Description
An iterative solver based on modified version of ADMM Alternating Direction Method of Multipliers.
See ChSystemDescriptor for more information about the problem formulation and the data structures passed to the solver.
#include <ChSolverADMM.h>
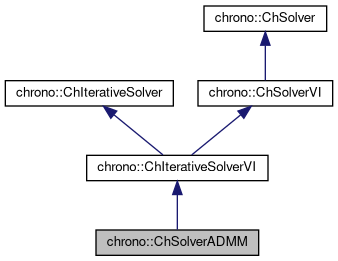
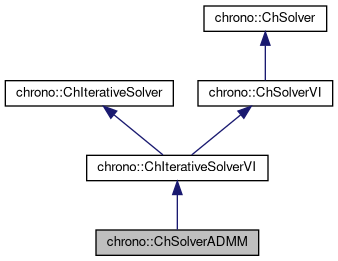
Public Types | |
enum | AdmmStepType { NONE = 0, BALANCED_UNSCALED, BALANCED_FAST, BALANCED_RANGE } |
Types of step adaption policies for the ADMM solver. | |
![]() | |
enum | Type { Type::PSOR = 0, Type::PSSOR, Type::PJACOBI, Type::PMINRES, Type::BARZILAIBORWEIN, Type::APGD, Type::ADDM, Type::SPARSE_LU, Type::SPARSE_QR, Type::PARDISO_MKL, Type::PARDISO_PROJECT, Type::MUMPS, Type::GMRES, Type::MINRES, Type::BICGSTAB, CUSTOM } |
Available types of solvers. More... | |
Public Member Functions | |
ChSolverADMM () | |
Default constructor: uses the SparseQR direct solver from Eigen, slow and non-optimal. More... | |
ChSolverADMM (std::shared_ptr< ChDirectSolverLS > my_LS_engine) | |
Constructor where you can pass a better direct solver than the default SparseQR solver. More... | |
virtual Type | GetType () const override |
Return type of the solver. | |
virtual double | Solve (ChSystemDescriptor &sysd) override |
Performs the solution of the problem. More... | |
void | SetDiagonalPreconditioner (bool mp) |
Enable the diagonal preconditioner. | |
bool | GetDiagonalPreconditioner () |
void | SetRho (double mr) |
Set the initial ADMM step. Could change later if adaptive step is used. | |
double | GetRho () |
void | SetRhoBilaterals (double mr) |
Set the ADMM step for bilateral constraints only. | |
double | GetRhoBilaterals () |
void | SetSigma (double mr) |
Set the sigma regularization. | |
double | GetSigma () |
void | SetStepAdjustEach (int mr) |
Set how frequently the rho step is adjusted. | |
int | GetStepAdjustEach () |
void | SetStepAdjustThreshold (double mr) |
Set the step adjust threshold T, ex. More... | |
double | GetStepAdjustThreshold () |
void | SetStepAdjustMaxfactor (double mr) |
Set the step adjust max factor F, if new step scaling is higher than F it is clamped to F, if lower than 1/F it is clamped to F, to avoid exploding results. | |
double | GetStepAdjustMaxfactor () |
void | SetStepAdjustPolicy (AdmmStepType mr) |
Set the step adjust max factor F, if new step scaling is higher than F it is clamped to F, if lower than 1/F it is clamped to F, to avoid exploding results. | |
AdmmStepType | GetStepAdjustPolicy () |
void | SetTolerancePrimal (double mr) |
Set the absolute tolerance for the primal residual (force impulses error), if the iteration falls below this and the dual tolerance, it stops iterating. | |
double | GetTolerancePrimal () |
void | SetToleranceDual (double mr) |
Set the absolute tolerance for the dual residual (constraint speed error), if the iteration falls below this and the primal tolerance, it stops iterating. | |
double | GetToleranceDual () |
double | GetErrorPrimal () const |
Return the primal residual (force impulses error) reached during the last solve. | |
double | GetErrorDual () const |
Return the dual residual (constraint speed error) reached during the last solve. | |
virtual double | GetError () const override |
Return the number of iterations performed during the last solve. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de serialization of transient data from archives. | |
![]() | |
void | SetOmega (double mval) |
Set the overrelaxation factor (default: 1.0). More... | |
void | SetSharpnessLambda (double mval) |
Set the sharpness factor (default: 1.0). More... | |
void | SetRecordViolation (bool mval) |
Enable/disable recording of the constraint violation history. More... | |
double | GetOmega () const |
Return the current value of the overrelaxation factor. | |
double | GetSharpnessLambda () const |
Return the current value of the sharpness factor. | |
