Description
Base class for a powertrain system.
#include <ChPowertrain.h>
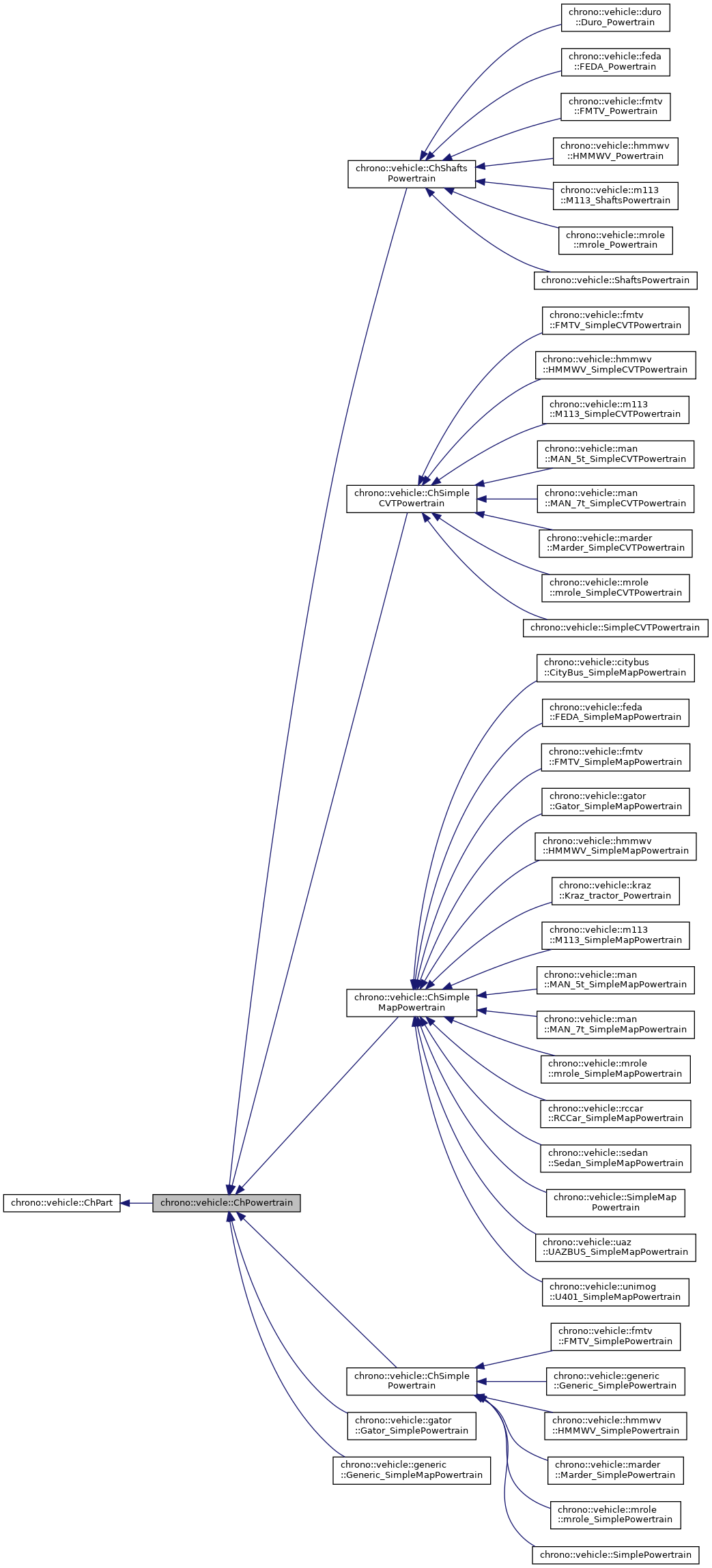
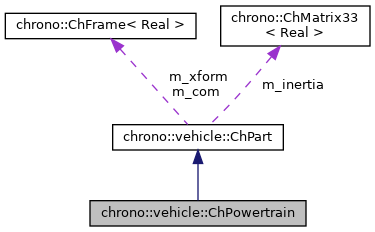
Public Types | |
enum | DriveMode { DriveMode::FORWARD, DriveMode::NEUTRAL, DriveMode::REVERSE } |
Driving modes. More... | |
enum | TransmissionMode { TransmissionMode::AUTOMATIC, TransmissionMode::MANUAL } |
Transmission mode. More... | |
Public Member Functions | |
virtual double | GetMotorSpeed () const =0 |
Return the current engine speed. | |
virtual double | GetMotorTorque () const =0 |
Return the current engine torque. | |
virtual double | GetTorqueConverterSlippage () const =0 |
Return the value of slippage in the torque converter. | |
virtual double | GetTorqueConverterInputTorque () const =0 |
Return the input torque to the torque converter. | |
virtual double | GetTorqueConverterOutputTorque () const =0 |
Return the output torque from the torque converter. | |
virtual double | GetTorqueConverterOutputSpeed () const =0 |
Return the torque converter output shaft speed. | |
int | GetCurrentTransmissionGear () const |
Return the current transmission gear. More... | |
virtual double | GetOutputTorque () const =0 |
Return the output torque from the powertrain. More... | |
void | SetDriveMode (DriveMode mode) |
Set the drive mode. | |
DriveMode | GetDriveMode () const |
Return the current drive mode. | |
void | SetTransmissionMode (TransmissionMode mode) |
Set the transmission mode (automatic or manual). More... | |
TransmissionMode | GetTransmissionMode () const |
Get the current transmission mode. | |
void | ShiftUp () |
Shift up. | |
void | ShiftDown () |
Shift down. | |
void | SetGear (int gear) |
Shift to the specified gear. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Protected Member Functions | |
ChPowertrain (const std::string &name) | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis) |
Initialize this powertrain system by attaching it to an existing vehicle chassis. More... | |
virtual void | SetGearRatios (std::vector< double > &fwd, double &rev)=0 |
Set the transmission gear ratios (one or more forward gear ratios and a single reverse gear ratio). | |
virtual void | OnGearShift () |
Perform any action required on a gear shift (the new gear and gear ratio are available). | |
virtual void | OnNeutralShift () |
Perform any action required on placing the transmission in neutral. | |
virtual void | Synchronize (double time, const DriverInputs &driver_inputs, double shaft_speed)=0 |
Synchronize the state of this powertrain system at the current time. More... | |
virtual void | Advance (double step) |
