Description
This is a specialized type of socket: the TCP socket.
It can be used to talk with third party applications because it is frequent that other simulators (ex. Simulink) support the TCP socket communication.
#include <ChSocket.h>
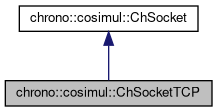
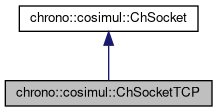
Public Member Functions | |
ChSocketTCP (int portId) | |
Constructor. Used to create a new TCP socket given a port. | |
int | sendMessage (std::string &) |
Send a std::string to the connected host. More... | |
int | receiveMessage (std::string &) |
Receive a std::string from the connected host. More... | |
int | SendBuffer (std::vector< char > &source_buf) |
Send a std::vector<char> (a buffer of bytes) to the connected host, without the header as in SendMessage (so the receiver must know in advance the length of the buffer). More... | |
int | ReceiveBuffer (std::vector< char > &dest_buf, int bsize) |
Receive a std::vector<char> (a buffer of bytes) from the connected host, without the header as in SendMessage (so one must know in advance the length of the buffer). More... | |
void | bindSocket () |
Binds the socket to an address and port number (a server call) | |
ChSocketTCP * | acceptClient (std::string &) |
Accepts a connecting client. More... | |
void | listenToClient (int numPorts=5) |
Listens to connecting clients, (a server call) | |
virtual void | connectToServer (std::string &, hostType) |
Connects to the server, a client call. | |
![]() | |
ChSocket (int) | |
void | setDebug (int) |
void | setReuseAddr (int) |
void | setKeepAlive (int) |
void | setLingerOnOff (bool) |
void | setLingerSeconds (int) |
void | setSocketBlocking (int) |
void | setSendBufSize (int) |
void | setReceiveBufSize (int) |
int | getDebug () |
int | getReuseAddr () |
int | getKeepAlive () |
int | getSendBufSize () |
int | getReceiveBufSize () |
int | getSocketBlocking () |
int | getLingerSeconds () |
bool | getLingerOnOff () |
int | getSocketId () |
int | getPortNumber () |
Additional Inherited Members | |
![]() | |
void | setSocketId (int socketFd) |
![]() | |
int | portNumber |
int | socketId |
int | blocking |
int | bindFlag |
struct sockaddr_in | clientAddr |
Member Function Documentation
◆ acceptClient()
ChSocketTCP * chrono::cosimul::ChSocketTCP::acceptClient | ( | std::string & | clientHost | ) |
Accepts a connecting client.
The address of the connected client is stored in the parameter (a server call)
◆ ReceiveBuffer()
int chrono::cosimul::ChSocketTCP::ReceiveBuffer | ( | std::vector< char > & | dest_buf, |
int | bsize | ||
) |
Receive a std::vector<char> (a buffer of bytes) from the connected host, without the header as in SendMessage (so one must know in advance the length of the buffer).
If the receiving buffer size is not =bsize, it will be resized before receiving.
- Parameters
-
dest_buf destination buffer - will be resized bsize size in bytes of expected received buffer.
◆ receiveMessage()
int chrono::cosimul::ChSocketTCP::receiveMessage | ( | std::string & | message | ) |
Receive a std::string from the connected host.
Note that the string size is handled automatically because there is an headed that tells the length of the string in bytes.
◆ SendBuffer()
int chrono::cosimul::ChSocketTCP::SendBuffer | ( | std::vector< char > & | source_buf | ) |
Send a std::vector<char> (a buffer of bytes) to the connected host, without the header as in SendMessage (so the receiver must know in advance the length of the buffer).
- Parameters
-
source_buf source buffer
◆ sendMessage()
int chrono::cosimul::ChSocketTCP::sendMessage | ( | std::string & | message | ) |
Send a std::string to the connected host.
Note that the string size is handled automatically because there is an headed that tells the length of the string in bytes.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_cosimulation/ChSocket.h
- /builds/uwsbel/chrono/src/chrono_cosimulation/ChSocket.cpp