virtual int | GetIterations () const override |
Return the number of iterations performed during the last solve. | |
const std::vector< double > & | GetViolationHistory () const |
Access the vector of constraint violation history. More... | |
const std::vector< double > & | GetDeltalambdaHistory () const |
Access the vector with history of maximum change in Lagrange multipliers Note that collection of constraint violations must be enabled through SetRecordViolation. | |
![]() | |
void | SetMaxIterations (int max_iterations) |
Set the maximum number of iterations. | |
void | SetTolerance (double tolerance) |
Set the tolerance threshold used by the stopping criteria. | |
void | EnableDiagonalPreconditioner (bool val) |
Enable/disable use of a simple diagonal preconditioner (default: true). More... | |
void | EnableWarmStart (bool val) |
Enable/disable warm starting by providing an initial guess (default: false). More... | |
int | GetMaxIterations () const |
Get the current maximum number of iterations. | |
double | GetTolerance () const |
Get the current tolerance value. | |
![]() | |
virtual bool | Setup (ChSystemDescriptor &sysd) |
This function does the setup operations for the solver. More... | |
void | SetVerbose (bool mv) |
Set verbose output from solver. | |
Additional Inherited Members | |
![]() | |
void | AtIterationEnd (double mmaxviolation, double mdeltalambda, unsigned int iternum) |
This method MUST be called by all iterative methods INSIDE their iteration loops (at the end). More... | |
virtual bool | SolveRequiresMatrix () const override |
Indicate whether ot not the Solve() phase requires an up-to-date problem matrix. More... | |
![]() | |
ChIterativeSolver (int max_iterations, double tolerance, bool use_precond, bool warm_start) | |
void | SaveMatrix (ChSystemDescriptor &sysd) |
double | CheckSolution (ChSystemDescriptor &sysd, const ChVectorDynamic<> &x) |
![]() | |
int | m_iterations |
total number of iterations performed by the solver | |
double | m_omega |
over-relaxation factor | |
double | m_shlambda |
sharpness factor | |
bool | record_violation_history |
std::vector< double > | violation_history |
std::vector< double > | dlambda_history |
![]() | |
bool | m_use_precond |
use diagonal preconditioning? | |
bool | m_warm_start |
use initial guesss? | |
int | m_max_iterations |
maximum number of iterations | |
double | m_tolerance |
tolerance threshold in stopping criteria | |
![]() | |
bool | verbose |
Constructor & Destructor Documentation
◆ ChSolverADMM() [1/2]
chrono::ChSolverADMM::ChSolverADMM | ( | ) |
Default constructor: uses the SparseQR direct solver from Eigen, slow and non-optimal.
Prefer the other constructor where you can pass a different directo solver, e.g. Pardiso.
◆ ChSolverADMM() [2/2]
chrono::ChSolverADMM::ChSolverADMM | ( | std::shared_ptr< ChDirectSolverLS > | my_LS_engine | ) |
Constructor where you can pass a better direct solver than the default SparseQR solver.
The custom direct solver will be used in the factorization and solves in the inner loop.
Member Function Documentation
◆ GetError()
|
inlineoverridevirtual |
Return the number of iterations performed during the last solve.
Return the tolerance error reached during the last solve (here refer to the ADMM dual residual)
Implements chrono::ChIterativeSolver.
◆ SetStepAdjustThreshold()
|
inline |
Set the step adjust threshold T, ex.
1.5; if new step scaling is in the interval [1/T, T], no adjustment is done anyway, to save CPU effort.
◆ Solve()
|
overridevirtual |
Performs the solution of the problem.
- Returns
- the maximum constraint violation after termination, as dual (speed) residual
- Parameters
-
sysd system description with constraints and variables
Implements chrono::ChSolver.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChSolverADMM.h
- /builds/uwsbel/chrono/src/chrono/solver/ChSolverADMM.cpp