Description
Base class for a tire system.
A tire subsystem is a force element. It is passed position and velocity information of the wheel body and it produces ground reaction forces and moments to be applied to the wheel body.
#include <ChTire.h>
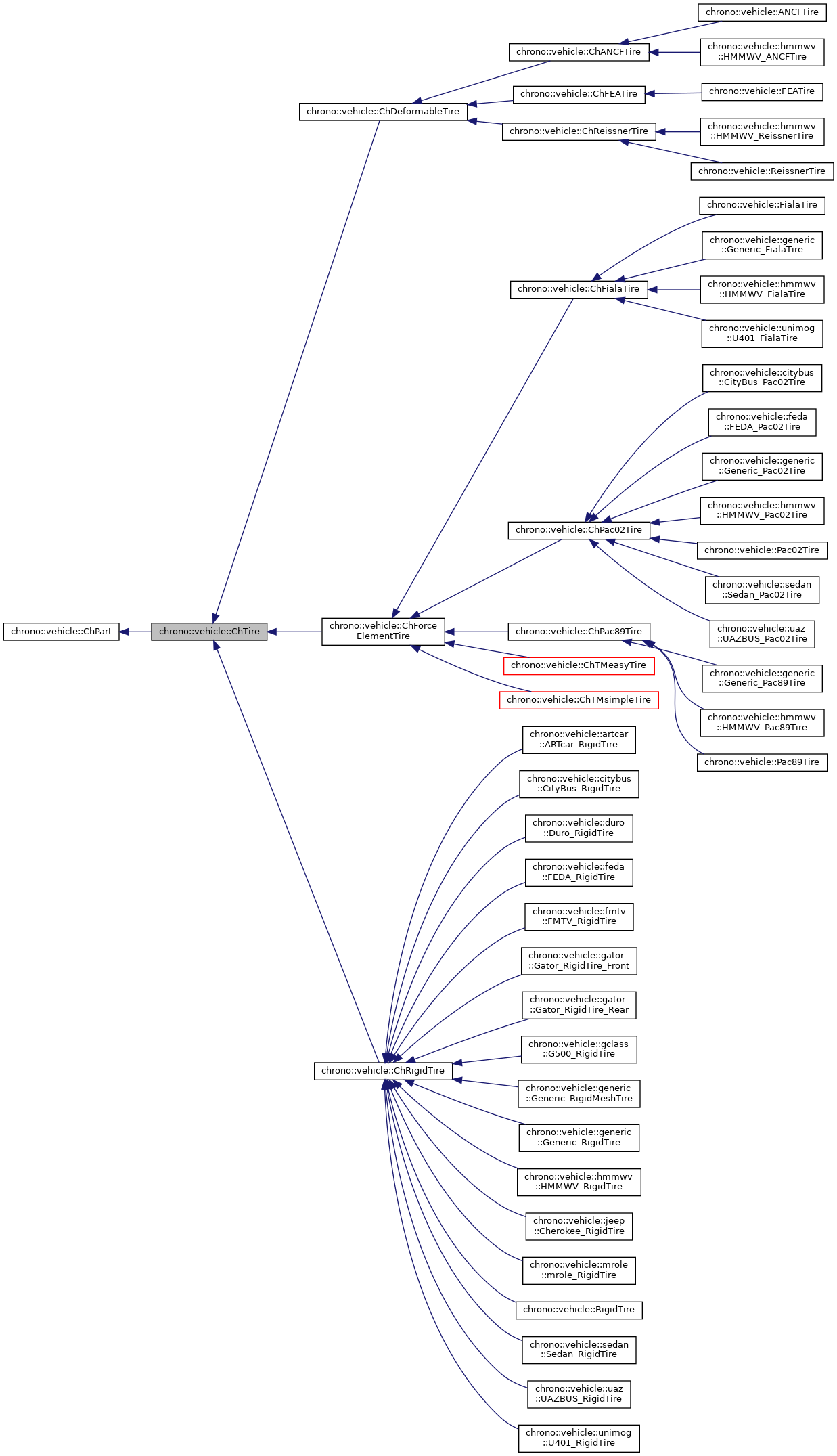
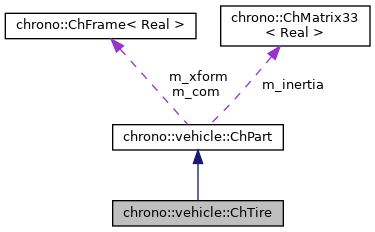
Public Types | |
enum | CollisionType { SINGLE_POINT, FOUR_POINTS, ENVELOPE } |
Public Member Functions | |
void | SetStepsize (double val) |
Set the value of the integration step size for the underlying dynamics (if applicable). More... | |
double | GetStepsize () const |
Get the current value of the integration step size. | |
void | SetCollisionType (CollisionType collision_type) |
Set the collision type for tire-terrain interaction. More... | |
void | SetPressure (double pressure) |
Set the internal tire pressure [Pa]. More... | |
double | GetPressure () const |
Get the internal tire pressure [Pa]. | |
virtual double | GetRadius () const =0 |
Get the tire radius. | |
virtual double | GetWidth () const =0 |
Get the tire width. | |
virtual double | GetTireMass () const =0 |
Return the tire mass. | |
virtual ChVector3d | GetTireInertia () const =0 |
Return the tire moments of inertia (in the tire centroidal frame). | |
virtual TerrainForce | ReportTireForce (ChTerrain *terrain) const =0 |
Report the tire force and moment. More... | |
virtual TerrainForce | ReportTireForceLocal (ChTerrain *terrain, ChCoordsys<> &tire_frame) const =0 |
Get the tire force and moment expressed in the tire frame. More... | |
double | GetSlipAngle () const |
Return the tire slip angle calculated based on the current state of the associated wheel body. More... | |
double | GetLongitudinalSlip () const |
Return the tire longitudinal slip calculated based on the current state of the associated wheel body. More... | |
double | GetCamberAngle () const |
Return the tire camber angle calculated based on the current state of the associated wheel body. More... | |
virtual double | GetDeflection () const |
Report the tire deflection. | |
const std::string & | GetMeshFilename () const |
Get the name of the Wavefront file with tire visualization mesh. More... | |
virtual void | Initialize (std::shared_ptr< ChWheel > wheel) |
Initialize this tire subsystem by associating it to an existing wheel subsystem. | |
virtual void | Synchronize (double time, const ChTerrain &terrain) |
Update the state of this tire system at the current time. More... | |
virtual void | Advance (double step) |
Advance the state of this tire by the specified time step. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Static Public Member Functions | |
static ChVector3d | EstimateInertia (double tire_width, double aspect_ratio, double rim_diameter, double tire_mass, double t_factor=2) |
Utility function for estimating the tire moments of inertia. More... | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
Protected Member Functions | |
ChTire (const std::string &name) | |
Construct a tire subsystem with given name. | |
void | CalculateKinematics (const WheelState &wheel_state, const ChCoordsys<> &tire_frame) |
