chrono::ChOptimizerGenetic Class Reference
Description
Class for global optimization with the genetic method (evolutive simulation).
#include <ChSolvmin.h>
Inheritance diagram for chrono::ChOptimizerGenetic:
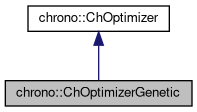
Collaboration diagram for chrono::ChOptimizerGenetic:
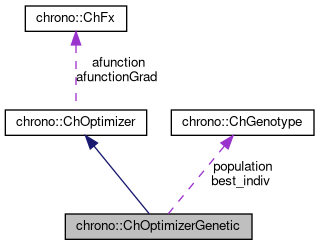
Public Member Functions | |
ChOptimizerGenetic (const ChOptimizerGenetic &other) | |
virtual bool | DoOptimize () override |
This function computes the optimal xv[]. More... | |
bool | CreatePopulation (ChGenotype **&my_population, int my_popsize) |
bool | DeletePopulation (ChGenotype **&my_population, int my_popsize) |
ChGenotype * | Select_roulette (ChGenotype **my_population) |
ChGenotype * | Select_best (ChGenotype **my_population) |
ChGenotype * | Select_worst (ChGenotype **my_population) |
double | Get_fitness_interval (ChGenotype **my_population) |
double | ComputeFitness (ChGenotype *) |
void | ApplyCrossover (ChGenotype *par1, ChGenotype *par2, ChGenotype &child1, ChGenotype &child2) |
bool | InitializePopulation () |
bool | ComputeAllFitness () |
void | Selection () |
void | Crossover () |
void | Mutation () |
void | PopulationStats (double &average, double &max, double &min, double &stdeviation) |
void | LogOut (bool filelog) |
![]() | |
ChOptimizer (const ChOptimizer &other) | |
virtual void | SetObjective (ChFx *mformula) |
Sets the objective function to maximize. | |
virtual ChFx * | GetObjective () const |
virtual void | SetObjectiveGrad (ChFx *mformula) |
Sets the objective function gradient (not mandatory, because if not set, the default bacward differentiation is used). | |
virtual ChFx * | GetObjectiveGrad () const |
virtual void | SetNumOfVars (int mv) |
Set the number of optimization variables. More... | |
virtual int | GetNumOfVars () const |
Returns the number of optimization variables. | |
double * | GetXv () const |
double * | GetXv_sup () const |
double * | GetXv_inf () const |
void | SetXv (double *mx) |
void | SetXv_sup (double *mx) |
void | SetXv_inf (double *mx) |
double | Eval_fx (double x[]) |
Returns the value of the functional, for given state of variables and with the given "database" multibody system. More... | |
double | Eval_fx (const ChVectorDynamic<> &x) |
void | Eval_grad (double x[], double gr[]) |
Computes the gradient of objective function, for given state of variables. More... | |
void | Eval_grad (const ChVectorDynamic<> &x, ChVectorDynamic<> &gr) |
virtual bool | PreOptimize () |
Performs the optimization of the ChSystem pointed by "database" (or whatever object which can evaluate the string "function" and the "optvarlist") using the current parameters. More... | |
virtual bool | PostOptimize () |
Finalization and cleanup. | |
virtual bool | Optimize () |
Does the three steps in sequence PreOptimize, DoOptimize, PostOptimize. More... | |
void | DoBreakCheck () |
Each break_cycles number of times this fx is called, the function break_funct() is evaluated (if any) and if positive, the variable user_break becomes true. | |
Public Attributes | |
int | popsize |
def = 100; initial population size in array "population" | |
ChGenotype ** | population |
Array of pointers to population genotypes. | |
ChGenotype * | best_indiv |
Copy of the individual with best fitness found in latest optimization. | |
int | max_generations |
max number of generations to perform | |
SelectionType | selection |
reproduction (selection) type | |
CrossoverType | crossover |
crossover type | |
MutationType | mutation |
mutation type; | |
bool | elite |
if true, best parent is always kept after crossover | |
CrossoverChangeType | crossv_change |
see codes above, if NO_CHANGE the crossover type is always the same | |
CrossoverType | crossv_changeto |
the type of new crossover if using crossv_change | |
long | crossv_changewhen |
generation number, when the "change of crossover type" takes place | |
double | mutation_prob |
probability of mutation, 0..1, default = 0.001 | |
double | crossover_prob |
crossover probability, default = 0.3; | |
bool | speciation_mating |
if true, marriage happens between similar individuals; | |
bool | incest_taboo |
if true, avoids marriage between individuals too similar | |
ReplaceMode | replacement |
replacement mode | |
double | eugenetics |
range (0..1); if 0, no eugenetics, otherwise clamp for fitness (normalized in 0..1) | |
bool | stop_by_stdeviation |
if true... (def: false) | |
double | stop_stdeviation |
stop search if stdeviation becomes lower than this value (def.0) | |
bool | stop_by_fitness |
if true... (def. false) | |
double | stop_fitness |
stop search if fitness of best individual exceed this value (def.0) | |
double | average |
the average fitness of individuals | |
double | stdeviation |
the unbiased standard deviation (=sqrt(variance)) of fitness | |
double | min_fitness |
double | max_fitness |
long | generations_done |
number of generations performed | |
long | mutants |
mutated individuals since start of optimizations | |
ChVectorDynamic * | his_average |
ChVectorDynamic * | his_stdeviation |
ChVectorDynamic * | his_maxfitness |
ChVectorDynamic * | his_minfitness |
![]() | |
bool | minimize |
default = false; just maximize | |
double | grad_step |
default = 1.e-12; step size for evaluation of gradient | |
double | opt_fx |
best resulting value of objective function | |
char | err_message [200] |
the ok/warning/error messages are written here | |
int | error_code |
long | fx_evaluations |
number of function evaluations | |
long | grad_evaluations |
number of gradient evaluations | |
int(* | break_funct )() |
if not null, this function is called each 'break_cycles' evaluations | |
int | break_cycles |
how many fx evaluations per check | |
int | user_break |
if break_funct() reported true, this flag is ON, and optimizers should exit all cycles | |
int | break_cyclecounter |
internal | |
Additional Inherited Members | |
![]() | |
ChFx * | afunction |
the function to be maximized | |
ChFx * | afunctionGrad |
the gradient of the function to be maximized, or null for default BDF. | |
int | C_vars |
number of input variables | |
double * | xv |
Vector of variables, also 1st approximation. | |
double * | xv_sup |
These are the hi/lo limits for the variables,. | |
double * | xv_inf |
these are not used by all optimizer, and can be NULL for gradient, but needed for genetic. | |
Member Function Documentation
◆ Crossover()
void chrono::ChOptimizerGenetic::Crossover | ( | ) |
if (par1->genes->Equals(par2->genes)) /cout << "\n --incest";
◆ DoOptimize()
|
overridevirtual |
This function computes the optimal xv[].
It must be implemented by derived classes
Reimplemented from chrono::ChOptimizer.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChSolvmin.h
- /builds/uwsbel/chrono/src/chrono/solver/ChSolvmin.cpp