chrono::ChSolverSparseLU Class Reference
Description
Sparse LU direct solver.
Interface to Eigen's SparseLU solver, a supernodal LU factorization for general matrices.
Cannot handle VI and complementarity problems, so it cannot be used with NSC formulations.
See ChDirectSolverLS for more details.
#include <ChDirectSolverLS.h>
Inheritance diagram for chrono::ChSolverSparseLU:
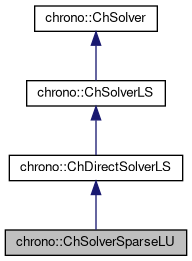
Collaboration diagram for chrono::ChSolverSparseLU:
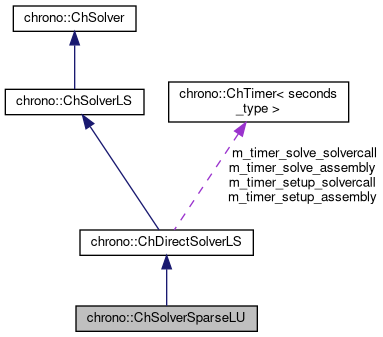
Public Member Functions | |
virtual Type | GetType () const override |
Return type of the solver. | |
![]() | |
void | LockSparsityPattern (bool val) |
Enable/disable locking the sparsity pattern (default: false). More... | |
void | UseSparsityPatternLearner (bool val) |
Enable/disable use of the sparsity pattern learner (default: enabled). More... | |
void | ForceSparsityPatternUpdate () |
Force a call to the sparsity pattern learner to update sparsity pattern on the underlying matrix. More... | |
void | SetSparsityEstimate (double sparsity) |
Set estimate for matrix sparsity, a value in [0,1], with 0 indicating a fully dense matrix (default: 0.9). More... | |
virtual void | SetMatrixSymmetryType (MatrixSymmetryType symmetry) |
Set the matrix symmetry type (default: GENERAL). | |
void | UsePermutationVector (bool val) |
Enable/disable use of permutation vector (default: false). More... | |
void | LeverageRhsSparsity (bool val) |
Enable/disable leveraging sparsity in right-hand side vector (default: false). More... | |
virtual void | EnableNullPivotDetection (bool val, double threshold=0) |
Enable detection of null pivots. More... | |
void | ResetTimers () |
Reset timers for internal phases in Solve and Setup. | |
double | GetTimeSolve_Assembly () const |
Get cumulative time for assembly operations in Solve phase. | |
double | GetTimeSolve_SolverCall () const |
Get cumulative time for Pardiso calls in Solve phase. | |
double | GetTimeSetup_Assembly () const |
Get cumulative time for assembly operations in Setup phase. | |
double | GetTimeSetup_SolverCall () const |
Get cumulative time for Pardiso calls in Setup phase. | |
int | GetNumSetupCalls () const |
Return the number of calls to the solver's Setup function. | |
int | GetNumSolveCalls () const |
Return the number of calls to the solver's Setup function. | |
ChSparseMatrix & | GetMatrix () |
Get a handle to the underlying matrix. | |
ChSparseMatrix & | A () |
Get shortcut handle to underlying A matrix, for A*x=b. | |
ChVectorDynamic< double > & | x () |
Get shortcut handle to underlying x solution vector, for A*x=b. | |
ChVectorDynamic< double > & | b () |
Get shortcut handle to underlying b right hand-side known vector, for A*x=b. | |
virtual bool | Setup (ChSystemDescriptor &sysd) override |
Perform the solver setup operations. More... | |
virtual double | Solve (ChSystemDescriptor &sysd) override |
Solve linear system. More... | |
virtual bool | SetupCurrent () |
Generic setup-solve without passing through the ChSystemDescriptor, in cases where a sparse matrix has been already assembled. More... | |
virtual double | SolveCurrent () |
Generic setup-solve without passing through the ChSystemDescriptor, in cases where the a sparse matrix has been already assembled. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de serialization of transient data from archives. | |
![]() | |
void | SetVerbose (bool mv) |
Set verbose output from solver. | |
Additional Inherited Members | |
![]() | |
enum | MatrixSymmetryType { MatrixSymmetryType::GENERAL, MatrixSymmetryType::SYMMETRIC_POSDEF, MatrixSymmetryType::SYMMETRIC_INDEF, MatrixSymmetryType::STRUCTURAL_SYMMETRIC } |
![]() | |
enum | Type { Type::PSOR = 0, Type::PSSOR, Type::PJACOBI, Type::PMINRES, Type::BARZILAIBORWEIN, Type::APGD, Type::ADDM, Type::SPARSE_LU, Type::SPARSE_QR, Type::PARDISO_MKL, Type::PARDISO_PROJECT, Type::MUMPS, Type::GMRES, Type::MINRES, Type::BICGSTAB, CUSTOM } |
Available types of solvers. More... | |
![]() | |
virtual bool | SolveRequiresMatrix () const override |
Indicate whether or not the Solve() phase requires an up-to-date problem matrix. More... | |
![]() | |
ChSparseMatrix | m_mat |
problem matrix | |
int | m_dim |
problem size | |
MatrixSymmetryType | m_symmetry |
symmetry of problem matrix | |
double | m_sparsity |
user-supplied estimate of matrix sparsity | |
ChVectorDynamic< double > | m_rhs |
right-hand side vector | |
ChVectorDynamic< double > | m_sol |
solution vector | |
int | m_solve_call |
counter for calls to Solve | |
int | m_setup_call |
counter for calls to Setup | |
bool | m_lock |
is the matrix sparsity pattern locked? | |
bool | m_use_learner |
use the sparsity pattern learner? | |
bool | m_force_update |
force a call to the sparsity pattern learner? | |
bool | m_use_perm |
use of the permutation vector? | |
bool | m_use_rhs_sparsity |
leverage right-hand side sparsity? | |
bool | m_null_pivot_detection |
enable detection of zero pivots? | |
ChTimer | m_timer_setup_assembly |
timer for matrix assembly | |
ChTimer | m_timer_setup_solvercall |
timer for factorization | |
ChTimer | m_timer_solve_assembly |
timer for RHS assembly | |
ChTimer | m_timer_solve_solvercall |
timer for solution | |
![]() | |
bool | verbose |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChDirectSolverLS.h
- /builds/uwsbel/chrono/src/chrono/solver/ChDirectSolverLS.cpp