Calculate kinematics quantities based on the given state of the associated wheel body. More... | |
double | GetOffset () const |
Get offset from spindle center. More... | |
virtual double | GetAddedMass () const =0 |
Get the mass added to the associated spindle body. More... | |
virtual ChVector3d | GetAddedInertia () const =0 |
Get the inertia added to the associated spindle body. More... | |
virtual TerrainForce | GetTireForce () const =0 |
Get the tire force and moment. More... | |
std::shared_ptr< ChVisualShapeTriangleMesh > | AddVisualizationMesh (const std::string &mesh_file_left, const std::string &mesh_file_right) |
Add mesh visualization to the body associated with this tire (a wheel spindle body). More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
virtual void | InitializeInertiaProperties ()=0 |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties ()=0 |
Update subsystem inertia properties. More... | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Static Protected Member Functions | |
static bool | DiscTerrainCollision (CollisionType method, const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, double width, const ChFunctionInterp &areaDep, ChCoordsys<> &contact, double &depth, float &mu) |
Perform disc-terrain collision detection, using the specified method. More... | |
static void | ConstructAreaDepthTable (double disc_radius, ChFunctionInterp &areaDep) |
Utility function to construct a loopkup table for penetration depth as function of intersection area, for a given tire radius. More... | |
static bool | DiscTerrainCollision1pt (const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, ChCoordsys<> &contact, double &depth, float &mu) |
Perform disc-terrain collision detection. More... | |
static bool | DiscTerrainCollision4pt (const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, double width, ChCoordsys<> &contact, double &depth, float &mu) |
Perform disc-terrain collision detection considering the curvature of the road surface. More... | |
static bool | DiscTerrainCollisionEnvelope (const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, double width, const ChFunctionInterp &areaDep, ChCoordsys<> &contact, double &depth, float &mu) |
Collsion algorithm based on a paper of J. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Protected Attributes | |
std::shared_ptr< ChWheel > | m_wheel |
associated wheel subsystem | |
double | m_stepsize |
tire integration step size (if applicable) | |
double | m_pressure |
internal tire pressure | |
CollisionType | m_collision_type |
method used for tire-terrain collision | |
std::string | m_vis_mesh_file |
name of OBJ file for visualization of this tire (may be empty) | |
double | m_slip_angle |
double | m_longitudinal_slip |
double | m_camber_angle |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Friends | |
class | ChWheel |
class | ChWheeledVehicle |
class | ChWheeledTrailer |
Member Function Documentation
◆ AddVisualizationMesh()
|
protected |
Add mesh visualization to the body associated with this tire (a wheel spindle body).
