Description
Class for referencing a set of 'glyphs', that are simple symbols such as arrows or points to be drawn for showing vector directions etc.
Remember that depending on the type of visualization system (POVray, Irrlicht,etc.) this asset might not be supported.
#include <ChGlyphs.h>
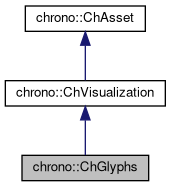
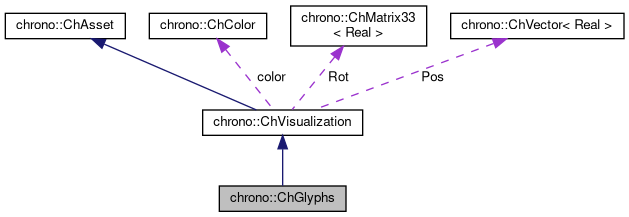
Public Types | |
enum | eCh_GlyphType { GLYPH_POINT = 0, GLYPH_VECTOR, GLYPH_COORDSYS } |
Public Member Functions | |
eCh_GlyphType | GetDrawMode () const |
Get the way that glyphs must be rendered. | |
void | SetDrawMode (eCh_GlyphType mmode) |
Set the way that glyphs must be rendered. | |
void | Reserve (unsigned int n_glyphs) |
Resize the vectors of data so that memory management is faster than calling SetGlyphPoint() etc. More... | |
size_t | GetNumberOfGlyphs () const |
Get the number of glyphs. | |
double | GetGlyphsSize () const |
Get the 'size' (thickness of symbol, depending on the rendering system) of the glyph symbols. | |
void | SetGlyphsSize (double msize) |
Set the 'size' (thickness of symbol, depending on the rendering system) of the glyph symbols. | |
void | SetZbufferHide (bool mhide) |
bool | GetZbufferHide () const |
void | SetGlyphPoint (unsigned int id, ChVector<> mpoint, ChColor mcolor=ChColor(1, 0, 0)) |
Fast method to set a glyph for GLYPH_POINT draw mode. More... | |
void | SetGlyphVector (unsigned int id, ChVector<> mpoint, ChVector<> mvector, ChColor mcolor=ChColor(1, 0, 0)) |
Fast method to set a glyph for GLYPH_VECTOR draw mode. More... | |
void | SetGlyphCoordsys (unsigned int id, ChCoordsys<> mcoord) |
Fast method to set a glyph for GLYPH_COORDSYS draw mode. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
void | SetVisible (bool mv) |
Set this visualization asset as visible. | |
bool | IsVisible () const |
Return true if the asset is set as visible. | |
void | SetColor (const ChColor &mc) |
Set the color of the surface (default: white). More... | |
const ChColor & | GetColor () const |
Return the color assigned to this asset. | |
void | SetFading (const float mc) |
Set the fading level, a value in [0,1] (default: 0). More... | |
float | GetFading () const |
Get the fading level. | |
void | SetStatic (bool val) |
Set this visualization asset as static (default: false). More... | |
bool | IsStatic () const |
Return true if the visualization asset is marked as static. | |
![]() | |
virtual void | Update (ChPhysicsItem *updater, const ChCoordsys<> &coords) |
This is called by the owner, i.e. More... | |
Public Attributes | |
std::vector< ChVector< double > > | points |
std::vector< ChColor > | colors |
std::vector< ChVector< double > > | vectors |
std::vector< ChQuaternion< double > > | rotations |
![]() | |
ChVector | Pos |
Position of Asset. | |
ChMatrix33 | Rot |
Rotation of Asset. | |
Protected Attributes | |
eCh_GlyphType | draw_mode |
double | size |
bool | zbuffer_hide |
![]() | |
bool | visible |
bool | is_static |
ChColor | color |
float | fading |
Additional Inherited Members |
Member Function Documentation
◆ Reserve()
void chrono::ChGlyphs::Reserve | ( | unsigned int | n_glyphs | ) |
Resize the vectors of data so that memory management is faster than calling SetGlyphPoint() etc.
by starting with zero size vectors that will be inflated when needed..
◆ SetGlyphCoordsys()
void chrono::ChGlyphs::SetGlyphCoordsys | ( | unsigned int | id, |
ChCoordsys<> | mcoord | ||
) |
Fast method to set a glyph for GLYPH_COORDSYS draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the csys are inflated.
◆ SetGlyphPoint()
void chrono::ChGlyphs::SetGlyphPoint | ( | unsigned int | id, |
ChVector<> | mpoint, | ||
ChColor | mcolor = ChColor(1, 0, 0) |
||
) |
Fast method to set a glyph for GLYPH_POINT draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the vectors are inflated.
◆ SetGlyphVector()
void chrono::ChGlyphs::SetGlyphVector | ( | unsigned int | id, |
ChVector<> | mpoint, | ||
ChVector<> | mvector, | ||
ChColor | mcolor = ChColor(1, 0, 0) |
||
) |
Fast method to set a glyph for GLYPH_VECTOR draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the vectors are inflated.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/assets/ChGlyphs.h
- /builds/uwsbel/chrono/src/chrono/assets/ChGlyphs.cpp