Advance the state of this powertrain system by the specified time step. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector<> &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
TransmissionMode | m_transmission_mode |
transmission mode (automatic or manual) | |
DriveMode | m_drive_mode |
drive mode (neutral, forward, or reverse) | |
std::shared_ptr< ChDriveline > | m_driveline |
associated driveline subsystem | |
std::vector< double > | m_gear_ratios |
gear ratios (0: reverse, 1+: forward) | |
int | m_current_gear |
current transmission gear (0: reverse, 1+: forward) | |
double | m_current_gear_ratio |
current gear ratio (positive for forward, negative for reverse) | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Friends | |
class | ChWheeledVehicle |
class | ChTrackedVehicle |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Enumeration Documentation
◆ DriveMode
|
strong |
◆ TransmissionMode
|
strong |
Member Function Documentation
◆ GetCurrentTransmissionGear()
|
inline |
Return the current transmission gear.
A return value of 0 indicates reverse; a positive value indicates a forward gear.
◆ GetOutputTorque()
|
pure virtual |
Return the output torque from the powertrain.
This is the torque that is passed to a vehicle system, thus providing the interface between the powertrain and vehicle co-simulation modules.
Implemented in chrono::vehicle::ChShaftsPowertrain, chrono::vehicle::ChSimpleMapPowertrain, chrono::vehicle::generic::Generic_SimpleMapPowertrain, chrono::vehicle::ChSimpleCVTPowertrain, chrono::vehicle::ChSimplePowertrain, and chrono::vehicle::gator::Gator_SimplePowertrain.
◆ Initialize()
|
protectedvirtual |
Initialize this powertrain system by attaching it to an existing vehicle chassis.
A derived class override must first call this base class version.
◆ InitializeInertiaProperties()
|
overrideprotectedvirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ SetGear()
void chrono::vehicle::ChPowertrain::SetGear | ( | int | gear | ) |
Shift to the specified gear.
Note that reverse gear is index 0 and forward gears are index > 0.
◆ SetTransmissionMode()
|
inline |
Set the transmission mode (automatic or manual).
Note that a derived powertrain class may ignore this is the selected mode is not supported.
◆ Synchronize()
|
protectedpure virtual |
Synchronize the state of this powertrain system at the current time.
The powertrain system is provided the current driver throttle input, a value in the range [0,1].
- Parameters
-
[in] time current time [in] driver_inputs current driver inputs [in] shaft_speed driveshaft speed
◆ UpdateInertiaProperties()
|
overrideprotectedvirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/ChPowertrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/ChPowertrain.cpp