The two meshes are assumed to be specified with respect to a frame with origin at the center of the tire and Y axis pointing towards the outside. This function uses one of the two provided OBJ files, depending on the side on which the tire is mounted. The name of the output mesh shape is set to be the stem of the input filename.
◆ CalculateKinematics()
|
protected |
Calculate kinematics quantities based on the given state of the associated wheel body.
- Parameters
-
[in] wheel_state current state of associated wheel body [in] tire_frame tire contact frame
◆ ConstructAreaDepthTable()
|
staticprotected |
Utility function to construct a loopkup table for penetration depth as function of intersection area, for a given tire radius.
The return map can be used in DiscTerrainCollisionEnvelope.
◆ DiscTerrainCollision()
|
staticprotected |
Perform disc-terrain collision detection, using the specified method.
- Parameters
-
[in] method tire-terrain collision detection method [in] terrain reference to terrain system [in] disc_center global location of the disc center [in] disc_normal disc normal, expressed in the global frame [in] disc_radius disc radius [in] width tire width [in] areaDep lookup table to calculate depth from intersection area [out] contact contact coordinate system (relative to the global frame) [out] depth penetration depth (positive if contact occurred) [out] mu coefficient of friction at contact
◆ DiscTerrainCollision1pt()
|
staticprotected |
Perform disc-terrain collision detection.
This utility function checks for contact between a disc of specified radius with given position and orientation (specified as the location of its center and a unit vector normal to the disc plane) and the terrain system associated with this tire. It returns true if the disc contacts the terrain and false otherwise. If contact occurs, it returns a coordinate system with the Z axis along the contact normal and the X axis along the "rolling" direction, as well as a positive penetration depth (i.e. the height below the terrain of the lowest point on the disc).
- Parameters
-
[in] terrain reference to terrain system [in] disc_center global location of the disc center [in] disc_normal disc normal, expressed in the global frame [in] disc_radius disc radius [out] contact contact coordinate system (relative to the global frame) [out] depth penetration depth (positive if contact occurred) [out] mu coefficient of friction at contact
◆ DiscTerrainCollision4pt()
|
staticprotected |
Perform disc-terrain collision detection considering the curvature of the road surface.
The surface normal is calculated based on 4 different height values below the wheel center. The effective height is calculated as average value of the four height values. This utility function checks for contact between a disc of specified radius with given position and orientation (specified as the location of its center and a unit vector normal to the disc plane) and the terrain system associated with this tire. It returns true if the disc contacts the terrain and false otherwise. If contact occurs, it returns a coordinate system with the Z axis along the contact normal and the X axis along the "rolling" direction, as well as a positive penetration depth (i.e. the height below the terrain of the lowest point on the disc).
- Parameters
-
[in] terrain reference to terrain system [in] disc_center global location of the disc center [in] disc_normal disc normal, expressed in the global frame [in] disc_radius disc radius [in] width tire width [out] contact contact coordinate system (relative to the global frame) [out] depth penetration depth (positive if contact occurred) [out] mu coefficient of friction at contact
◆ DiscTerrainCollisionEnvelope()
|
staticprotected |
Collsion algorithm based on a paper of J.
Shane Sui and John A. Hirshey II: "A New Analytical Tire Model for Vehicle Dynamic Analysis" presented at 2001 MSC User Meeting
- Parameters
-
[in] terrain reference to terrain system [in] disc_center global location of the disc center [in] disc_normal disc normal, expressed in the global frame [in] disc_radius disc radius [in] width tire width [in] areaDep lookup table to calculate depth from intersection area [out] contact contact coordinate system (relative to the global frame) [out] depth penetration depth (positive if contact occurred) [out] mu coefficient of friction at contact
◆ EstimateInertia()
|
static |
Utility function for estimating the tire moments of inertia.
