | |
ChSuspensionTestRig (const std::string &filename, int axle_index, double displ_limit, std::shared_ptr< ChTire > tire_left, std::shared_ptr< ChTire > tire_right, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a test rig for a specified axle of a given vehicle. More... | |
ChSuspensionTestRig (const std::string &filename, std::shared_ptr< ChTire > tire_left, std::shared_ptr< ChTire > tire_right, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a test rig from specified (JSON) file. More... | |
void | InitializeSubsystems () |
Initialize all underlying vehicle subsystems. | |
virtual void | Output (int frame, ChVehicleOutput &database) const override |
Output data for all modeling components in the suspension test rig system. | |
virtual double | CalcDisplacementOffset ()=0 |
virtual double | CalcTerrainHeight (VehicleSide side)=0 |
virtual void | UpdateActuators (double displ_left, double displ_speed_left, double displ_right, double displ_speed_right)=0 |
virtual void | CollectPlotData (double time)=0 |
![]() | |
ChVehicle (const std::string &name, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a vehicle system with an underlying ChSystem. More... | |
ChVehicle (const std::string &name, ChSystem *system) | |
Construct a vehicle system using the specified ChSystem. More... | |
Protected Attributes | |
std::shared_ptr< ChSuspension > | m_suspension |
suspension subsystem | |
std::shared_ptr< ChSteering > | m_steering |
steering subsystem | |
std::shared_ptr< ChAntirollBar > | m_antirollbar |
anti-roll bar subsystem | |
std::shared_ptr< ChShaft > | m_dummy_shaft |
dummy driveshaft | |
std::shared_ptr< ChWheel > | m_wheel [2] |
wheel subsystems | |
std::shared_ptr< ChTire > | m_tire [2] |
tire subsystems | |
double | m_ride_height |
ride height | |
double | m_displ_offset |
post displacement offset (to set reference position) | |
double | m_displ_delay |
time interval for assuming reference position | |
double | m_displ_limit |
scale factor for post displacement | |
bool | m_plot_output |
double | m_plot_output_step |
double | m_next_plot_output_time |
utils::CSV_writer * | m_csv |
![]() | |
std::string | m_name |
vehicle name | |
ChSystem * | m_system |
pointer to the Chrono system | |
bool | m_ownsSystem |
true if system created at construction | |
bool | m_output |
generate ouput for this vehicle system | |
ChVehicleOutput * | m_output_db |
vehicle output database | |
double | m_output_step |
output time step | |
double | m_next_output_time |
time for next output | |
int | m_output_frame |
current output frame | |
std::shared_ptr< ChChassis > | m_chassis |
handle to the main chassis subsystem | |
ChChassisRearList | m_chassis_rear |
list of rear chassis subsystems (can be empty) | |
ChChassisConnectorList | m_chassis_connectors |
list of chassis connector (must match m_chassis_rear) | |
Additional Inherited Members | |
![]() | |
template<typename T > | |
static bool | AnyOutput (const std::vector< std::shared_ptr< T >> &list) |
Utility function for testing if any subsystem in a list generates output. | |
Constructor & Destructor Documentation
◆ ChSuspensionTestRig() [1/3]
|
protected |
Construct a test rig for a specified axle of a given vehicle.
This version uses a concrete vehicle object.
- Parameters
-
vehicle vehicle source axle_index index of the suspension to be tested steering_index index of associated steering subsystem (-1 for no steering) displ_limit limits for post displacement tire_left left tire tire_right right tire contact_method contact method
◆ ChSuspensionTestRig() [2/3]
|
protected |
Construct a test rig for a specified axle of a given vehicle.
This version assumes the vehicle is specified through a JSON file.
- Parameters
-
filename JSON file with vehicle specification axle_index index of the suspension to be tested displ_limit limits for post displacement tire_left left tire tire_right right tire contact_method contact method
◆ ChSuspensionTestRig() [3/3]
|
protected |
Construct a test rig from specified (JSON) file.
- Parameters
-
filename JSON file with test rig specification tire_left left tire tire_right right tire contact_method contact method
Member Function Documentation
◆ GetMass()
double chrono::vehicle::ChSuspensionTestRig::GetMass | ( | ) | const |
Get the rig total mass.
This includes the mass of the suspension and wheels, and (if present) the mass of the steering mechanism.
◆ GetRideHeight()
|
pure virtual |
Get current ride height (relative to the chassis reference frame).
This estimate uses the average of the left and right posts.
Implemented in chrono::vehicle::ChSuspensionTestRigPushrod, and chrono::vehicle::ChSuspensionTestRigPlatform.
◆ PlotOutput()
|
pure virtual |
Plot collected data.
When called (typically at the end of the simulation loop), the collected data is saved in ASCII format in a file with the specified name (and 'txt' extension) in the specified output directory. If the Chrono::Postprocess module is available (with gnuplot support), selected plots are generated.
Implemented in chrono::vehicle::ChSuspensionTestRigPushrod, and chrono::vehicle::ChSuspensionTestRigPlatform.
◆ SetAntirollbarOutput()
void chrono::vehicle::ChSuspensionTestRig::SetAntirollbarOutput | ( | bool | state | ) |
Enable/disable output from the anti-roll bar subsystem (if one exists).
See also ChVehicle::SetOuput.
◆ SetInitialRideHeight()
|
inline |
Set the initial ride height (relative to the chassis reference frame).
If not specified, the reference height is the suspension design configuration.
◆ SetPlotOutput()
void chrono::vehicle::ChSuspensionTestRig::SetPlotOutput | ( | double | output_step | ) |
Enable/disable data collection for plot generation (default: false).
See ChSuspensionTestRigPlatform::plotOutput and ChSuspensionTestRigPushrod::plotOutput for details on data collected for a specific type of test rig.
◆ SetSteeringOutput()
void chrono::vehicle::ChSuspensionTestRig::SetSteeringOutput | ( | bool | state | ) |
Enable/disable output from the steering subsystem (if one exists).
See also ChVehicle::SetOuput.
◆ SetSuspensionOutput()
void chrono::vehicle::ChSuspensionTestRig::SetSuspensionOutput | ( | bool | state | ) |
Enable/disable output from the suspension subsystem.
See also ChVehicle::SetOuput.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRig.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRig.cpp