Description
template<class Ta, class Tb>
class chrono::ChContactSMC< Ta, Tb >
Class for smooth (penalty-based) contact between two generic contactable objects.
Ta and Tb are of ChContactable sub classes.
#include <ChContactSMC.h>
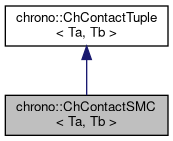

Public Types | |
typedef ChContactTuple< Ta, Tb >::typecarr_a | typecarr_a |
typedef ChContactTuple< Ta, Tb >::typecarr_b | typecarr_b |
![]() | |
typedef Ta::type_variable_tuple_carrier | typecarr_a |
typedef Tb::type_variable_tuple_carrier | typecarr_b |
Public Member Functions | |
ChContactSMC (ChContactContainer *mcontainer, Ta *mobjA, Tb *mobjB, const collision::ChCollisionInfo &cinfo) | |
virtual ChVector | GetContactForce () const override |
Get the contact force, if computed, in contact coordinate system. | |
double | GetContactPenetration () const |
Get the contact penetration (positive if there is overlap). | |
ChVector | GetContactForceAbs () const |
Get the contact force, expressed in the frame of the contact. | |
const ChKblockGeneric * | GetJacobianKRM () const |
Access the proxy to the Jacobian. | |
const ChMatrixDynamic< double > * | GetJacobianK () const |
const ChMatrixDynamic< double > * | GetJacobianR () const |
virtual void | Reset (Ta *mobjA, Tb *mobjB, const collision::ChCollisionInfo &cinfo) override |
Reinitialize this contact. More... | |
ChVector | CalculateForce (double delta, const ChVector<> &normal_dir, const ChVector<> &vel1, const ChVector<> &vel2, const ChMaterialCompositeSMC &mat) |
Calculate contact force, expressed in absolute coordinates. More... | |
void | CalculateQ (const ChState &stateA_x, const ChStateDelta &stateA_w, const ChState &stateB_x, const ChStateDelta &stateB_w, const ChMaterialCompositeSMC &mat, ChVectorDynamic<> &Q) |
Compute all forces in a contiguous array. More... | |
void | CreateJacobians () |
Create the Jacobian matrices. More... | |
void | CalculateJacobians (const ChMaterialCompositeSMC &mat) |
Calculate Jacobian of generalized contact forces. | |
virtual void | ContIntLoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Apply contact forces to the two objects. More... | |
virtual void | ContInjectKRMmatrices (ChSystemDescriptor &mdescriptor) override |
Inject Jacobian blocks into the system descriptor. More... | |
virtual void | ContKRMmatricesLoad (double Kfactor, double Rfactor) override |
Compute Jacobian of contact forces. | |
![]() | |
ChContactTuple (ChContactContainer *mcontainer, Ta *mobjA, Tb *mobjB, const collision::ChCollisionInfo &cinfo) | |
Ta * | GetObjA () |
Get the colliding object A, with point P1. | |
Tb * | GetObjB () |
Get the colliding object B, with point P2. | |
ChCoordsys | GetContactCoords () const |
Get the contact coordinate system, expressed in absolute frame. More... | |
const ChMatrix33 & | GetContactPlane () const |
Returns the pointer to a contained 3x3 matrix representing the UV and normal directions of the contact. More... | |
const ChVector & | GetContactP1 () const |
Get the contact point 1, in absolute coordinates. | |
const ChVector & | GetContactP2 () const |
Get the contact point 2, in absolute coordinates. | |
const ChVector & | GetContactNormal () const |
Get the contact normal, in absolute coordinates. | |
double | GetContactDistance () const |
Get the contact distance. | |
double | GetEffectiveCurvatureRadius () const |
Get the effective radius of curvature. | |
virtual void | ContIntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) |
virtual void | ContIntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) |
virtual void | ContIntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) |
virtual void | ContIntLoadConstraint_C (const unsigned int off_L, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) |
virtual void | ContIntToDescriptor (const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) |
virtual void | ContIntFromDescriptor (const unsigned int off_L, ChVectorDynamic<> &L) |
virtual void | InjectConstraints (ChSystemDescriptor &mdescriptor) |
virtual void | ConstraintsBiReset () |
virtual void | ConstraintsBiLoad_C (double factor=1., double recovery_clamp=0.1, bool do_clamp=false) |
virtual void | ConstraintsFetch_react (double factor) |
Additional Inherited Members | |
![]() | |
ChContactContainer * | container |
associated contact container | |
Ta * | objA |
first ChContactable object in the pair | |
Tb * | objB |
second ChContactable object in the pair | |
ChVector | p1 |
max penetration point on geo1, after refining, in abs space | |
ChVector | p2 |
max penetration point on geo2, after refining, in abs space | |
ChVector | normal |
normal, on surface of master reference (geo1) | |
ChMatrix33 | contact_plane |
the plane of contact (X is normal direction) | |
double | norm_dist |
penetration distance (negative if going inside) after refining | |
double | eff_radius |
effective radius of curvature at contact | |
Constructor & Destructor Documentation
◆ ChContactSMC()
|
inline |
- Parameters
-
mcontainer contact container mobjA collidable object A mobjB collidable object B cinfo data for the contact pair
Member Function Documentation
◆ CalculateForce()
|
inline |
Calculate contact force, expressed in absolute coordinates.
- Parameters
-
delta overlap in normal direction normal_dir normal contact direction (expressed in global frame) vel1 velocity of contact point on objA (expressed in global frame) vel2 velocity of contact point on objB (expressed in global frame) mat composite material for contact pair
◆ CalculateQ()
|
inline |
Compute all forces in a contiguous array.
Used in finite-difference Jacobian approximation.
- Parameters
-
stateA_x state positions for objA stateA_w state velocities for objA stateB_x state positions for objB stateB_w state velocities for objB mat composite material for contact pair Q output generalized forces
◆ ContInjectKRMmatrices()
|
inlineoverridevirtual |
Inject Jacobian blocks into the system descriptor.
Tell to a system descriptor that there are item(s) of type ChKblock in this object (for further passing it to a solver)
Reimplemented from chrono::ChContactTuple< Ta, Tb >.
◆ ContIntLoadResidual_F()
|
inlineoverridevirtual |
Apply contact forces to the two objects.
(new version, for interfacing to ChTimestepper and ChIntegrable)
Reimplemented from chrono::ChContactTuple< Ta, Tb >.
◆ CreateJacobians()
|
inline |
Create the Jacobian matrices.
These matrices are created/resized as needed.
◆ Reset()
|
inlineoverridevirtual |
Reinitialize this contact.
- Parameters
-
mobjA collidable object A mobjB collidable object B cinfo data for the contact pair
Reimplemented from chrono::ChContactTuple< Ta, Tb >.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono/physics/ChContactSMC.h