The tire is assumed to be specified with the common scheme (e.g. 215/65R15) and the mass of the tire (excluding the wheel) provided.
- Parameters
-
tire_width tire width [mm] aspect_ratio aspect ratio: height to width [percentage] rim_diameter rim diameter [in] tire_mass mass of the tire [kg] t_factor tread to sidewall thickness factor
◆ GetAddedInertia()
|
protectedpure virtual |
Get the inertia added to the associated spindle body.
Certain tires (e.g., those FEA-based) have their own physical representation and hence do not add mass and inertia to the spindle body. All others increment the spindle body moments of inertia by the amount reported by this function.
Implemented in chrono::vehicle::ChDeformableTire, and chrono::vehicle::ChRigidTire.
◆ GetAddedMass()
|
protectedpure virtual |
Get the mass added to the associated spindle body.
Certain tires (e.g., those FEA-based) have their own physical representation and hence do not add mass and inertia to the spindle body. All others increment the spindle body mass by the amount repoirted by this function.
Implemented in chrono::vehicle::ChDeformableTire, and chrono::vehicle::ChRigidTire.
◆ GetCamberAngle()
|
inline |
Return the tire camber angle calculated based on the current state of the associated wheel body.
Note that this is a "dynamic" or "effective" camber angle, defined as the angle of the wheel from a plane normal to the current tire contact patch. The return value is in radians. A positive value corresponds to the upper side tipping to the left, while a negative value indicates the upper side tipping to the right.
◆ GetLongitudinalSlip()
|
inline |
Return the tire longitudinal slip calculated based on the current state of the associated wheel body.
A positive value corresponds to a driving wheel, while a negative sign indicates braking.
◆ GetMeshFilename()
|
inline |
Get the name of the Wavefront file with tire visualization mesh.
An empty string is returned if no mesh was specified.
◆ GetOffset()
|
inlineprotected |
Get offset from spindle center.
This queries the associated wheel, so it must be called only after the wheel was initialized.
◆ GetSlipAngle()
|
inline |
Return the tire slip angle calculated based on the current state of the associated wheel body.
The return value is in radians with a positive sign for a left turn and a negative sign for a right turn.
◆ GetTireForce()
|
protectedpure virtual |
Get the tire force and moment.
This represents the output from this tire system that is passed to the vehicle system. The return application point, force, and moment are assumed to be expressed in the global reference frame. Typically, the vehicle subsystem will pass the tire force to the appropriate suspension subsystem which applies it as an external force on the wheel body. NOTE: tire models that rely on underlying Chrono functionality (e.g., the Chrono contact system or Chrono constraints) must always return zero forces and moments, else tire forces are double counted.
Implemented in chrono::vehicle::ChDeformableTire, chrono::vehicle::ChRigidTire, and chrono::vehicle::ChForceElementTire.
◆ ReportTireForce()
|
pure virtual |
Report the tire force and moment.
This function can be used for reporting purposes or else to calculate tire forces in a co-simulation framework. The return application point, force, and moment are assumed to be expressed in the global reference frame.
Implemented in chrono::vehicle::ChDeformableTire, chrono::vehicle::ChRigidTire, and chrono::vehicle::ChForceElementTire.
◆ ReportTireForceLocal()
|
pure virtual |
Get the tire force and moment expressed in the tire frame.
The tire frame has its origin in the contact patch, the X axis in the tire heading direction and the Z axis in the terrain normal at the contact point. If the tire is not in contact, the tire frame is not set and the function returns zero force and moment.
Implemented in chrono::vehicle::ChDeformableTire, chrono::vehicle::ChRigidTire, and chrono::vehicle::ChForceElementTire.
◆ SetCollisionType()
|
inline |
Set the collision type for tire-terrain interaction.
Default: SINGLE_POINT
◆ SetPressure()
|
inline |
Set the internal tire pressure [Pa].
Default value: 0. Derived classes (concrete tire models) may or may not use this setting.
◆ SetStepsize()
|
inline |
Set the value of the integration step size for the underlying dynamics (if applicable).
Default value: 1ms.
◆ Synchronize()
|
inlinevirtual |
Update the state of this tire system at the current time.
A derived class should also set the current slip, longitudinal slip, and camber angle.
Reimplemented in chrono::vehicle::ChPac02Tire, chrono::vehicle::ChTMeasyTire, chrono::vehicle::ChTMsimpleTire, chrono::vehicle::ChPac89Tire, chrono::vehicle::ChRigidTire, and chrono::vehicle::ChFialaTire.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChTire.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChTire.cpp